The Anatomy of a Rust Engineer Interview: What to Expect and How to Prepare
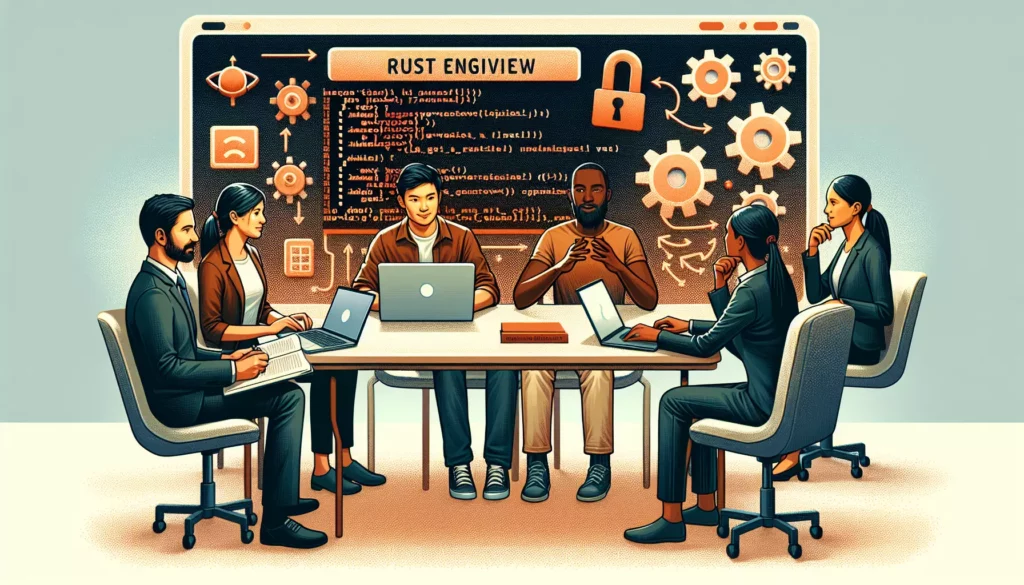
As the demand for Rust engineers continues to grow, more and more developers are finding themselves facing the prospect of a Rust-specific technical interview. Whether you’re a seasoned Rust developer or just starting your journey with this powerful systems programming language, understanding the anatomy of a Rust engineer interview is crucial for success. In this comprehensive guide, we’ll explore what to expect during a Rust interview and provide you with the tools and strategies to prepare effectively.
Understanding the Rust Interview Landscape
Before diving into the specifics of a Rust interview, it’s important to understand why companies are increasingly seeking Rust developers. Rust has gained popularity due to its focus on safety, concurrency, and performance. It’s being used in various domains, including:
- Systems programming
- Web development
- Embedded systems
- Game development
- Blockchain and cryptocurrency
As a result, Rust interviews can vary significantly depending on the company and the specific role you’re applying for. However, there are common elements that you’re likely to encounter in most Rust engineer interviews.
The Structure of a Typical Rust Interview
A Rust interview process typically consists of several stages:
- Initial screening or phone interview
- Technical assessment or coding challenge
- In-depth technical interview(s)
- System design discussion (for more senior roles)
- Behavioral interview
Let’s break down each of these stages and explore what you can expect.
1. Initial Screening or Phone Interview
The first stage is usually a brief conversation with a recruiter or hiring manager. They’ll assess your background, experience with Rust, and overall fit for the role. Be prepared to discuss:
- Your experience with Rust and other programming languages
- Projects you’ve worked on using Rust
- Your understanding of Rust’s key features and benefits
- Your motivation for working with Rust
2. Technical Assessment or Coding Challenge
Many companies include a take-home coding challenge or an online assessment as part of their interview process. This stage aims to evaluate your practical Rust coding skills. You might be asked to:
- Implement a specific algorithm or data structure in Rust
- Build a small application or service using Rust
- Debug and optimize existing Rust code
When completing these challenges, focus on writing clean, idiomatic Rust code that demonstrates your understanding of Rust’s unique features like ownership, borrowing, and lifetimes.
3. In-depth Technical Interview(s)
This is often the most challenging part of the Rust interview process. You’ll typically face one or more technical interviews with Rust engineers or senior developers. These interviews may include:
- Coding exercises on a whiteboard or shared coding environment
- Discussions about Rust concepts and best practices
- Questions about your previous Rust projects
- Problem-solving scenarios related to Rust development
Be prepared to explain your thought process, discuss trade-offs, and demonstrate your problem-solving skills in addition to your Rust knowledge.
