The Anatomy of a NextJS Engineer Interview: Mastering the Technical Challenge
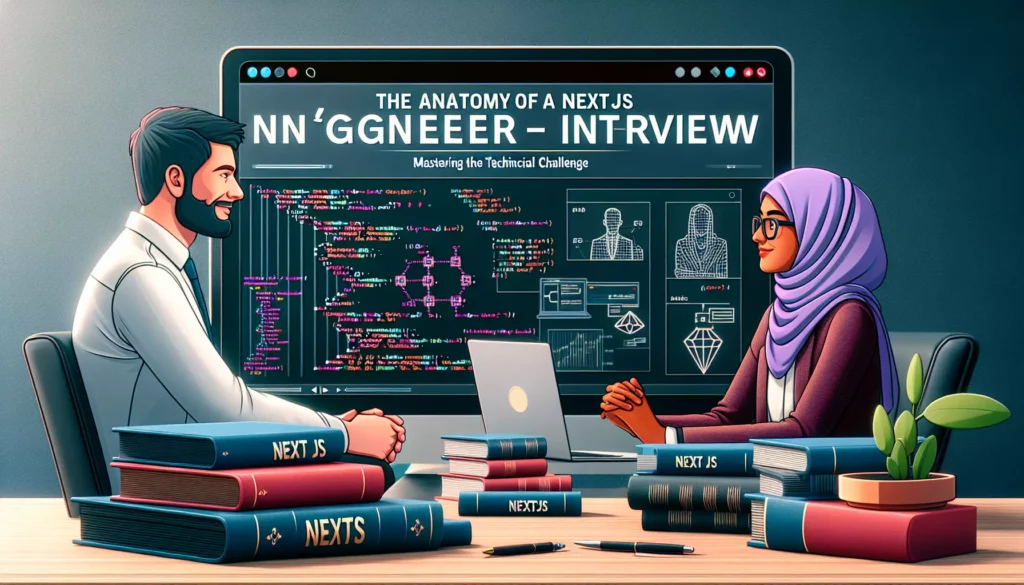
In the ever-evolving landscape of web development, NextJS has emerged as a powerhouse framework, combining the best of React with server-side rendering and static site generation. As its popularity soars, so does the demand for skilled NextJS engineers. If you’re preparing for a NextJS engineer interview, you’re in the right place. This comprehensive guide will dissect the anatomy of a typical NextJS interview, equipping you with the knowledge and strategies to excel.
Understanding the NextJS Interview Landscape
Before we dive into the specifics, it’s crucial to understand that a NextJS engineer interview is multifaceted. It’s not just about your coding skills; it’s a holistic assessment of your problem-solving abilities, your understanding of web development concepts, and your proficiency with NextJS and its ecosystem. Here’s what you can typically expect:
- Technical questions about NextJS concepts
- Coding challenges specific to NextJS applications
- System design discussions
- Behavioral questions to assess your teamwork and communication skills
Core NextJS Concepts You Must Master
To ace your NextJS interview, you need a solid grasp of the framework’s core concepts. Here are the key areas you should focus on:
1. Server-Side Rendering (SSR) vs. Static Site Generation (SSG)
NextJS’s ability to render pages on the server or generate static sites at build time is a game-changer. Be prepared to explain:
- The differences between SSR and SSG
- When to use each approach
- How to implement SSR and SSG in NextJS
Example question: “In a NextJS application, how would you decide whether to use getServerSideProps or getStaticProps for a particular page?”
2. Routing in NextJS
NextJS’s file-based routing system is one of its standout features. Make sure you understand:
- How to create dynamic routes
- The use of catch-all routes
- How to implement programmatic navigation
Example question: “Explain how you would create a dynamic route for blog posts in NextJS, where each post has a unique slug.”
3. API Routes
NextJS allows you to create API endpoints as part of your application. Be ready to discuss:
- How to create and use API routes
- Handling different HTTP methods
- Best practices for API route security
Example question: “How would you implement a protected API route in NextJS that requires authentication?”
4. Data Fetching Methods
NextJS provides various methods for fetching data. Familiarize yourself with:
- getServerSideProps
- getStaticProps
- getStaticPaths
- Client-side data fetching with SWR or React Query
Example question: “Compare and contrast getServerSideProps and getStaticProps. In what scenarios would you choose one over the other?”
5. Image Optimization
NextJS’s Image component is a powerful tool for optimizing images. Be prepared to discuss:
- The benefits of using the Next/Image component
- How to configure and use the Image component
- Best practices for image optimization in NextJS
Example question: “Explain how the Next/Image component improves performance compared to using a standard <img> tag.”
Coding Challenges: What to Expect
Coding challenges are an integral part of the NextJS engineer interview process. These challenges are designed to test your ability to apply NextJS concepts in real-world scenarios. Here are some types of coding challenges you might encounter:
1. Building a Simple NextJS Application
You might be asked to create a basic NextJS application from scratch. This could involve:
- Setting up a new NextJS project
- Creating pages and components
- Implementing basic routing
- Fetching and displaying data
Example challenge: “Create a simple blog application using NextJS. The application should have a home page displaying a list of blog posts and individual pages for each post. Use static site generation for the blog posts.”
