The Anatomy of an Android Engineer Interview: A Comprehensive Guide
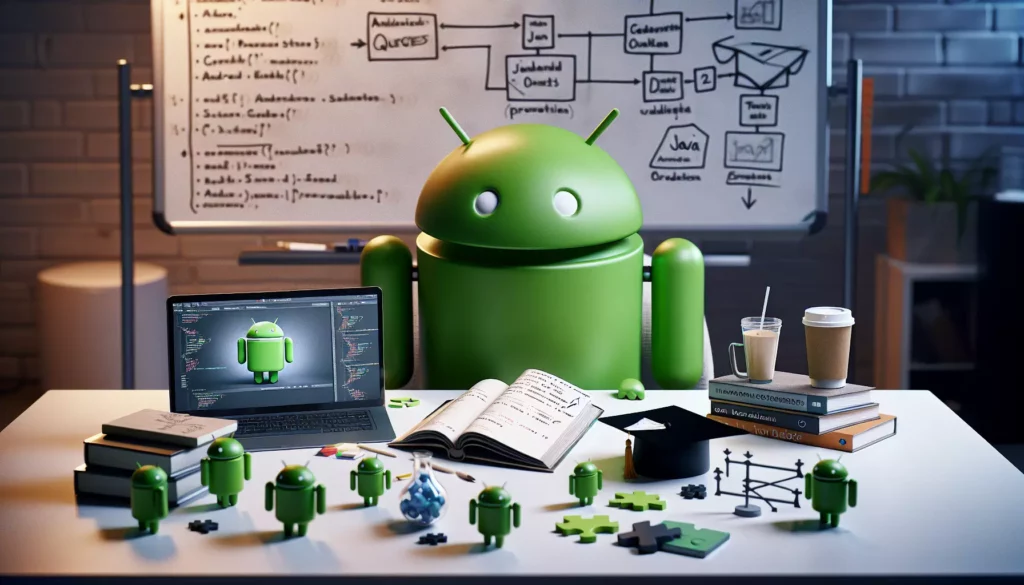
In the ever-evolving world of technology, Android development remains a crucial and in-demand skill. As companies continue to expand their mobile presence, the need for talented Android engineers grows. If you’re aspiring to land a coveted position as an Android engineer, understanding the intricacies of the interview process is essential. This comprehensive guide will walk you through the anatomy of an Android engineer interview, providing you with the knowledge and strategies needed to succeed.
1. Introduction to Android Engineer Interviews
Android engineer interviews are designed to assess your technical skills, problem-solving abilities, and cultural fit within the organization. These interviews typically consist of multiple rounds, each focusing on different aspects of your expertise and potential as an Android developer.
The interview process for Android engineers usually includes:
- Phone or video screening
- Technical phone interview
- On-site interviews (or virtual equivalents)
- Coding challenges
- System design discussions
- Behavioral interviews
Let’s dive deeper into each of these components to help you prepare effectively.
2. Phone or Video Screening
The first step in the Android engineer interview process is often a phone or video screening. This initial conversation is typically conducted by a recruiter or HR representative and serves as an opportunity for both parties to assess mutual interest.
What to Expect:
- Brief overview of your background and experience
- Discussion of your interest in the company and the role
- High-level questions about your Android development skills
- Clarification of job requirements and expectations
- Opportunity for you to ask questions about the company and position
Tips for Success:
- Research the company thoroughly before the call
- Prepare a concise summary of your relevant experience
- Have a list of thoughtful questions ready for the interviewer
- Be enthusiastic and articulate about your passion for Android development
3. Technical Phone Interview
Following the initial screening, you’ll likely face a technical phone interview. This round is designed to assess your fundamental Android development knowledge and problem-solving skills.
What to Expect:
- Questions about Android fundamentals (e.g., Activity lifecycle, Fragments, Intents)
- Discussions on Android architecture components (e.g., ViewModel, LiveData, Room)
- Basic coding problems related to data structures and algorithms
- Scenarios to test your understanding of Android-specific concepts
Sample Questions:
- Explain the Activity lifecycle and its methods.
- What is the difference between Implicit and Explicit Intents?
- How would you implement a singleton pattern in Kotlin?
- Describe the MVVM architecture pattern and its benefits in Android development.
