The Art of Writing Helper Functions During Interviews
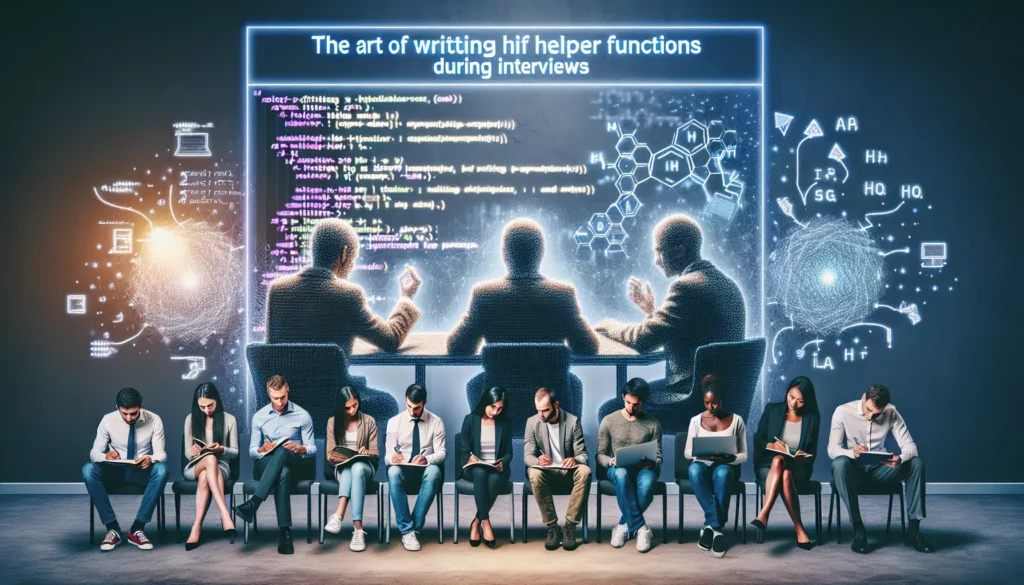
When it comes to technical interviews, especially those at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering the art of writing helper functions can be a game-changer. This skill not only demonstrates your ability to break down complex problems into manageable pieces but also showcases your understanding of clean code principles and modular design. In this comprehensive guide, we’ll explore the importance of helper functions, strategies for creating them effectively, and how they can elevate your performance during coding interviews.
Why Helper Functions Matter in Interviews
Before diving into the specifics of writing helper functions, it’s crucial to understand why they’re so valuable in the context of technical interviews:
- Improved Readability: Helper functions make your main algorithm easier to understand by abstracting away complex operations.
- Modularity: They allow you to break down a large problem into smaller, more manageable pieces.
- Reusability: Well-designed helper functions can be reused in different parts of your solution or even in other problems.
- Easier Debugging: Isolating functionality in helper functions makes it easier to identify and fix issues.
- Demonstrating Skills: Using helper functions effectively shows interviewers that you can write clean, organized code.
Strategies for Writing Effective Helper Functions
1. Identify Repetitive or Complex Operations
The first step in creating helper functions is recognizing when they’re needed. Look for:
- Code that you’re writing multiple times
- Operations that are complex and can be abstracted
- Sections of code that perform a distinct, nameable task
For example, if you’re working on a problem involving binary trees, you might create helper functions for tasks like finding the height of a tree or checking if a tree is balanced.
2. Choose Descriptive Names
Naming is crucial in programming, and it’s even more important during interviews. Choose names that clearly describe what the function does. This makes your code self-documenting and easier for the interviewer to follow.
// Instead of this:
function h(n) {
// ...
}
// Do this:
function calculateTreeHeight(node) {
// ...
}
3. Keep Functions Focused
Each helper function should do one thing and do it well. This principle, known as the Single Responsibility Principle, makes your code more maintainable and easier to understand.
// Instead of this:
function processAndValidateInput(input) {
// Validate input
// Process input
// Return result
}
// Do this:
function validateInput(input) {
// Validation logic
}
function processInput(input) {
// Processing logic
}
4. Use Clear Parameter Names
Just like function names, parameter names should be descriptive. This helps anyone reading your code understand what the function expects without having to dive into its implementation.
// Instead of this:
function find(a, b) {
// ...
}
// Do this:
function findElementInArray(element, array) {
// ...
}
5. Consider Return Values Carefully
Think about what your helper function should return. Sometimes it might modify a data structure in place, other times it might return a new value. Be consistent and make sure the return value is useful for the calling function.
function reverseLinkedList(head) {
if (!head || !head.next) return head;
let prev = null;
let current = head;
while (current) {
let next = current.next;
current.next = prev;
prev = current;
current = next;
}
return prev; // Return the new head of the reversed list
}
6. Handle Edge Cases
Demonstrate your attention to detail by handling edge cases within your helper functions. This might include checking for null inputs, empty arrays, or other special conditions.
function findMinimum(array) {
if (!array || array.length === 0) {
throw new Error("Array is empty or null");
}
let min = array[0];
for (let i = 1; i < array.length; i++) {
if (array[i] < min) {
min = array[i];
}
}
return min;
}
Practical Examples of Helper Functions in Common Interview Problems
Let’s look at some practical examples of how helper functions can be used in typical interview problems:
Example 1: Binary Tree Traversal
When working with binary trees, helper functions can greatly simplify your code. Here’s an example of in-order traversal using a helper function:
function inorderTraversal(root) {
const result = [];
function traverse(node) {
if (node) {
traverse(node.left);
result.push(node.val);
traverse(node.right);
}
}
traverse(root);
return result;
}
In this example, the traverse
helper function encapsulates the recursive logic of the in-order traversal, making the main function cleaner and easier to understand.
