The Art of Building a Portfolio Website That’s Never Quite Finished
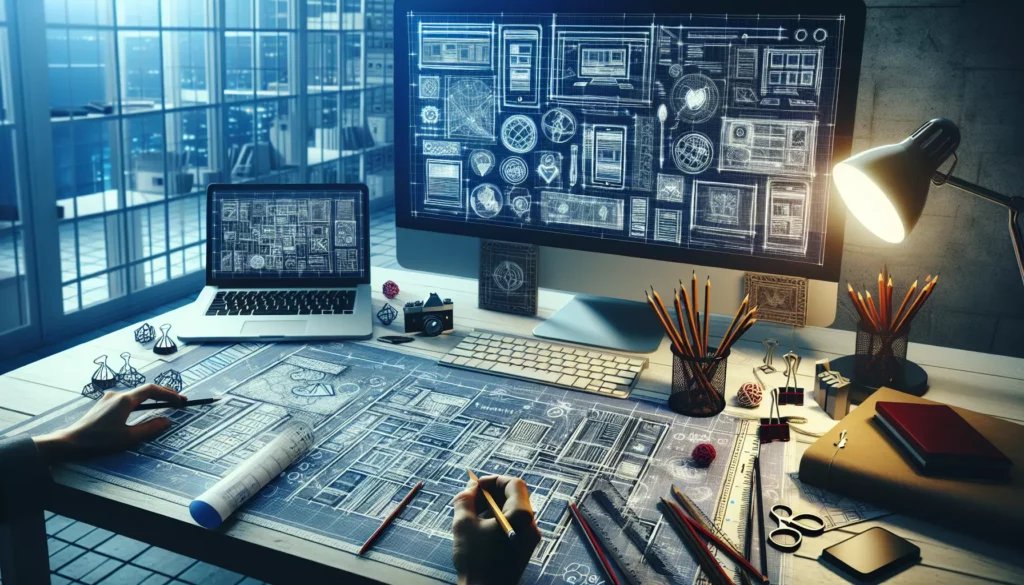
As aspiring developers and coding enthusiasts, we often hear about the importance of having a portfolio website. It’s our digital calling card, a showcase of our skills, and a testament to our journey in the world of programming. But here’s a secret that seasoned developers know all too well: a portfolio website is never truly finished. In this comprehensive guide, we’ll explore the art of building a portfolio website that evolves with you, reflecting your growth and adapting to the ever-changing landscape of web development.
Why Your Portfolio Website Should Be a Work in Progress
Before we dive into the nitty-gritty of building a portfolio, let’s address the elephant in the room: why should your portfolio website be in a constant state of flux?
- Reflection of Growth: As you learn new skills and complete new projects, your portfolio should showcase your latest achievements.
- Keeping Up with Trends: Web design and development trends change rapidly. An evolving portfolio demonstrates that you’re keeping pace with industry standards.
- Experimentation Ground: Your portfolio is the perfect place to try out new technologies and techniques you’re learning.
- Continuous Learning: The process of updating your portfolio reinforces your skills and encourages you to learn more.
Starting with a Solid Foundation
While your portfolio will be ever-changing, it’s crucial to start with a strong foundation. Here are the key elements to consider:
1. Choose Your Technology Stack
Selecting the right technologies for your portfolio is crucial. Consider these options:
- HTML, CSS, and JavaScript: The tried-and-true trio for static websites.
- React, Vue, or Angular: For a more dynamic and interactive experience.
- Static Site Generators: Like Gatsby or Next.js for performance and ease of updates.
- Content Management Systems: WordPress or Contentful for easy content management.
Remember, the best choice depends on your skills and the complexity you want to showcase.
2. Design with Flexibility in Mind
Create a design that’s easy to update and expand. Consider these principles:
- Use a modular design approach
- Implement a responsive layout
- Choose a color scheme and typography that can grow with you
- Plan for scalability from the start
3. Essential Sections to Include
While your portfolio will evolve, certain sections should be present from the beginning:
- About Me: A brief introduction to who you are and what you do.
- Skills: A list or visual representation of your technical skills.
- Projects: Showcase your best work with descriptions and links.
- Contact Information: Make it easy for potential employers or clients to reach you.
- Blog or Articles: (Optional) A space for sharing your thoughts and knowledge.
Building for Continuous Improvement
Now that we have our foundation, let’s explore strategies to ensure your portfolio remains a living, breathing representation of your skills.
1. Implement a Version Control System
Using Git and hosting your portfolio on platforms like GitHub or GitLab offers several advantages:
- Track changes over time
- Easily revert to previous versions if needed
- Collaborate with others or get feedback on your code
- Showcase your Git skills to potential employers
Here’s a simple example of how to initialize a Git repository for your portfolio:
cd /path/to/your/portfolio
git init
git add .
git commit -m "Initial commit of my portfolio website"
git remote add origin https://github.com/yourusername/portfolio.git
git push -u origin master
2. Automate Deployment with CI/CD
Set up a Continuous Integration/Continuous Deployment (CI/CD) pipeline to automatically deploy your changes. This not only saves time but also demonstrates your DevOps skills. Popular options include:
- GitHub Actions
- GitLab CI/CD
- Netlify
- Vercel
Here’s a basic GitHub Actions workflow for deploying a static site:
name: Deploy Portfolio
on:
push:
branches: [ main ]
jobs:
build-and-deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Use Node.js
uses: actions/setup-node@v2
with:
node-version: '14.x'
- run: npm ci
- run: npm run build
- name: Deploy to GitHub Pages
uses: peaceiris/actions-gh-pages@v3
with:
github_token: ${{ secrets.GITHUB_TOKEN }}
publish_dir: ./build
3. Implement a Content Management System (CMS)
A headless CMS can make updating your portfolio’s content much easier. Some popular options include:
- Contentful
- Strapi
- Sanity.io
By separating your content from your code, you can update your projects and skills without touching the codebase.
