The Art of Writing Code Comments That Are Actually Passive-Aggressive Remarks
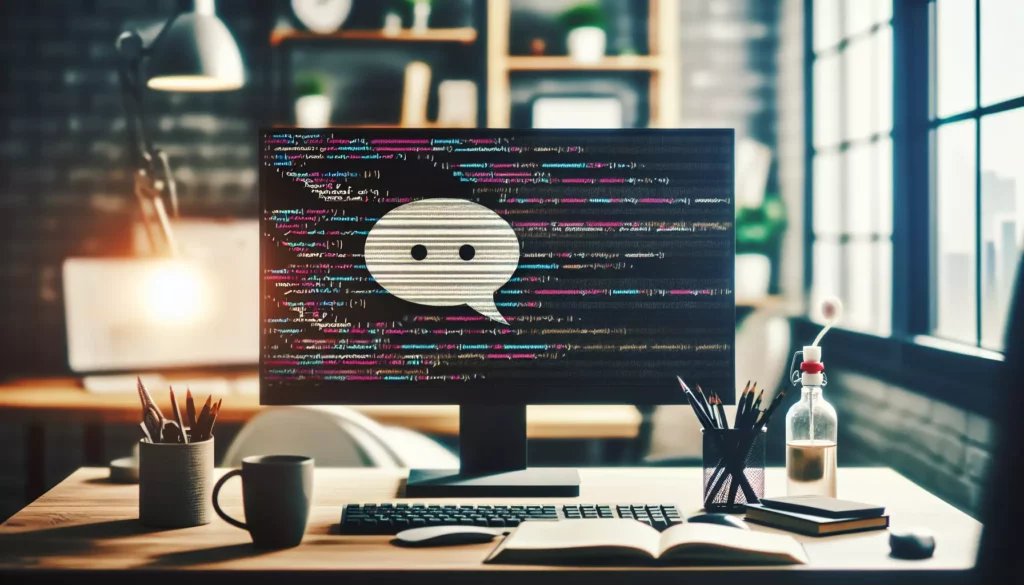
In the world of programming, comments are meant to be helpful explanations that guide developers through complex code. However, what if we could turn this noble practice into an opportunity for subtle jabs and witty retorts? Welcome to the unconventional guide on crafting code comments that double as passive-aggressive remarks. While we at AlgoCademy always advocate for clear, constructive, and professional communication in coding, sometimes a little humor can lighten the mood in the development process.
The Importance of Comments in Code
Before we dive into the realm of passive-aggressive commenting, let’s remind ourselves why comments are crucial in programming:
- They explain complex logic
- They provide context for future developers
- They can serve as temporary documentation
- They help in debugging and maintenance
Now, let’s explore how we can subvert these noble intentions for our amusement (but remember, this is all in good fun – in real-world scenarios, always strive for clear and helpful comments!).
1. The “I Can’t Believe I Have to Explain This” Comment
This type of comment expresses disbelief at having to explain something that the author considers blatantly obvious.
// For those who skipped kindergarten, this loop counts from 1 to 10
for (int i = 1; i <= 10; i++) {
System.out.println(i);
}
While this comment might elicit a chuckle, it’s important to remember that what’s obvious to one developer might not be to another, especially those new to programming or the specific codebase.
2. The “I’m Not Angry, Just Disappointed” Comment
This comment style conveys a sense of resignation and disappointment, often directed at past decisions or code quality.
// I'm sure there was a good reason for using a global variable here.
// I just can't think of one right now. Or ever.
global_var = some_function();
While it’s tempting to criticize past decisions, it’s more constructive to focus on improving the code rather than casting blame.
3. The “Sarcastic Explanation” Comment
Here, the comment provides an explanation dripping with sarcasm, often highlighting perceived flaws in the code or design decisions.
// Ah yes, nothing says "optimized code" like nested loops with O(n^3) complexity
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
for (int k = 0; k < n; k++) {
// Some incredibly important operation, I'm sure
}
}
}
While this comment humorously points out a potential performance issue, a more constructive approach would be to suggest optimizations or explain why this complexity is necessary (if it is).
4. The “Passive-Aggressive TODO” Comment
TODO comments are common in code, but they can be turned into subtle digs at team members or future maintainers.
// TODO: Refactor this mess. Good luck to whoever draws the short straw.
function someComplexFunction() {
// 200 lines of spaghetti code
}
Instead of leaving such discouraging comments, it’s better to create detailed, actionable TODO items that explain what needs to be done and why.
5. The “Overly Detailed for No Reason” Comment
This type of comment provides an absurdly detailed explanation for a simple operation, implying that the reader might not understand even the most basic concepts.
