The Anatomy of an Embedded Systems Developer Interview
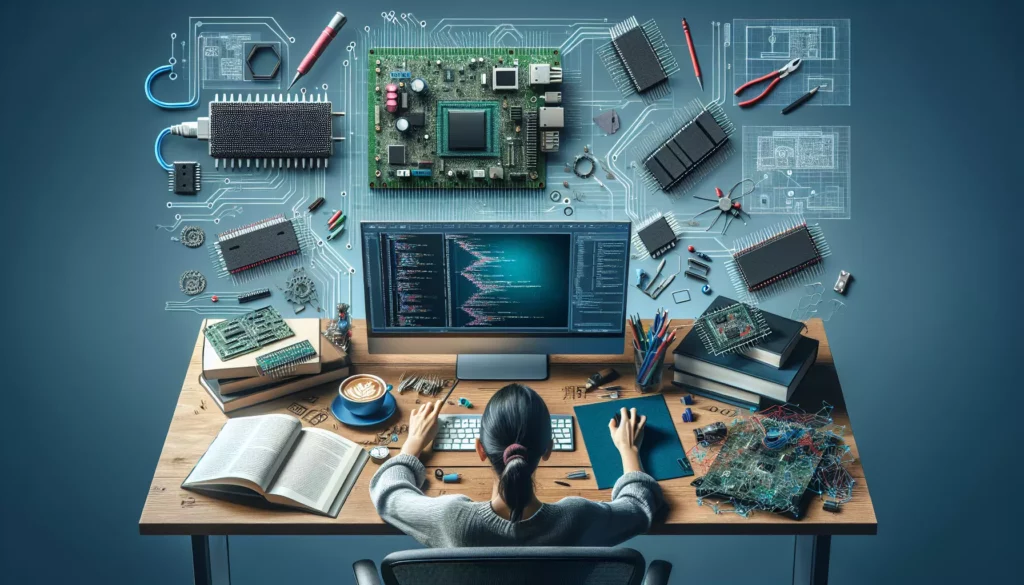
In today’s rapidly evolving technological landscape, embedded systems play a crucial role in powering the devices we interact with daily. From smart thermostats to wearable fitness trackers, these compact yet powerful systems are the backbone of the Internet of Things (IoT) revolution. As the demand for skilled embedded systems developers continues to grow, it’s essential for aspiring programmers and seasoned professionals alike to understand what it takes to excel in an embedded systems developer interview.
This comprehensive guide will walk you through the key components of an embedded systems developer interview, providing insights into the skills, knowledge, and preparation required to succeed. Whether you’re a recent graduate looking to break into the field or an experienced developer aiming to transition into embedded systems, this article will equip you with the tools you need to navigate the interview process with confidence.
1. Understanding the Role of an Embedded Systems Developer
Before diving into the specifics of the interview process, it’s crucial to have a clear understanding of what an embedded systems developer does. Embedded systems developers are responsible for creating software that runs on hardware devices with limited resources, such as microcontrollers or single-board computers. These devices are often found in IoT applications, consumer electronics, automotive systems, and industrial automation.
Key responsibilities of an embedded systems developer include:
- Designing and implementing software for embedded devices
- Optimizing code for performance and resource efficiency
- Integrating hardware and software components
- Debugging and troubleshooting hardware-software interactions
- Ensuring real-time performance and reliability
- Collaborating with hardware engineers and other team members
With this role overview in mind, let’s explore the essential skills and knowledge areas that interviewers typically assess during an embedded systems developer interview.
2. Core Technical Skills
Embedded systems development requires a unique blend of software and hardware expertise. During the interview process, you can expect to be evaluated on the following core technical skills:
2.1. Programming Languages
Proficiency in low-level programming languages is crucial for embedded systems development. The most commonly used languages in this field are:
- C: The lingua franca of embedded systems, C offers low-level control and efficiency.
- C++: Provides object-oriented features while maintaining the performance benefits of C.
- Assembly: Used for performance-critical sections and direct hardware manipulation.
