The Art of Pseudocode in Problem Solving
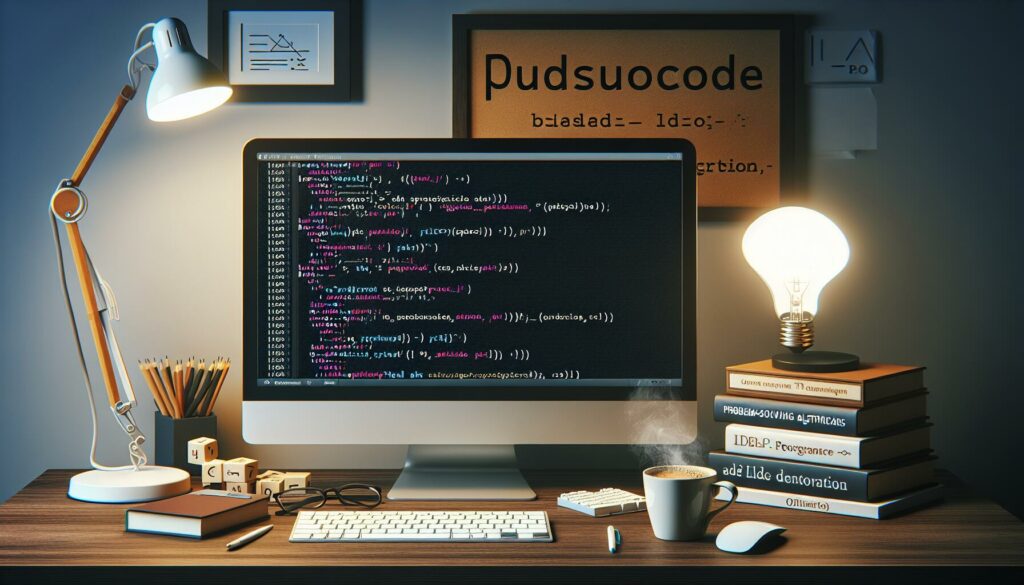
In the world of programming and software development, problem-solving is a crucial skill that separates great developers from the rest. One of the most effective tools in a programmer’s arsenal for tackling complex problems is pseudocode. This powerful technique allows developers to break down intricate issues into manageable steps, plan their approach, and communicate ideas clearly before diving into actual coding. In this comprehensive guide, we’ll explore the art of pseudocode and how it can revolutionize your problem-solving process.
What is Pseudocode?
Pseudocode is a informal, high-level description of a computer program or algorithm. It uses structural conventions of a normal programming language, but is intended for human reading rather than machine reading. Essentially, it’s a way of planning out your code in plain language before translating it into a specific programming language.
The beauty of pseudocode lies in its flexibility and accessibility. It doesn’t adhere to the strict syntax of any particular programming language, making it an ideal tool for both beginners and experienced programmers alike. By using pseudocode, developers can focus on the logic and structure of their solution without getting bogged down in language-specific details.
The Benefits of Using Pseudocode
Incorporating pseudocode into your problem-solving process offers numerous advantages:
- Clarity of Thought: Writing pseudocode forces you to think through your solution step-by-step, helping to clarify your thoughts and identify potential issues early in the development process.
- Language Independence: Since pseudocode isn’t tied to any specific programming language, it allows you to plan your solution without worrying about syntax or language-specific constructs.
- Easier Collaboration: Pseudocode can be easily understood by other developers, making it an excellent tool for team collaboration and code reviews.
- Faster Development: By planning your solution in pseudocode first, you can often write actual code more quickly and with fewer errors.
- Improved Documentation: Pseudocode can serve as a form of documentation, helping others (or your future self) understand the logic behind your code.
How to Write Effective Pseudocode
While there’s no strict set of rules for writing pseudocode, following some best practices can help you create clear and effective pseudocode:
1. Use Clear and Concise Language
Write your pseudocode in plain, easy-to-understand language. Avoid jargon or overly technical terms unless necessary. The goal is to make your logic as clear as possible.
2. Be Consistent
Develop a consistent style for your pseudocode. This might include using specific keywords for common operations (e.g., “IF”, “ELSE”, “WHILE”, “FOR”) or maintaining a consistent indentation style.
3. Use Appropriate Level of Detail
Strike a balance between being too vague and too specific. Your pseudocode should provide enough detail to guide your coding process without getting bogged down in implementation details.
4. Include Input and Output
Clearly define the inputs your algorithm expects and the outputs it should produce. This helps set clear boundaries for your solution.
5. Break Down Complex Problems
Use pseudocode to break complex problems into smaller, more manageable sub-problems. This can help you tackle difficult issues more effectively.
Pseudocode Examples
Let’s look at some examples of pseudocode to see how these principles can be applied in practice.
Example 1: Finding the Maximum Number in an Array
FUNCTION find_max(numbers):
IF length of numbers is 0:
RETURN null
max = first element of numbers
FOR each number in numbers:
IF number > max:
max = number
RETURN max
This pseudocode outlines a simple algorithm for finding the maximum number in an array. Notice how it uses clear, concise language and a consistent structure to describe the logic.
Example 2: Binary Search Algorithm
FUNCTION binary_search(sorted_array, target):
left = 0
right = length of sorted_array - 1
WHILE left <= right:
mid = (left + right) / 2
IF sorted_array[mid] == target:
RETURN mid
ELSE IF sorted_array[mid] < target:
left = mid + 1
ELSE:
right = mid - 1
RETURN -1 // Target not found
This example demonstrates how pseudocode can be used to describe a more complex algorithm like binary search. The logic is broken down into clear steps, making it easier to understand and implement.
