The Anatomy of a React Engineer Interview: A Comprehensive Guide
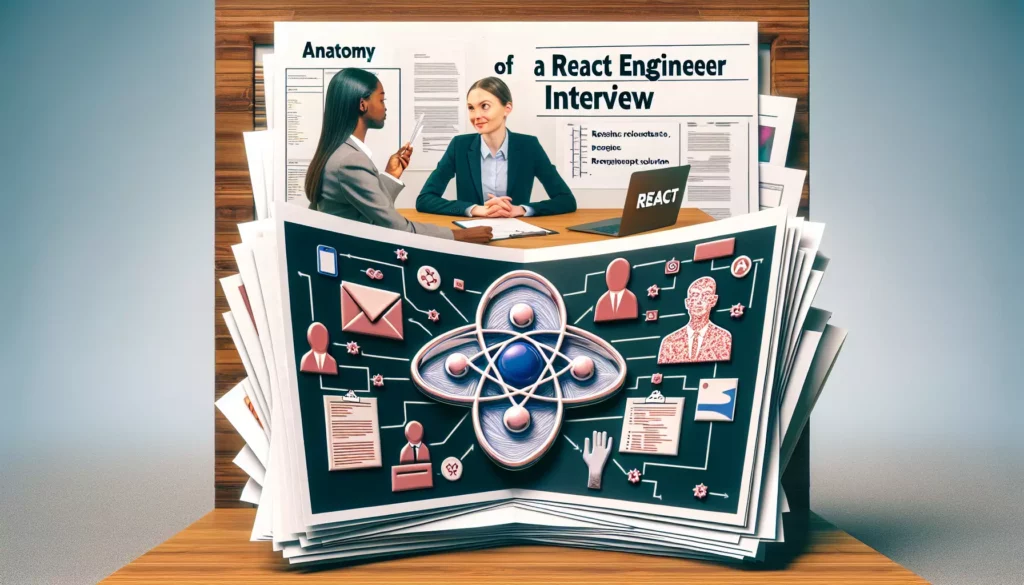
As the demand for skilled React engineers continues to soar, mastering the art of the React interview has become crucial for developers looking to land their dream jobs. Whether you’re a seasoned professional or a newcomer to the React ecosystem, understanding the intricacies of a React engineer interview can significantly boost your chances of success. In this comprehensive guide, we’ll dissect the anatomy of a React engineer interview, providing you with invaluable insights and strategies to help you navigate this challenging process with confidence.
1. The Importance of React in Modern Web Development
Before diving into the specifics of the interview process, it’s essential to understand why React has become such a pivotal technology in the world of web development. React, developed and maintained by Facebook, has revolutionized the way we build user interfaces for web applications. Its component-based architecture, virtual DOM, and efficient rendering mechanisms have made it a go-to choice for companies of all sizes, from startups to tech giants.
The popularity of React has led to a surge in demand for skilled React engineers, making it a highly competitive field. As a result, companies have developed rigorous interview processes to identify the most talented and knowledgeable React developers. Understanding the anatomy of these interviews is crucial for anyone looking to excel in this field.
2. Pre-Interview Preparation
Success in a React engineer interview begins long before you step into the interview room or join a video call. Thorough preparation is key to showcasing your skills and knowledge effectively. Here are some essential steps to take in your pre-interview preparation:
2.1. Brush Up on React Fundamentals
Ensure you have a solid grasp of React’s core concepts, including:
- Components and their lifecycle
- JSX syntax
- State and props
- Hooks (useState, useEffect, useContext, etc.)
- React Router for navigation
- Context API for state management
2.2. Stay Updated with the Latest React Features
React is constantly evolving, so it’s crucial to stay informed about the latest features and best practices. Keep an eye on the official React documentation and popular React blogs to stay up-to-date.
2.3. Practice Coding Challenges
Many React interviews include coding challenges or live coding sessions. Prepare by practicing on platforms like LeetCode, HackerRank, or CodeSignal, focusing on problems that involve React concepts and JavaScript fundamentals.
