The Art of Refactoring: Why You Should Fall in Love with Improving Old Code
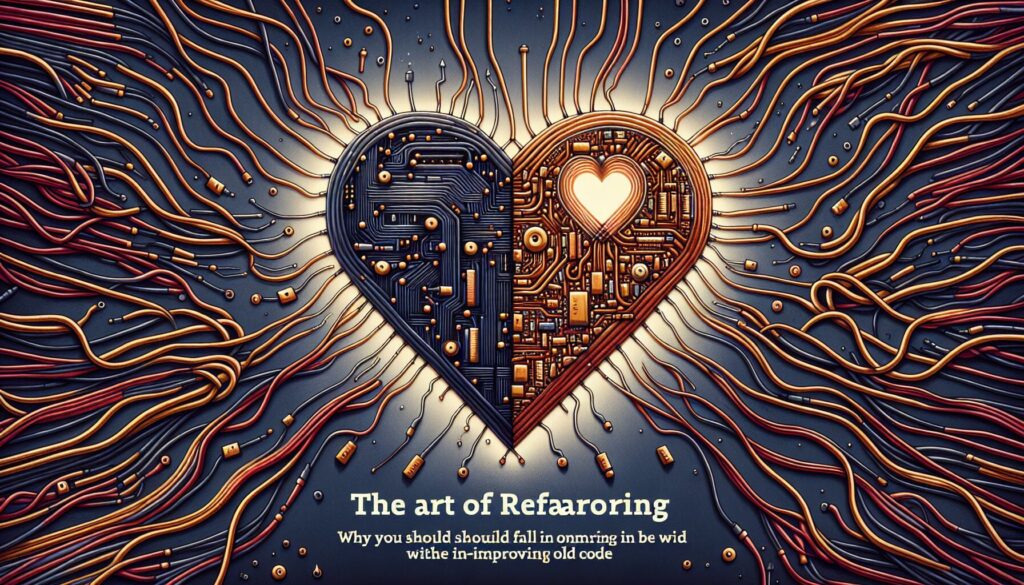
In the ever-evolving world of software development, writing code is just the beginning. As projects grow and requirements change, the need to revisit and improve existing code becomes increasingly important. This is where the art of refactoring comes into play. Refactoring is not just a technical practice; it’s a mindset that can transform the way you approach coding and elevate your skills as a developer. In this comprehensive guide, we’ll explore the importance of refactoring, its benefits, and how to master this essential skill.
What is Refactoring?
Refactoring is the process of restructuring existing computer code without changing its external behavior. It’s about improving the internal structure of the software while preserving its functionality. Think of it as giving your code a makeover – enhancing its readability, maintainability, and efficiency without altering what it does.
Martin Fowler, a pioneer in the field of refactoring, defines it as “a disciplined technique for restructuring an existing body of code, altering its internal structure without changing its external behavior.”
Why Should You Care About Refactoring?
As a developer, especially one aspiring to work at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding and practicing refactoring is crucial. Here’s why:
- Improved Code Quality: Refactoring helps in eliminating code smells, reducing complexity, and making the codebase more maintainable.
- Enhanced Readability: Clean, well-structured code is easier to understand, not just for you but for your team members as well.
- Increased Efficiency: Refactoring can lead to performance improvements by optimizing algorithms and data structures.
- Better Testability: Refactored code is often easier to test, leading to more robust and reliable software.
- Easier Debugging: When code is well-organized, finding and fixing bugs becomes much simpler.
- Facilitates Feature Addition: A clean codebase makes it easier to add new features without breaking existing functionality.
The Refactoring Process
Refactoring is not a one-time task but an ongoing process. Here’s a general approach to refactoring:
- Identify the Need: Recognize code smells or areas that need improvement.
- Plan the Refactoring: Decide on the specific refactoring techniques you’ll use.
- Write Tests: Ensure you have adequate test coverage before making changes.
- Make Small, Incremental Changes: Refactor in small steps to maintain control and avoid introducing bugs.
- Run Tests: After each change, run your tests to ensure functionality is preserved.
- Review and Iterate: Assess the improvements and repeat the process if necessary.
Common Refactoring Techniques
There are numerous refactoring techniques, each addressing different aspects of code improvement. Let’s explore some of the most common ones:
1. Extract Method
This technique involves taking a code fragment and turning it into a method. It’s useful when you have a long method or a piece of code that needs to be used in multiple places.