Common Pseudocode Constructs
While pseudocode is flexible, there are some common constructs that you’ll often use:
1. Sequence
A sequence is a series of steps to be executed in order. In pseudocode, you can simply list these steps one after another:
STEP 1: Do this
STEP 2: Then do that
STEP 3: Finally, do this
2. Selection (If-Then-Else)
Selection structures allow your algorithm to make decisions based on certain conditions:
IF condition is true:
Do this
ELSE:
Do that
3. Iteration (Loops)
Iteration allows you to repeat a set of instructions multiple times. Common loop structures include:
FOR each item in collection:
Do something with item
WHILE condition is true:
Do something
4. Functions/Procedures
Functions or procedures in pseudocode can be represented as follows:
FUNCTION name_of_function(parameters):
Do something
RETURN result
Pseudocode vs. Flowcharts
While pseudocode is a textual representation of an algorithm, flowcharts provide a visual representation. Both tools have their place in problem-solving and algorithm design:
- Pseudocode is typically more detailed and can more easily represent complex logic. It’s often quicker to write and modify than flowcharts.
- Flowcharts provide a visual overview of the algorithm, which can be helpful for understanding the overall flow and structure at a glance.
Many developers use both tools in conjunction: flowcharts for high-level planning and communication, and pseudocode for more detailed algorithm design.
From Pseudocode to Code
Once you’ve developed your solution in pseudocode, translating it into actual code becomes much easier. Here’s a general process you can follow:
- Review Your Pseudocode: Make sure your pseudocode covers all aspects of the problem and that the logic is sound.
- Choose Your Programming Language: Select the appropriate language for your project.
- Translate Step by Step: Go through your pseudocode line by line, translating each step into the syntax of your chosen language.
- Handle Language-Specific Details: As you translate, you’ll need to address language-specific details like variable declarations, function signatures, etc.
- Test and Debug: Once you’ve translated your pseudocode, test your actual code to ensure it works as expected. Debug any issues that arise.
Let’s see an example of how pseudocode can be translated into actual code. We’ll use the “find maximum number” algorithm from earlier and translate it into Python:
Pseudocode:
FUNCTION find_max(numbers):
IF length of numbers is 0:
RETURN null
max = first element of numbers
FOR each number in numbers:
IF number > max:
max = number
RETURN max
Python code:
def find_max(numbers):
if len(numbers) == 0:
return None
max_num = numbers[0]
for num in numbers:
if num > max_num:
max_num = num
return max_num
As you can see, the translation from pseudocode to actual code is quite straightforward. The logical structure remains the same, with only syntax-specific changes needed.
Common Pitfalls in Writing Pseudocode
While pseudocode is a powerful tool, there are some common mistakes to avoid:
- Being Too Vague: Pseudocode that’s too high-level may not provide enough guidance when it comes time to write actual code.
- Being Too Detailed: On the flip side, pseudocode that includes too many implementation details can defeat the purpose of using pseudocode in the first place.
- Ignoring Edge Cases: Make sure your pseudocode accounts for potential edge cases and error conditions.
- Lack of Structure: Even though pseudocode is informal, it should still have a clear structure that mirrors the flow of your algorithm.
- Inconsistent Style: Using inconsistent terminology or structure can make your pseudocode harder to read and understand.
Pseudocode in Professional Development
In professional software development, pseudocode plays a crucial role in various stages of the development process:
1. Planning and Design
During the planning phase of a project, developers often use pseudocode to sketch out potential solutions and discuss different approaches with team members.
2. Documentation
Pseudocode can serve as a form of documentation, providing a high-level overview of how a particular algorithm or system works.
3. Code Reviews
When reviewing complex algorithms or logic, reviewers might ask for pseudocode to better understand the intended behavior before diving into the actual code.
4. Interview Process
Many technical interviews involve writing pseudocode to solve problems, as it allows interviewers to assess a candidate’s problem-solving skills without getting bogged down in language-specific details.
Tools and Resources for Pseudocode
While pseudocode can be written with any text editor, there are some tools and resources that can enhance your pseudocode writing experience:
- PseudoCode.io: An online pseudocode editor with syntax highlighting.
- Flowchart Makers: Tools like Lucidchart or Draw.io can be used to create flowcharts alongside your pseudocode.
- IDEs with Pseudocode Support: Some IDEs, like PyCharm, offer plugins for pseudocode syntax highlighting.
- Online Courses: Platforms like Coursera and edX offer courses on algorithm design that often cover pseudocode writing.
Conclusion
Mastering the art of pseudocode is a valuable skill for any programmer or problem solver. It provides a bridge between the conceptual understanding of a problem and its implementation in code, allowing for clearer thinking, better communication, and more efficient development processes.
By breaking down complex problems into manageable steps, pseudocode helps demystify the problem-solving process and makes tackling difficult challenges less daunting. Whether you’re a beginner just starting out in programming or an experienced developer working on complex systems, incorporating pseudocode into your workflow can significantly enhance your problem-solving capabilities.
Remember, the goal of pseudocode is to clarify your thinking and communicate your ideas effectively. Don’t get too caught up in the specifics of syntax or style – focus on clearly expressing the logic and structure of your solution. With practice, you’ll find that pseudocode becomes an invaluable tool in your programming toolkit, helping you to solve problems more efficiently and effectively.
So the next time you’re faced with a challenging programming problem, take a step back, grab a pen and paper (or open your favorite text editor), and start sketching out your solution in pseudocode. You might be surprised at how much clearer the path forward becomes.