The Anatomy of a Software Engineering Interview: A Comprehensive Guide
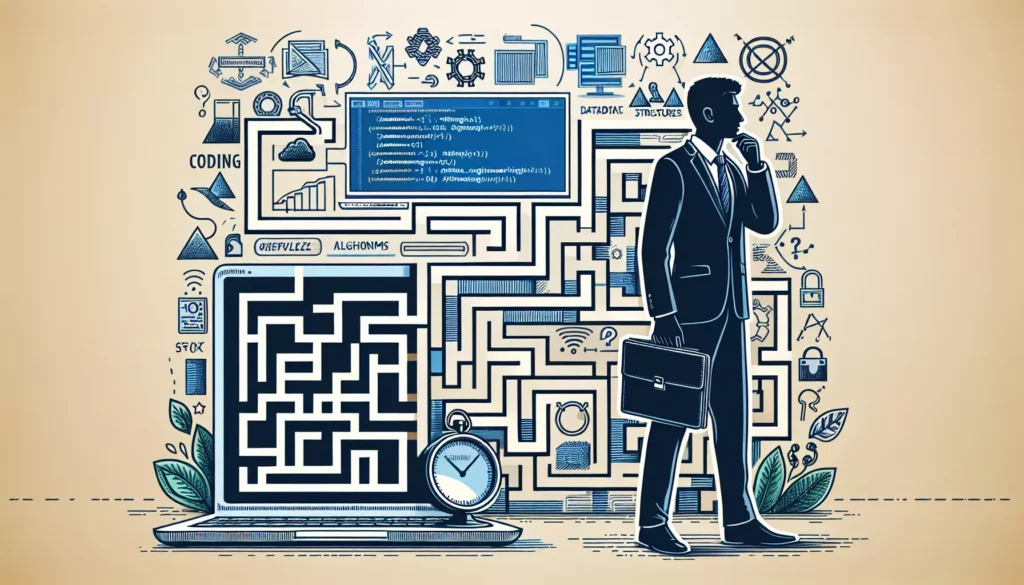
In the competitive landscape of the tech industry, securing a position as a software engineer at a prestigious company is a goal for many aspiring developers. Whether you’re eyeing a role at one of the FAANG (Facebook, Amazon, Apple, Netflix, Google) companies or any other tech giant, understanding the anatomy of a software engineering interview is crucial for success. This comprehensive guide will walk you through the various components of a typical software engineering interview, providing insights and strategies to help you navigate this challenging process.
1. The Application Process
Before diving into the interview itself, it’s important to understand the application process. This initial stage sets the foundation for your entire interview journey.
1.1 Resume Screening
Your journey begins with submitting your resume. Hiring managers and recruiters will review your application to determine if your skills and experience align with the job requirements. To increase your chances of moving forward:
- Tailor your resume to the specific role and company
- Highlight relevant projects, skills, and technologies
- Quantify your achievements where possible
- Ensure your resume is well-formatted and error-free
1.2 Online Coding Assessments
Many companies use online coding assessments as an initial filter. These typically involve solving one or more algorithmic problems within a given time frame. To prepare:
- Practice coding problems on platforms like LeetCode, HackerRank, or AlgoCademy
- Familiarize yourself with common data structures and algorithms
- Work on improving your problem-solving speed
2. The Phone Screen
If you pass the initial screening, you’ll likely move on to a phone screen. This is usually a brief conversation with a recruiter or a technical team member.
2.1 Non-Technical Phone Screen
A non-technical phone screen typically focuses on:
- Verifying your background and experience
- Assessing your interest in the role and company
- Discussing your career goals and expectations
- Providing information about the role and company culture
2.2 Technical Phone Screen
A technical phone screen may involve:
- Basic technical questions about your experience and skills
- A coding problem solved in a shared online editor
- Discussion of your approach to problem-solving
To excel in the phone screen:
- Research the company thoroughly
- Prepare concise answers to common behavioral questions
- Be ready to code in a shared environment
- Practice explaining your thought process clearly
3. The On-Site Interview
The on-site interview (which may be conducted virtually in some cases) is the most comprehensive part of the process. It typically consists of multiple rounds, each focusing on different aspects of your skills and fit for the role.
3.1 Coding Interviews
Coding interviews are the cornerstone of the software engineering interview process. You’ll be expected to solve algorithmic problems in real-time, often on a whiteboard or in a shared coding environment. Key aspects include:
- Problem-solving skills
- Code quality and efficiency
- Ability to optimize solutions
- Communication of your thought process
To prepare for coding interviews:
- Study fundamental data structures (arrays, linked lists, trees, graphs, etc.)
- Master common algorithms (sorting, searching, dynamic programming, etc.)
