The Anatomy of a Vue Engineer Interview: What to Expect and How to Prepare
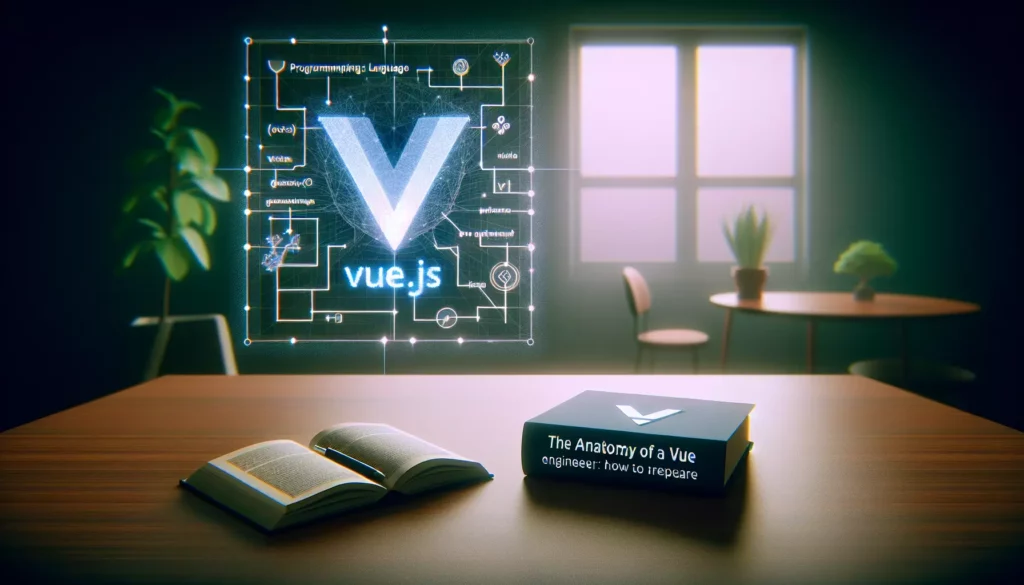
As the demand for skilled Vue.js developers continues to rise, mastering the art of the Vue engineer interview has become crucial for aspiring developers and seasoned professionals alike. Whether you’re aiming to land your first Vue role or looking to advance your career in the world of front-end development, understanding the intricacies of a Vue engineer interview can make all the difference. In this comprehensive guide, we’ll dissect the anatomy of a Vue engineer interview, exploring what to expect and providing valuable insights on how to prepare effectively.
1. The Importance of Vue.js in Modern Web Development
Before diving into the specifics of the interview process, it’s essential to understand why Vue.js has become such a sought-after skill in the tech industry. Vue.js, often referred to simply as Vue, is a progressive JavaScript framework for building user interfaces. Its popularity stems from its simplicity, flexibility, and performance, making it a favorite among developers and companies alike.
Some key reasons for Vue’s prominence include:
- Gentle learning curve compared to other frameworks
- Excellent documentation and community support
- Lightweight and fast performance
- Versatility in both small and large-scale applications
- Integration capabilities with existing projects
As a result, companies ranging from startups to large enterprises are increasingly adopting Vue for their web development needs, creating a high demand for skilled Vue engineers.
2. Types of Vue Engineer Interviews
Vue engineer interviews can take various forms, depending on the company and the specific role. Here are some common types of interviews you might encounter:
2.1 Technical Phone Screen
This is often the first step in the interview process. A recruiter or a technical team member will ask you basic questions about your experience with Vue.js and front-end development in general. They may also inquire about your familiarity with related technologies and your problem-solving approach.
2.2 Coding Challenge
Many companies send out take-home coding challenges to assess your practical skills. These challenges typically involve building a small Vue application or component within a specified timeframe. The goal is to evaluate your coding style, problem-solving abilities, and familiarity with Vue best practices.
2.3 Technical Interview
This is usually a more in-depth discussion of your technical skills. You might be asked to explain concepts, solve problems on a whiteboard, or review and discuss code. The interviewer will assess your understanding of Vue.js, JavaScript, and general web development principles.
2.4 Pair Programming Session
Some companies prefer a hands-on approach where you work alongside a team member on a real-world problem. This allows them to see how you think, code, and collaborate in real-time.
2.5 System Design Interview
For more senior positions, you might be asked to design a larger system or architecture. This tests your ability to think at a higher level and make decisions about the overall structure of a Vue application.
2.6 Cultural Fit Interview
While not strictly technical, this interview assesses how well you would fit into the company’s culture and work environment. It’s an opportunity for both you and the company to determine if it’s a good match.
