The Anatomy of a Go Engineer Interview: Mastering the Process
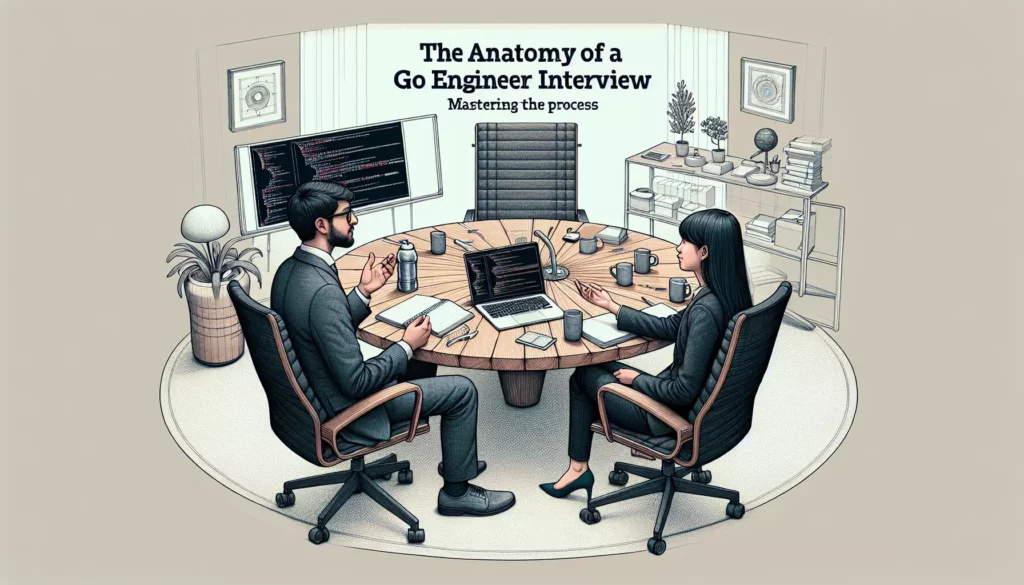
In the fast-paced world of software development, Go (or Golang) has emerged as a powerhouse language, known for its simplicity, efficiency, and robust standard library. As more companies adopt Go for their backend systems, microservices, and cloud-native applications, the demand for skilled Go engineers has skyrocketed. If you’re aspiring to land a job as a Go engineer or looking to level up your interview skills, understanding the anatomy of a Go engineer interview is crucial.
This comprehensive guide will walk you through the various components of a typical Go engineer interview, providing insights, tips, and strategies to help you succeed. We’ll cover everything from the initial screening to the final stages, focusing on the unique aspects of Go programming and the skills that interviewers are looking for in potential candidates.
1. The Initial Screening
The journey to becoming a Go engineer often begins with an initial screening process. This phase is designed to filter out candidates who don’t meet the basic requirements for the position. Here’s what you can expect:
Resume Review
Your resume is your first opportunity to make an impression. Ensure it highlights your Go-specific skills and experiences. Include:
- Go projects you’ve worked on
- Contributions to open-source Go projects
- Go-related certifications or courses
- Experience with Go tools and frameworks (e.g., Gin, Echo, Gorilla)
Phone or Video Screening
If your resume passes muster, you’ll likely have a brief phone or video call with a recruiter or hiring manager. They’ll ask about your background and may pose some basic Go-related questions. Be prepared to discuss:
- Your experience with Go and why you chose to learn it
- Basic Go concepts (e.g., goroutines, channels, interfaces)
- Recent Go projects you’ve worked on
- Your understanding of concurrent programming in Go
2. Technical Assessment
Once you’ve cleared the initial screening, you’ll face a more rigorous technical assessment. This stage is crucial in evaluating your practical Go programming skills.
Coding Challenge
Many companies send out a take-home coding challenge or require candidates to complete an online coding test. These challenges often involve:
- Implementing a specific algorithm or data structure in Go
- Building a small Go application or microservice
- Solving Go-specific puzzles or optimization problems
When tackling these challenges, focus on:
- Writing clean, idiomatic Go code
- Proper error handling and logging
- Efficient use of Go’s concurrency features
- Writing clear documentation and comments
- Including unit tests for your code
Live Coding Session
Some companies prefer a live coding session where you’ll solve problems in real-time while sharing your screen with the interviewer. This format tests not only your coding skills but also your ability to think on your feet and communicate your thought process.
Tips for live coding sessions:
- Think out loud and explain your approach
- Start with a simple solution and then optimize
- Ask clarifying questions when needed
- Be familiar with Go’s standard library
- Practice coding without relying heavily on IDE features
3. In-Depth Technical Interview
The heart of the Go engineer interview process is the in-depth technical interview. This phase dives deep into your Go knowledge, problem-solving skills, and understanding of software engineering principles.
Go Language Fundamentals
Expect questions that test your understanding of Go’s core concepts:
- Goroutines and channels
- Interfaces and type assertions
- Pointers and memory management
- Error handling and panic recovery
- Go’s standard library
Example question: “Explain the difference between buffered and unbuffered channels in Go, and provide an example of when you might use each.”
