The Anatomy of an AI/Deep Learning Engineer Interview: What to Expect and How to Prepare
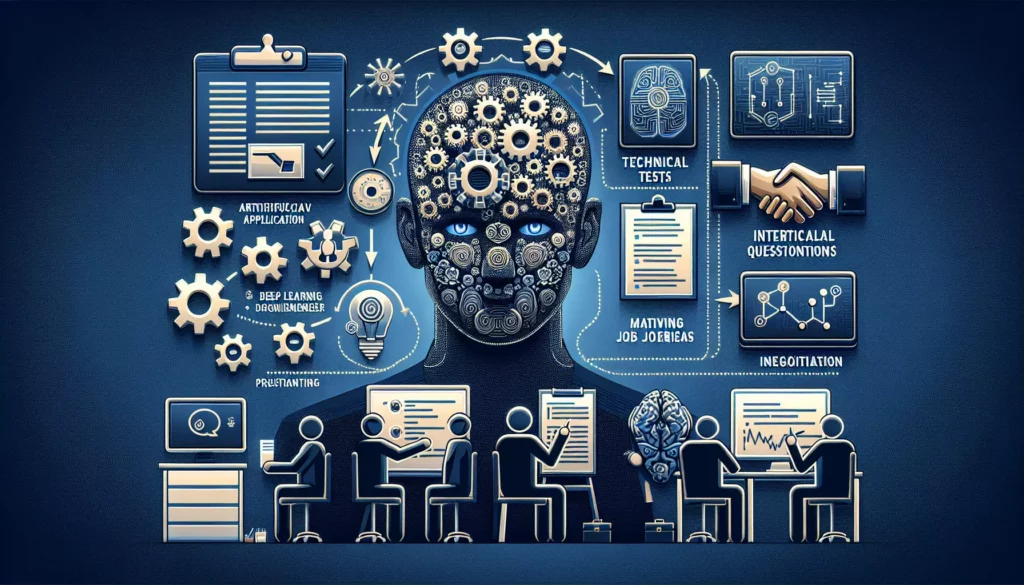
In today’s rapidly evolving tech landscape, artificial intelligence (AI) and deep learning have become pivotal in driving innovation across industries. As a result, the demand for skilled AI and deep learning engineers has skyrocketed, making it one of the most sought-after roles in the tech industry. If you’re aspiring to become an AI/Deep Learning Engineer or looking to advance your career in this field, understanding the intricacies of the interview process is crucial. In this comprehensive guide, we’ll dissect the anatomy of an AI/Deep Learning Engineer interview, providing you with invaluable insights on what to expect and how to prepare effectively.
1. Understanding the Role of an AI/Deep Learning Engineer
Before diving into the interview process, it’s essential to grasp the responsibilities and expectations associated with the role of an AI/Deep Learning Engineer. These professionals are at the forefront of developing cutting-edge artificial intelligence systems, including neural networks and deep learning algorithms. Their primary focus is on:
- Designing and implementing machine learning models
- Developing and optimizing deep learning algorithms
- Creating and maintaining AI infrastructure
- Collaborating with cross-functional teams to integrate AI solutions
- Staying updated with the latest advancements in AI and deep learning
With this understanding, let’s explore the key components of an AI/Deep Learning Engineer interview.
2. Technical Skills Assessment
A significant portion of the interview process will be dedicated to evaluating your technical skills. Interviewers will assess your proficiency in various areas crucial for AI and deep learning development.
2.1. Programming Languages
Python is the de facto language for AI and deep learning, so expect in-depth questions and coding challenges in Python. You should be comfortable with:
- Python syntax and data structures
- Object-oriented programming concepts
- Functional programming paradigms
- Writing efficient and clean code
Example question: “Implement a function to perform matrix multiplication using NumPy.”
import numpy as np
def matrix_multiplication(A, B):
return np.dot(A, B)
# Example usage
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
result = matrix_multiplication(A, B)
print(result)
2.2. Deep Learning Frameworks
Proficiency in popular deep learning frameworks is crucial. You should be well-versed in:
- TensorFlow
- Keras
- PyTorch
Expect questions about the architecture, advantages, and use cases of these frameworks.