3. Key Areas of Focus in Vue Engineer Interviews
Vue engineer interviews typically cover a wide range of topics. Here are some key areas you should be prepared to discuss:
3.1 Vue.js Fundamentals
Expect questions about core Vue concepts such as:
- Components and component lifecycle
- Reactivity and the Virtual DOM
- Directives and their usage
- Vue instance and its properties
- Template syntax and rendering
3.2 State Management
You should be familiar with state management in Vue, particularly:
- Vuex and its core concepts (state, getters, mutations, actions)
- When to use Vuex vs. component-level state
- Implementing and organizing stores
3.3 Routing
Understanding Vue Router is crucial. Be prepared to discuss:
- Route configuration and navigation
- Nested routes and route parameters
- Navigation guards and route meta fields
3.4 Component Communication
Interviewers often focus on how components interact:
- Props and events
- Event bus
- Provide/inject API
- Slots and scoped slots
3.5 Performance Optimization
Optimizing Vue applications is a critical skill. Be ready to discuss:
- Lazy loading and code splitting
- Keeping components lean
- Optimizing re-renders
- Using computed properties and watchers effectively
3.6 Testing
Testing is an integral part of development. Familiarize yourself with:
- Unit testing with Jest and Vue Test Utils
- End-to-end testing with tools like Cypress
- Component testing strategies
3.7 Build Tools and Ecosystem
Knowledge of the Vue ecosystem is important:
- Vue CLI and project setup
- Single-File Components
- Webpack and build processes
- Popular Vue libraries and plugins
3.8 Vue 3 Specifics
If the role involves Vue 3, be prepared to discuss:
- Composition API vs. Options API
- Teleport and Fragments
- Multiple root elements in templates
- Improved TypeScript support
4. Common Vue.js Interview Questions and How to Answer Them
While every interview is unique, there are some common questions that frequently come up in Vue engineer interviews. Let’s explore a few of these questions and how to approach them:
4.1 “Can you explain the Vue.js lifecycle hooks?”
This question tests your understanding of how Vue components work under the hood. A good answer would include:
- Explaining the main lifecycle hooks: created, mounted, updated, and destroyed
- Describing what each hook does and when it’s called
- Providing examples of when you might use each hook
- Mentioning less common hooks like beforeCreate, beforeMount, beforeUpdate, and beforeDestroy
Here’s a simple example demonstrating the use of lifecycle hooks:
<script>
export default {
data() {
return {
message: 'Hello, Vue!'
}
},
created() {
console.log('Component is created');
},
mounted() {
console.log('Component is mounted to the DOM');
},
updated() {
console.log('Component is updated');
},
destroyed() {
console.log('Component is destroyed');
}
}
</script>
4.2 “How does Vue’s reactivity system work?”
This question assesses your understanding of one of Vue’s core features. A comprehensive answer should cover:
- Explaining that Vue uses a getter/setter based reactivity system
- Describing how Vue converts data properties into getter/setters during initialization
- Explaining the role of the Virtual DOM in efficiently updating the UI
- Mentioning limitations, such as the inability to detect property addition or deletion
- Discussing Vue 3’s Proxy-based reactivity system if relevant
4.3 “What is the difference between computed properties and watchers?”
This question tests your understanding of two important Vue features. A good answer would include:
- Explaining that computed properties are cached based on their dependencies
- Noting that watchers are more useful for asynchronous or expensive operations
- Providing examples of when to use each
Here’s an example illustrating the difference:
<script>
export default {
data() {
return {
firstName: 'John',
lastName: 'Doe',
fullName: 'John Doe'
}
},
computed: {
// Computed property: updates automatically when dependencies change
computedFullName() {
return this.firstName + ' ' + this.lastName;
}
},
watch: {
// Watcher: performs action when firstName or lastName changes
firstName(newVal) {
this.fullName = newVal + ' ' + this.lastName;
},
lastName(newVal) {
this.fullName = this.firstName + ' ' + newVal;
}
}
}
</script>
4.4 “How would you handle state management in a large Vue application?”
This question assesses your ability to work on larger, more complex applications. A strong answer would:
- Mention Vuex as Vue’s official state management solution
- Explain the core concepts of Vuex: state, getters, mutations, and actions
- Discuss when to use Vuex vs. component-level state
- Mention alternative state management solutions if relevant
4.5 “Can you explain the concept of mixins in Vue?”