Interviewers may ask you to write code snippets or explain concepts specific to these languages. For example:
// C example: Bit manipulation
uint8_t set_bit(uint8_t value, uint8_t bit_position) {
return value | (1 << bit_position);
}
uint8_t clear_bit(uint8_t value, uint8_t bit_position) {
return value & ~(1 << bit_position);
}
uint8_t toggle_bit(uint8_t value, uint8_t bit_position) {
return value ^ (1 << bit_position);
}
2.2. Microcontroller Architecture
A solid understanding of microcontroller architecture is essential for embedded systems development. You should be familiar with:
- CPU architectures (e.g., ARM, AVR, PIC)
- Memory types and management (RAM, ROM, Flash)
- Peripherals and interfaces (GPIO, UART, SPI, I2C)
- Interrupt handling and prioritization
Interviewers might ask questions about specific microcontroller families or how to interface with various peripherals. For instance:
// Example: Configuring a GPIO pin on an ARM Cortex-M microcontroller
#define GPIO_PORT_A_BASE 0x40020000
#define GPIO_MODER_OFFSET 0x00
#define GPIO_ODR_OFFSET 0x14
void configure_gpio_output(uint32_t pin) {
volatile uint32_t *GPIOA_MODER = (uint32_t *)(GPIO_PORT_A_BASE + GPIO_MODER_OFFSET);
*GPIOA_MODER |= (1 << (pin * 2)); // Set pin as output
}
void set_gpio_pin(uint32_t pin) {
volatile uint32_t *GPIOA_ODR = (uint32_t *)(GPIO_PORT_A_BASE + GPIO_ODR_OFFSET);
*GPIOA_ODR |= (1 << pin); // Set pin high
}
2.3. Real-Time Operating Systems (RTOS)
Many embedded systems require real-time performance, making knowledge of RTOS concepts and popular RTOS platforms crucial. Key areas to focus on include:
- Task scheduling and prioritization
- Inter-task communication (semaphores, mutexes, message queues)
- Memory management in an RTOS environment
- Familiarity with popular RTOS platforms (e.g., FreeRTOS, VxWorks, QNX)
You might be asked to explain RTOS concepts or write code that demonstrates your understanding of real-time programming principles:
// FreeRTOS task creation example
void led_task(void *pvParameters) {
while (1) {
toggle_led();
vTaskDelay(pdMS_TO_TICKS(500)); // Delay for 500ms
}
}
int main(void) {
// ... initialization code ...
xTaskCreate(
led_task, // Function that implements the task
"LED Task", // Text name for the task
100, // Stack size in words
NULL, // Task input parameter
1, // Priority
NULL // Task handle
);
vTaskStartScheduler(); // Start the scheduler
// We should never get here
while (1) {}
}
3. Problem-Solving and Algorithms
While embedded systems development often focuses on hardware-specific challenges, strong problem-solving skills and algorithmic thinking are still crucial. Interviewers may assess your ability to:
- Optimize algorithms for memory and CPU-constrained environments
- Implement efficient data structures suitable for embedded systems
- Solve coding challenges with an emphasis on resource efficiency
You might encounter questions that require you to implement algorithms with specific constraints, such as:
// Implement a circular buffer for a UART receive handler
#define BUFFER_SIZE 64
typedef struct {
uint8_t data[BUFFER_SIZE];
uint32_t head;
uint32_t tail;
uint32_t count;
} CircularBuffer;
void buffer_init(CircularBuffer *buffer) {
buffer->head = 0;
buffer->tail = 0;
buffer->count = 0;
}
bool buffer_push(CircularBuffer *buffer, uint8_t value) {
if (buffer->count == BUFFER_SIZE) {
return false; // Buffer is full
}
buffer->data[buffer->head] = value;
buffer->head = (buffer->head + 1) % BUFFER_SIZE;
buffer->count++;
return true;
}
bool buffer_pop(CircularBuffer *buffer, uint8_t *value) {
if (buffer->count == 0) {
return false; // Buffer is empty
}
*value = buffer->data[buffer->tail];
buffer->tail = (buffer->tail + 1) % BUFFER_SIZE;
buffer->count--;
return true;
}
4. Hardware Interfacing and Protocols
Embedded systems developers must be adept at interfacing with various hardware components and implementing communication protocols. Key areas of focus include:
- Serial communication protocols (UART, SPI, I2C)
- Analog-to-Digital Conversion (ADC) and Digital-to-Analog Conversion (DAC)
- Pulse Width Modulation (PWM)
- Networking protocols (e.g., TCP/IP, Bluetooth, Zigbee)
Interviewers may ask you to explain how these protocols work or write code to implement them. For example:
// SPI communication example (simplified)
#define SPI_BASE 0x40013000
#define SPI_CR1 (*(volatile uint32_t *)(SPI_BASE + 0x00))
#define SPI_SR (*(volatile uint32_t *)(SPI_BASE + 0x08))
#define SPI_DR (*(volatile uint32_t *)(SPI_BASE + 0x0C))
void spi_init(void) {
// Configure SPI: Master mode, 8-bit data, MSB first, etc.
