The Anatomy of a Game Developer Interview: Navigating Your Path to Success
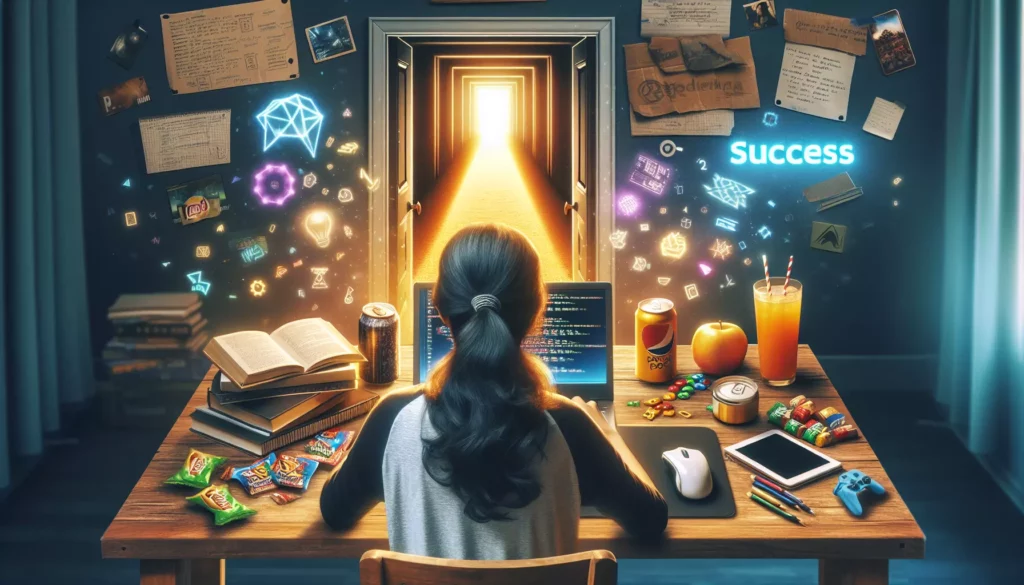
In the ever-evolving world of video game development, landing your dream job requires more than just a passion for gaming. Whether you’re aspiring to create immersive console experiences, cutting-edge PC titles, or addictive mobile games, understanding the intricacies of a game developer interview is crucial. This comprehensive guide will walk you through the essential components of a game developer interview, focusing on key skills, technical knowledge, and practical demonstrations that can set you apart in this competitive field.
1. Understanding the Game Development Landscape
Before diving into the specifics of the interview process, it’s important to grasp the current state of the game development industry. The market is diverse, encompassing various platforms:
- Console gaming (PlayStation, Xbox, Nintendo)
- PC gaming
- Mobile gaming (iOS, Android)
- Virtual Reality (VR) and Augmented Reality (AR)
- Cloud gaming services
Each platform has its unique challenges and opportunities, and as a game developer, you should be aware of the trends and technologies shaping the industry. This knowledge will not only help you during the interview but also guide your career path in the long run.
2. Essential Skills for Game Developers
Game development is a multifaceted field that requires a blend of technical expertise and creative prowess. Here are some of the core skills that interviewers will be looking for:
2.1. Programming Languages
Proficiency in programming languages is the foundation of game development. The most sought-after languages include:
- C++: Often considered the industry standard for game development due to its performance and low-level control.
- C#: Commonly used with Unity and other game engines.
- Java: Popular for Android game development.
- Swift: Used for iOS game development.
- Lua: Often used for scripting in game engines.
During your interview, be prepared to discuss your experience with these languages and how you’ve applied them in your projects.
2.2. Game Engines
Familiarity with popular game engines is crucial. The two most prominent engines in the industry are:
- Unity: Widely used for both 2D and 3D game development across multiple platforms.
- Unreal Engine: Known for its high-fidelity graphics and used in many AAA game titles.
Interviewers will likely ask about your experience with these engines, so be ready to discuss projects you’ve worked on and the specific features you’ve utilized.
2.3. 3D Modeling and Animation
While not all game developers need to be expert 3D artists, a basic understanding of 3D modeling and animation principles is valuable. Familiarity with tools like Maya, Blender, or 3ds Max can be a significant advantage.
2.4. Game Physics and Graphics Programming
Understanding the principles of game physics and graphics programming is essential for creating realistic and visually appealing games. Be prepared to discuss concepts such as:
- Collision detection and response
- Particle systems
- Shader programming
- Lighting and shadow techniques
2.5. Multiplayer and Networking
With the rising popularity of online multiplayer games, knowledge of networking principles and multiplayer game architecture is increasingly important. Be ready to discuss topics like:
- Client-server architecture
- Peer-to-peer networking
- Latency compensation techniques
- State synchronization
3. The Interview Process
Game developer interviews often follow a multi-stage process designed to assess your technical skills, problem-solving abilities, and cultural fit. Here’s what you can typically expect:
3.1. Initial Screening
This usually involves a phone or video call with a recruiter or HR representative. They’ll assess your basic qualifications, experience, and interest in the role. Be prepared to discuss your background, notable projects, and why you’re interested in the company.
3.2. Technical Phone Interview
This stage often involves a conversation with a senior developer or technical lead. They’ll dive deeper into your technical knowledge and may ask you to solve coding problems in real-time. Common topics include:
- Algorithm implementation
- Data structures
- Game-specific programming challenges
For example, you might be asked to implement a simple game mechanic or optimize a piece of code for better performance.