2.4. Review Your Projects
Be prepared to discuss React projects you’ve worked on in detail. Understand the architecture, challenges faced, and solutions implemented in these projects.
2.5. Familiarize Yourself with Common React Patterns
Study and understand common React patterns and best practices, such as:
- Higher-Order Components (HOCs)
- Render Props
- Compound Components
- Custom Hooks
3. The Interview Process
React engineer interviews typically consist of several stages, each designed to assess different aspects of your skills and knowledge. Let’s break down these stages and explore what you can expect in each:
3.1. Initial Screening
The first stage is often a phone or video call with a recruiter or HR representative. This call is designed to:
- Verify your basic qualifications
- Assess your communication skills
- Discuss your experience with React
- Gauge your interest in the position and company
Tips for success:
- Be concise and clear in your responses
- Show enthusiasm for the role and the company
- Prepare a brief overview of your React experience
- Have questions ready about the company and the role
3.2. Technical Phone Screen
If you pass the initial screening, you’ll likely move on to a technical phone screen with a React engineer or technical team member. This stage typically involves:
- Basic React and JavaScript questions
- Discussion of your past projects and experiences
- A simple coding challenge or conceptual problem-solving exercise
Example questions you might encounter:
- What is the difference between state and props in React?
- Explain the concept of virtual DOM in React.
- How do you handle side effects in React components?
- What are the key differences between class components and functional components?
Tips for success:
- Practice explaining React concepts clearly and concisely
- Be prepared to write code or pseudo-code to illustrate your points
- Have examples ready from your past projects to support your answers
3.3. Coding Challenge
Many companies include a take-home coding challenge as part of their interview process. This challenge is designed to assess your ability to implement React concepts in a practical setting. The challenge might involve:
- Building a small React application from scratch
- Adding features to an existing React codebase
- Refactoring a poorly written React component
- Implementing a specific React pattern or feature
Tips for success:
- Read the requirements carefully and ask for clarification if needed
- Focus on code quality, not just functionality
- Use modern React practices and patterns
- Include comments to explain your thought process
- Consider edge cases and error handling
- If time allows, add unit tests to demonstrate your testing skills
3.4. On-Site or Virtual Technical Interview
The final stage of the interview process is typically an on-site visit or a series of virtual interviews. This stage is the most comprehensive and may include:
- Multiple technical interviews with different team members
- Live coding sessions or whiteboard problem-solving
- System design discussions
- Behavioral interviews
Let’s break down each of these components:
3.4.1. Technical Interviews
These interviews dive deep into your React knowledge and problem-solving skills. You may be asked to:
- Explain complex React concepts in detail
- Discuss trade-offs between different React patterns or libraries
- Analyze and debug React code
- Optimize React components for performance
Example questions:
- How does React’s reconciliation algorithm work?
- Explain the concept of lifting state up in React. When and why would you use it?
- What are the pros and cons of using Redux for state management in a React application?
- How would you optimize the rendering performance of a React component with a large list of items?