This question tests your knowledge of code reuse in Vue. A good answer would:
- Explain that mixins are a flexible way to distribute reusable functionalities for Vue components
- Describe how mixins are merged with the component’s own options
- Discuss the merge strategy for conflicts between mixin and component options
- Mention potential drawbacks, such as naming conflicts and reduced transparency
Here’s a simple example of a mixin:
// mixin.js
export const myMixin = {
created() {
console.log('Mixin hook called');
},
methods: {
hello() {
console.log('Hello from mixin!');
}
}
}
// Component using the mixin
import { myMixin } from './mixin.js';
export default {
mixins: [myMixin],
created() {
console.log('Component hook called');
}
}
5. Practical Coding Challenges in Vue Engineer Interviews
Many Vue engineer interviews include practical coding challenges. These can range from small tasks completed during the interview to more extensive take-home projects. Here are some common types of challenges you might encounter:
5.1 Building a Simple Component
You might be asked to create a Vue component from scratch. This could involve:
- Creating a form with validation
- Building a dynamic list with add/remove functionality
- Implementing a search feature with filtering
Here’s an example of a simple counter component:
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
<button @click="decrement">Decrement</button>
</div>
</template>
<script>
export default {
data() {
return {
count: 0
}
},
methods: {
increment() {
this.count++;
},
decrement() {
this.count--;
}
}
}
</script>
5.2 Integrating with an API
You might be asked to fetch data from an API and display it in a Vue component. This tests your ability to:
- Make HTTP requests (often using Axios)
- Handle asynchronous operations
- Manage loading and error states
5.3 Implementing State Management
For more advanced roles, you might need to implement a state management solution. This could involve:
- Setting up a Vuex store
- Creating actions, mutations, and getters
- Connecting components to the store
5.4 Optimizing Performance
You might be given a poorly performing Vue application and asked to optimize it. This could include:
- Identifying and fixing performance bottlenecks
- Implementing lazy loading for components or routes
- Optimizing computed properties and watchers
5.5 Writing Tests
Some interviews include writing tests for Vue components. This might involve:
- Setting up a testing environment (e.g., Jest and Vue Test Utils)
- Writing unit tests for components
- Mocking Vuex store or router if necessary
Here’s an example of a simple test for our counter component:
import { shallowMount } from '@vue/test-utils'
import Counter from '@/components/Counter.vue'
describe('Counter.vue', () => {
it('increments count when button is clicked', async () => {
const wrapper = shallowMount(Counter)
await wrapper.find('button').trigger('click')
expect(wrapper.vm.count).toBe(1)
})
})
6. Soft Skills and Cultural Fit in Vue Engineer Interviews
While technical skills are crucial, soft skills and cultural fit are equally important in many Vue engineer interviews. Here are some areas that interviewers often assess:
6.1 Communication Skills
As a Vue engineer, you’ll likely be working in a team and potentially interacting with non-technical stakeholders. Interviewers will be looking for:
- Ability to explain technical concepts clearly
- Active listening skills
- Comfort in asking questions and seeking clarification
6.2 Problem-Solving Approach
Your approach to solving problems is as important as the solution itself. Interviewers will be observing:
- How you break down complex problems
- Your ability to consider different solutions
- How you handle ambiguity or incomplete information
6.3 Teamwork and Collaboration
Most Vue development happens in a team context. Be prepared to discuss:
- Your experience working in Agile environments
- How you handle code reviews and feedback
- Your approach to mentoring or being mentored
6.4 Continuous Learning
The world of front-end development evolves rapidly. Interviewers want to see:
- Your enthusiasm for learning new technologies
- How you stay updated with Vue.js and related ecosystems
- Your involvement in the developer community (if any)
6.5 Adaptability
The ability to adapt to new situations and technologies is crucial. Be ready to discuss:
- How you’ve handled major changes in projects or technologies
- Your experience with different development methodologies
- How you approach learning new frameworks or tools
7. Preparing for Your Vue Engineer Interview
Now that we’ve covered what to expect in a Vue engineer interview, let’s discuss how to prepare effectively:
7.1 Brush Up on Vue.js Fundamentals
- Review the official Vue.js documentation
- Practice building small applications or components
- Ensure you understand core concepts like reactivity, components, and the Vue instance
7.2 Stay Updated with Vue Ecosystem
- Familiarize yourself with Vue 3 if you haven’t already
- Explore popular Vue libraries and tools (Vuex, Vue Router, Nuxt.js)
- Follow Vue.js news and updates
7.3 Practice Coding Challenges
- Solve Vue-specific coding problems on platforms like LeetCode or HackerRank
- Build small projects to reinforce your skills
- Practice explaining your code and thought process out loud
7.4 Prepare for Behavioral Questions
- Reflect on your past experiences and prepare specific examples
- Practice the STAR method (Situation, Task, Action, Result) for answering behavioral questions
- Think about how your experiences align with the company’s values and culture
7.5 Research the Company
- Understand the company’s products or services
- Research their tech stack and how Vue.js fits into it
- Prepare thoughtful questions about the role and the company
7.6 Mock Interviews
- Practice with a friend or mentor
- Record yourself answering questions to improve your communication
- Seek feedback on your responses and coding style
8. Conclusion: Mastering the Vue Engineer Interview
Preparing for a Vue engineer interview can seem daunting, but with the right approach, it can be an exciting opportunity to showcase your skills and passion for Vue.js development. Remember that interviews are not just about demonstrating your technical prowess, but also about showing your problem-solving abilities, communication skills, and cultural fit.
Key takeaways for success in your Vue engineer interview:
- Master the fundamentals of Vue.js and stay updated with the latest developments
- Practice coding challenges and be prepared to explain your thought process
- Understand the broader context of front-end development and how Vue fits into it
- Develop your soft skills, especially communication and problem-solving
- Show enthusiasm for continuous learning and adaptability
- Research the company and prepare thoughtful questions
By thoroughly preparing and approaching the interview with confidence, you’ll be well-equipped to navigate the Vue engineer interview process successfully. Remember, each interview is also an opportunity for you to learn and grow as a developer. Good luck with your Vue.js journey!