Tips for Success:
- Review core Android concepts and best practices
- Practice explaining technical concepts clearly and concisely
- Be prepared to write code or pseudocode during the call
- Use a quiet environment with a stable internet connection
4. On-site Interviews (or Virtual Equivalents)
The on-site interview is typically the most comprehensive part of the Android engineer interview process. Due to recent global changes, many companies now conduct these interviews virtually. Regardless of the format, this stage usually consists of multiple rounds with different interviewers.
What to Expect:
- In-depth technical interviews
- Coding challenges
- System design discussions
- Behavioral interviews
- Team fit assessments
Tips for Success:
- Be prepared for a full day of interviews
- Bring a notebook and pen for taking notes
- Dress professionally, even for virtual interviews
- Stay hydrated and take breaks when offered
- Maintain a positive attitude throughout the process
5. Coding Challenges
Coding challenges are a crucial component of the Android engineer interview process. These challenges assess your ability to write clean, efficient, and functional code under pressure.
What to Expect:
- Algorithm and data structure problems
- Android-specific coding tasks
- Time-constrained coding exercises
- Code review and optimization discussions
Sample Coding Challenge:
Implement a function that determines if a given string is a palindrome, ignoring non-alphanumeric characters and case sensitivity.
fun isPalindrome(s: String): Boolean {
val cleanString = s.toLowerCase().filter { it.isLetterOrDigit() }
return cleanString == cleanString.reversed()
}
// Test cases
println(isPalindrome("A man, a plan, a canal: Panama")) // true
println(isPalindrome("race a car")) // false
println(isPalindrome("Was it a car or a cat I saw?")) // true
Tips for Success:
- Practice coding problems regularly on platforms like LeetCode or HackerRank
- Focus on writing clean, readable, and efficient code
- Explain your thought process as you code
- Be prepared to optimize your solution if asked
- Test your code with various input scenarios
6. System Design Discussions
System design discussions evaluate your ability to architect large-scale Android applications. These conversations assess your understanding of Android architecture, scalability, and performance optimization.
What to Expect:
- High-level design of Android applications
- Discussions on app architecture patterns (e.g., MVVM, Clean Architecture)
- Scalability and performance considerations
- Integration with backend services and APIs
- Handling offline functionality and data synchronization
Sample System Design Question:
Design a music streaming app that allows users to browse, search, and play music, create playlists, and download songs for offline listening.
Key Considerations:
- User authentication and profile management
- Efficient music catalog browsing and search functionality
- Streaming and playback mechanisms
- Playlist creation and management
- Offline mode and local storage management
- Integration with music licensing and royalty systems
- Scalability to handle millions of users and songs
Tips for Success:
- Start with clarifying questions to understand the requirements
- Break down the problem into smaller components
- Consider both client-side and server-side aspects
- Discuss trade-offs between different design choices
- Be prepared to dive into specific implementation details if asked
7. Behavioral Interviews
Behavioral interviews assess your soft skills, work ethic, and cultural fit within the organization. These questions often focus on past experiences and how you’ve handled various situations.
What to Expect:
- Questions about your past projects and experiences
- Scenarios to assess your problem-solving and teamwork skills
- Discussions about your career goals and motivations
- Inquiries about how you handle challenges and conflicts
Sample Behavioral Questions:
- Describe a challenging Android project you worked on and how you overcame obstacles.
- Tell me about a time when you had to work with a difficult team member. How did you handle it?
- How do you stay updated with the latest Android development trends and technologies?
- Describe a situation where you had to make a trade-off between performance and user experience in an Android app.
Tips for Success:
- Use the STAR method (Situation, Task, Action, Result) to structure your answers
- Prepare specific examples from your past experiences
- Be honest and authentic in your responses
- Highlight your strengths and areas of expertise
- Show enthusiasm for continuous learning and growth
8. Technical Knowledge Areas to Focus On
To excel in an Android engineer interview, it’s crucial to have a strong foundation in various technical areas. Here are some key topics to review and master:
Android Fundamentals:
- Activity and Fragment lifecycles
- Intents and Intent Filters
- Android Manifest file
- Permissions and security
- Resources and resource management
User Interface and Layouts:
- XML layouts and View hierarchy
- ConstraintLayout and other layout types
- Custom Views and ViewGroups
- Material Design principles
- Responsive design for different screen sizes
Android Architecture Components:
- ViewModel and LiveData
- Room database
- Navigation component
- DataBinding and ViewBinding
- WorkManager for background tasks
Networking and Data Management:
- RESTful API integration (e.g., Retrofit)
- JSON parsing (e.g., Gson, Moshi)
- Image loading and caching (e.g., Glide, Coil)
- Local data storage (SharedPreferences, SQLite)
- Content Providers and Loaders
Concurrency and Asynchronous Programming:
- Coroutines and Flow
- RxJava (if relevant to the company)
- AsyncTask and its limitations
- Thread management and synchronization
Testing and Debugging:
- Unit testing with JUnit and Mockito
- UI testing with Espresso
- Integration testing
- Debugging tools and techniques
- Profiling and performance optimization
Kotlin Programming:
- Kotlin syntax and features
- Null safety and smart casts
- Extension functions and properties
- Higher-order functions and lambdas
- Coroutines and Flow in Kotlin
9. Preparing for the Interview
Thorough preparation is key to succeeding in an Android engineer interview. Here are some strategies to help you get ready:
1. Review Core Concepts:
Go through Android documentation, focusing on fundamental concepts and recent updates. Ensure you have a solid understanding of Android architecture components and best practices.