4. Use Component-Based Architecture
Whether you’re using a framework like React or vanilla JavaScript, structuring your portfolio with reusable components makes it easier to add, remove, or modify sections. For example, in React:
import React from 'react';
const ProjectCard = ({ title, description, link }) => (
<div className="project-card">
<h3>{title}</h3>
<p>{description}</p>
<a href={link}>View Project</a>
</div>
);
const ProjectsSection = ({ projects }) => (
<section id="projects">
<h2>My Projects</h2>
{projects.map(project => (
<ProjectCard key={project.id} {...project} />
))}
</section>
);
export default ProjectsSection;
Strategies for Continuous Improvement
With the technical foundation in place, let’s explore strategies to keep your portfolio fresh and relevant.
1. Set Regular Update Schedules
Commit to updating your portfolio at regular intervals:
- Monthly: Add new skills or minor project updates
- Quarterly: Add new projects or major skill improvements
- Annually: Perform a comprehensive review and redesign if necessary
2. Incorporate Learning Progress
As you progress through coding challenges on platforms like AlgoCademy, update your portfolio to reflect your growth:
- Add a section showcasing algorithms you’ve mastered
- Include badges or certificates from completed courses
- Link to your AlgoCademy profile to show your problem-solving skills
3. Experiment with New Technologies
Use your portfolio as a playground for new tech:
- Implement a new CSS framework or try CSS-in-JS
- Add interactive elements with libraries like Three.js or D3.js
- Experiment with serverless functions for dynamic content
4. Seek and Incorporate Feedback
Regularly ask for feedback from peers, mentors, and potential employers:
- Share your portfolio on developer forums and social media
- Attend networking events and get opinions from industry professionals
- Use analytics tools to understand how visitors interact with your site
Advanced Techniques for a Dynamic Portfolio
As you become more comfortable with maintaining your portfolio, consider these advanced techniques to make it truly stand out.
1. Implement Dark Mode
Adding a dark mode option not only looks cool but also demonstrates your CSS skills. Here’s a simple implementation using CSS variables:
/* In your CSS file */
:root {
--background-color: white;
--text-color: black;
}
@media (prefers-color-scheme: dark) {
:root {
--background-color: #1a1a1a;
--text-color: white;
}
}
body {
background-color: var(--background-color);
color: var(--text-color);
}
/* In your JavaScript file */
const toggleDarkMode = () => {
document.body.classList.toggle('dark-mode');
};
2. Add Internationalization (i18n)
If you’re targeting an international audience, consider adding multiple language support. React-intl is a great library for this:
import React from 'react';
import { FormattedMessage } from 'react-intl';
const Greeting = () => (
<h1>
<FormattedMessage
id="app.greeting"
defaultMessage="Welcome to my portfolio!"
/>
</h1>
);
3. Implement Microinteractions
Small, subtle animations can greatly enhance user experience. Consider adding microinteractions to your portfolio:
- Hover effects on buttons and links
- Loading animations for dynamic content
- Scroll-triggered animations for section entries
Here’s a simple CSS animation for a button hover effect:
.button {
transition: transform 0.3s ease;
}
.button:hover {
transform: scale(1.05);
}
4. Integrate a Blog or Tutorial Section
Sharing your knowledge not only helps others but also positions you as an expert in your field. Consider adding a blog or tutorial section to your portfolio. You can use static site generators like Gatsby or Next.js to easily integrate a blog into your portfolio site.
Measuring Success and Iterating
As you continue to evolve your portfolio, it’s important to measure its effectiveness and make data-driven decisions for improvements.
1. Implement Analytics
Use tools like Google Analytics or Plausible to track visitor behavior:
- Monitor page views and time spent on each section
- Track clicks on project links and contact information
- Analyze user flow to optimize the layout
2. A/B Testing
Experiment with different layouts, color schemes, or content organization:
- Use tools like Google Optimize for simple A/B tests
- Test different versions of your project descriptions
- Experiment with various call-to-action placements
3. Collect User Feedback
Implement a feedback mechanism on your portfolio:
- Add a simple contact form for visitors to leave comments
- Use tools like Hotjar for heatmaps and user recordings
- Conduct user interviews with peers or mentors
Conclusion: Embracing the Journey
Building a portfolio website that’s never quite finished is not just about showcasing your work—it’s about embracing the journey of continuous learning and improvement. Your portfolio is a living document of your growth as a developer, a playground for new ideas, and a reflection of your evolving skills.
Remember, the goal is not perfection, but progress. Each update, no matter how small, is a step forward in your development journey. By treating your portfolio as a constant work in progress, you’re not just building a website—you’re cultivating a mindset of lifelong learning that will serve you well throughout your career.
As you continue to learn and grow with platforms like AlgoCademy, let your portfolio be a testament to your dedication and passion for coding. Embrace the challenges, celebrate the victories, and most importantly, enjoy the process of creating a portfolio that’s as dynamic and evolving as you are.
Happy coding, and may your portfolio always be a work in progress!