Before refactoring:
public void printOwing() {
printBanner();
// Print details
System.out.println("name: " + name);
System.out.println("amount: " + getOutstanding());
}
After refactoring:
public void printOwing() {
printBanner();
printDetails(getOutstanding());
}
private void printDetails(double outstanding) {
System.out.println("name: " + name);
System.out.println("amount: " + outstanding);
}
2. Rename Method
Renaming a method to better reflect its purpose can significantly improve code readability.
Before refactoring:
public class Customer {
public double calcTotal() {
// calculation logic
}
}
After refactoring:
public class Customer {
public double calculateTotalPrice() {
// calculation logic
}
}
3. Move Method
This technique involves moving a method from one class to another where it’s more logically placed.
Before refactoring:
class Account {
private AccountType type;
private int daysOverdrawn;
double overdraftCharge() {
if (type.isPremium()) {
double result = 10;
if (daysOverdrawn > 7) result += (daysOverdrawn - 7) * 0.85;
return result;
}
else return daysOverdrawn * 1.75;
}
}
After refactoring:
class Account {
private AccountType type;
private int daysOverdrawn;
double overdraftCharge() {
return type.overdraftCharge(daysOverdrawn);
}
}
class AccountType {
double overdraftCharge(int daysOverdrawn) {
if (isPremium()) {
double result = 10;
if (daysOverdrawn > 7) result += (daysOverdrawn - 7) * 0.85;
return result;
}
else return daysOverdrawn * 1.75;
}
}
4. Replace Conditional with Polymorphism
This technique helps in removing complex conditional logic by using polymorphism.
Before refactoring:
class Bird {
private String type;
double getSpeed() {
switch (type) {
case "EUROPEAN":
return getBaseSpeed();
case "AFRICAN":
return getBaseSpeed() - getLoadFactor() * numberOfCoconuts;
case "NORWEGIAN_BLUE":
return (isNailed) ? 0 : getBaseSpeed(voltage);
default:
throw new RuntimeException("Should be unreachable");
}
}
}
After refactoring:
abstract class Bird {
abstract double getSpeed();
}
class European extends Bird {
double getSpeed() {
return getBaseSpeed();
}
}
class African extends Bird {
double getSpeed() {
return getBaseSpeed() - getLoadFactor() * numberOfCoconuts;
}
}
class NorwegianBlue extends Bird {
double getSpeed() {
return (isNailed) ? 0 : getBaseSpeed(voltage);
}
}
Tools for Refactoring
While refactoring can be done manually, there are several tools available that can assist in the process:
- IDEs: Most modern IDEs like IntelliJ IDEA, Eclipse, and Visual Studio Code offer built-in refactoring tools.
- SonarQube: A platform for continuous inspection of code quality that can detect code smells and suggest refactoring opportunities.
- ReSharper: A popular Visual Studio extension that provides advanced refactoring capabilities for C# developers.
- Checkstyle: A development tool for Java that helps programmers write code that adheres to a coding standard.
- ESLint: A pluggable linting utility for JavaScript and JSX that can help identify and fix code quality issues.
Best Practices for Refactoring
To make the most of your refactoring efforts, consider these best practices:
- Refactor Regularly: Make refactoring a part of your daily coding routine rather than a one-time event.
- Follow the Boy Scout Rule: Always leave the code better than you found it.
- Test Before and After: Ensure you have a solid test suite in place before refactoring and run tests after each change.
- Commit Often: Make small, incremental changes and commit frequently to maintain a clear history of your refactoring process.
- Communicate with Your Team: If you’re working on a shared codebase, inform your team about significant refactoring efforts.
- Understand the Code: Before refactoring, make sure you fully understand the code and its purpose.
- Focus on Readability: Prioritize making the code more readable and self-explanatory.
- Don’t Optimize Prematurely: Focus on clean, readable code first. Optimize only when necessary and after profiling.
The Impact of Refactoring on Your Career
Mastering the art of refactoring can have a significant impact on your career as a software developer, especially if you’re aiming for positions at top tech companies:
- Improved Problem-Solving Skills: Refactoring challenges you to think critically about code structure and design, enhancing your overall problem-solving abilities.