3.4.2. Live Coding Sessions
In these sessions, you’ll be asked to write React code in real-time, often while explaining your thought process. Common tasks include:
- Implementing a specific React component or feature
- Solving algorithmic problems using React
- Debugging and refactoring existing React code
Tips for success:
- Practice coding while explaining your thought process out loud
- Start with a high-level approach before diving into implementation details
- Don’t be afraid to ask clarifying questions
- If you’re stuck, explain your thought process and potential approaches
3.4.3. System Design Discussions
For more senior positions, you may be asked to design a large-scale React application or discuss architectural decisions. Topics might include:
- Component structure and organization
- State management strategies
- Performance optimization techniques
- Integration with backend services
Tips for success:
- Start with clarifying the requirements and constraints
- Explain your design decisions and trade-offs
- Consider scalability, maintainability, and performance in your design
- Be prepared to adapt your design based on feedback or new requirements
3.4.4. Behavioral Interviews
These interviews assess your soft skills and cultural fit. You may be asked about:
- Your experience working in teams
- How you handle conflicts or challenges
- Your approach to learning new technologies
- Your career goals and motivations
Tips for success:
- Use the STAR method (Situation, Task, Action, Result) to structure your responses
- Prepare specific examples from your past experiences
- Show enthusiasm for continuous learning and growth
- Demonstrate your ability to collaborate and communicate effectively
4. Key React Concepts to Master
To excel in a React engineer interview, you should have a deep understanding of the following key concepts:
4.1. Component Lifecycle
Understand the lifecycle of both class and functional components, including:
- Mounting, updating, and unmounting phases
- Lifecycle methods in class components
- useEffect hook for managing side effects in functional components
4.2. State Management
Be familiar with various state management approaches, including:
- Local component state using useState
- Context API for sharing state across components
- Redux and other state management libraries
4.3. Hooks
Master the use of built-in hooks and be able to create custom hooks:
- useState for local state management
- useEffect for side effects
- useContext for consuming context
- useReducer for complex state logic
- useMemo and useCallback for performance optimization
4.4. Performance Optimization
Understand techniques for optimizing React applications:
- Memoization with React.memo and useMemo
- Code splitting and lazy loading
- Virtualization for large lists
- Profiling and identifying performance bottlenecks
4.5. Testing
Be familiar with testing React components and applications:
- Unit testing with Jest and React Testing Library
- Integration testing
- End-to-end testing with tools like Cypress
5. Common Pitfalls and How to Avoid Them
Even experienced React developers can fall into certain traps during interviews. Here are some common pitfalls and how to avoid them:
5.1. Overengineering Solutions
In an effort to impress, candidates sometimes propose overly complex solutions to simple problems. Remember that simplicity and readability are valued in professional codebases.
How to avoid:
- Start with the simplest solution that meets the requirements
- Explain your thought process and mention potential optimizations if needed
- Be prepared to discuss trade-offs between simple and more complex approaches
5.2. Neglecting Best Practices
In the heat of the moment, it’s easy to forget about React best practices and patterns.
How to avoid:
- Review React best practices regularly
- Practice implementing common patterns in your coding exercises
- Explain the reasoning behind your code structure and decisions
5.3. Failing to Communicate Effectively
Technical skills alone are not enough; clear communication is crucial in a collaborative development environment.
How to avoid:
- Practice explaining complex concepts in simple terms
- Ask clarifying questions when needed
- Think out loud during problem-solving to showcase your thought process
5.4. Ignoring Edge Cases
Focusing solely on the happy path and neglecting error handling or edge cases is a common mistake.
How to avoid:
- Always consider potential error scenarios
- Discuss how you would handle edge cases, even if you don’t implement them all
- Show awareness of security and performance implications
6. Post-Interview Follow-Up
The interview process doesn’t end when you leave the room or end the video call. Proper follow-up can leave a lasting positive impression:
- Send a thank-you email to your interviewers within 24 hours
- Reiterate your interest in the position and the company
- If you thought of a better solution to a problem after the interview, it’s okay to mention it briefly
- Ask about the next steps in the process
7. Continuous Learning and Improvement
The field of React development is constantly evolving. To stay competitive and excel in interviews, commit to continuous learning:
- Follow React blogs, podcasts, and YouTube channels
- Contribute to open-source React projects
- Attend React conferences and meetups (virtual or in-person)
- Build side projects to experiment with new React features and patterns
- Participate in coding challenges and hackathons
Conclusion
Mastering the React engineer interview is a journey that requires dedication, practice, and a deep understanding of React’s ecosystem. By thoroughly preparing, understanding the interview process, mastering key concepts, avoiding common pitfalls, and committing to continuous learning, you’ll be well-equipped to showcase your skills and land your dream React engineering role.
Remember that each interview is also a learning experience. Reflect on your performance after each interview, identify areas for improvement, and use that knowledge to refine your skills and approach for future opportunities.
As you embark on your React interview journey, stay curious, remain passionate about the technology, and never stop pushing yourself to grow as a developer. With the right mindset and preparation, you’ll be well on your way to acing your next React engineer interview and taking your career to new heights.