The Art of Using Programming Paradigms to Understand Your Family Dynamics
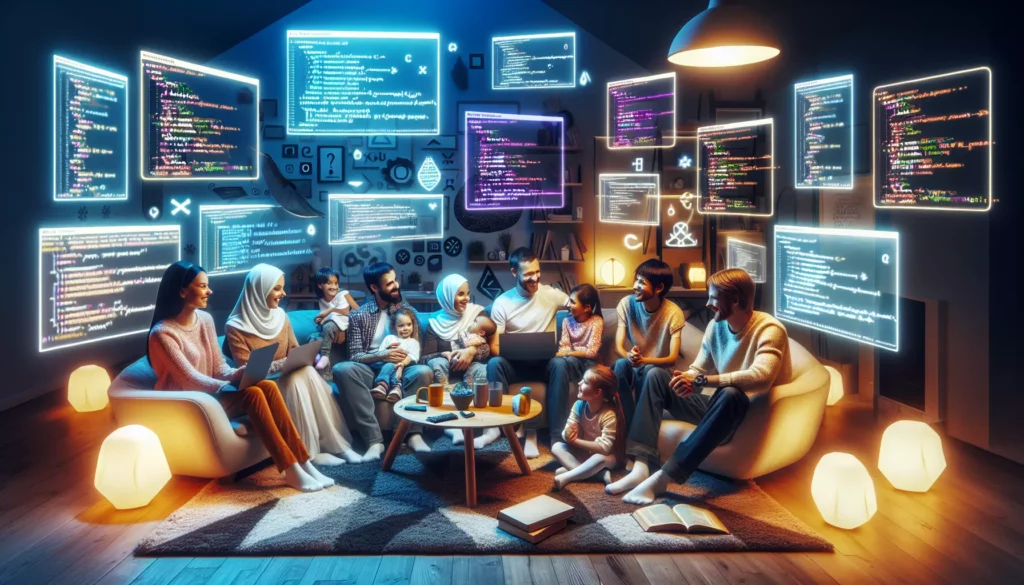
In the world of coding, we often encounter various programming paradigms that shape how we approach problem-solving and structure our code. But what if we told you that these same paradigms could be applied to understand and navigate the complex world of family dynamics? In this unique exploration, we’ll dive into how the principles of object-oriented programming, functional programming, and other coding concepts can provide fresh insights into our familial relationships.
1. Object-Oriented Programming: Modeling Family Members as Objects
Object-Oriented Programming (OOP) is a programming paradigm that organizes code into objects, which are instances of classes. Each object has its own properties and methods. Let’s see how we can apply this concept to family dynamics:
1.1 Classes and Inheritance
In OOP, we can create a base class called “FamilyMember” with common attributes and behaviors. Then, we can create subclasses for specific roles like “Parent,” “Child,” or “Sibling.” This hierarchy mirrors the structure of many families:
class FamilyMember {
constructor(name, age) {
this.name = name;
this.age = age;
}
introduce() {
console.log(`Hi, I'm ${this.name} and I'm ${this.age} years old.`);
}
}
class Parent extends FamilyMember {
constructor(name, age, children) {
super(name, age);
this.children = children;
}
nurture() {
console.log(`${this.name} is taking care of their children.`);
}
}
class Child extends FamilyMember {
constructor(name, age, parents) {
super(name, age);
this.parents = parents;
}
learn() {
console.log(`${this.name} is learning from their parents.`);
}
}
This structure helps us understand how different family members have unique roles and responsibilities while sharing common traits.
1.2 Encapsulation: Privacy and Boundaries
Encapsulation in OOP involves hiding internal details and providing a public interface. In family dynamics, this concept can represent personal boundaries and privacy:
class FamilyMember {
#privateThoughts = [];
constructor(name) {
this.name = name;
}
addPrivateThought(thought) {
this.#privateThoughts.push(thought);
}
shareThought() {
return this.#privateThoughts[Math.floor(Math.random() * this.#privateThoughts.length)];
}
}
This example shows how family members can have private thoughts (encapsulated within the object) while choosing what to share with others.