Example question: “Explain the difference between eager execution and graph execution in TensorFlow.”
2.3. Neural Network Architectures
You’ll be expected to demonstrate a deep understanding of various neural network architectures, including:
- Convolutional Neural Networks (CNNs)
- Recurrent Neural Networks (RNNs)
- Long Short-Term Memory (LSTM) networks
- Transformers
Be prepared to explain the architecture, use cases, and potential limitations of each type.
Example question: “Design a CNN architecture for image classification and explain each layer’s purpose.”
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
def create_cnn_model():
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(224, 224, 3)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
Flatten(),
Dense(64, activation='relu'),
Dense(10, activation='softmax')
])
return model
model = create_cnn_model()
model.summary()
2.4. Machine Learning Algorithms
While deep learning is a subset of machine learning, you should also be familiar with traditional machine learning algorithms and concepts, such as:
- Supervised vs. unsupervised learning
- Decision trees and random forests
- Support Vector Machines (SVM)
- K-means clustering
- Principal Component Analysis (PCA)
Example question: “Explain the difference between L1 and L2 regularization and when you would use each.”
2.5. Optimization Techniques
Understanding various optimization algorithms and techniques is crucial for improving model performance. Be prepared to discuss:
- Gradient descent and its variants (e.g., Stochastic Gradient Descent, Mini-batch Gradient Descent)
- Adam, RMSprop, and other adaptive learning rate methods
- Batch normalization
- Dropout
Example question: “How does dropout help prevent overfitting in neural networks?”
3. Problem-Solving and Algorithmic Thinking
AI and deep learning engineers must possess strong problem-solving skills and the ability to think algorithmically. Interviewers will assess these skills through various coding challenges and theoretical questions.
3.1. Coding Challenges
Expect to solve coding problems related to AI and deep learning concepts. These may include:
- Implementing basic neural network components from scratch
- Optimizing existing algorithms for better performance
- Solving data preprocessing and feature engineering problems
Example challenge: “Implement a simple feedforward neural network using NumPy.”
import numpy as np
class SimpleNeuralNetwork:
def __init__(self, input_size, hidden_size, output_size):
self.W1 = np.random.randn(input_size, hidden_size)
self.b1 = np.zeros((1, hidden_size))
self.W2 = np.random.randn(hidden_size, output_size)
self.b2 = np.zeros((1, output_size))
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def forward(self, X):
self.z1 = np.dot(X, self.W1) + self.b1
self.a1 = self.sigmoid(self.z1)
self.z2 = np.dot(self.a1, self.W2) + self.b2
self.a2 = self.sigmoid(self.z2)
return self.a2
# Example usage
nn = SimpleNeuralNetwork(3, 4, 2)
X = np.array([[0.1, 0.2, 0.3]])
output = nn.forward(X)
print(output)
3.2. Algorithm Design and Analysis
You may be asked to design algorithms for specific AI tasks or analyze the time and space complexity of given algorithms. Focus on:
- Efficiency and scalability
- Handling edge cases
- Optimizing for specific constraints (e.g., memory limitations)
Example question: “Design an algorithm to efficiently find the k-nearest neighbors in a large dataset.”
4. Deep Learning Concepts and Techniques
A thorough understanding of deep learning concepts and techniques is essential. Be prepared to discuss and explain:
4.1. Activation Functions
- ReLU, Sigmoid, Tanh
- Leaky ReLU, ELU
- Softmax for multi-class classification
Example question: “Compare and contrast ReLU and Leaky ReLU activation functions.”
4.2. Loss Functions
- Mean Squared Error (MSE)
- Cross-entropy loss
- Hinge loss
Example question: “When would you use categorical cross-entropy loss versus binary cross-entropy loss?”
