The Anatomy of a NextJS Engineer Interview: A Comprehensive Guide
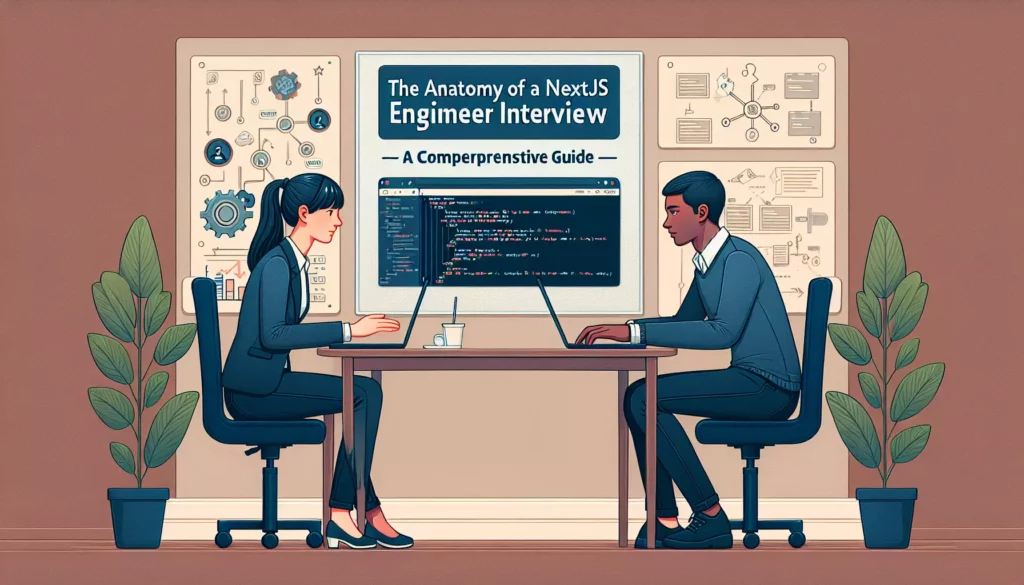
In the ever-evolving landscape of web development, NextJS has emerged as a powerful framework for building modern, server-side rendered React applications. As its popularity continues to grow, so does the demand for skilled NextJS engineers. If you’re preparing for a NextJS engineer interview, it’s crucial to understand the various components that make up this process. This comprehensive guide will walk you through the anatomy of a NextJS engineer interview, providing insights into what to expect and how to prepare effectively.
1. Introduction to NextJS and Its Ecosystem
Before diving into the specifics of the interview process, it’s essential to have a solid understanding of NextJS and its ecosystem. NextJS is a React-based framework that enables server-side rendering, static site generation, and other performance optimizations out of the box. It’s built on top of React and leverages its component-based architecture while providing additional features to enhance the development experience and application performance.
Key aspects of NextJS that you should be familiar with include:
- Server-side rendering (SSR) and static site generation (SSG)
- Automatic code splitting and optimized performance
- Built-in routing system
- API routes for backend functionality
- Image optimization
- TypeScript support
- CSS-in-JS solutions
During your interview, you may be asked to explain these concepts and how they contribute to building efficient web applications.
