The Art of Naming Variables After Your Ex-Lovers: A Programmer’s Guide to Emotional Coding
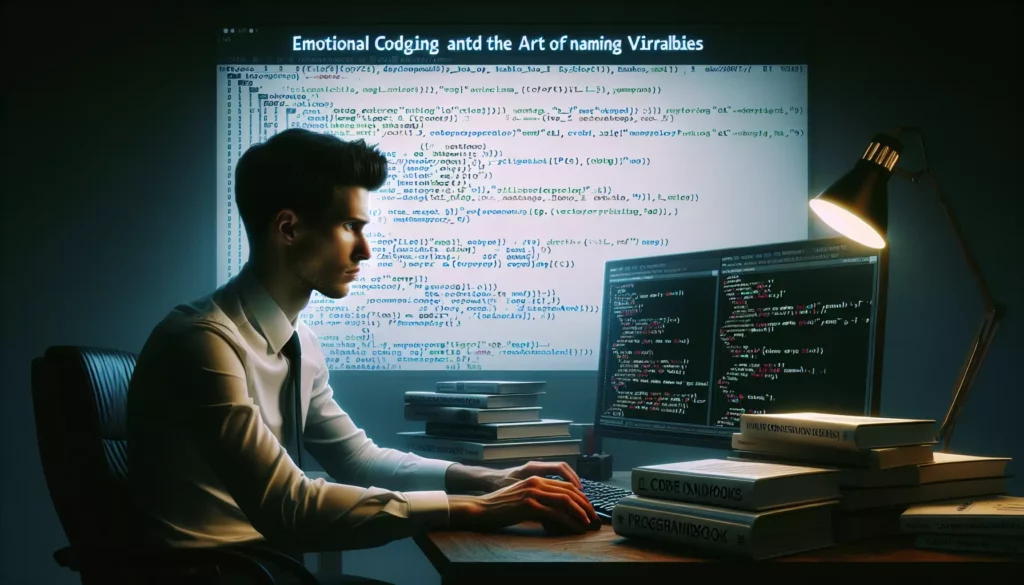
Welcome to AlgoCademy’s most unconventional tutorial yet! Today, we’re diving into the hilarious and slightly controversial world of naming variables after your ex-lovers. While this practice isn’t recommended for professional settings, it can be a fun way to add a personal touch to your coding projects and perhaps work through some lingering emotions. So, grab your favorite breakup playlist, a pint of ice cream, and let’s explore the art of emotionally charged variable naming!
1. The Importance of Variable Naming in Programming
Before we delve into the comedic aspects of naming variables after exes, let’s briefly discuss why variable naming is crucial in programming:
- Readability: Well-named variables make your code easier to understand.
- Maintainability: Clear variable names help other developers (or future you) maintain the code.
- Debugging: Descriptive names can make identifying and fixing bugs easier.
- Documentation: Good variable names can serve as a form of self-documentation.
Now, let’s see how we can creatively (and humorously) apply these principles while naming variables after our ex-lovers.
2. The Ex-Lover Naming Convention
When naming variables after your exes, consider the following guidelines:
- Use their name or a variation of it as the base of the variable name.
- Incorporate a trait or memory associated with them.
- Keep it PG (remember, someone else might read your code someday).
- Follow your language’s naming conventions (camelCase, snake_case, etc.).
Let’s look at some examples:
// JavaScript
let sarahTheHeartbreaker = true;
const tomAlwaysLate = 30; // minutes
var emilyStonewaller = null;
# Python
alice_never_calls = False
bob_the_snorer = 85 # decibels
carol_indecisive = None
// Java
boolean gregTheFlakyOne = true;
int oliviaMessagesSeen = 0;
String davidBrokenPromises = "I'll change, I swear";
3. Emotional Data Types: Choosing the Right Type for Your Ex
Different data types can represent various aspects of your past relationships. Let’s explore how we can match data types to our exes’ personalities or behaviors:
3.1. Booleans: The Black and White Exes
Use booleans for exes who were very straightforward or had a tendency to see things in binary terms.
// JavaScript
let jackAllOrNothing = true;
const lisaHotAndCold = false;
# Python
emma_yes_or_no = True
ryan_in_or_out = False
// Java
boolean hannahCommitmentPhobe = false;
boolean mikeAlwaysRight = true;
3.2. Integers: Quantifying Your Ex’s Quirks
Integers are perfect for representing countable traits or habits of your exes.
// JavaScript
let sophiaCatCount = 7;
const alexLateMinutes = 45;
# Python
jake_forgotten_anniversaries = 3
lucy_mood_swings_per_day = 5
// Java
int oliverUnreturnnedTexts = 17;
int rachelShoeCollection = 142;
3.3. Floating-Point Numbers: For the Exes Who Weren’t Quite Whole
Use floating-point numbers for exes who were a bit wishy-washy or never quite gave their all.
// JavaScript
let ethanCommitmentLevel = 0.5;
const zoeLoyaltyPercentage = 75.5;
# Python
charlie_attention_span = 0.3
diana_trust_factor = 0.99
// Java
double frankEmotionalAvailability = 0.25;
float graceReliabilityIndex = 0.8f;
3.4. Strings: Capturing Their Memorable (or Not So Memorable) Words
Strings are perfect for preserving those unforgettable (or regrettable) quotes from your exes.
// JavaScript
let samLastWords = "It's not you, it's me";
const kateFavoriteExcuse = "I'm just really busy right now";
# Python
leo_classic_line = "We need to talk"
ivy_breakup_text = "I think we should see other people"
// Java
String maxEmptyPromise = "I'll call you tomorrow";
String claireClassicComeback = "Whatever, I never liked you anyway";
3.5. Arrays: For the Exes with Multiple Personalities
Arrays can represent exes who had many facets to their personality or a list of their traits.