4.3. Regularization Techniques
- L1 and L2 regularization
- Dropout
- Early stopping
Example question: “Explain how dropout works and implement it in a simple neural network layer.”
import numpy as np
def dropout_layer(X, dropout_rate):
mask = np.random.binomial(1, 1 - dropout_rate, size=X.shape) / (1 - dropout_rate)
return X * mask
4.4. Transfer Learning
- Fine-tuning pre-trained models
- Feature extraction
- Domain adaptation
Example question: “Describe a scenario where transfer learning would be beneficial and explain how you would implement it.”
4.5. Generative Models
- Generative Adversarial Networks (GANs)
- Variational Autoencoders (VAEs)
Example question: “Explain the architecture and training process of a GAN.”
5. Practical Experience and Projects
Interviewers will be keen to understand your practical experience in applying AI and deep learning techniques to real-world problems. Be prepared to discuss:
5.1. Previous Projects
- Describe the problem you were solving
- Explain your approach and methodology
- Discuss the challenges you faced and how you overcame them
- Highlight the results and impact of your solution
Example question: “Tell me about a deep learning project you’ve worked on and the key insights you gained from it.”
5.2. Case Studies
You may be presented with hypothetical scenarios or real-world case studies to assess your problem-solving skills and domain knowledge. For example:
- Designing a recommendation system for an e-commerce platform
- Developing a sentiment analysis model for social media data
- Creating a facial recognition system for security applications
Example question: “How would you approach building a language translation model using deep learning techniques?”
6. AI Ethics and Responsible Development
As AI continues to impact various aspects of society, ethical considerations have become increasingly important. Be prepared to discuss:
- Bias in AI systems and mitigation strategies
- Privacy concerns and data protection
- Explainable AI and model interpretability
- Ethical implications of AI in decision-making processes
Example question: “How would you ensure fairness and reduce bias in a machine learning model used for hiring decisions?”
7. Staying Current with AI Advancements
The field of AI and deep learning is rapidly evolving, and interviewers will want to know how you stay updated with the latest developments. Be prepared to discuss:
- Recent research papers you’ve read and found interesting
- Conferences or workshops you’ve attended
- Online courses or certifications you’ve completed
- Open-source projects you’ve contributed to
Example question: “What do you think is the most exciting recent development in deep learning, and why?”
8. Soft Skills and Collaboration
While technical skills are crucial, soft skills and the ability to collaborate effectively are equally important for AI/Deep Learning Engineers. Be prepared to discuss:
- Experience working in cross-functional teams
- Communication skills, especially when explaining complex concepts to non-technical stakeholders
- Problem-solving and critical thinking abilities
- Adaptability and willingness to learn new technologies
Example question: “Describe a situation where you had to explain a complex AI concept to a non-technical team member. How did you approach it?”
9. Interview Preparation Tips
To maximize your chances of success in an AI/Deep Learning Engineer interview, consider the following preparation tips:
- Review fundamental concepts in linear algebra, calculus, and statistics
- Practice implementing common deep learning algorithms from scratch
- Work on personal projects or contribute to open-source AI projects
- Stay updated with the latest research papers and industry trends
- Participate in online coding challenges and AI competitions
- Prepare concise explanations for complex AI concepts
- Practice whiteboard coding and system design exercises
- Familiarize yourself with the company’s AI initiatives and products
10. Conclusion
The interview process for an AI/Deep Learning Engineer position is comprehensive and challenging, designed to assess both your technical expertise and problem-solving abilities. By thoroughly preparing for each aspect of the interview, from coding challenges to discussing ethical considerations, you’ll be well-equipped to showcase your skills and land your dream job in this exciting field.
Remember that the key to success lies not only in your technical knowledge but also in your passion for AI and your ability to apply that knowledge to solve real-world problems. As you prepare for your interview, focus on developing a deep understanding of the fundamentals, gaining practical experience, and staying curious about the latest advancements in AI and deep learning.
With dedication, practice, and a solid grasp of the concepts and skills outlined in this guide, you’ll be well-prepared to tackle any AI/Deep Learning Engineer interview and take the next step in your career. Good luck!