2. Optimizing Performance
Performance optimization is crucial in web development. You might be given a scenario where you need to improve the performance of a NextJS application. This could involve:
- Implementing code splitting
- Optimizing images
- Improving data fetching strategies
- Implementing caching mechanisms
Example challenge: “Given a NextJS e-commerce application, implement lazy loading for product images and optimize the product listing page to improve its Lighthouse score.”
3. Integrating with External APIs
Many real-world applications require integration with external APIs. You might be asked to:
- Fetch data from an external API
- Implement server-side rendering with API data
- Handle API errors and loading states
- Implement caching for API responses
Example challenge: “Create a NextJS application that fetches and displays weather data for different cities using a public weather API. Implement server-side rendering and caching to optimize performance.”
4. State Management in NextJS
While NextJS doesn’t prescribe a specific state management solution, you should be familiar with common approaches. You might be asked to:
- Implement global state management using Context API or Redux
- Handle complex form state
- Manage server state using tools like SWR or React Query
Example challenge: “Implement a shopping cart feature in a NextJS e-commerce application. Use Context API for global state management and persist the cart state across page reloads.”
System Design: Thinking at Scale
As a NextJS engineer, you’ll often be asked to think about system design, especially for more senior positions. Here are some areas to focus on:
1. Scalability
Be prepared to discuss how you would scale a NextJS application to handle high traffic. This might involve:
- Implementing caching strategies
- Using Content Delivery Networks (CDNs)
- Optimizing database queries
- Implementing load balancing
Example question: “How would you design a NextJS application to handle millions of daily active users?”
2. Microservices Architecture
Understanding how NextJS fits into a microservices architecture is crucial. Be ready to discuss:
- How to integrate NextJS with microservices
- Implementing API gateways
- Handling inter-service communication
Example question: “Design a microservices architecture for an e-commerce platform where NextJS is used for the frontend. How would you handle product catalog, user authentication, and order processing?”
3. Serverless Deployment
NextJS works well with serverless platforms. Be prepared to discuss:
- Deploying NextJS applications to serverless platforms like Vercel or AWS Lambda
- Advantages and challenges of serverless architecture
- Implementing serverless functions with NextJS API routes
Example question: “Compare deploying a NextJS application to a traditional server versus a serverless platform. What are the trade-offs?”
Behavioral Questions: Showcasing Your Soft Skills
While technical skills are crucial, don’t underestimate the importance of soft skills in a NextJS engineer interview. Here are some common behavioral questions you might encounter:
- “Describe a challenging project you worked on using NextJS. What were the main obstacles, and how did you overcome them?”
- “How do you stay updated with the latest developments in NextJS and the broader JavaScript ecosystem?”
- “Tell me about a time when you had to refactor a large codebase. How did you approach it?”
- “How do you collaborate with designers and backend developers in a project?”
- “Describe a situation where you had to make a difficult technical decision. How did you approach it?”
When answering these questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your experience.
Preparing for Your NextJS Interview: Best Practices
Now that we’ve covered the main components of a NextJS engineer interview, let’s discuss some best practices to help you prepare:
1. Build Projects
There’s no substitute for hands-on experience. Build several NextJS projects, each focusing on different aspects of the framework. This will give you practical experience and projects to discuss during your interview.
2. Contribute to Open Source
Contributing to NextJS or related open-source projects can demonstrate your expertise and commitment to the community. It’s also a great way to learn best practices and stay updated with the latest developments.
3. Practice Coding Challenges
Use platforms like LeetCode, HackerRank, or CodeSignal to practice coding challenges. While these platforms might not have NextJS-specific challenges, they’ll help you improve your problem-solving skills and JavaScript proficiency.
4. Stay Updated
Follow the NextJS blog, Twitter account, and GitHub repository to stay informed about the latest features and best practices. Attend NextJS conferences or watch conference talks online to deepen your understanding.
5. Mock Interviews
Practice with a friend or use mock interview services to get comfortable with the interview process. This can help you identify areas for improvement and reduce anxiety on the day of the actual interview.
6. Review the Company and Role
Research the company you’re interviewing with and the specific role you’re applying for. This will help you tailor your responses and ask relevant questions during the interview.
Common Pitfalls to Avoid
As you prepare for your NextJS engineer interview, be aware of these common pitfalls:
- Neglecting the basics: While NextJS-specific knowledge is important, don’t forget to brush up on fundamental JavaScript and React concepts.
- Overengineering solutions: In coding challenges, aim for clean, efficient solutions rather than overly complex ones.
- Ignoring performance considerations: NextJS is often chosen for its performance benefits. Be prepared to discuss and implement performance optimizations.
- Failing to ask questions: Don’t hesitate to ask for clarification during the interview. It shows engagement and helps you provide more accurate solutions.
- Neglecting to explain your thought process: As you solve problems or answer questions, explain your reasoning. This gives the interviewer insight into how you think and problem-solve.
Conclusion: Embracing the Challenge
A NextJS engineer interview can be challenging, but with thorough preparation and the right mindset, it’s an opportunity to showcase your skills and passion for web development. Remember, the interviewer is not just assessing your current knowledge but also your potential to learn and grow.
As you prepare, leverage resources like the official NextJS documentation, community forums, and online courses. Practice regularly, stay curious, and approach each interview as a learning experience. With dedication and persistence, you’ll be well-equipped to ace your NextJS engineer interview and land your dream role.
Good luck, and may your next NextJS interview be the stepping stone to an exciting career in modern web development!