The Anatomy of a Software Architect Interview: A Comprehensive Guide
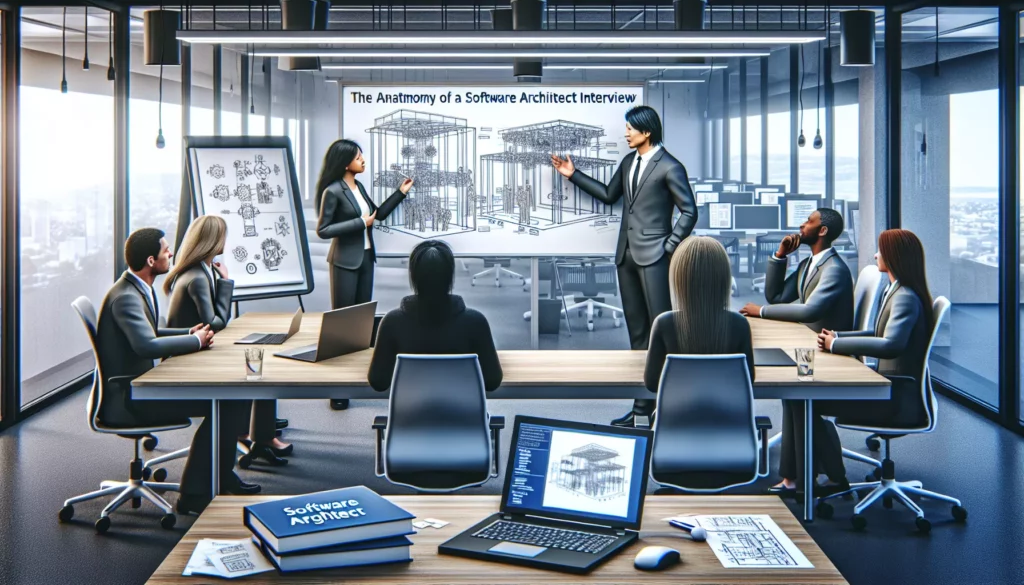
In the ever-evolving landscape of technology, the role of a Software Architect stands as a pinnacle of technical expertise and leadership. As organizations increasingly recognize the critical importance of robust, scalable, and efficient software systems, the demand for skilled Software Architects continues to grow. However, securing a position as a Software Architect involves navigating through a complex and multifaceted interview process that tests not only technical prowess but also soft skills, vision, and leadership potential.
This comprehensive guide will delve into the anatomy of a Software Architect interview, providing aspiring architects and seasoned professionals alike with invaluable insights into what to expect and how to prepare. We’ll explore the various components of the interview process, the key areas of focus, and strategies to showcase your expertise effectively.
1. Understanding the Role of a Software Architect
Before diving into the interview process, it’s crucial to have a clear understanding of what a Software Architect does and the expectations associated with the role. A Software Architect is responsible for:
- Designing high-level software structures and systems
- Making critical decisions about technical standards, tools, and platforms
- Ensuring scalability, performance, and reliability of software solutions
- Bridging the gap between business requirements and technical implementation
- Mentoring and guiding development teams
- Evaluating and mitigating technical risks
- Staying abreast of emerging technologies and industry trends
With this role in mind, let’s explore the typical stages of a Software Architect interview process.
2. The Interview Process: An Overview
The interview process for a Software Architect position typically consists of several stages, each designed to assess different aspects of the candidate’s skills and experience:
- Initial Screening
- Technical Phone Interview
- Coding and Problem-Solving Assessment
- System Design Interview
- Behavioral and Leadership Interview
- Architecture Case Study or Presentation
- Final Panel Interview
Let’s examine each of these stages in detail.
