The Art of Asking Good Questions to Improve Your Learning
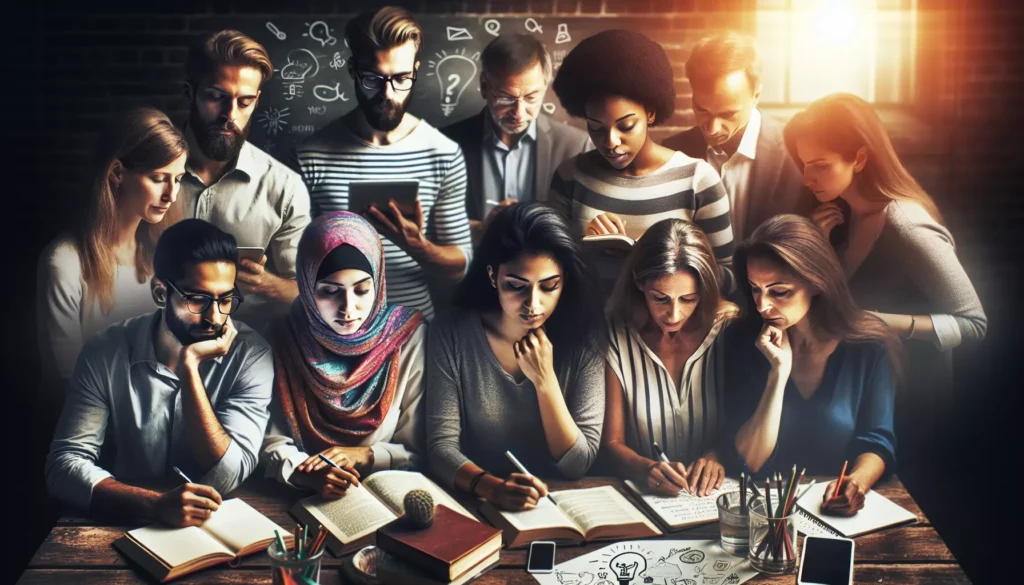
In the world of coding education and programming skills development, one of the most valuable tools at your disposal is the ability to ask good questions. Whether you’re a beginner just starting your journey or an experienced developer preparing for technical interviews at major tech companies, mastering the art of inquiry can significantly accelerate your learning process and problem-solving abilities. In this comprehensive guide, we’ll explore the importance of asking good questions, techniques for formulating effective queries, and how this skill can enhance your coding education and career prospects.
Why Asking Good Questions Matters in Coding Education
Before diving into the specifics of how to ask good questions, it’s crucial to understand why this skill is particularly important in the context of coding education and programming skills development.
1. Clarifying Complex Concepts
Programming often involves abstract concepts and intricate logic. By asking well-formulated questions, you can break down these complexities into more manageable pieces, making it easier to grasp and internalize new information.
2. Debugging and Problem-Solving
When faced with a coding challenge or a bug in your program, asking the right questions can help you identify the root cause more quickly. This skill is essential for efficient debugging and problem-solving, which are core competencies for any programmer.
3. Enhancing Collaboration
In the world of software development, collaboration is key. The ability to ask good questions allows you to communicate more effectively with your peers, mentors, or instructors, leading to more productive learning experiences and teamwork.
4. Preparing for Technical Interviews
Many technical interviews, especially those at major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, and Google), involve problem-solving scenarios where asking clarifying questions is not only allowed but encouraged. Demonstrating this skill can set you apart as a candidate who thinks critically and communicates effectively.
Techniques for Formulating Effective Questions
Now that we understand the importance of asking good questions, let’s explore some techniques for formulating effective queries in the context of coding education and programming skills development.
1. Be Specific and Concise
When asking a question, aim to be as specific and concise as possible. Instead of asking a broad question like “How do I use arrays?”, try something more focused such as “What’s the most efficient way to find the maximum value in an unsorted array in Python?”
Example:
// Instead of:
"How do I work with strings?"
// Try:
"What's the difference between String and StringBuilder in Java, and when should I use each?"
2. Provide Context
When seeking help with a coding problem, provide relevant context. This includes the programming language you’re using, any error messages you’re encountering, and what you’ve already tried. This context helps others understand your situation better and provide more targeted assistance.