2. Practice Coding:
Solve coding problems regularly on platforms like LeetCode, HackerRank, or AlgoCademy. Focus on both general algorithmic problems and Android-specific challenges.
3. Build Projects:
Create personal Android projects to apply your knowledge and showcase your skills. This hands-on experience will be valuable during interviews.
4. Stay Updated:
Follow Android development blogs, attend conferences or webinars, and participate in online communities to stay current with the latest trends and technologies.
5. Mock Interviews:
Practice with friends, mentors, or online platforms that offer mock interview services. This will help you get comfortable with the interview process and receive feedback on your performance.
6. Review Your Past Projects:
Be prepared to discuss your previous Android projects in detail, including challenges faced, solutions implemented, and lessons learned.
7. Prepare Questions:
Develop a list of thoughtful questions to ask your interviewers about the company, team, and role. This demonstrates your genuine interest and engagement.
10. Common Pitfalls to Avoid
While preparing for your Android engineer interview, be aware of these common pitfalls and take steps to avoid them:
1. Neglecting Fundamentals:
Don’t focus solely on advanced topics at the expense of core Android concepts. Interviewers often assess your understanding of basics like Activity lifecycle or Intent usage.
2. Overlooking Soft Skills:
Technical skills are crucial, but don’t underestimate the importance of communication, teamwork, and problem-solving abilities. Be prepared to demonstrate these soft skills during behavioral interviews.
3. Failing to Ask Questions:
Not asking questions about the role or company can be interpreted as a lack of interest. Prepare thoughtful questions to show your engagement and curiosity.
4. Rushing Through Problems:
Take your time to understand the problem fully before diving into a solution. Communicate your thought process clearly and consider edge cases.
5. Ignoring Code Quality:
Don’t sacrifice code readability and best practices for the sake of speed. Write clean, well-structured code even under time pressure.
6. Not Admitting Knowledge Gaps:
It’s okay not to know everything. If you’re unsure about something, be honest and express your willingness to learn.
7. Neglecting to Follow Up:
After the interview, send a thank-you email to your interviewers. This is an opportunity to reiterate your interest and address any points you may have missed during the interview.
11. Post-Interview Steps
After completing your Android engineer interview, there are several important steps to take:
1. Send Thank-You Notes:
Email your interviewers within 24 hours to express gratitude for their time and reiterate your interest in the position.
2. Reflect on the Experience:
Take some time to review the questions asked and your responses. Identify areas where you performed well and areas for improvement.
3. Follow Up Appropriately:
If you haven’t heard back within the timeframe provided, it’s acceptable to follow up politely with your main point of contact.
4. Continue Learning:
Regardless of the outcome, use the interview experience as a learning opportunity. Focus on strengthening any weak areas identified during the process.
5. Be Patient:
The hiring process can take time, especially for larger companies. Stay positive and continue your job search while waiting for a response.
Conclusion
Navigating an Android engineer interview can be challenging, but with thorough preparation and the right mindset, you can succeed. Remember that the interview process is not just about showcasing your technical skills, but also about demonstrating your passion for Android development, your problem-solving abilities, and your potential as a team member.
By understanding the anatomy of an Android engineer interview and following the tips and strategies outlined in this guide, you’ll be well-equipped to tackle each stage of the process with confidence. Whether you’re a seasoned Android developer or just starting your career, continuous learning and practice are key to staying competitive in this dynamic field.
Good luck with your Android engineer interviews, and may your coding journey be filled with exciting opportunities and groundbreaking apps!