3. Initial Screening
The initial screening is often conducted by a recruiter or HR representative. This stage aims to:
- Verify basic qualifications and experience
- Assess communication skills
- Determine salary expectations and availability
- Provide an overview of the role and company
To prepare for this stage:
- Review your resume and be prepared to discuss your experience in detail
- Research the company and the specific role
- Prepare concise answers to common questions about your career goals and motivations
- Have questions ready about the role and the company
4. Technical Phone Interview
The technical phone interview is typically conducted by a senior technical team member or another architect. This stage assesses your technical knowledge and problem-solving abilities. Key areas of focus include:
- Programming languages and paradigms
- Software design patterns and principles
- Database design and management
- Web services and APIs
- Cloud computing and distributed systems
- Security principles
- Performance optimization
To excel in this stage:
- Review fundamental concepts in software architecture and design
- Practice explaining complex technical concepts clearly and concisely
- Be prepared to discuss your past projects and the architectural decisions you made
- Stay updated on current trends and technologies in software architecture
5. Coding and Problem-Solving Assessment
While Software Architects are not typically involved in day-to-day coding, they are expected to have strong programming skills. This stage may involve:
- Solving algorithmic problems
- Writing clean, efficient code
- Debugging and optimizing existing code
- Explaining your thought process and approach to problem-solving
To prepare for this stage:
- Practice coding problems on platforms like LeetCode, HackerRank, or AlgoCademy
- Review data structures and algorithms
- Practice explaining your thought process while solving problems
- Familiarize yourself with multiple programming languages
Here’s an example of a coding problem you might encounter:
// Problem: Design a cache with a given capacity that evicts the least recently used item when it reaches capacity.
class LRUCache {
private int capacity;
private Map<Integer, Integer> cache;
private Deque<Integer> lruOrder;
public LRUCache(int capacity) {
this.capacity = capacity;
this.cache = new HashMap<>();
this.lruOrder = new LinkedList<>();
}
public int get(int key) {
if (!cache.containsKey(key)) {
return -1;
}
updateLRU(key);
return cache.get(key);
}
public void put(int key, int value) {
if (cache.containsKey(key)) {
updateLRU(key);
} else {
if (cache.size() >= capacity) {
int leastUsed = lruOrder.removeLast();
cache.remove(leastUsed);
}
lruOrder.addFirst(key);
}
cache.put(key, value);
}
private void updateLRU(int key) {
lruOrder.remove(key);
lruOrder.addFirst(key);
}
}
6. System Design Interview
The system design interview is a critical component of the Software Architect interview process. It assesses your ability to design large-scale distributed systems, make appropriate trade-offs, and explain your design decisions. You might be asked to design systems like:
- A social media platform
- An e-commerce website
- A ride-sharing application
- A distributed file storage system
Key areas to focus on during a system design interview include:
- Scalability
- Reliability
- Availability
- Performance
- Security
- Data storage and management
- Caching strategies
- Load balancing
- Microservices architecture
To prepare for this stage:
- Study system design principles and patterns
- Practice designing systems on a whiteboard or using online tools
- Learn to ask clarifying questions to understand requirements
- Be prepared to explain trade-offs in your design decisions
- Familiarize yourself with common system components and their uses
Here’s an example of how you might approach a high-level system design for a social media platform:
<!-- High-level system design for a social media platform -->
1. User Service:
- Handle user registration, authentication, and profile management
- Use a relational database (e.g., PostgreSQL) for user data
- Implement caching (e.g., Redis) for frequently accessed user information
2. Post Service:
- Manage creation, retrieval, and deletion of posts
- Use a NoSQL database (e.g., Cassandra) for scalable storage of posts
- Implement a distributed file system (e.g., HDFS) for media storage
3. News Feed Service:
- Generate personalized news feeds for users
- Use a combination of batch processing (e.g., Hadoop) and real-time processing (e.g., Apache Kafka) for feed generation
4. Notification Service:
- Handle push notifications, email notifications, and in-app notifications
- Use a message queue (e.g., RabbitMQ) for reliable message delivery
5. Search Service:
- Provide full-text search capabilities for posts and users
- Use an search engine (e.g., Elasticsearch) for efficient indexing and searching
6. Analytics Service:
- Collect and analyze user behavior data
- Use a data warehouse (e.g., Amazon Redshift) for storing and querying large datasets
7. CDN (Content Delivery Network):
- Distribute static content and media files globally for faster access
8. Load Balancer:
- Distribute incoming traffic across multiple server instances for improved performance and reliability
9. API Gateway:
- Provide a single entry point for all client requests
- Handle authentication, rate limiting, and request routing
10. Caching Layer:
- Implement distributed caching (e.g., Memcached) to reduce database load and improve response times
7. Behavioral and Leadership Interview
As a Software Architect, you’re expected to possess strong leadership and communication skills. This stage of the interview process assesses your ability to work with diverse teams, handle conflicts, and lead technical initiatives. You may be asked about:
- Your experience leading technical teams
- How you handle disagreements with team members or stakeholders
- Your approach to mentoring and developing junior team members
- How you communicate complex technical concepts to non-technical stakeholders
- Your experience in project management and meeting deadlines
- How you stay updated with new technologies and industry trends
To prepare for this stage:
- Reflect on your past experiences and prepare specific examples that demonstrate your leadership skills
- Practice using the STAR method (Situation, Task, Action, Result) to structure your responses
- Think about challenges you’ve faced and how you overcame them
- Consider how you’ve contributed to the success of your team and organization
8. Architecture Case Study or Presentation
Some companies may require you to prepare and present a case study or give a presentation on a specific architectural topic. This stage allows you to showcase your depth of knowledge, presentation skills, and ability to defend your architectural decisions.