// JavaScript
let benMoodSwings = ["happy", "angry", "jealous", "apologetic"];
const meganHobbies = ["yoga", "ghosting", "Instagram", "avocado toast"];
# Python
noah_excuses = ["traffic", "alarm didn't go off", "dog ate my phone"]
ava_favorite_breakup_spots = ["coffee shop", "park bench", "via text"]
// Java
String[] liamPersonalities = {"charming", "moody", "mysterious", "absent"};
int[] zoeRelationshipStages = {1, 2, 3, 2, 1, 0}; // 0 is breakup
3.6. Objects: For the Complex Exes
Objects (or dictionaries in Python) are ideal for exes with multiple attributes you want to capture.
// JavaScript
let taylor = {
looks: 9,
personality: 3,
cookingSkills: -1,
excuses: ["working late", "family emergency", "alien abduction"]
};
# Python
jordan = {
"charm_level": 8,
"commitment_phobia": True,
"red_flags_ignored": 15,
"favorite_line": "Let's just be friends"
}
// Java
public class ExLover {
public String name = "Casey";
public int dramaLevel = 11;
public boolean callsAtMidnight = true;
public String[] moodSwings = {"ecstatic", "furious", "indifferent"};
}
4. Function Names: Encapsulating Your Ex’s Behaviors
Functions can represent actions or behaviors associated with your exes. This is where you can really get creative!
// JavaScript
function alexGhostsAgain() {
return "Read";
}
function sarahChangesHerMind(decision) {
return !decision;
}
# Python
def chris_makes_empty_promises():
return "I'll definitely be there this time"
def emily_starts_unnecessary_drama(peaceful_day):
return not peaceful_day
// Java
public boolean jasonForgetsBirthday(int month, int day) {
return true; // He always forgets
}
public String oliviaSendsMixedSignals(String mood) {
return mood.equals("interested") ? "not interested" : "interested";
}
5. Error Handling: Dealing with Relationship Bugs
Error handling in programming can humorously parallel how we deal with relationship issues. Let’s look at some examples:
// JavaScript
try {
letsTryAgainWithJessica();
} catch (heartbreakError) {
console.log("Should have known better");
} finally {
moveOn();
}
# Python
try:
trust_mark_one_last_time()
except TrustIssues as ti:
print(f"Trust broken again: {ti}")
block_number()
else:
print("Miracle occurred")
finally:
focus_on_self()
// Java
try {
giveKarenAnotherChance();
} catch (UnreliableException ue) {
System.out.println("Karen flaked again: " + ue.getMessage());
} catch (DramaOverflowException doe) {
System.out.println("Too much drama to handle");
} finally {
deleteNumber();
hitTheGym();
}
6. Comments: Documenting the Relationship History
Comments in your code can serve as a humorous diary of your past relationships:
// JavaScript
// TODO: Figure out why I keep falling for Tina's tricks
let tinaCharmLevel = 100;
// FIXME: David's reliability is broken
const davidShowsUpOnTime = false;
# Python
# NOTE: Emma's mood swings are unpredictable. Proceed with caution.
emma_current_mood = random.choice(["happy", "angry", "hangry"])
# WARNING: Jake's promises have a high failure rate
jake_promise = "I'll be different this time"
// Java
// HACK: Temporarily boosting Megan's communication skills
int meganTextResponseTime = Integer.MAX_VALUE / 2;
/*
* The Great Sophia Experiment
* Attempt #37 to make a relationship work
* Results pending...
*/
boolean sophiaExperimentSuccess = false;
7. The Ethical Considerations and Professional Boundaries
While this tutorial has been a humorous exploration of variable naming, it’s crucial to address the ethical considerations and maintain professional boundaries in real-world coding practices:
- Workplace Appropriateness: Never use this naming convention in professional or collaborative projects. It’s unprofessional and could create a hostile work environment.
- Code Readability: In practice, variable names should be clear, concise, and related to their function in the program.
- Emotional Health: While coding can be a creative outlet, it’s important to process emotions in healthy ways. If you’re struggling with a breakup, consider talking to friends, family, or a therapist instead of embedding your feelings in code.
- Privacy Concerns: Even in personal projects, be mindful of privacy. Using real names or identifiable information could be problematic if the code ever becomes public.
- Maintaining Professionalism: As you progress in your coding journey, cultivate a professional naming convention that will serve you well in your career.
8. Conclusion: Compiling Your Emotional Code
We’ve journeyed through the humorous landscape of naming variables after ex-lovers, exploring various data types, functions, and even error handling to represent the complexities of past relationships. While this approach can be a fun way to practice coding and perhaps add a little levity to the learning process, it’s crucial to remember that it’s not suitable for professional environments.
As you continue your coding education with AlgoCademy, focus on developing clear, descriptive, and professional naming conventions. These skills will be invaluable as you progress from beginner-level coding to tackling complex algorithms and preparing for technical interviews at top tech companies.
Remember, the true art of variable naming lies in creating code that is readable, maintainable, and effectively communicates its purpose. As you practice and grow, you’ll develop a coding style that is uniquely yours – no exes required!
Happy coding, and may your future relationships be as bug-free as your code!
9. Further Learning Resources
To continue your journey in mastering the art of coding (with more professional naming conventions), check out these AlgoCademy resources:
- Clean Code Practices: Writing Maintainable and Readable Code
- Mastering Variable Naming Conventions Across Different Programming Languages
- Advanced Error Handling Techniques for Robust Applications
- Preparing for Technical Interviews: Best Practices and Common Pitfalls
- Emotional Intelligence in Programming: Balancing Creativity and Professionalism
Remember, at AlgoCademy, we’re here to guide you from your first lines of code to landing your dream job at a top tech company. Keep practicing, stay curious, and never stop learning!