2. Technical Knowledge Assessment
A significant portion of your NextJS engineer interview will likely focus on assessing your technical knowledge. This section may include questions about React, JavaScript, and NextJS-specific concepts. Here are some areas you should be prepared to discuss:
2.1. React Fundamentals
As NextJS is built on top of React, a strong foundation in React is crucial. Be prepared to answer questions about:
- Components and their lifecycle
- State management (useState, useReducer)
- Context API
- Hooks (useEffect, useMemo, useCallback)
- React performance optimization techniques
2.2. JavaScript Concepts
A solid understanding of JavaScript is essential for any NextJS developer. You may be asked about:
- ES6+ features (arrow functions, destructuring, spread operator)
- Promises and async/await
- Closures and scope
- Event loop and asynchronous programming
- Functional programming concepts
2.3. NextJS-Specific Knowledge
Your interviewer will likely delve into NextJS-specific topics to gauge your expertise. Be prepared to discuss:
- The NextJS build process and how it differs from a standard React application
- Server-side rendering vs. client-side rendering
- Static site generation and when to use it
- The NextJS routing system and how it works
- API routes and their use cases
- NextJS’s image optimization features
- Styling options in NextJS (CSS Modules, Styled Components, Tailwind CSS)
3. Coding Challenges and Problem-Solving
Many NextJS engineer interviews include coding challenges or problem-solving exercises. These are designed to assess your ability to implement solutions using NextJS and related technologies. Here are some types of challenges you might encounter:
3.1. Component Implementation
You may be asked to implement a React component that solves a specific problem or demonstrates a particular concept. For example:
<!-- Example: Implement a countdown timer component -->
import React, { useState, useEffect } from 'react';
const CountdownTimer = ({ initialTime }) => {
const [time, setTime] = useState(initialTime);
useEffect(() => {
if (time > 0) {
const timer = setTimeout(() => setTime(time - 1), 1000);
return () => clearTimeout(timer);
}
}, [time]);
return <div>Time remaining: {time} seconds</div>;
};
export default CountdownTimer;
3.2. NextJS-Specific Implementations
You might be asked to demonstrate your understanding of NextJS-specific features by implementing solutions that leverage these capabilities. For instance:
<!-- Example: Implement a basic API route in NextJS -->
// pages/api/hello.js
export default function handler(req, res) {
res.status(200).json({ message: 'Hello, NextJS!' });
}
3.3. Performance Optimization
Given NextJS’s focus on performance, you may be asked to optimize a piece of code or explain how you would improve the performance of a NextJS application. This could involve techniques like:
- Implementing code splitting
- Optimizing images using NextJS’s Image component
- Implementing caching strategies
- Utilizing server-side rendering or static site generation appropriately
4. System Design and Architecture
For more senior positions, you may be asked to discuss system design and architecture in the context of NextJS applications. This could involve:
4.1. Scalability Considerations
Be prepared to discuss how you would design a NextJS application to handle high traffic and scale effectively. This might include topics like:
- Implementing caching strategies (e.g., using Redis)
- Leveraging CDNs for static assets
- Designing efficient database schemas and queries
- Implementing load balancing for API routes
4.2. State Management at Scale
For large applications, state management becomes crucial. Be ready to discuss:
- When to use global state management solutions (e.g., Redux, MobX)
- Implementing server-side state management
- Optimizing data fetching and caching strategies
4.3. Microservices and NextJS
You might be asked about integrating NextJS applications into a microservices architecture. Topics could include:
- Designing API gateways for NextJS applications
- Implementing service discovery and communication between microservices
- Handling authentication and authorization in a distributed system
5. Testing and Quality Assurance
Quality assurance is a crucial aspect of any software development process. In a NextJS engineer interview, you may be asked about your approach to testing and ensuring code quality. Be prepared to discuss:
5.1. Unit Testing
Demonstrate your knowledge of unit testing React components and NextJS-specific features. You might be asked to write a simple unit test using a framework like Jest:
import { render, screen } from '@testing-library/react';
import Home from '../pages/index';
describe('Home', () => {
it('renders a heading', () => {
render(<Home />);
const heading = screen.getByRole('heading', { name: /welcome to next\.js!/i });
expect(heading).toBeInTheDocument();
});
});
5.2. Integration Testing
Discuss your approach to integration testing in a NextJS application, including:
- Testing API routes
- Testing server-side rendering functionality
- Testing client-side navigation
5.3. End-to-End Testing
Be prepared to talk about end-to-end testing strategies for NextJS applications, such as:
- Using tools like Cypress or Playwright
- Setting up test environments
- Implementing continuous integration and deployment (CI/CD) pipelines
6. Performance Monitoring and Optimization
As a NextJS engineer, you should be familiar with performance monitoring and optimization techniques. Be ready to discuss:
6.1. Performance Metrics
Understand and be able to explain key performance metrics for web applications, such as:
- First Contentful Paint (FCP)
- Largest Contentful Paint (LCP)
- Time to Interactive (TTI)
- Cumulative Layout Shift (CLS)
6.2. Performance Monitoring Tools
Be familiar with tools and techniques for monitoring NextJS application performance, including:
- NextJS Analytics
- Lighthouse
- Chrome DevTools Performance tab
- Third-party monitoring services (e.g., New Relic, Datadog)
6.3. Optimization Techniques
Demonstrate your knowledge of various optimization techniques specific to NextJS applications:
- Implementing dynamic imports for code splitting
- Optimizing images using the NextJS Image component
- Leveraging getStaticProps and getServerSideProps for efficient data fetching
- Implementing caching strategies for API routes
7. Deployment and DevOps
Understanding the deployment process and DevOps practices is crucial for a NextJS engineer. Be prepared to discuss:
7.1. Deployment Platforms
Familiarize yourself with various deployment options for NextJS applications, such as:
- Vercel (the platform created by the NextJS team)
- Netlify
- AWS (using services like Elastic Beanstalk or ECS)
- Google Cloud Platform
- Heroku
7.2. Containerization
Be ready to discuss containerization strategies for NextJS applications:
- Creating Dockerfiles for NextJS apps
- Implementing multi-stage builds for optimized production images
- Orchestrating containers using Kubernetes or Docker Swarm
7.3. Continuous Integration and Deployment (CI/CD)
Demonstrate your understanding of CI/CD practices for NextJS applications:
- Setting up GitHub Actions or GitLab CI for automated testing and deployment
- Implementing staging environments for pre-production testing
- Managing environment variables and secrets in a CI/CD pipeline
8. Soft Skills and Collaboration
While technical skills are crucial, soft skills and the ability to collaborate effectively are equally important in a NextJS engineer role. Be prepared to discuss:
8.1. Communication Skills
Demonstrate your ability to communicate complex technical concepts clearly and concisely. This might involve:
- Explaining NextJS concepts to non-technical team members
- Documenting code and architectural decisions
- Presenting technical solutions to stakeholders
8.2. Teamwork and Collaboration
Discuss your experience working in team environments and your approach to collaboration:
- Participating in code reviews
- Mentoring junior developers
- Contributing to team discussions and decision-making processes
8.3. Problem-Solving and Adaptability
Highlight your problem-solving skills and ability to adapt to new challenges:
- Describe a difficult technical problem you’ve solved using NextJS
- Discuss how you stay updated with the rapidly evolving NextJS ecosystem
- Share your approach to learning new technologies and frameworks
9. Project Experience and Case Studies
Be prepared to discuss your previous experience with NextJS projects in detail. This might include:
9.1. Project Overview
Provide a high-level overview of a significant NextJS project you’ve worked on:
- The project’s goals and objectives
- Your role and responsibilities
- The tech stack and architecture used
9.2. Challenges and Solutions
Discuss specific challenges you encountered during the project and how you overcame them:
- Performance optimization issues
- Scalability challenges
- Integration with legacy systems
9.3. Lessons Learned
Reflect on the lessons learned from your NextJS projects:
- Best practices you’ve adopted
- Mistakes you’ve made and how you’ve grown from them
- Insights into working with NextJS at scale
10. Future of NextJS and Web Development
Demonstrate your passion for the field by discussing your thoughts on the future of NextJS and web development:
10.1. Emerging Trends
Share your insights on emerging trends in the NextJS ecosystem:
- Server Components and React Server Components
- Edge Computing and NextJS Edge Runtime
- Incremental Static Regeneration (ISR) improvements
10.2. Potential Challenges
Discuss potential challenges facing NextJS and web development in general:
- Balancing performance and developer experience
- Addressing accessibility concerns in modern web applications
- Navigating the increasingly complex web development ecosystem
10.3. Your Vision
Share your vision for the future of web development and how NextJS fits into that picture:
- How do you see NextJS evolving in the coming years?
- What features or improvements would you like to see in future NextJS releases?
- How do you plan to contribute to the NextJS community and ecosystem?
Conclusion
Preparing for a NextJS engineer interview requires a comprehensive understanding of the framework, its ecosystem, and the broader context of modern web development. By focusing on the areas outlined in this guide – from technical knowledge and coding skills to system design, testing, and soft skills – you’ll be well-equipped to showcase your expertise and passion for NextJS development.
Remember that interviews are not just about demonstrating your current knowledge, but also about showing your potential for growth and your ability to adapt to new challenges. Approach your NextJS engineer interview with confidence, curiosity, and a willingness to learn, and you’ll be well on your way to success in your career as a NextJS developer.
Good luck with your interview preparation, and may your journey in the world of NextJS be filled with exciting opportunities and continuous learning!