4. System Design Discussion (for more senior roles)
For senior Rust engineer positions, you may be asked to participate in a system design discussion. This stage evaluates your ability to architect large-scale systems using Rust. You might be asked to:
- Design a distributed system using Rust
- Discuss how you would implement concurrency in a Rust-based application
- Explain how you would optimize a Rust system for performance
5. Behavioral Interview
The final stage often includes a behavioral interview, which assesses your soft skills and cultural fit. Be ready to discuss:
- Your experience working in teams
- How you handle challenges and conflicts
- Your approach to learning and staying updated with Rust developments
- Your long-term career goals in the Rust ecosystem
Key Topics to Master for a Rust Interview
To excel in a Rust interview, you should have a solid grasp of the following topics:
1. Rust Fundamentals
- Variables and mutability
- Data types and structures
- Functions and methods
- Control flow (if, match, loops)
- Error handling
2. Ownership and Borrowing
- Ownership rules
- Borrowing and references
- Lifetimes
- Smart pointers (Box, Rc, Arc)
3. Concurrency
- Threads and thread safety
- Channels for message passing
- Mutex and atomic types
- Async/await and futures
4. Memory Management
- Stack vs. heap allocation
- RAII (Resource Acquisition Is Initialization)
- Memory safety guarantees
5. Rust Standard Library
- Collections (Vec, HashMap, etc.)
- Iterators and closures
- Option and Result types
- I/O operations
6. Rust Ecosystem
- Cargo and package management
- Popular crates and their use cases
- Testing in Rust
- Rust tooling (rustc, rustfmt, clippy)
Common Rust Interview Questions
While the specific questions you’ll encounter in a Rust interview can vary, here are some common types of questions you should be prepared to answer:
Conceptual Questions
- Explain the concept of ownership in Rust. How does it differ from garbage collection?
- What are lifetimes in Rust, and why are they important?
- Describe the differences between &str and String in Rust.
- How does Rust ensure memory safety without a garbage collector?
- Explain the purpose of the Option and Result types in Rust.
Coding Questions
- Implement a function that reverses a string in place without using additional memory.
- Write a Rust program that demonstrates the use of generics and traits.
- Implement a simple concurrent program using threads and channels.
- Create a custom error type and use it in a function that can return multiple error types.
- Implement a basic data structure (e.g., linked list, binary tree) in Rust.
Problem-Solving Questions
- How would you optimize a Rust program that’s consuming too much memory?
- Describe how you would implement a thread-safe cache in Rust.
- Explain how you would design a Rust library for handling large-scale data processing.
- How would you approach writing a cross-platform GUI application in Rust?
- Discuss the trade-offs between using Arc<Mutex<T>> and channels for sharing data between threads.
Preparing for Your Rust Interview
Now that you understand what to expect in a Rust interview, here are some strategies to help you prepare:
1. Study and Practice Rust Concepts
Review the key topics mentioned earlier and ensure you have a solid understanding of each. Practice implementing these concepts in code. The Rust Book is an excellent resource for this.
2. Solve Coding Problems
Practice solving algorithmic problems using Rust. Platforms like LeetCode, HackerRank, and Exercism offer Rust-specific coding challenges. Focus on writing idiomatic Rust code and leveraging the language’s unique features.
3. Build Projects
Develop small to medium-sized projects using Rust. This will give you practical experience and provide concrete examples to discuss during your interview. Consider contributing to open-source Rust projects as well.
4. Stay Updated
Keep up with the latest developments in the Rust ecosystem. Follow the official Rust blog, participate in Rust forums, and attend Rust conferences or meetups if possible.
5. Practice Explaining Complex Concepts
Work on articulating Rust concepts clearly and concisely. Practice explaining topics like ownership, borrowing, and lifetimes to others, as you’ll likely need to do this during the interview.
6. Mock Interviews
Conduct mock interviews with fellow Rust developers or use online platforms that offer mock interview services. This will help you get comfortable with the interview format and receive feedback on your performance.
Sample Rust Code for Interview Preparation
To help you prepare, here are a few examples of Rust code that demonstrate important concepts often discussed in interviews:
1. Ownership and Borrowing
fn main() {
let s1 = String::from("hello");
let len = calculate_length(&s1);
println!("The length of '{}' is {}.", s1, len);
}
fn calculate_length(s: &String) -> usize {
s.len()
}
2. Error Handling with Result
use std::fs::File;
use std::io::Read;
fn read_file_contents(path: &str) -> Result<String, std::io::Error> {
let mut file = File::open(path)?;
let mut contents = String::new();
file.read_to_string(&mut contents)?;
Ok(contents)
}
fn main() {
match read_file_contents("example.txt") {
Ok(contents) => println!("File contents: {}", contents),
Err(error) => println!("Error reading file: {}", error),
}
}
3. Concurrency with Threads and Channels
use std::thread;
use std::sync::mpsc;
fn main() {
let (tx, rx) = mpsc::channel();
thread::spawn(move || {
let val = String::from("hello from thread");
tx.send(val).unwrap();
});
let received = rx.recv().unwrap();
println!("Got: {}", received);
}
Conclusion
Preparing for a Rust engineer interview can be challenging, but with the right approach and dedication, you can significantly increase your chances of success. Remember that interviewers are not just looking for technical knowledge but also for problem-solving skills, clear communication, and a passion for Rust development.
As you prepare, focus on understanding Rust’s core concepts deeply, practice coding regularly, and work on articulating your thoughts clearly. Don’t forget to showcase your enthusiasm for Rust and your willingness to continue learning and growing in this exciting field.
By following the guidelines and strategies outlined in this article, you’ll be well-equipped to tackle your Rust engineer interview with confidence. Good luck, and may your Rust career be filled with success and innovation!