Example:
"I'm trying to implement a binary search tree in C++. Here's my current code:
[Insert your code snippet here]
I'm getting a segmentation fault when I try to insert more than 5 elements. I've already checked that my pointers are initialized correctly. What else should I look for?"
3. Use the Rubber Duck Method
Before asking others, try explaining your problem to an inanimate object, like a rubber duck. This technique, known as “rubber duck debugging,” often helps you clarify your thoughts and sometimes even solve the problem on your own.
4. Follow the XY Problem Principle
The XY problem occurs when you ask about your attempted solution (Y) rather than your actual problem (X). To avoid this, start by explaining the problem you’re trying to solve, not just the issue with your current approach.
Example:
// Instead of:
"How do I convert a string to an integer in JavaScript without using parseInt()?"
// Try:
"I need to perform arithmetic operations on numeric data that's currently stored as strings. What's the most efficient way to handle this in JavaScript?"
5. Use the STAR Method
When asking for help with a coding challenge or algorithm problem, use the STAR method:
- Situation: Describe the coding task or problem you’re facing.
- Task: Explain what you’re trying to achieve.
- Action: Detail the steps you’ve taken so far.
- Result: Share the outcome of your actions, including any errors or unexpected results.
Example:
"Situation: I'm working on a LeetCode problem that asks to find the longest palindromic substring in a given string.
Task: I need to implement an efficient solution that works for strings up to 1000 characters long.
Action: I've tried using a brute force approach, checking all possible substrings, but it's too slow for larger inputs.
Result: My current solution times out on test cases with strings longer than 100 characters. How can I optimize this algorithm?"
Leveraging Good Questions in Different Learning Scenarios
Let’s explore how the art of asking good questions can be applied in various learning scenarios within the context of coding education and programming skills development.
1. Interactive Coding Tutorials
Many platforms, including AlgoCademy, offer interactive coding tutorials. When working through these tutorials, don’t hesitate to ask questions that go beyond the immediate task at hand. For example:
"I understand how to implement a basic linked list, but how would this data structure be used in a real-world application? Can you provide an example?"
This type of question helps you connect theoretical knowledge with practical applications, enhancing your overall understanding.
2. AI-Powered Assistance
When using AI-powered coding assistants, formulate your questions to maximize the value of the AI’s capabilities. For instance:
"Can you explain the time and space complexity of this quicksort implementation? Also, are there any edge cases where its performance might degrade significantly?"
This question not only asks for an explanation but also prompts the AI to provide insights about potential pitfalls, helping you develop a more nuanced understanding of the algorithm.
3. Peer Learning and Forums
When engaging with peers or in coding forums, ask questions that invite discussion and multiple perspectives. For example:
"I've implemented a solution for the 'Two Sum' problem using a hash map, which has O(n) time complexity. Are there any other approaches that might be more memory-efficient, even if they sacrifice some time efficiency?"
This type of question can spark interesting discussions about trade-offs in algorithm design, exposing you to diverse viewpoints and approaches.
4. Technical Interview Preparation
When preparing for technical interviews, especially for major tech companies, practice asking clarifying questions about the problem statements. For instance:
"For this graph traversal problem, should I assume the graph is connected? Also, is there a preference for depth-first search over breadth-first search in this context?"
Such questions demonstrate your ability to think critically about problem constraints and consider different approaches, skills highly valued in technical interviews.
Common Pitfalls to Avoid When Asking Questions
While learning to ask good questions, be aware of these common pitfalls:
1. Asking Without Research
Before asking a question, make sure you’ve done some basic research. Many coding questions can be answered by reading documentation or searching online resources. Showing that you’ve put in effort before asking demonstrates initiative and respect for others’ time.
2. Asking Overly Broad Questions
Avoid asking questions that are too general or open-ended. For example, “How do I become a good programmer?” is too broad. Instead, ask more focused questions like “What are some effective strategies for improving my algorithmic thinking skills?”
3. Not Providing Enough Context
Failing to provide necessary context can lead to misunderstandings and unhelpful responses. Always include relevant details such as the programming language, framework, or specific problem you’re working on.