- Better Code Reviews: Understanding refactoring techniques makes you better at reviewing others’ code and providing constructive feedback.
- Increased Value to Employers: Developers who can effectively refactor and improve existing codebases are highly valued in the industry.
- Enhanced System Design Skills: Regular refactoring improves your understanding of good system design principles.
- Preparation for Technical Interviews: Many technical interviews, especially at FAANG companies, include questions about code optimization and refactoring.
Refactoring in Different Programming Paradigms
While the core principles of refactoring remain the same, the specific techniques can vary depending on the programming paradigm you’re working with:
Object-Oriented Programming (OOP)
In OOP, refactoring often focuses on improving class structures, enhancing encapsulation, and leveraging inheritance and polymorphism effectively. Common techniques include:
- Extract Class/Interface
- Pull Up/Push Down Method
- Replace Inheritance with Delegation
- Encapsulate Field
Functional Programming
Functional programming refactoring techniques often aim to improve function composition, reduce side effects, and enhance immutability. Some techniques include:
- Extract Function
- Inline Function
- Convert Imperative Loop to Functional Style
- Replace Mutable Variable with Immutable Value
Procedural Programming
In procedural programming, refactoring often focuses on improving the structure and organization of procedures and data. Techniques might include:
- Extract Procedure
- Inline Procedure
- Replace Global Variable with Parameter
- Split Loop
Refactoring and Technical Debt
Refactoring plays a crucial role in managing technical debt. Technical debt refers to the implied cost of additional rework caused by choosing an easy (limited) solution now instead of using a better approach that would take longer.
Regular refactoring helps in:
- Reducing Accumulated Technical Debt: By continuously improving code quality, you prevent the buildup of technical debt.
- Improving System Longevity: Well-maintained code is more adaptable to future changes and less likely to become legacy code that no one wants to touch.
- Balancing Short-term and Long-term Goals: While feature development is important, refactoring ensures that the codebase remains healthy for future development.
Challenges in Refactoring
While refactoring is beneficial, it’s not without its challenges:
- Time Constraints: In fast-paced development environments, finding time for refactoring can be difficult.
- Risk of Introducing Bugs: Changing existing code always carries the risk of introducing new bugs.
- Resistance from Stakeholders: Non-technical stakeholders might not see the immediate value in refactoring.
- Scope Creep: It’s easy for a small refactoring task to expand into a major overhaul if not managed properly.
- Lack of Tests: Refactoring becomes risky and challenging when there’s inadequate test coverage.
Refactoring and Performance Optimization
While refactoring primarily focuses on improving code structure and readability, it can also lead to performance improvements. However, it’s important to distinguish between refactoring for clarity and optimizing for performance:
- Refactoring for Clarity: This focuses on making the code more understandable and maintainable, which indirectly can lead to performance improvements as inefficiencies become more apparent.
- Performance Optimization: This specifically targets improving the speed or resource usage of the code, sometimes at the expense of readability.
It’s generally recommended to refactor for clarity first, and then optimize for performance if necessary. This approach ensures that you’re working with clean, understandable code when you start optimizing.
Refactoring in Agile Development
In Agile methodologies, refactoring is often an integral part of the development process:
- Continuous Refactoring: Agile teams often practice continuous refactoring, making small improvements with each iteration.
- Refactoring User Stories: Some teams include dedicated refactoring user stories in their sprints to ensure technical debt is addressed regularly.
- Pair Programming: This Agile practice can be particularly effective for refactoring, as it combines different perspectives and reduces the risk of introducing errors.
Conclusion: Embracing the Art of Refactoring
Refactoring is more than just a technical practice; it’s an art form that requires creativity, discipline, and a deep understanding of code design principles. By embracing refactoring, you not only improve the quality of your code but also continuously enhance your skills as a developer.
As you progress in your coding journey, whether you’re preparing for technical interviews at top tech companies or working on personal projects, make refactoring an integral part of your development process. Remember, great code isn’t just written; it’s refined and polished over time.
By falling in love with the art of refactoring, you’re not just improving old code – you’re investing in your growth as a developer, creating more maintainable software, and setting yourself up for long-term success in the ever-evolving world of software development.
So, the next time you look at a piece of code and think, “This could be better,” don’t just move on. Take the time to refactor, improve, and perfect. Your future self, your team, and the users of your software will thank you for it.