1.3 Polymorphism: Adapting to Different Roles
Polymorphism allows objects to take on multiple forms. In families, this can represent how individuals adapt to different roles:
class FamilyMember {
communicate() {
console.log("Communicating...");
}
}
class Parent extends FamilyMember {
communicate() {
console.log("Giving advice and guidance");
}
}
class Child extends FamilyMember {
communicate() {
console.log("Expressing needs and learning");
}
}
function familyDiscussion(members) {
members.forEach(member => member.communicate());
}
const family = [new Parent("Mom"), new Child("Son"), new Parent("Dad")];
familyDiscussion(family);
This demonstrates how family members can have different ways of communicating based on their roles, yet still participate in family discussions.
2. Functional Programming: Pure Functions and Immutability in Family Interactions
Functional programming emphasizes the use of pure functions and immutable data. Let’s explore how these concepts can apply to family dynamics:
2.1 Pure Functions: Predictable Interactions
Pure functions always produce the same output for the same input, without side effects. In family dynamics, this could represent consistent, reliable behaviors:
const greet = (name) => `Hello, ${name}!`;
const calculateAllowance = (age, behavior) => age * (behavior === 'good' ? 2 : 1);
console.log(greet("Mom")); // Always "Hello, Mom!"
console.log(calculateAllowance(10, 'good')); // Always 20
These functions represent predictable family interactions, where greetings and rewards are consistent based on input.
2.2 Immutability: Respecting Personal Growth
Immutability in functional programming means that once data is created, it cannot be changed. In family dynamics, this can represent respecting each member’s personal growth and decisions:
const familyMember = {
name: "Alice",
age: 25,
interests: ["reading", "cooking"]
};
const updatedMember = {
...familyMember,
age: 26,
interests: [...familyMember.interests, "painting"]
};
console.log(familyMember); // Original object unchanged
console.log(updatedMember); // New object with updates
This approach shows how we can acknowledge changes in family members without trying to forcibly change them, instead creating new “versions” that incorporate growth and new interests.
2.3 Higher-Order Functions: Family Traditions and Rituals
Higher-order functions can take functions as arguments or return them. This concept can be used to model family traditions and rituals:
const createFamilyTradition = (activity) => {
return (family) => {
console.log(`The ${family} family is ${activity} together.`);
};
};
const holidayDinner = createFamilyTradition("having a holiday dinner");
const weekendHike = createFamilyTradition("going on a weekend hike");
holidayDinner("Smith");
weekendHike("Johnson");
This example shows how families can have their own unique traditions, represented as functions that can be passed around and invoked.
3. Procedural Programming: Step-by-Step Family Problem Solving
Procedural programming focuses on creating a sequence of steps to solve a problem. This paradigm can be applied to family problem-solving strategies:
function resolveConflict(person1, person2, issue) {
console.log(`1. ${person1} and ${person2} acknowledge the conflict over ${issue}.`);
console.log("2. Both parties express their feelings and concerns.");
console.log("3. Active listening is practiced by both sides.");
console.log("4. Possible solutions are brainstormed together.");
console.log("5. A mutually agreeable solution is chosen.");
console.log("6. The solution is implemented and later reviewed for effectiveness.");
}
resolveConflict("Mom", "Dad", "household chores");
This procedural approach provides a clear, step-by-step method for resolving family conflicts, much like how procedural programming breaks down complex tasks into manageable steps.
4. Event-Driven Programming: Reacting to Family Events
Event-driven programming is based on the concept of events and event handlers. This paradigm can be used to model how families react to various life events:
class FamilyEventEmitter {
constructor() {
this.events = {};
}
on(eventName, callback) {
if (!this.events[eventName]) {
this.events[eventName] = [];
}
this.events[eventName].push(callback);
}
emit(eventName, data) {
if (this.events[eventName]) {
this.events[eventName].forEach(callback => callback(data));
}
}
}
const family = new FamilyEventEmitter();
family.on('birthday', (person) => {
console.log(`It's ${person}'s birthday! Time to celebrate!`);
});
family.on('graduation', (person) => {
console.log(`Congratulations to ${person} on their graduation!`);
});
family.emit('birthday', 'Mom');
family.emit('graduation', 'Sister');
This event-driven approach shows how families can have predefined responses to various life events, creating a system of support and celebration.
