The Anatomy of an iOS Engineer Interview: A Comprehensive Guide
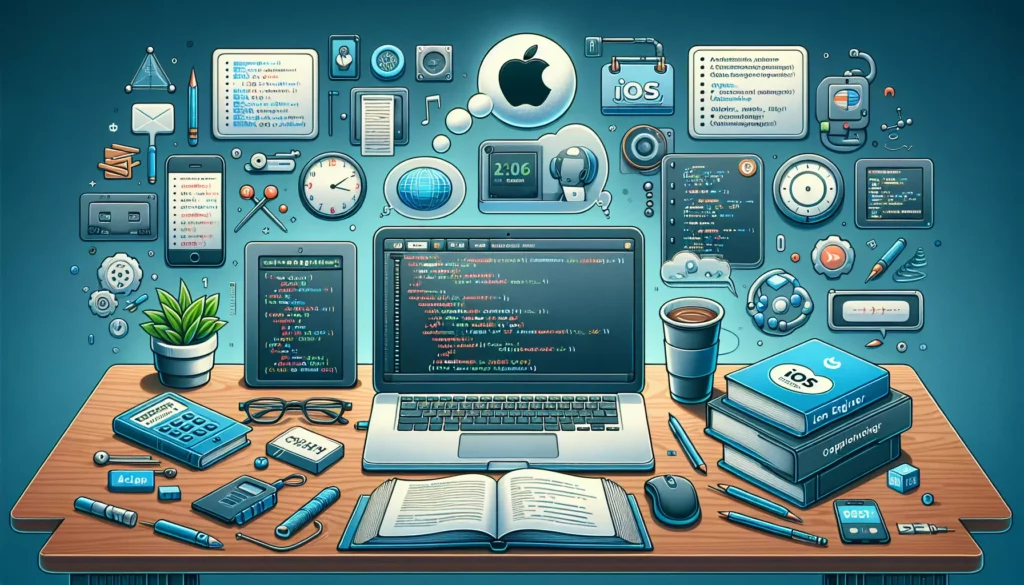
As the mobile app industry continues to thrive, iOS engineers are in high demand. If you’re aspiring to join the ranks of iOS developers at top tech companies, you’ll need to be well-prepared for the interview process. This comprehensive guide will walk you through the anatomy of an iOS engineer interview, providing insights into what to expect and how to prepare effectively.
Table of Contents
- Introduction
- The Structure of an iOS Engineer Interview
- Technical Skills Assessment
- Coding Challenges and Problem Solving
- iOS-Specific System Design Questions
- Behavioral Questions and Soft Skills
- Preparation Tips and Resources
- Common Mistakes to Avoid
- Post-Interview Process
- Conclusion
1. Introduction
The iOS engineer interview process is designed to assess your technical skills, problem-solving abilities, and cultural fit within the organization. Whether you’re interviewing at a startup or a FAANG company (Facebook, Amazon, Apple, Netflix, Google), understanding the structure and expectations of these interviews is crucial for success.
As an iOS engineer, you’ll be expected to demonstrate proficiency in Swift programming, iOS frameworks, and mobile app development best practices. Additionally, you should be prepared to showcase your ability to think critically, communicate effectively, and work collaboratively in a team environment.
2. The Structure of an iOS Engineer Interview
The interview process for iOS engineers typically consists of several stages:
- Initial Screening: This may involve a phone call or online assessment to evaluate your basic qualifications and interest in the position.
- Technical Phone Screen: A more in-depth discussion of your technical background and possibly some coding questions.
- Take-Home Project: Some companies may assign a small iOS project to assess your coding skills and style.
- On-Site Interviews: A series of face-to-face interviews (or virtual equivalents) that dive deep into your technical abilities, problem-solving skills, and cultural fit.
- Final Decision: The hiring team reviews all feedback and makes an offer or provides constructive feedback.
Each stage is designed to evaluate different aspects of your skills and potential as an iOS engineer. Let’s explore these components in more detail.
