The Art of Turning Your Coding Frustrations into a Successful Music Career
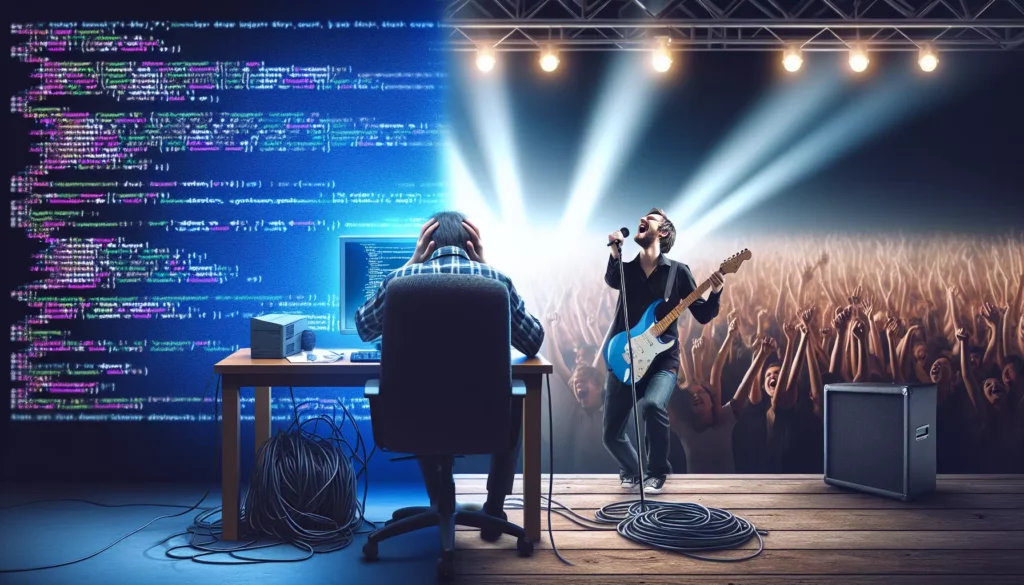
In the world of programming, frustration is an all-too-familiar companion. From elusive bugs to complex algorithms, the journey of a coder is often paved with challenges that can test even the most patient of souls. But what if I told you that these very frustrations could be the key to unlocking a successful music career? It may sound far-fetched, but the connection between coding and music is stronger than you might think. In this article, we’ll explore how the skills you develop as a programmer can translate into musical prowess, and how you can channel your coding frustrations into creative energy for your music.
The Unexpected Harmony Between Coding and Music
At first glance, coding and music might seem like two completely different worlds. One involves logical thinking and problem-solving, while the other is often associated with creativity and emotion. However, upon closer inspection, you’ll find that these two disciplines share more similarities than differences:
- Pattern Recognition: Both coding and music rely heavily on recognizing and creating patterns. In programming, you look for patterns in code to optimize algorithms, while in music, you identify patterns in melodies and rhythms to create harmonious compositions.
- Structure and Logic: Writing code requires a structured approach and logical thinking. Similarly, composing music involves arranging notes and rhythms in a logical sequence to create a cohesive piece.
- Problem-Solving: Debugging code and resolving musical dissonance both require creative problem-solving skills.
- Attention to Detail: Just as a single misplaced semicolon can break your code, a single off-key note can disrupt an entire musical piece.
- Continuous Learning: Both fields are constantly evolving, requiring practitioners to stay updated with new technologies and techniques.
Understanding these parallels can help you leverage your coding skills in your musical endeavors. Let’s dive deeper into how you can turn your coding frustrations into musical inspiration.
Debugging Your Way to Musical Inspiration
Every programmer knows the feeling of staring at a screen for hours, trying to figure out why their code isn’t working. While frustrating, this process of debugging can actually be a wellspring of musical inspiration. Here’s how:
1. Embrace the Problem-Solving Mindset
When you’re stuck on a coding problem, you often need to approach it from different angles. This same mindset can be applied to music creation. If you’re struggling with a melody or chord progression, try approaching it from a different perspective. Just as you might refactor your code, try rearranging your musical elements to see if a new structure works better.
2. Use Rubber Duck Debugging for Songwriting
Rubber duck debugging is a method where programmers explain their code line-by-line to an inanimate object (often a rubber duck) to find errors. Apply this technique to your songwriting process. Explain your song structure, lyrics, or melody to an imaginary listener. This can help you identify weak points in your composition and spark new ideas.
