The Anatomy of an Angular Engineer Interview: A Comprehensive Guide
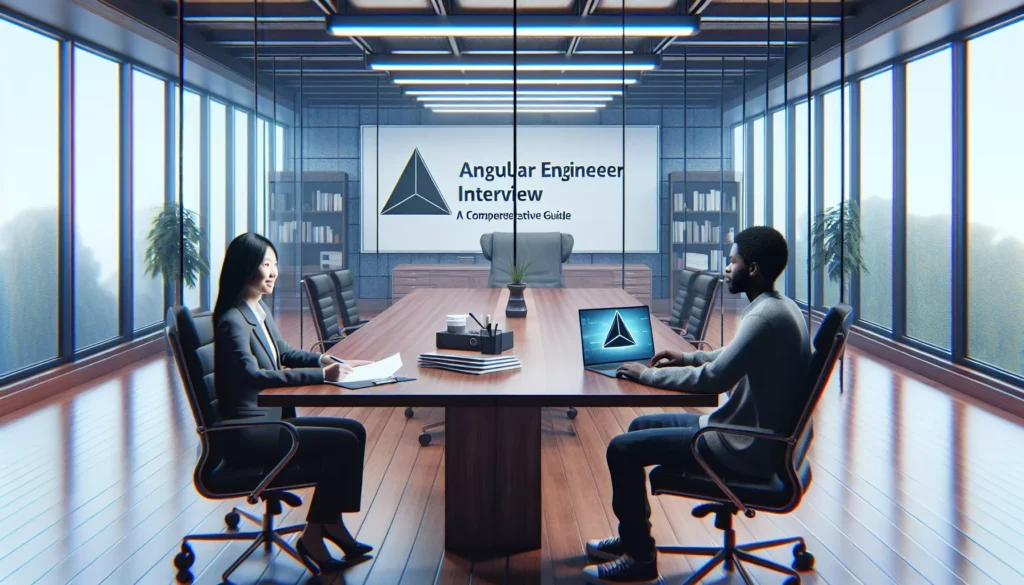
In the ever-evolving landscape of web development, Angular has established itself as a powerhouse framework for building robust and scalable applications. As the demand for skilled Angular engineers continues to grow, so does the complexity of the interview process. This comprehensive guide will walk you through the anatomy of an Angular engineer interview, helping you prepare for success in your next career opportunity.
1. Understanding the Angular Ecosystem
Before diving into the specifics of the interview process, it’s crucial to have a solid grasp of the Angular ecosystem. Angular is not just a framework; it’s a complete platform for building web applications. Here are some key components you should be familiar with:
- Angular CLI: The command-line interface for scaffolding, developing, and maintaining Angular projects
- TypeScript: The superset of JavaScript that Angular is built upon
- RxJS: The reactive programming library used extensively in Angular for handling asynchronous operations
- Angular Material: The UI component library that implements Material Design principles
- NgRx: The state management library for Angular applications
Interviewers will expect you to have a working knowledge of these tools and how they integrate within the Angular framework.
2. Technical Knowledge Assessment
The core of any Angular engineer interview will involve assessing your technical knowledge. Here are some key areas you should be prepared to discuss:
2.1. Angular Core Concepts
Be ready to explain and demonstrate your understanding of fundamental Angular concepts such as:
- Components and component lifecycle hooks
- Templates and data binding
- Directives and pipes
- Services and dependency injection
- Modules and lazy loading
- Routing and navigation
For example, you might be asked to explain the difference between ViewChild and ContentChild decorators or describe the purpose of NgZone in Angular applications.
2.2. Angular Performance Optimization
Performance is a critical aspect of any web application. Be prepared to discuss techniques for optimizing Angular applications, such as:
- Change detection strategies
- AOT compilation
- Tree-shaking
- Lazy loading of modules
- Use of pure pipes and memoization
An interviewer might ask you to explain how OnPush change detection strategy works and when you would use it in a real-world scenario.
2.3. State Management
Managing state in complex Angular applications is crucial. Be ready to discuss:
- NgRx architecture and core concepts (Store, Actions, Reducers, Effects, Selectors)
- Alternatives to NgRx (e.g., NGXS, Akita)
- When to use local component state vs. global state management
You might be asked to design a simple state management solution for a given scenario or explain the benefits of using NgRx in large-scale applications.
2.4. Testing in Angular
Testing is an integral part of the development process. Be prepared to discuss:
- Unit testing with Jasmine and Karma
- Integration testing with TestBed
- End-to-end testing with Protractor or Cypress
- Test-driven development (TDD) practices
An interviewer might ask you to write a simple unit test for an Angular service or component.
3. Coding Challenges
Most Angular engineer interviews will include one or more coding challenges. These can range from simple exercises to more complex problem-solving tasks. Here are some types of challenges you might encounter:
3.1. Component Creation
You may be asked to create a simple Angular component from scratch. For example:
<!-- Create a reusable button component with customizable text and click event -->
<app-custom-button [text]="'Click me'" (onClick)="handleClick()"></app-custom-button>
Be prepared to implement the component, including its TypeScript class, template, and any necessary styling.
3.2. Data Binding and Event Handling
Interviewers often ask candidates to demonstrate their understanding of data binding and event handling in Angular. You might be given a task like:
<!-- Create a simple todo list component with add and remove functionality -->
<app-todo-list [items]="todoItems" (addItem)="onAddItem($event)" (removeItem)="onRemoveItem($event)"></app-todo-list>
You would need to implement the component, handling both input properties and output events.
