The Art of Using Function Names to Express Your Innermost Feelings
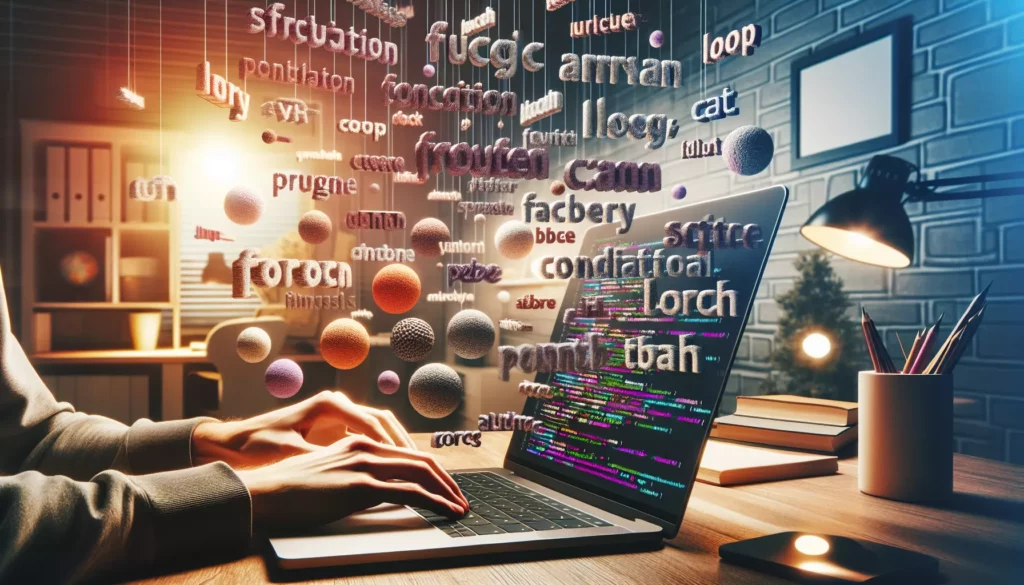
In the vast realm of coding, where logic reigns supreme and syntax is king, there lies an unexpected canvas for emotional expression: function names. While most developers focus on creating clear, descriptive names for their functions, there’s a hidden art form waiting to be explored. Today, we’re diving into the whimsical world of using function names to convey your deepest emotions, all while maintaining code readability and adhering to best practices.
The Importance of Naming in Programming
Before we embark on our journey of emotional expression through function names, let’s remind ourselves why naming is crucial in programming:
- Readability: Well-named functions make code easier to understand at a glance.
- Maintainability: Clear names reduce the cognitive load when revisiting code later.
- Collaboration: Good naming conventions help team members grasp the purpose of code quickly.
- Documentation: Descriptive names can serve as a form of self-documentation.
With these principles in mind, let’s explore how we can infuse our code with emotion while still adhering to good coding practices.
Expressing Joy and Excitement
When you’re feeling particularly joyful about a piece of functionality you’ve just implemented, why not let it show in your function names? Here are some examples:
function calculateTotalWithGlee(items) {
// Your gleeful calculation logic here
}
function fetchDataAndDance(url) {
// Fetch data while doing a little dance
}
function renderUIWithJazzyFlair(component) {
// Add some pizzazz to your UI rendering
}
These function names still clearly convey their purpose while adding a touch of enthusiasm. The key is to maintain clarity while injecting a bit of personality.
Channeling Frustration and Despair
Let’s face it, coding can sometimes be frustrating. When you’re dealing with a particularly tricky bug or a stubborn piece of logic, your function names can reflect that struggle:
function attemptToFixThisMessAgain(data) {
// Yet another attempt to salvage the situation
}
function pleaseJustWorkThisTime(input) {
// A desperate plea to the coding gods
}
function bandaidSolutionUntilRefactor(problem) {
// A temporary fix that you hope won't become permanent
}
While these names might bring a smile to a fellow developer’s face, it’s important to use them judiciously. They can be great for prototype code or personal projects, but might not be appropriate for production environments.
Expressing Love and Affection
For those times when you’re feeling particularly fond of your code or want to spread some positivity, consider these loving function names:
function embraceAndEnhanceData(rawData) {
// Nurture your data to its full potential
}
function gentlyValidateUserInput(input) {
// Handle user input with care and understanding
}
function createHarmoniousUILayout() {
// Craft a UI that's a joy to behold
}
These names not only convey the function’s purpose but also add a touch of warmth to your codebase. They can be particularly effective in projects focused on user experience or when working on features that require a gentle touch.
Conveying Determination and Resilience
When faced with challenging tasks or ambitious goals, let your function names reflect your unwavering determination:
function optimizeUntilPerfection(algorithm) {
// Relentlessly improve until it's flawless
}
function conquerComplexityWithGrit(problem) {
// Take on complex problems with determination
}
function persistThroughAdversity(retries) {
// Keep trying no matter what obstacles arise
}
These names can serve as motivational reminders of the perseverance required in software development. They encapsulate both the function’s purpose and the spirit with which you approach the task.
Balancing Emotion with Professionalism
While expressing emotions through function names can be fun and potentially boost team morale, it’s crucial to strike a balance with professionalism. Here are some guidelines to keep in mind:
- Clarity First: The primary goal of a function name should always be to clearly communicate its purpose.
- Context Matters: What’s appropriate for a personal project might not be suitable for enterprise software.
- Team Agreement: If you’re working in a team, make sure everyone is on board with more expressive naming conventions.
- Avoid Offensive Language: Keep it light and fun without crossing into territory that could make others uncomfortable.