Concurrency and Parallelism
Given Go’s strength in concurrent programming, be prepared to demonstrate your knowledge in this area:
- Goroutines vs. OS threads
- Synchronization primitives (Mutex, WaitGroup, etc.)
- Concurrency patterns (worker pools, fan-out/fan-in)
- Race conditions and deadlocks
Example question: “Design a concurrent web crawler in Go that respects rate limits for each domain it crawls.”
Data Structures and Algorithms
While Go-specific knowledge is crucial, you’ll also need to showcase your general computer science skills:
- Implementing common data structures in Go (linked lists, trees, graphs)
- Sorting and searching algorithms
- Time and space complexity analysis
- Dynamic programming
Example question: “Implement a concurrent binary search tree in Go with thread-safe insert and search operations.”
System Design
For more senior positions, expect questions about designing scalable systems using Go:
- Microservices architecture with Go
- RESTful API design and implementation
- Database interactions (SQL and NoSQL)
- Caching strategies
- Message queues and event-driven architectures
Example question: “Design a high-throughput, fault-tolerant URL shortener service using Go and explain how you’d handle scaling issues.”
4. Coding Best Practices and Tools
Go places a strong emphasis on clean, readable code. Be prepared to discuss and demonstrate:
Go Code Style and Idioms
- Following the official Go style guide
- Using gofmt for consistent formatting
- Implementing common Go idioms (e.g., error wrapping, functional options)
Testing in Go
- Writing unit tests using the testing package
- Table-driven tests
- Benchmarking and profiling Go code
- Mocking external dependencies
Go Tools and Ecosystem
- Using go mod for dependency management
- Familiarity with go vet, golint, and other static analysis tools
- Continuous Integration/Continuous Deployment (CI/CD) for Go projects
- Containerization of Go applications (Docker)
Example question: “Write a Go function that reads a large CSV file concurrently and processes each row. Include appropriate error handling and unit tests.”
5. Behavioral Interview
While technical skills are crucial, companies also want to ensure you’re a good cultural fit and can work effectively in a team. Prepare for questions about:
- Your experience working on Go projects in a team setting
- How you handle code reviews and feedback
- Your approach to learning new technologies
- Dealing with disagreements or conflicts in a professional setting
- Your contributions to the Go community (if any)
Example question: “Tell me about a time when you had to optimize a poorly performing Go application. What was your approach, and what was the outcome?”
6. The Final Stages
If you’ve made it this far, congratulations! The final stages of a Go engineer interview process may include:
Take-Home Project
Some companies assign a more substantial project to be completed over a few days. This allows you to showcase your skills in a real-world scenario. Tips for success:
- Follow the project requirements closely
- Implement proper error handling and logging
- Write clear documentation, including a README
- Include unit tests and benchmarks
- Be prepared to explain your design decisions
On-Site Interview (or Virtual Equivalent)
The final round often involves multiple interviews with different team members, including potential colleagues and senior leadership. Be prepared to:
- Dive deeper into technical discussions
- Explain your thought process for solving complex problems
- Discuss your career goals and how they align with the company’s vision
- Ask thoughtful questions about the team, projects, and company culture
7. Preparation Strategies
To excel in a Go engineer interview, consider the following preparation strategies:
Practice Coding
- Solve algorithm problems on platforms like LeetCode or HackerRank, focusing on Go implementations
- Contribute to open-source Go projects to gain real-world experience
- Build side projects in Go to showcase on your GitHub profile
Study Go-Specific Resources
- Read “The Go Programming Language” by Alan A. A. Donovan and Brian W. Kernighan
- Follow the Go blog and release notes to stay updated on language features
- Explore Go’s official documentation and standard library
Mock Interviews
- Practice with peers or use online platforms that offer mock technical interviews
- Record yourself explaining Go concepts to improve your communication skills
Stay Informed
- Follow Go-related blogs, podcasts, and YouTube channels
- Attend Go meetups or conferences (virtual or in-person)
- Engage with the Go community on forums like Reddit’s r/golang or the official Go Slack channel
8. Common Pitfalls to Avoid
As you prepare for your Go engineer interview, be aware of these common pitfalls:
- Neglecting to handle errors properly in your Go code
- Overusing goroutines without considering the overhead
- Failing to leverage Go’s standard library effectively
- Ignoring best practices for writing idiomatic Go code
- Not being familiar with recent Go language features and updates
9. Post-Interview Follow-Up
After your interview, don’t forget to:
- Send a thank-you email to your interviewers
- Reflect on the interview experience and note areas for improvement
- Follow up with the recruiter if you haven’t heard back within the expected timeframe
Conclusion
Mastering the Go engineer interview process requires a combination of deep technical knowledge, problem-solving skills, and effective communication. By understanding the anatomy of the interview and preparing thoroughly, you’ll be well-equipped to showcase your Go expertise and land your dream job.
Remember that interviewing is a skill that improves with practice. Even if you don’t succeed on your first attempt, each interview is an opportunity to learn and grow as a Go engineer. Stay persistent, keep coding, and embrace the Go philosophy of simplicity and efficiency in your journey to becoming a top-tier Go developer.
Good luck with your Go engineer interviews, and may your code run as smoothly as a well-optimized goroutine!