3. Turn Error Messages into Lyrics
Those cryptic error messages that drive you crazy? They could be the starting point for unique and quirky lyrics. Take the essence of an error message and turn it into a metaphor for life’s challenges. For example, “Null Pointer Exception” could become a song about feeling lost and disconnected in the digital age.
Algorithmic Thinking in Music Composition
Your experience with algorithms can be a powerful tool in music composition. Here’s how you can apply algorithmic thinking to create unique musical pieces:
1. Create Musical Algorithms
Just as you write algorithms to solve coding problems, you can create algorithms for generating musical patterns. For example, you could create a simple algorithm that generates a melody based on the Fibonacci sequence:
function fibonacciMelody(length) {
let sequence = [0, 1];
let melody = [];
for (let i = 2; i < length; i++) {
sequence[i] = sequence[i-1] + sequence[i-2];
melody.push(sequence[i] % 12); // Map to 12 notes in an octave
}
return melody;
}
This algorithm would generate a sequence of notes based on the Fibonacci numbers, potentially creating an interesting and mathematically-inspired melody.
2. Use Recursion in Music
Recursion is a powerful concept in programming, and it can be equally powerful in music. Think of how you could apply recursive patterns to your compositions. For instance, you could create a melody that calls itself with slight variations, creating a complex, layered piece of music.
3. Implement Sorting Algorithms Musically
Sorting algorithms like bubble sort or quicksort can be translated into musical arrangements. Assign different pitches to elements in an array and then “play” the sorting process. This can create interesting auditory representations of these algorithms and potentially lead to unique musical ideas.
From Stack Overflow to Music Overflow
Every programmer’s best friend (or sometimes frenemy) is Stack Overflow. The platform’s collaborative problem-solving approach can be adapted to your music-making process:
1. Collaborative Songwriting
Just as you might post a coding question on Stack Overflow, consider reaching out to online music communities with specific songwriting challenges. Platforms like Reddit’s r/WeAreTheMusicMakers or music-specific forums can be great places to get feedback and ideas.
2. Sample and Remix
In programming, we often use libraries and frameworks created by others. Apply this concept to music by sampling and remixing existing tracks. This not only saves time but can also lead to unique combinations and fresh sounds.
3. Document Your Process
Good programmers document their code. Apply this habit to your music-making process. Keep detailed notes about your composition process, instrument settings, and production techniques. This documentation can be invaluable when you want to replicate a sound or technique in the future.
Turning Coding Concepts into Musical Techniques
Many programming concepts have direct parallels in music production and composition. Let’s explore how you can leverage your coding knowledge in your music career:
1. Variables as Musical Motifs
In programming, variables store and represent data. In music, think of motifs or recurring themes as variables. Just as you would manipulate a variable in code, you can transform and develop these musical motifs throughout a piece.
2. Functions as Musical Phrases
Functions in programming perform specific tasks and can be called multiple times. Similarly, you can create musical phrases that act like functions – reusable melodic or rhythmic patterns that you can call upon and modify throughout your composition.
3. Loops for Repetition and Variation
Loops are fundamental in programming, and they’re equally important in music. Use loop structures to create repetitive patterns in your music, but don’t forget to include variation to keep things interesting. Here’s a pseudo-code example of how you might structure a musical loop with variation:
function createMusicalLoop(baseBeat, variations, repetitions) {
let loop = [];
for (let i = 0; i < repetitions; i++) {
if (i % 4 === 0 && i !== 0) {
loop.push(variations[Math.floor(Math.random() * variations.length)]);
} else {
loop.push(baseBeat);
}
}
return loop;
}
This function creates a loop that introduces a random variation every fourth repetition, adding interest to the repetitive structure.
4. Conditionals for Dynamic Compositions
Use conditional statements to create dynamic, responsive music. For example, you could write a program that changes the key or tempo of a piece based on input from the listener or environmental factors like time of day or weather.
5. Object-Oriented Programming in Music
Apply object-oriented programming concepts to your music production. Think of different instruments or sounds as objects with properties (like pitch, volume, effects) and methods (like play, stop, loop). This structured approach can help you organize complex compositions and create reusable sound elements.
From Syntax Errors to Synth Textures
Those pesky syntax errors that plague your code can be a source of inspiration for creating unique synth textures and sound effects. Here’s how:
1. Glitch Music
Glitch music is a genre that embraces errors and malfunctions in electronic systems. Take inspiration from the glitches and errors you encounter in your code to create interesting sound textures. For example, you could recreate the sound of a buffer overflow or a stack trace in your music.