- Use Sparingly: Emotional function names should be the exception, not the rule, to maintain overall code readability.
The Psychology Behind Emotional Coding
Incorporating emotions into your code through function names isn’t just about having fun; it can have psychological benefits as well:
- Stress Relief: Expressing frustration or joy through code can be a healthy outlet for the emotions that come with programming.
- Increased Engagement: Adding personality to your code can make the development process more engaging and enjoyable.
- Team Bonding: Shared humor in code can foster a sense of camaraderie among team members.
- Creativity Boost: Thinking about how to express emotions in function names can stimulate creative thinking in other areas of development.
Real-World Examples and Anecdotes
While we’ve explored hypothetical function names, there are real-world instances where developers have injected emotion into their code:
The Case of the Angry Function
One developer shared a story of inheriting a codebase with a function named killItWithFire()
. Upon investigation, they discovered it was a last-resort error handling function that essentially reset the entire system state. While humorous, it also quickly conveyed the drastic nature of the function to anyone reading the code.
The Optimistic Startup
A startup known for its positive company culture had a convention of prefixing their main functions with uplifting words. For example:
function cheerfullyProcessPayment(amount) {
// Handle payment processing with a smile
}
function enthusiasticallyLoadUserProfile(userId) {
// Load user data with boundless energy
}
While unconventional, this approach aligned with their brand and made their codebase uniquely theirs.
Emotional Function Names in Different Programming Paradigms
The art of emotional function naming can be adapted to various programming paradigms:
Object-Oriented Programming (OOP)
In OOP, you can express emotions through method names within classes:
class DataProcessor {
joyfullyTransformData(data) {
// Transform data with glee
}
stubbornlyValidateInput(input) {
// Rigorously check input, leaving no stone unturned
}
}
Functional Programming
Functional programming often emphasizes pure functions, but that doesn’t mean they can’t have personality:
const blissfullyMapData = (data, transform) => data.map(transform);
const stoicallyReduceToEssence = (data, reducer, initial) => data.reduce(reducer, initial);
Procedural Programming
Even in straightforward procedural code, there’s room for emotional expression:
function main() {
let data = determinedlyGatherInput();
let result = passionatelyProcessData(data);
triumphantlyDisplayResult(result);
}
The Impact on Code Reviews and Documentation
Introducing emotional function names can have interesting effects on the code review process and documentation:
Code Reviews
Emotional function names can make code reviews more engaging. They might:
- Spark discussions about the intent behind certain implementations
- Lighten the mood during potentially tense review sessions
- Encourage reviewers to think about the developer’s state of mind when writing the code
However, it’s important to ensure that the emotional names don’t distract from the technical aspects of the review.
Documentation
When it comes to documentation, emotional function names can add a layer of context:
/**
* Stubbornly attempts to connect to the database.
* This function will not give up easily, reflecting our determination
* to establish a connection despite potential network issues.
*/
function stubbornlyConnectToDatabase(config) {
// Persistent connection logic here
}
This approach can make documentation more memorable and give insight into the function’s behavior beyond just its technical description.
Emotional Function Names in Learning and Education
In the context of coding education, such as the interactive tutorials and resources provided by AlgoCademy, emotional function names can serve several purposes:
Engagement
For beginners, encountering function names like bravelyAttemptFirstLoop()
or celebrateSuccessfulCompilation()
can make the learning process more engaging and less intimidating.
Memorability
Unique, emotion-laden function names can help concepts stick in learners’ minds. For example, a sorting algorithm might be introduced as:
function patientlyBubbleSort(array) {
// Implementation of bubble sort
}
This name not only describes what the function does but also hints at the nature of the algorithm (it’s not the fastest, but it gets there eventually).
Emotional Intelligence in Coding
Using emotional function names in educational contexts can help develop emotional intelligence in coding. It teaches students that programming isn’t just about logic and syntax, but also about expressing ideas and solving human problems.
The Future of Emotional Coding
As we look to the future of software development, the concept of emotional function naming could evolve in interesting ways:
AI-Generated Emotional Names
With advancements in AI, we might see tools that suggest emotional function names based on the code’s purpose and the project’s overall mood or brand personality.
Emotion-Aware IDEs
Integrated Development Environments (IDEs) could incorporate features that allow developers to tag functions with emotions, potentially using emojis or color-coding to visually represent the emotional context of different code sections.
Standardization of Emotional Coding Practices
As the practice gains traction, we might see the emergence of style guides and best practices for incorporating emotions into code in a standardized, professional manner.
Conclusion: Embracing the Human Side of Coding
The art of using function names to express your innermost feelings is more than just a quirky coding practice. It’s a reminder that behind every line of code, there’s a human with thoughts, feelings, and a unique perspective. By allowing a bit of emotional expression into our code, we can:
- Make coding more enjoyable and personally meaningful
- Foster creativity and engagement in development teams
- Add an extra layer of context to our code’s intent
- Create more memorable and relatable learning experiences for new programmers
As we continue to push the boundaries of what’s possible with technology, let’s not forget the artistry and humanity that goes into creating it. So the next time you’re naming a function, don’t be afraid to let it reflect not just what the code does, but how you feel about it. Who knows? Your joyfullyOptimizeAlgorithm()
might just bring a smile to a fellow developer’s face and make their day a little brighter.
Remember, in the world of AlgoCademy and beyond, coding is not just about solving problems—it’s about expressing ideas, fostering growth, and sometimes, letting your code speak to the very essence of what it means to be a passionate, feeling developer in a world of logic and algorithms.