3. Technical Skills Assessment
During the technical portions of the interview, you can expect to be assessed on a wide range of iOS development skills. Some key areas include:
Swift Programming Language
You should be well-versed in Swift, including:
- Basic syntax and data types
- Object-oriented programming concepts
- Functional programming features
- Protocol-oriented programming
- Memory management and ARC (Automatic Reference Counting)
- Error handling
- Concurrency and multithreading
iOS Frameworks and APIs
Familiarity with core iOS frameworks is essential:
- UIKit for building user interfaces
- Core Data for data persistence
- Core Animation for creating smooth animations
- Core Location for location-based services
- Push Notifications
- Networking with URLSession
- SwiftUI (for more recent positions)
iOS App Architecture
Understanding common architectural patterns is crucial:
- MVC (Model-View-Controller)
- MVVM (Model-View-ViewModel)
- Clean Architecture
- VIPER (View-Interactor-Presenter-Entity-Router)
Testing and Debugging
You should be able to discuss:
- Unit testing with XCTest
- UI testing
- Debugging techniques using Xcode’s tools
- Performance profiling
App Store and Distribution
Knowledge of the app submission process is valuable:
- App Store guidelines
- Provisioning profiles and certificates
- Continuous integration and delivery (CI/CD) for iOS apps
4. Coding Challenges and Problem Solving
A significant portion of the iOS engineer interview will involve coding challenges. These are designed to assess your ability to write clean, efficient code and solve problems in real-time. Here are some types of challenges you might encounter:
Algorithm and Data Structure Problems
While not as extensive as for general software engineering roles, you may still face questions on:
- Array manipulation
- String processing
- Tree and graph traversals
- Dynamic programming
- Time and space complexity analysis
Here’s an example of a simple coding challenge you might encounter:
// Write a function that reverses a string in Swift
func reverseString(_ s: String) -> String {
return String(s.reversed())
}
// Test the function
let original = "Hello, World!"
let reversed = reverseString(original)
print(reversed) // Output: "!dlroW ,olleH"
iOS-Specific Coding Tasks
You may be asked to implement iOS-specific functionalities, such as:
- Creating a custom UIView subclass
- Implementing a table view with custom cells
- Fetching and parsing JSON data from an API
- Handling user input and form validation
- Implementing local data persistence
Here’s an example of a more iOS-specific coding task:
import UIKit
class CustomTableViewCell: UITableViewCell {
let titleLabel: UILabel = {
let label = UILabel()
label.font = UIFont.boldSystemFont(ofSize: 16)
label.translatesAutoresizingMaskIntoConstraints = false
return label
}()
let subtitleLabel: UILabel = {
let label = UILabel()
label.font = UIFont.systemFont(ofSize: 14)
label.textColor = .gray
label.translatesAutoresizingMaskIntoConstraints = false
return label
}()
override init(style: UITableViewCell.CellStyle, reuseIdentifier: String?) {
super.init(style: style, reuseIdentifier: reuseIdentifier)
setupViews()
}
required init?(coder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
private func setupViews() {
contentView.addSubview(titleLabel)
contentView.addSubview(subtitleLabel)
NSLayoutConstraint.activate([
titleLabel.topAnchor.constraint(equalTo: contentView.topAnchor, constant: 8),
titleLabel.leadingAnchor.constraint(equalTo: contentView.leadingAnchor, constant: 16),
titleLabel.trailingAnchor.constraint(equalTo: contentView.trailingAnchor, constant: -16),
subtitleLabel.topAnchor.constraint(equalTo: titleLabel.bottomAnchor, constant: 4),
subtitleLabel.leadingAnchor.constraint(equalTo: titleLabel.leadingAnchor),
subtitleLabel.trailingAnchor.constraint(equalTo: titleLabel.trailingAnchor),
subtitleLabel.bottomAnchor.constraint(equalTo: contentView.bottomAnchor, constant: -8)
])
}
func configure(title: String, subtitle: String) {
titleLabel.text = title
subtitleLabel.text = subtitle
}
}
This example demonstrates the creation of a custom table view cell with programmatic UI, which is a common task in iOS development.
Problem-Solving Approach
When tackling coding challenges, remember to:
- Clarify the problem and requirements
- Think out loud and communicate your thought process
- Consider edge cases and potential optimizations
- Write clean, readable code
- Test your solution with sample inputs
5. iOS-Specific System Design Questions
System design questions for iOS engineers often focus on mobile-specific architectures and patterns. You might be asked to design:
- A photo-sharing app with real-time updates
- A music streaming service with offline capabilities
- A ride-sharing app with location tracking
- A messaging app with end-to-end encryption
When approaching these questions, consider:
- User interface and experience design
- Data models and persistence strategies
- Network architecture and API design
- Offline functionality and data syncing
- Performance optimization and battery life considerations
- Security and privacy measures
- Scalability and maintainability
Here’s a high-level example of how you might approach designing a photo-sharing app:
// Photo-sharing App Architecture
1. User Interface:
- Tab-based navigation (Home, Explore, Camera, Notifications, Profile)
- Custom camera interface
- Infinite scrolling feed
- Image viewer with zoom capabilities
2. Data Models:
- User: id, username, email, profile_picture, etc.