// The following line increments the variable "counter" by exactly one unit.
// This is achieved through the use of the increment operator, represented by
// two plus signs (++). The operation is performed post-line execution, ensuring
// that the current value of "counter" is used in any expressions on this line
// before the increment takes effect. The new value will be reflected in subsequent
// uses of the "counter" variable. This is a fundamental operation in computer
// programming and is used extensively in loop constructs and other scenarios
// where sequential counting is required.
counter++;
While thorough explanations can be helpful, overdoing it for simple operations can come across as condescending. It’s important to strike a balance and provide detailed comments only where they add value.
6. The “Blame-Shifting” Comment
This comment attempts to preemptively shift blame for any issues that might arise from the code.
// I was told to do it this way. If it breaks, talk to management.
function riskyOperation() {
// Potentially problematic code
}
Instead of deflecting responsibility, it’s more professional to document the reasons behind certain decisions and any known risks or limitations.
7. The “Passive-Aggressive Performance Prediction” Comment
This type of comment sarcastically predicts the performance implications of the code.
// I'm sure this recursive function will work great with large datasets.
// Who needs stack space anyway?
function recursiveNightmare(data) {
if (data.length === 0) return;
// Process data
recursiveNightmare(data.slice(1));
}
While it’s important to be aware of performance implications, a more constructive approach would be to suggest alternatives or explain why this approach was chosen despite potential drawbacks.
8. The “Unnecessarily Cryptic” Comment
This comment style uses overly complex language or obscure references to explain simple concepts, potentially confusing readers more than helping them.
// Behold, the Schrödinger's Cat of boolean operations
boolean isAlive = quantumState && observerPresent;
While clever comments can be entertaining, they should not come at the cost of clarity. The primary goal of comments is to aid understanding, not to showcase the author’s wit.
9. The “Historical Reenactment” Comment
This type of comment narrates the code’s history in a dramatic, often exaggerated manner.
// In the dark days of Sprint 34, this function was born.
// Forged in the fires of Mount Deadline, it has survived
// countless refactors and outlived many of its creator's brain cells.
function ancientLegacyCode() {
// Code that somehow still works
}
While it can be amusing to recount a piece of code’s history, it’s more helpful to focus on its current purpose and any ongoing maintenance considerations.
10. The “Passive-Aggressive Optimization Suggestion” Comment
This comment style sarcastically suggests optimizations, often for trivial operations.
// Maybe we should use a quantum computer to calculate this sum more efficiently
int sum = a + b;
Instead of making sarcastic suggestions, it’s better to provide genuine optimization tips where they’re actually needed and can make a significant difference.
The Fine Line Between Humor and Unprofessionalism
While these examples might provide a chuckle, it’s crucial to remember that in professional settings, code comments should be clear, helpful, and respectful. The line between a harmless joke and unprofessional conduct can be thin, and what seems funny to one person might be offensive or discouraging to another.
At AlgoCademy, we emphasize the importance of writing clean, well-documented code. Here are some guidelines for writing effective, professional comments:
- Be clear and concise
- Explain the “why” behind complex logic
- Keep comments up-to-date with code changes
- Use comments to provide context, not to restate the obvious
- Be respectful and assume good intentions from other developers
The Role of Comments in Learning and Interview Preparation
For those using AlgoCademy to prepare for coding interviews or improve their programming skills, understanding how to write effective comments is crucial. Many technical interviews include code review segments where you might be asked to explain your code or improve existing documentation.
Well-written comments can:
- Demonstrate your ability to communicate complex ideas clearly
- Show your consideration for other developers who might work with your code
- Highlight your understanding of best practices in software development
- Prove your ability to think about long-term code maintenance
When practicing coding problems on AlgoCademy or preparing for interviews, make it a habit to include meaningful comments in your solutions. This will not only help you in interview scenarios but also develop good habits for your professional career.
Conclusion: The True Art of Commenting
While we’ve had some fun exploring the world of passive-aggressive commenting, the true art lies in writing comments that genuinely enhance code understanding and maintainability. As you continue your coding journey with AlgoCademy, remember that clear, concise, and helpful comments are an essential part of good coding practices.
Whether you’re working on algorithmic challenges, preparing for technical interviews, or collaborating on projects, your ability to communicate effectively through code and comments will set you apart as a developer. Strive to write comments that future you (and other developers) will appreciate – comments that illuminate rather than obscure, guide rather than confuse, and support rather than undermine.
Happy coding, and may your comments always be helpful, your code readable, and your debugging sessions short!