The Art of Starting a Project Without an Instruction Manual
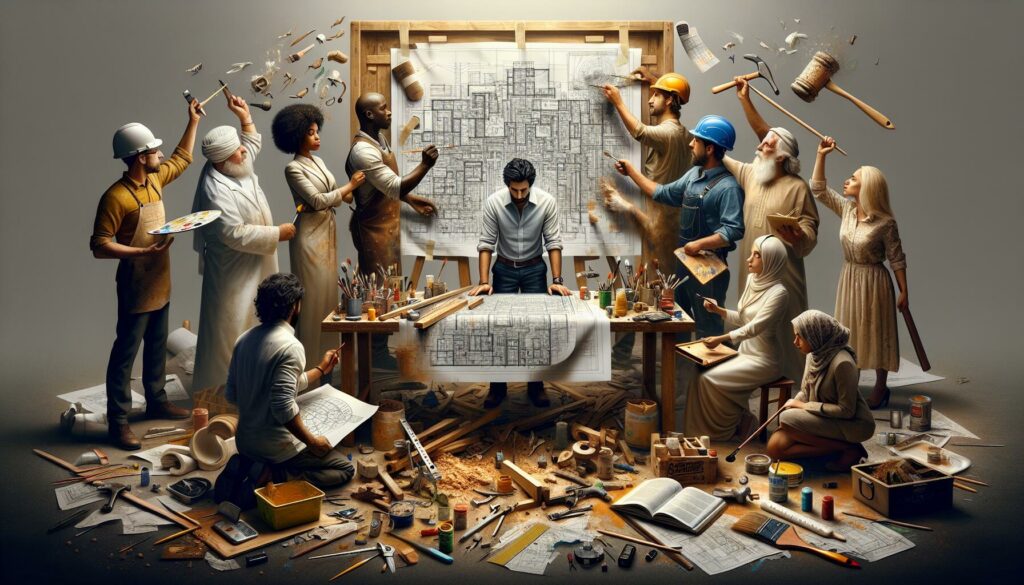
In the world of coding and software development, there’s a thrilling yet daunting challenge that every programmer faces at some point in their career: starting a project from scratch without a detailed instruction manual. This scenario is not just common in professional settings but is also a crucial skill for aspiring developers looking to break into the tech industry, especially when aiming for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google).
At AlgoCademy, we understand that mastering the art of initiating projects independently is a key component of coding education and programming skills development. It’s a skill that goes beyond mere coding proficiency, encompassing problem-solving, creativity, and strategic thinking. In this comprehensive guide, we’ll explore the intricacies of embarking on a project without explicit instructions, providing you with the tools and mindset needed to excel in such situations.
1. Understanding the Importance of Self-Directed Projects
Before diving into the practical aspects, it’s crucial to understand why the ability to start a project without an instruction manual is so valuable:
- Real-world Simulation: In professional settings, you’ll often face scenarios where requirements are vague or evolving.
- Skill Development: It forces you to think critically and develop problem-solving skills.
- Creativity Boost: Without strict guidelines, you have the freedom to explore innovative solutions.
- Interview Preparation: Many technical interviews, especially for FAANG companies, involve open-ended problem-solving tasks.
2. The Initial Approach: Gathering Information
When faced with a project lacking clear instructions, your first step should be information gathering:
2.1 Define the Problem
Start by clearly articulating what the project aims to achieve. Ask yourself:
- What is the main goal of this project?
- Who are the end-users or stakeholders?
- What problem does this project solve?
2.2 Research Similar Projects
Look for existing solutions or similar projects. This can provide inspiration and help you understand industry standards:
- Search GitHub for open-source projects in the same domain.
- Study documentation of related technologies or frameworks.
- Analyze competitor products if applicable.