- Post: id, user_id, image_url, caption, timestamp, likes, comments
- Comment: id, post_id, user_id, text, timestamp
3. Local Data Storage:
- Core Data for caching posts, user data, and offline access
- FileManager for storing captured images before upload
4. Networking:
- RESTful API for user authentication, post creation, and social interactions
- WebSocket for real-time notifications and feed updates
- Image upload using multipart form data
5. Key Features:
- Image filters and editing tools
- Geolocation tagging
- Hashtags and user mentions
- Push notifications for likes, comments, and follows
6. Performance Considerations:
- Lazy loading of images in feed
- Background fetch for updating feed
- Efficient image caching using NSCache
7. Security:
- HTTPS for all network requests
- Secure storage of user credentials using Keychain
- Image compression before upload to reduce data usage
8. Third-party Integrations:
- Social media sharing (Facebook, Twitter, Instagram)
- Analytics (Firebase, Mixpanel)
- Crash reporting (Crashlytics)
9. Scalability:
- Use of CDN for image delivery
- Pagination of API responses
- Efficient use of background tasks for data syncing
Remember to discuss trade-offs and justify your design decisions during the interview.
6. Behavioral Questions and Soft Skills
In addition to technical skills, interviewers will assess your soft skills and cultural fit. Be prepared to answer questions about:
- Your experience working in team environments
- How you handle conflicts or disagreements
- Your approach to learning new technologies
- Times when you’ve had to meet tight deadlines
- How you prioritize tasks and manage your time
- Your biggest achievements or challenges in previous projects
Use the STAR method (Situation, Task, Action, Result) to structure your responses to behavioral questions. Here’s an example:
Question: “Tell me about a time when you had to optimize the performance of an iOS app.”
Answer:
Situation: In my previous role, we noticed that our e-commerce app was becoming sluggish, especially when loading product listings.
Task: I was tasked with identifying the performance bottlenecks and improving the app’s overall speed and responsiveness.
Action: I used Instruments to profile the app and discovered that image loading was the main culprit. I implemented the following optimizations:
- Implemented lazy loading for images in table views
- Added an efficient caching mechanism using NSCache
- Optimized network requests by implementing pagination
- Used background fetching to preload data when possible
Result: These optimizations resulted in a 40% reduction in initial load time and a much smoother scrolling experience. User engagement increased by 15%, and we received positive feedback in app store reviews about the improved performance.
7. Preparation Tips and Resources
To excel in your iOS engineer interview, consider the following preparation strategies:
Study and Practice
- Review iOS development concepts and Swift programming
- Solve coding challenges on platforms like LeetCode, HackerRank, or Codewars
- Build sample apps to practice implementing common iOS features
- Stay updated with the latest iOS technologies and best practices
Mock Interviews
- Practice with a friend or mentor who has iOS development experience
- Use online platforms that offer mock technical interviews
- Record yourself answering questions to improve your communication skills
Resources
- Apple’s official documentation and WWDC videos
- Ray Wenderlich tutorials and books
- Stanford’s CS193p course on iOS development
- iOS-specific blogs and podcasts
- GitHub repositories with iOS interview questions and answers
Open Source Contributions
Contributing to open-source iOS projects can demonstrate your skills and passion for the platform. It also provides real-world experience working with other developers’ code.
8. Common Mistakes to Avoid
Be aware of these common pitfalls during iOS engineer interviews:
- Neglecting iOS-specific knowledge: While general programming skills are important, make sure you’re well-versed in iOS-specific concepts and best practices.
- Overlooking UI/UX considerations: Remember that iOS development is not just about functionality; user experience is crucial.
- Ignoring memory management: Be prepared to discuss ARC and potential memory leaks in iOS apps.
- Failing to consider backwards compatibility: Discuss how you approach supporting multiple iOS versions when implementing new features.
- Not asking clarifying questions: Don’t hesitate to ask for more information or clarification during coding challenges or system design questions.
- Rushing through problems: Take your time to think through solutions and explain your thought process.
- Neglecting to test your code: Always consider edge cases and demonstrate how you would test your implementations.
9. Post-Interview Process
After completing your interviews, there are several steps you can take to increase your chances of success:
Follow-up
- Send a thank-you email to your interviewers within 24 hours
- Reiterate your interest in the position and the company
- Briefly mention any key points from the interview or additional thoughts you’ve had
Reflection
- Review your performance and note areas for improvement
- Consider any feedback provided during the interview process
- Think about questions you struggled with and research the correct answers
Patience
The hiring process can take time, especially for larger companies. Be patient and avoid constantly checking in, but don’t hesitate to politely inquire about the timeline if you haven’t heard back after a week or two.
Continuous Learning
Regardless of the outcome, use the interview experience as a learning opportunity. Continue to improve your skills and stay updated with the latest iOS developments.
10. Conclusion
Preparing for an iOS engineer interview requires a combination of technical proficiency, problem-solving skills, and effective communication. By understanding the anatomy of the interview process and thoroughly preparing for each component, you’ll be well-equipped to showcase your abilities and land your dream iOS development role.
Remember that interviewing is a skill that improves with practice. Each interview, whether successful or not, provides valuable experience and insights that will help you in future opportunities. Stay curious, keep learning, and approach each interview as a chance to demonstrate your passion for iOS development.
Good luck with your iOS engineer interviews!