4. Asking Multiple Questions at Once
Avoid bundling multiple unrelated questions into a single query. This can overwhelm respondents and result in partial or confusing answers. Instead, focus on one specific issue at a time.
5. Not Following Up
If someone takes the time to answer your question, make sure to follow up. Let them know if their answer helped or if you need further clarification. This not only shows appreciation but also helps refine your understanding.
Developing a Question-Asking Mindset
To truly master the art of asking good questions, it’s important to develop a question-asking mindset. This involves cultivating curiosity, embracing a growth mindset, and viewing questions as opportunities for learning rather than admissions of ignorance.
1. Cultivate Curiosity
Approach your coding education with genuine curiosity. Don’t just accept things at face value; always seek to understand the “why” behind concepts and practices. For example, when learning about different sorting algorithms, you might ask:
"Why does quicksort generally perform better than merge sort in practice, even though they have the same average time complexity?"
2. Embrace a Growth Mindset
View challenges and gaps in your knowledge as opportunities for growth rather than personal shortcomings. This mindset encourages you to ask questions without fear of judgment. For instance:
"I'm struggling to understand the concept of recursion. Could you recommend some exercises or real-world examples that might help me grasp it better?"
3. Practice Active Listening
When receiving explanations or instructions, practice active listening. This involves fully concentrating, understanding, responding, and then remembering what is being said. Follow up with questions that demonstrate your engagement and desire to understand deeply:
"If I understand correctly, a hash table provides average O(1) time complexity for insertions and lookups. Are there any scenarios where this performance might degrade significantly?"
4. Reflect on Your Learning Process
Regularly reflect on your learning process and identify areas where you might need more clarity. Use these reflections to formulate questions that address your specific learning needs. For example:
"I notice that I often struggle with dynamic programming problems. Are there any specific strategies or mental frameworks I can use to approach these types of problems more effectively?"
Leveraging Questions for Career Development
As you progress in your coding education and prepare for a career in software development, the ability to ask good questions becomes increasingly valuable. Here’s how you can leverage this skill for career development:
1. During Job Interviews
Use thoughtful questions to demonstrate your interest and engagement during job interviews. For example:
"Could you tell me more about how your team approaches continuous integration and deployment? I'm particularly interested in learning about any unique challenges you've faced in implementing these practices."
2. In Professional Development Conversations
When discussing your career path with mentors or managers, ask questions that show your commitment to growth and your understanding of the industry. For instance:
"Given the current trends in cloud computing and microservices architecture, what skills do you think will be most valuable for a backend developer to focus on in the next 3-5 years?"
3. During Code Reviews
Use code reviews as an opportunity to learn from your peers by asking insightful questions about their code and design decisions:
"I noticed you used a factory pattern here. Could you explain the benefits of this approach over a more straightforward object instantiation in this specific context?"
4. In Technical Discussions
During team meetings or technical discussions, ask questions that demonstrate your ability to think critically about system design and architecture:
"As we scale our application, have we considered the potential bottlenecks in our current database schema? Would it be worth exploring a NoSQL solution for certain parts of our data model?"
Conclusion
The art of asking good questions is a powerful tool in your journey through coding education and programming skills development. It can help you clarify complex concepts, solve problems more efficiently, collaborate effectively with peers, and stand out in technical interviews. By applying the techniques discussed in this article and developing a question-asking mindset, you’ll not only enhance your learning experience but also set yourself up for success in your future career as a software developer.
Remember, there’s no such thing as a “stupid question” when it’s asked with genuine curiosity and a desire to learn. Embrace the power of inquiry, and watch as it transforms your approach to coding challenges and accelerates your growth as a programmer. Whether you’re working through interactive tutorials on platforms like AlgoCademy, preparing for technical interviews with major tech companies, or collaborating on complex projects, the ability to ask good questions will serve you well throughout your coding journey and beyond.
So, the next time you encounter a challenging concept or a tricky bug, pause for a moment. Instead of immediately seeking a solution, first ask yourself: “What’s the right question I should be asking here?” This simple shift in perspective can open up new avenues of understanding and problem-solving, propelling you forward in your coding education and career.