- Practice coding without an IDE to simulate whiteboard conditions
- Learn to analyze time and space complexity
Here’s an example of a typical coding problem you might encounter:
// Problem: Implement a function to reverse a linked list
class ListNode {
int val;
ListNode next;
ListNode(int x) { val = x; }
}
public ListNode reverseList(ListNode head) {
ListNode prev = null;
ListNode current = head;
while (current != null) {
ListNode nextTemp = current.next;
current.next = prev;
prev = current;
current = nextTemp;
}
return prev;
}
3.2 System Design Interviews
System design interviews assess your ability to design large-scale distributed systems. These are particularly important for more senior roles. Key aspects include:
- Understanding of scalability and performance
- Knowledge of various components of distributed systems
- Ability to make trade-offs and justify decisions
To prepare for system design interviews:
- Study common system design patterns and architectures
- Understand concepts like load balancing, caching, and database sharding
- Practice designing popular systems (e.g., a URL shortener, a social media feed)
- Learn to estimate system capacity and performance requirements
3.3 Behavioral Interviews
Behavioral interviews assess your soft skills and cultural fit. They often involve questions about your past experiences and how you handled specific situations. Key aspects include:
- Communication skills
- Teamwork and collaboration
- Problem-solving approach
- Leadership potential
To prepare for behavioral interviews:
- Use the STAR (Situation, Task, Action, Result) method to structure your answers
- Prepare examples of past projects and challenges you’ve overcome
- Research the company’s values and culture to align your responses
- Practice active listening and asking clarifying questions
3.4 Domain-Specific Interviews
Depending on the role and your experience, you may face domain-specific interviews. These could cover:
- Front-end development (HTML, CSS, JavaScript frameworks)
- Back-end development (databases, APIs, server technologies)
- Mobile development (iOS, Android)
- Machine learning and AI
- DevOps and infrastructure
To prepare for domain-specific interviews:
- Review the latest trends and best practices in your domain
- Be ready to discuss projects you’ve worked on in detail
- Understand the trade-offs between different technologies in your field
4. The Mock Interview: A Crucial Preparation Step
One of the most effective ways to prepare for a software engineering interview is to participate in mock interviews. These simulated interview experiences can help you:
- Get comfortable with the interview format
- Improve your ability to think and code under pressure
- Receive feedback on your performance
- Identify areas for improvement
Consider using platforms like AlgoCademy, which offer AI-powered mock interviews and personalized feedback to help you refine your skills.
5. Common Pitfalls and How to Avoid Them
Even well-prepared candidates can fall into common traps during interviews. Here are some pitfalls to watch out for:
5.1 Jumping into Coding Too Quickly
Many candidates start coding immediately after hearing the problem. Instead:
- Take time to understand the problem thoroughly
- Ask clarifying questions
- Discuss your approach before coding
5.2 Poor Communication
Failing to explain your thought process can hurt your chances. To improve:
- Think out loud as you solve problems
- Explain your reasoning for design decisions
- Be open to feedback and suggestions
5.3 Neglecting Edge Cases
Overlooking edge cases can indicate a lack of thoroughness. To avoid this:
- Consider boundary conditions
- Think about potential error scenarios
- Test your solution with various inputs
5.4 Failing to Optimize
Presenting an inefficient solution without attempting to optimize can be a red flag. Instead:
- Start with a working solution, then optimize
- Discuss trade-offs between different approaches
- Analyze the time and space complexity of your solution
6. Post-Interview Steps
The interview process doesn’t end when you walk out of the room (or close the video call). Here’s what to do after your interview:
6.1 Send a Thank You Note
Within 24 hours of your interview, send a personalized thank you email to your interviewers. This shows professionalism and keeps you fresh in their minds.
6.2 Reflect on Your Performance
Take some time to review your interview experience:
- What questions were challenging?
- How could you have improved your responses?
- What areas do you need to study more?
6.3 Follow Up
If you haven’t heard back within the timeframe provided, it’s appropriate to follow up with your recruiter for an update on the process.
7. Continuous Learning and Improvement
Regardless of the outcome, each interview is a learning opportunity. Use your experiences to continually refine your skills and knowledge. Some ways to do this include:
- Regularly solving coding problems on platforms like AlgoCademy
- Contributing to open-source projects
- Building personal projects to expand your portfolio
- Staying updated with the latest technologies and industry trends
Conclusion
Mastering the software engineering interview process is a journey that requires dedication, practice, and continuous learning. By understanding the anatomy of these interviews and preparing thoroughly for each component, you can significantly increase your chances of success. Remember, the goal is not just to pass the interview, but to demonstrate your problem-solving skills, technical expertise, and potential as a valuable team member.
Whether you’re aiming for a position at a FAANG company or any other tech organization, the skills and strategies outlined in this guide will serve you well. Utilize resources like AlgoCademy to structure your preparation, gain hands-on practice, and receive personalized feedback to refine your skills. With persistence and the right approach, you’ll be well-equipped to tackle even the most challenging software engineering interviews and land your dream job in the tech industry.