Example 2: Graph DFS
When implementing depth-first search on a graph, helper functions can manage the visited set and recursive calls:
function dfs(graph) {
const visited = new Set();
const result = [];
function explore(vertex) {
if (visited.has(vertex)) return;
visited.add(vertex);
result.push(vertex);
for (let neighbor of graph[vertex]) {
explore(neighbor);
}
}
for (let vertex in graph) {
explore(vertex);
}
return result;
}
The explore
helper function handles the recursive exploration of the graph, keeping the main dfs
function clean and focused on initializing the search.
Example 3: Dynamic Programming
In dynamic programming problems, helper functions can manage memoization or handle complex state transitions. Here’s an example for the coin change problem:
function coinChange(coins, amount) {
const memo = new Array(amount + 1).fill(-1);
function dp(remainingAmount) {
if (remainingAmount === 0) return 0;
if (remainingAmount < 0) return Infinity;
if (memo[remainingAmount] !== -1) return memo[remainingAmount];
let minCoins = Infinity;
for (let coin of coins) {
minCoins = Math.min(minCoins, 1 + dp(remainingAmount - coin));
}
memo[remainingAmount] = minCoins;
return minCoins;
}
const result = dp(amount);
return result === Infinity ? -1 : result;
}
The dp
helper function encapsulates the recursive logic with memoization, making the solution more modular and easier to reason about.
Best Practices for Using Helper Functions in Interviews
Now that we’ve seen some examples, let’s discuss best practices for using helper functions during coding interviews:
1. Explain Your Approach
Before you start coding, explain to the interviewer that you plan to use helper functions and why. This demonstrates your thought process and problem-solving approach.
2. Start with the Main Function
Begin by writing the skeleton of your main function, then identify where helper functions would be beneficial. This shows that you can see the big picture before diving into details.
3. Use Helper Functions to Manage Complexity
If you find yourself writing nested loops or complex conditional logic, consider whether a helper function could simplify the code.
4. Be Prepared to Explain Your Helper Functions
The interviewer may ask you to explain the purpose and implementation of your helper functions. Be ready to discuss your design decisions.
5. Balance Between Helpers and Inline Code
While helper functions are valuable, don’t overuse them. Sometimes, a simple inline operation is clearer than extracting it to a separate function.
6. Consider Performance Implications
Be aware of any performance trade-offs when using helper functions, such as additional function calls. In most cases, the clarity they provide outweighs minor performance costs, but it’s good to be cognizant of this.
Common Pitfalls to Avoid
While helper functions can greatly improve your code, there are some pitfalls to watch out for:
1. Overcomplication
Don’t create helper functions for very simple operations that are clear when written inline. This can actually make your code harder to read.
2. Unclear Naming
Avoid vague names like helper
or util
. Always strive for clarity in your function names.
3. Too Many Parameters
If your helper function requires many parameters, it might be a sign that it’s doing too much or that you need to rethink your approach.
4. Ignoring Return Values
Make sure you’re using the return values of your helper functions appropriately. Ignoring them can lead to bugs and confusion.
5. Hidden Side Effects
Be cautious of helper functions that modify global state or have side effects that aren’t immediately apparent from their name or signature.
Advanced Techniques with Helper Functions
As you become more comfortable with helper functions, consider these advanced techniques:
1. Closures for State Management
Use closures to create helper functions that maintain state between calls without relying on global variables:
function createCounter() {
let count = 0;
return function() {
return ++count;
};
}
const counter = createCounter();
console.log(counter()); // 1
console.log(counter()); // 2
2. Higher-Order Functions
Create helper functions that take other functions as arguments or return functions. This can lead to highly reusable and flexible code:
function memoize(fn) {
const cache = new Map();
return function(...args) {
const key = JSON.stringify(args);
if (cache.has(key)) {
return cache.get(key);
}
const result = fn.apply(this, args);
cache.set(key, result);
return result;
};
}
const memoizedFactorial = memoize(function factorial(n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
});
3. Generator Functions
Use generator functions as helpers to create iterables or to manage complex state machines:
function* fibonacciGenerator() {
let a = 0, b = 1;
while (true) {
yield a;
[a, b] = [b, a + b];
}
}
const fib = fibonacciGenerator();
console.log(fib.next().value); // 0
console.log(fib.next().value); // 1
console.log(fib.next().value); // 1
console.log(fib.next().value); // 2
Conclusion
Mastering the art of writing helper functions is a valuable skill that can significantly improve your performance in coding interviews, especially for positions at top tech companies. By breaking down complex problems, improving code readability, and demonstrating your ability to write modular and reusable code, you’ll stand out as a strong candidate.
Remember, the key is to use helper functions judiciously, with clear naming and focused functionality. Practice incorporating these techniques into your problem-solving approach, and you’ll find yourself writing cleaner, more efficient code during interviews and in your day-to-day programming tasks.
As you continue to develop your skills, platforms like AlgoCademy can provide valuable resources and practice problems to hone your ability to write effective helper functions and tackle complex algorithmic challenges. Keep practicing, stay curious, and always look for opportunities to refine your code through smart use of helper functions.