2. Error-Inspired Sound Design
Use the characteristics of different types of coding errors to inspire your sound design. For instance:
- Infinite loops could inspire endlessly evolving ambient textures
- Stack overflows might translate to heavily layered, chaotic sounds
- Null pointer exceptions could be represented by sudden silences or drops in the music
3. Code-Generated Music
Take it a step further and write code that generates music based on its own errors. Here’s a simple example in Python that generates a melody based on the ASCII values of error messages:
import random
def generate_error_melody():
try:
# Intentionally cause an error
1 / 0
except Exception as e:
error_message = str(e)
melody = [ord(char) % 12 for char in error_message]
return melody
# Generate and play the melody
error_melody = generate_error_melody()
print("Error Melody:", error_melody)
This code intentionally causes a division by zero error, then uses the ASCII values of the error message characters to generate a sequence of notes (mapped to the 12 notes of an octave).
Version Control Your Music
Version control systems like Git are essential tools for programmers. Apply these concepts to your music production process:
1. Track Changes in Your Compositions
Use version control to keep track of changes in your music projects. This allows you to experiment freely, knowing you can always revert to a previous version if needed. Tools like Git can be used for this, or you can explore music-specific version control solutions.
2. Collaborate Like a Dev Team
Use platforms like GitHub to collaborate on music projects. You can share your music files, accept pull requests for contributions from others, and manage different versions of your tracks.
3. Branching for Experimentation
Create branches in your music projects to experiment with different ideas without affecting the main version. This can be particularly useful when trying out new arrangements or production techniques.
Optimizing Your Music Career Like You Optimize Code
Your experience in optimizing code can be directly applied to optimizing your music career:
1. Analyze Performance Metrics
Just as you would analyze code performance, analyze the performance of your music. Look at metrics like streaming numbers, engagement rates, and audience retention. Use this data to inform your musical decisions and career strategy.
2. A/B Testing in Music
Apply the concept of A/B testing to your music. Release different versions of a track to see which performs better with your audience. This can help you understand your listeners’ preferences and refine your sound.
3. Continuous Integration/Continuous Deployment (CI/CD) for Music Releases
Implement a CI/CD-like approach to your music releases. Continuously create and release music, gathering feedback and iterating quickly. This can help you maintain momentum and keep your audience engaged.
From Coding Bootcamps to Music Production Courses
Your experience with coding education platforms like AlgoCademy can guide your approach to learning music production:
1. Structured Learning Path
Just as AlgoCademy provides a structured path from beginner to interview-ready programmer, create a structured learning path for your music education. Start with the basics of music theory and gradually progress to advanced production techniques.
2. Interactive Learning
Look for interactive music learning platforms that offer hands-on experience, similar to the coding challenges on AlgoCademy. Platforms like Ableton Learning Synths or Soundgym offer interactive lessons for music production and ear training.
3. AI-Assisted Learning
Leverage AI-powered tools in your music learning journey, similar to the AI assistance provided by AlgoCademy. Tools like AIVA or Amper Music can help you understand composition by analyzing and generating musical pieces.
Conclusion: Composing Your Coding Frustrations into Musical Success
The journey from coding frustrations to a successful music career may seem like a leap, but as we’ve explored, the skills and mindset you’ve developed as a programmer are invaluable assets in the world of music. By applying your problem-solving skills, algorithmic thinking, and attention to detail to your musical endeavors, you can create unique and compelling compositions.
Remember, every bug you’ve squashed, every algorithm you’ve optimized, and every late-night coding session you’ve endured has equipped you with skills that can be translated into musical creativity. Your coding frustrations, rather than being obstacles, can become the very fuel that drives your musical innovation.
So the next time you find yourself pulling your hair out over a particularly tricky piece of code, take a step back and consider how you might channel that frustration into your music. That bug might just be the inspiration for your next hit song. After all, in both coding and music, the most beautiful creations often arise from overcoming the greatest challenges.
Embrace your unique background as a coder-turned-musician. Let your logical mind dance with your creative spirit. In the intersection of algorithms and melodies, codes and chords, you might just find your true calling. So go ahead, compose an algorithm, debug a melody, and let your coding frustrations fuel your journey to musical success. The stage is set, and your audience – both human and machine – awaits your performance.