SPI_CR1 = 0x0347;
}
uint8_t spi_transfer(uint8_t data) {
while (!(SPI_SR & 0x02)); // Wait until TX buffer is empty
SPI_DR = data;
while (!(SPI_SR & 0x01)); // Wait until RX buffer is not empty
return SPI_DR;
}
5. Debugging and Troubleshooting
Effective debugging is a critical skill for embedded systems developers. Interviewers will likely assess your ability to:
- Use debugging tools and techniques specific to embedded systems
- Analyze and interpret oscilloscope and logic analyzer output
- Troubleshoot hardware-software integration issues
- Implement error handling and recovery mechanisms
You might be presented with scenarios that require you to identify and solve common embedded systems issues. For instance:
// Implementing a watchdog timer to recover from software hangs
#define WATCHDOG_BASE 0x40002C00
#define WATCHDOG_LOAD (*(volatile uint32_t *)(WATCHDOG_BASE + 0x00))
#define WATCHDOG_CONTROL (*(volatile uint32_t *)(WATCHDOG_BASE + 0x08))
void watchdog_init(uint32_t timeout_ms) {
// Configure watchdog timer
WATCHDOG_LOAD = (timeout_ms * (SystemCoreClock / 1000)) / 4;
WATCHDOG_CONTROL = 0x01; // Enable watchdog
}
void watchdog_refresh(void) {
WATCHDOG_LOAD = WATCHDOG_LOAD; // Reload the watchdog timer
}
// In main application loop:
while (1) {
// Perform normal operations
watchdog_refresh(); // Refresh watchdog to prevent reset
}
6. Power Management and Optimization
Many embedded systems, especially IoT devices, have strict power consumption requirements. Interviewers may assess your knowledge of:
- Low-power modes and sleep states
- Dynamic frequency and voltage scaling
- Power-efficient coding practices
- Battery management techniques
You might be asked to explain power management concepts or optimize code for power efficiency:
// Example: Implementing a simple power-saving mode
#define POWER_CONTROL_REG (*(volatile uint32_t *)0x40000000)
#define SLEEP_MODE_BIT 0x01
void enter_sleep_mode(void) {
// Disable peripherals that are not needed in sleep mode
disable_unused_peripherals();
// Enter sleep mode
POWER_CONTROL_REG |= SLEEP_MODE_BIT;
// Wait for interrupt (WFI) instruction
__asm volatile ("wfi");
// CPU will resume execution here after waking up
// Re-enable necessary peripherals
enable_required_peripherals();
}
7. Software Development Practices
While embedded systems development has its unique challenges, following good software development practices is still crucial. Interviewers may evaluate your knowledge of:
- Version control systems (e.g., Git)
- Code review processes
- Testing methodologies for embedded systems
- Documentation and code commenting practices
You might be asked about your experience with these practices or how you would apply them in an embedded systems context:
/**
* @brief Initializes the ADC for temperature sensing
* @param adc_channel The ADC channel to use for temperature sensing
* @return 0 if initialization is successful, -1 otherwise
*/
int init_temperature_sensor(uint8_t adc_channel) {
// Configure ADC for temperature sensing
if (adc_channel > MAX_ADC_CHANNELS) {
return -1; // Invalid channel
}
// ADC initialization code here
// ...
return 0;
}
8. Industry Knowledge and Trends
Staying up-to-date with industry trends and emerging technologies is important for any developer, including those in the embedded systems field. Interviewers may ask about your awareness of:
- IoT and edge computing trends
- Security considerations for embedded systems
- New microcontroller architectures and features
- Emerging communication protocols and standards
Be prepared to discuss how these trends might impact embedded systems development and how you stay informed about industry developments.
9. Practical Experience and Projects
Many interviewers will be interested in your hands-on experience with embedded systems. Be prepared to discuss:
- Personal or professional projects you’ve worked on
- Challenges you’ve faced and how you overcame them
- Your experience with specific microcontroller platforms or development boards
- Any contributions to open-source embedded projects
Having a portfolio of projects or code samples can be a significant advantage during the interview process.
10. Soft Skills and Team Collaboration
While technical skills are crucial, embedded systems developers often work as part of a larger team, collaborating with hardware engineers, product managers, and other stakeholders. Interviewers may assess your:
- Communication skills, especially when explaining technical concepts
- Ability to work in a team and collaborate across disciplines
- Problem-solving approach and adaptability
- Time management and project planning skills
Be prepared to provide examples of how you’ve demonstrated these skills in past projects or work experiences.
11. Interview Preparation Tips
To maximize your chances of success in an embedded systems developer interview, consider the following preparation tips:
- Review fundamentals: Brush up on core concepts in C, C++, and assembly language programming.
- Practice coding challenges: Solve embedded-specific coding problems, focusing on optimizing for limited resources.
- Study microcontroller datasheets: Familiarize yourself with popular microcontroller architectures and their features.
- Hands-on projects: Work on personal embedded projects to gain practical experience and have real-world examples to discuss.
- Mock interviews: Practice explaining technical concepts and walking through your problem-solving process.
- Stay updated: Read industry publications and follow embedded systems blogs to stay current with trends and best practices.
- Prepare questions: Have thoughtful questions ready to ask your interviewer about the role and the company’s embedded systems projects.
Conclusion
The embedded systems developer interview process can be challenging, but with the right preparation and mindset, it’s an opportunity to showcase your skills and passion for this exciting field. By focusing on the key areas outlined in this guide – from core technical skills to industry knowledge and soft skills – you’ll be well-equipped to tackle the interview with confidence.
Remember that embedded systems development is a rapidly evolving field, and continuous learning is crucial for long-term success. Embrace the challenges, stay curious, and never stop exploring new technologies and techniques. With dedication and perseverance, you can build a rewarding career at the intersection of hardware and software, creating the smart devices that shape our connected world.
As you prepare for your embedded systems developer interview, consider leveraging resources like AlgoCademy to enhance your coding skills and problem-solving abilities. While AlgoCademy’s primary focus is on algorithmic thinking and preparing for technical interviews at major tech companies, many of the skills you’ll develop – such as efficient coding practices and optimized algorithms – are directly applicable to embedded systems development.
Good luck with your interview preparation, and may your embedded systems career be filled with innovation and success!