2.3 Identify Constraints and Resources
Understand the limitations and available resources:
- Time constraints
- Budget limitations
- Technical resources (hardware, software, APIs)
- Team size and expertise
3. Planning and Design
With initial information gathered, it’s time to plan and design your approach:
3.1 Create a High-Level Design
Sketch out the overall architecture of your project:
- Identify main components or modules
- Determine how these components will interact
- Choose appropriate technologies or frameworks
3.2 Break Down the Project into Smaller Tasks
Divide the project into manageable chunks:
- Create a task list or use project management tools like Trello or Jira
- Prioritize tasks based on dependencies and importance
- Estimate time for each task (be generous with your estimates)
3.3 Prototype and Validate
Before diving into full-scale development, create prototypes to validate your ideas:
- Build a minimal viable product (MVP) to test core functionalities
- Use wireframes or mockups for UI/UX design
- Conduct user testing if possible
4. Development Strategies
With a plan in place, it’s time to start coding. Here are some strategies to keep you on track:
4.1 Start with Core Functionality
Begin by implementing the most crucial features:
- Focus on the ‘must-have’ features first
- Implement a basic working version before adding complexity
4.2 Use Version Control
Implement version control from the start:
- Use Git for tracking changes and collaboration
- Create meaningful commit messages
- Utilize branches for different features or experiments
4.3 Write Clean, Documented Code
Maintain good coding practices:
- Follow coding standards and best practices for your chosen language
- Write clear, self-explanatory code
- Include comments and documentation where necessary
4.4 Implement Testing Early
Integrate testing into your development process:
- Write unit tests for individual components
- Implement integration tests for interacting modules
- Consider test-driven development (TDD) approach
5. Overcoming Common Challenges
Starting a project without instructions comes with its unique set of challenges. Here’s how to overcome them:
5.1 Dealing with Ambiguity
Embrace uncertainty and use it as an opportunity for creativity:
- Make assumptions and document them
- Be prepared to pivot if new information arises
- Use agile methodologies to adapt to changes quickly
5.2 Avoiding Scope Creep
Keep your project focused:
- Regularly review and adjust your project scope
- Learn to say no to non-essential features
- Use the MoSCoW method (Must have, Should have, Could have, Won’t have) for feature prioritization
5.3 Overcoming Technical Roadblocks
When faced with technical challenges:
- Break complex problems into smaller, manageable parts
- Utilize online resources like Stack Overflow or developer forums
- Don’t hesitate to ask for help or collaborate with peers
6. Leveraging AI and Tools
In today’s tech landscape, AI-powered tools can significantly aid in project development:
6.1 AI-Assisted Coding
Utilize AI coding assistants:
- Tools like GitHub Copilot or TabNine can help with code completion and generation
- Use these tools to speed up coding but always review and understand the generated code
6.2 Automated Testing Tools
Implement automated testing:
- Use tools like Selenium for automated UI testing
- Implement continuous integration/continuous deployment (CI/CD) pipelines
6.3 Project Management and Collaboration Tools
Leverage tools for efficient project management:
- Use platforms like Jira or Asana for task tracking
- Implement communication tools like Slack for team collaboration
7. Learning and Iteration
The process of starting a project without instructions is also a learning opportunity:
7.1 Continuous Learning
Embrace a mindset of continuous improvement:
- Stay updated with the latest technologies and best practices
- Attend webinars, conferences, or online courses to expand your knowledge
7.2 Reflection and Retrospectives
Regularly reflect on your progress:
- Conduct personal or team retrospectives
- Identify what worked well and areas for improvement
- Implement lessons learned in future projects
8. Case Study: Building a Web Application from Scratch
Let’s apply these principles to a practical example: building a simple web application without specific instructions.
8.1 Project Definition
Imagine you’re tasked with creating a web-based task management application. No further details are provided.
8.2 Information Gathering
- Research existing task management apps (Trello, Asana, etc.)
- Identify core features: task creation, assignment, due dates, status tracking
- Determine target users: small teams or individual professionals
8.3 Planning and Design
- Choose a tech stack: React for frontend, Node.js with Express for backend, MongoDB for database
- Design basic UI wireframes
- Plan API endpoints
8.4 Development Process
Here’s a basic outline of how you might approach the development:
// Backend: Set up Express server
const express = require('express');
const app = express();
app.get('/api/tasks', (req, res) => {
// Logic to fetch tasks
});
app.post('/api/tasks', (req, res) => {
// Logic to create a new task
});
// Frontend: React component for task list
import React, { useState, useEffect } from 'react';
function TaskList() {
const [tasks, setTasks] = useState([]);
useEffect(() => {
// Fetch tasks from API
}, []);
return (
<div>
{tasks.map(task => (
<TaskItem key={task.id} task={task} />
))}
</div>
);
}
8.5 Testing and Iteration
- Implement unit tests for API endpoints and React components
- Conduct user testing with a small group
- Iterate based on feedback, adding features like task prioritization or team collaboration
9. Preparing for Technical Interviews
The skills developed in starting projects without instructions are invaluable for technical interviews, especially for FAANG companies:
9.1 Problem-Solving Skills
Interviewers often present open-ended problems to assess your approach:
- Practice breaking down complex problems into smaller, manageable parts
- Learn to communicate your thought process clearly
- Be prepared to discuss trade-offs in your solutions
9.2 System Design Questions
Many interviews include system design questions that require you to architect a solution from scratch:
- Study common system design patterns and architectures
- Practice designing scalable systems for various scenarios
- Be ready to discuss considerations like scalability, reliability, and performance
9.3 Coding Challenges
Prepare for coding challenges that may not have clear instructions:
- Practice algorithmic problem-solving on platforms like LeetCode or HackerRank
- Focus on understanding the problem before jumping into coding
- Learn to ask clarifying questions when faced with ambiguous requirements
10. Conclusion: Embracing the Challenge
Starting a project without an instruction manual is both an art and a science. It requires a blend of technical skills, creativity, problem-solving abilities, and the confidence to navigate uncertainty. By embracing this challenge, you not only develop valuable skills for your career but also prepare yourself for the dynamic and often ambiguous nature of real-world software development.
At AlgoCademy, we believe that mastering this art is crucial for any aspiring developer, especially those aiming for positions at top tech companies. Our platform offers interactive coding tutorials, AI-powered assistance, and resources tailored to help you develop these essential skills. Whether you’re a beginner just starting your coding journey or an experienced developer looking to sharpen your skills, remember that each project without instructions is an opportunity to grow, innovate, and push your boundaries as a programmer.
As you continue your journey in software development, embrace the challenges that come with undefined projects. They are not just obstacles to overcome but opportunities to showcase your creativity, problem-solving skills, and technical prowess. With practice and perseverance, you’ll find that the art of starting a project without an instruction manual becomes not just a skill, but a thrilling adventure in the world of coding.