You might be asked to:
- Present an architecture you’ve designed in the past
- Propose an architecture for a given problem statement
- Discuss the evolution of an existing system’s architecture
- Compare different architectural approaches for a specific scenario
To excel in this stage:
- Practice creating clear, concise slides that effectively communicate your ideas
- Be prepared to explain your rationale for key decisions
- Anticipate potential questions and criticisms of your approach
- Consider including diagrams or visual aids to illustrate complex concepts
- Practice your presentation skills, including body language and voice modulation
9. Final Panel Interview
The final stage often involves a panel interview with senior technical leaders and executives. This stage aims to assess your overall fit for the role and the organization. You may be asked about:
- Your long-term career goals
- Your vision for the role of Software Architect in the organization
- How you would approach specific challenges the company is facing
- Your thoughts on emerging technologies and their potential impact
To prepare for this stage:
- Research the company’s products, services, and recent news
- Prepare thoughtful questions about the company’s technical strategy and future plans
- Be ready to discuss how your skills and experience align with the company’s needs
- Practice articulating your personal brand and what sets you apart as a Software Architect
10. Key Skills and Knowledge Areas to Master
Throughout the interview process, certain skills and knowledge areas are consistently valuable. Focus on developing expertise in:
- Software Design Patterns and Principles (e.g., SOLID principles, Gang of Four patterns)
- Distributed Systems Architecture
- Cloud Computing Platforms (e.g., AWS, Azure, Google Cloud)
- Containerization and Orchestration (e.g., Docker, Kubernetes)
- Microservices Architecture
- RESTful API Design
- Database Design and Management (both SQL and NoSQL)
- Security Best Practices
- Performance Optimization Techniques
- Agile and DevOps Methodologies
- Scalability and High Availability Concepts
- Emerging Technologies (e.g., Machine Learning, Blockchain, IoT)
11. Preparation Strategies
To effectively prepare for a Software Architect interview:
- Review fundamental concepts in computer science and software engineering
- Stay updated with the latest trends and technologies in software architecture
- Practice system design exercises regularly
- Enhance your coding skills through platforms like AlgoCademy
- Read technical blogs and books on software architecture
- Participate in open-source projects or hackathons
- Seek mentorship from experienced architects
- Practice explaining complex technical concepts in simple terms
- Develop your soft skills, including communication and leadership
- Create a portfolio of your architectural work and projects
12. Common Pitfalls to Avoid
Be aware of these common mistakes during Software Architect interviews:
- Focusing too much on implementation details and not enough on high-level design
- Failing to ask clarifying questions before diving into a solution
- Neglecting non-functional requirements like scalability, security, and maintainability
- Being inflexible or defensive when receiving feedback on your designs
- Overlooking the business context and focusing solely on technical aspects
- Failing to explain trade-offs in your design decisions
- Neglecting to showcase your leadership and communication skills
13. Post-Interview Follow-Up
After the interview:
- Send a thank-you email to your interviewers within 24 hours
- Reflect on the interview experience and note areas for improvement
- Follow up with the recruiter if you haven’t heard back within the specified timeframe
- If you receive an offer, carefully review the terms and ask any questions you may have
- If you don’t receive an offer, ask for feedback to help improve your performance in future interviews
Conclusion
The journey to becoming a Software Architect is challenging but rewarding. The interview process for this role is comprehensive, testing not only your technical skills but also your ability to think strategically, communicate effectively, and lead teams. By understanding the anatomy of a Software Architect interview and preparing thoroughly, you can significantly increase your chances of success.
Remember that becoming a great Software Architect is an ongoing process of learning and growth. Even if you don’t secure the position on your first attempt, each interview is an opportunity to gain valuable insights and improve your skills. Stay curious, keep learning, and continue to hone your craft. With persistence and dedication, you can achieve your goal of becoming a successful Software Architect.