3.3. Service Implementation
You might be asked to create a service that interacts with an API or manages some aspect of the application state. For example:
// Implement a UserService that fetches user data from an API
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
import { User } from './user.model';
@Injectable({
providedIn: 'root'
})
export class UserService {
constructor(private http: HttpClient) {}
getUsers(): Observable<User[]> {
// Implement this method
}
getUserById(id: number): Observable<User> {
// Implement this method
}
}
Be prepared to discuss error handling, caching strategies, and how you would use this service in a component.
3.4. Reactive Forms
Angular’s reactive forms are a powerful feature, and you may be asked to implement a form with validation. For instance:
<!-- Create a registration form with email and password fields, including validation -->
<form [formGroup]="registrationForm" (ngSubmit)="onSubmit()">
<input formControlName="email" type="email" placeholder="Email">
<input formControlName="password" type="password" placeholder="Password">
<button type="submit" [disabled]="!registrationForm.valid">Register</button>
</form>
You would need to implement the form in the component, including form controls, validators, and submission handling.
4. System Design and Architecture
For more senior positions, you may be asked about system design and architecture. This could involve designing the structure of a large-scale Angular application or discussing architectural patterns. Be prepared to talk about:
- Modular architecture and feature modules
- Scalability considerations for Angular applications
- Performance optimization at the application level
- Security best practices in Angular
- Micro-frontends architecture using Angular
You might be given a scenario like: “Design the architecture for a large e-commerce platform using Angular. Consider aspects like product catalog, user authentication, shopping cart, and order processing.”
5. Behavioral Questions
While technical skills are crucial, many companies also place a high value on soft skills and cultural fit. Be prepared to answer behavioral questions that assess your problem-solving abilities, teamwork, and communication skills. Some examples include:
- “Describe a challenging project you worked on and how you overcame obstacles.”
- “How do you stay updated with the latest Angular features and best practices?”
- “Tell me about a time when you had to refactor a large portion of an Angular application. What was your approach?”
- “How do you handle disagreements with team members about technical decisions?”
When answering these questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your experience.
6. Angular Ecosystem and Third-party Libraries
Angular’s ecosystem is vast, and many companies use various third-party libraries to enhance their applications. Be familiar with popular libraries and tools such as:
- Angular Material for UI components
- NgRx for state management
- RxJS for reactive programming
- NgBootstrap for Bootstrap integration
- Jasmine and Karma for testing
- Angular Universal for server-side rendering
You might be asked about your experience with these libraries or how you would integrate them into an Angular project.
7. Version Control and CI/CD
While not specific to Angular, version control and continuous integration/continuous deployment (CI/CD) are essential skills for any modern developer. Be prepared to discuss:
- Git workflows (e.g., Gitflow, trunk-based development)
- Code review practices
- Continuous integration tools (e.g., Jenkins, GitLab CI, CircleCI)
- Deployment strategies for Angular applications
You might be asked to describe your typical Git workflow or how you would set up a CI/CD pipeline for an Angular project.
8. Performance Optimization and Debugging
Angular applications can become complex, and optimizing performance is crucial. Be ready to discuss:
- Techniques for improving Angular application performance
- Tools for profiling and debugging Angular applications (e.g., Chrome DevTools, Angular DevTools)
- Common performance bottlenecks in Angular and how to address them
- Strategies for reducing bundle size
An interviewer might present you with a scenario of a slow-performing Angular application and ask you to walk through your process for identifying and resolving the issues.
9. Angular CLI and Build Process
Understanding the Angular CLI and the build process is important for any Angular developer. Be prepared to discuss:
- Angular CLI commands and their uses
- Customizing the build process
- Creating and using custom schematics
- Configuring environments for different deployment scenarios
You might be asked to explain how you would set up multiple environments (development, staging, production) in an Angular project or how you would create a custom Angular CLI command.
10. Accessibility and Internationalization
As web applications become more global and inclusive, accessibility and internationalization are increasingly important. Be ready to discuss:
- WCAG guidelines and how to implement them in Angular applications
- Using ARIA attributes in Angular templates
- Implementing internationalization (i18n) in Angular
- Right-to-left (RTL) support in Angular applications
An interviewer might ask you to describe how you would make an existing Angular component more accessible or how you would set up an Angular application for multiple languages.
11. Angular and Mobile Development
With the increasing importance of mobile-first development, be prepared to discuss Angular’s capabilities in this area:
- Creating responsive Angular applications
- Progressive Web Apps (PWAs) with Angular
- Using Ionic with Angular for hybrid mobile app development
- Performance considerations for Angular applications on mobile devices
You might be asked to describe your approach to building a mobile-friendly Angular application or to explain the benefits of using Angular for PWA development.
12. Security in Angular Applications
Security is a critical concern in web development. Be ready to discuss Angular’s built-in security features and best practices:
- Cross-Site Scripting (XSS) prevention in Angular
- CSRF protection
- Content Security Policy (CSP) implementation
- Secure authentication and authorization in Angular applications
An interviewer might ask you to explain how Angular helps prevent XSS attacks or to describe how you would implement secure authentication in an Angular application.
Conclusion
Preparing for an Angular engineer interview requires a comprehensive understanding of the framework, its ecosystem, and related web development concepts. By thoroughly reviewing the topics covered in this guide and practicing coding challenges, you’ll be well-equipped to showcase your skills and land your dream Angular role.
Remember, the key to success in any interview is not just knowing the answers, but also being able to communicate your thought process clearly. Don’t be afraid to ask clarifying questions during the interview, and always be ready to explain your reasoning behind technical decisions.
As you prepare, consider using platforms like AlgoCademy to sharpen your coding skills and practice algorithm challenges. These resources can help you build the confidence and problem-solving abilities that are crucial in technical interviews.
Good luck with your Angular engineer interview! With thorough preparation and a passion for Angular development, you’ll be well on your way to impressing your interviewers and taking the next step in your career.