5. Concurrent Programming: Balancing Multiple Family Activities
Concurrent programming deals with executing multiple tasks simultaneously. This concept can be applied to how families manage multiple activities and responsibilities:
async function familyWeekend() {
const tasks = [
async () => {
console.log("Mom is grocery shopping");
await new Promise(resolve => setTimeout(resolve, 2000));
console.log("Mom finished shopping");
},
async () => {
console.log("Dad is mowing the lawn");
await new Promise(resolve => setTimeout(resolve, 3000));
console.log("Dad finished mowing");
},
async () => {
console.log("Kids are doing homework");
await new Promise(resolve => setTimeout(resolve, 4000));
console.log("Kids finished homework");
}
];
await Promise.all(tasks.map(task => task()));
console.log("All family tasks completed!");
}
familyWeekend();
This example demonstrates how different family members can work on their tasks concurrently, much like how concurrent programming manages multiple threads of execution.
6. Declarative Programming: Expressing Family Goals and Values
Declarative programming focuses on describing what should be accomplished, rather than how to accomplish it. This can be applied to expressing family goals and values:
const familyValues = {
respect: true,
honesty: true,
kindness: true,
hardWork: true
};
const familyGoals = [
"Spend quality time together",
"Support each other's dreams",
"Maintain a healthy lifestyle",
"Contribute to the community"
];
function livingByValues(values, goals) {
console.log("Our family values:");
Object.entries(values).forEach(([value, important]) => {
if (important) console.log(`- ${value}`);
});
console.log("\nOur family goals:");
goals.forEach(goal => console.log(`- ${goal}`));
}
livingByValues(familyValues, familyGoals);
This declarative approach allows families to clearly state their values and goals without specifying how to achieve them, leaving room for individual interpretation and implementation.
7. Aspect-Oriented Programming: Cross-Cutting Family Concerns
Aspect-Oriented Programming (AOP) deals with cross-cutting concerns that affect multiple parts of a system. In family dynamics, this could represent overarching principles or rules that apply to all family interactions:
function aspect(target, methodName, advice) {
const original = target[methodName];
target[methodName] = function(...args) {
advice.before?.call(this, methodName, args);
const result = original.apply(this, args);
advice.after?.call(this, methodName, result);
return result;
};
}
const respectAspect = {
before(methodName) {
console.log(`Remember to be respectful before ${methodName}`);
},
after(methodName) {
console.log(`Thank you for being respectful during ${methodName}`);
}
};
class FamilyMember {
discuss(topic) {
console.log(`Discussing ${topic}`);
}
disagree(issue) {
console.log(`Disagreeing about ${issue}`);
}
}
const member = new FamilyMember();
aspect(member, 'discuss', respectAspect);
aspect(member, 'disagree', respectAspect);
member.discuss("vacation plans");
member.disagree("household chores");
This AOP-inspired approach shows how principles like respect can be applied across different types of family interactions, ensuring that core values are maintained regardless of the specific situation.
Conclusion: The Family Code
By exploring family dynamics through the lens of various programming paradigms, we gain a unique perspective on the complex system that is a family. Just as different programming paradigms offer various tools and approaches for solving coding problems, they also provide diverse frameworks for understanding and improving family relationships.
Object-Oriented Programming helps us model family members and their relationships, Functional Programming emphasizes consistency and respect for personal growth, Procedural Programming offers step-by-step problem-solving techniques, Event-Driven Programming shows how families can respond to life events, Concurrent Programming illustrates balancing multiple responsibilities, Declarative Programming allows for clear expression of family values and goals, and Aspect-Oriented Programming demonstrates how overarching principles can be applied across all family interactions.
While families are certainly more complex and nuanced than any programming system, these paradigms offer valuable metaphors and tools for analysis. They remind us that, like well-designed code, healthy families require structure, flexibility, clear communication, and a set of guiding principles.
As you reflect on your own family dynamics, consider which programming paradigms might offer insights or strategies for improvement. Remember, the goal isn’t to rigidly apply these concepts, but to use them as a creative lens for understanding and enhancing your family relationships. After all, family life, like programming, is an art that combines logic, creativity, and continuous learning.
By viewing your family through this unique “programmer’s lens,” you might just find new ways to debug conflicts, optimize communication, and create a more harmonious and efficient family system. Happy coding – and happy family building!