3.3. Take-Home Assignment
Many companies include a take-home project as part of the interview process. This could involve creating a small game or implementing a specific feature. For instance, you might be asked to:
- Develop a simple 2D platformer game
- Implement a physics-based puzzle mechanic
- Create a multiplayer lobby system
This stage allows you to showcase your skills in a less pressured environment and gives the company insight into your coding style and problem-solving approach.
3.4. On-Site Interview
The final stage typically involves an on-site visit (or a series of video calls for remote positions). This may include:
- Multiple technical interviews with team members
- Whiteboard coding sessions
- System design discussions
- Behavioral interviews
Be prepared to dive deep into your technical knowledge, discuss your past projects in detail, and demonstrate your problem-solving skills in real-time.
4. Technical Interview Deep Dive
The technical portion of the interview is often the most challenging and important. Here’s a closer look at what you might encounter:
4.1. Coding Challenges
Expect to face coding challenges that test your ability to implement game-related algorithms and data structures. These might include:
- Implementing a basic game loop
- Creating a simple AI for an NPC
- Optimizing a rendering pipeline
- Implementing a pathfinding algorithm
Here’s an example of a simple game loop implementation in C++:
class Game {
private:
bool isRunning;
// Other game state variables
public:
Game() : isRunning(false) {}
void initialize() {
// Initialize game resources
isRunning = true;
}
void processInput() {
// Handle user input
}
void update() {
// Update game state
}
void render() {
// Render game graphics
}
void run() {
initialize();
while (isRunning) {
processInput();
update();
render();
}
cleanup();
}
void cleanup() {
// Free resources and perform cleanup
}
};
Be prepared to explain your code, discuss potential optimizations, and handle follow-up questions about your implementation choices.
4.2. Game Engine Knowledge
If you’re interviewing for a position that uses a specific game engine, like Unity or Unreal, expect in-depth questions about that engine’s architecture and features. For example, with Unity, you might be asked about:
- The Unity component system
- Coroutines and their use cases
- Unity’s physics system
- Optimizing performance in Unity
Here’s a simple example of a Unity MonoBehaviour script that demonstrates basic player movement:
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float moveSpeed = 5f;
private Rigidbody2D rb;
void Start()
{
rb = GetComponent<Rigidbody2D>();
}
void Update()
{
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
Vector2 movement = new Vector2(moveHorizontal, moveVertical);
rb.velocity = movement * moveSpeed;
}
}
Be ready to explain concepts like the difference between Update() and FixedUpdate(), when to use GetComponent(), and how to optimize this code for better performance.
4.3. Graphics and Shader Programming
For more graphics-oriented roles, you might be asked about shader programming and graphics pipelines. Topics could include:
- Vertex and fragment shaders
- Lighting models
- Post-processing effects
- Optimization techniques for mobile or VR platforms
Here’s a simple example of a vertex shader in GLSL:
#version 330 core
layout (location = 0) in vec3 aPos;
layout (location = 1) in vec2 aTexCoord;
out vec2 TexCoord;
uniform mat4 model;
uniform mat4 view;
uniform mat4 projection;
void main()
{
gl_Position = projection * view * model * vec4(aPos, 1.0);
TexCoord = aTexCoord;
}
Be prepared to explain the purpose of each line, discuss how you would modify this shader for different effects, and talk about performance considerations.
4.4. Game Physics
Understanding game physics is crucial for many game development roles. You might be asked about:
- Collision detection algorithms
- Rigid body dynamics
- Soft body physics
- Optimizing physics calculations
Here’s a simple example of implementing basic 2D collision detection:
struct AABB {
Vector2 min;
Vector2 max;
};
bool checkCollision(const AABB& a, const AABB& b) {
return (a.min.x <= b.max.x && a.max.x >= b.min.x) &&
(a.min.y <= b.max.y && a.max.y >= b.min.y);
}
Be ready to discuss more complex collision detection methods, how to handle collision response, and techniques for optimizing physics calculations in a game environment.
5. Demonstrating Your Skills: The Portfolio and Code Samples
Your portfolio and code samples are crucial elements of your game developer interview. They provide tangible evidence of your skills and creativity. Here’s how to make the most of them:
5.1. Building an Impressive Portfolio
Your portfolio should showcase your best work and demonstrate your versatility as a game developer. Consider including:
- Completed games or game prototypes
- Technical demos highlighting specific skills (e.g., a custom shader, a physics simulation)
- Contributions to open-source game projects
- Game jams or hackathon projects
For each project, be prepared to discuss:
- Your role and responsibilities
- The technologies and tools used
- Challenges faced and how you overcame them
- What you learned from the experience
5.2. Preparing Code Samples
Many interviews will ask you to provide code samples. These should be clean, well-commented, and demonstrate your coding style and problem-solving skills. Consider preparing samples that showcase:
- Game mechanics implementation
- AI algorithms
- Optimized rendering techniques
- Networking code for multiplayer games
Here’s an example of a well-commented code sample for a simple inventory system:
using System;
using System.Collections.Generic;
public class InventorySystem
{
// Maximum number of items the inventory can hold
private const int MAX_INVENTORY_SIZE = 20;
// Dictionary to store items and their quantities
private Dictionary<string, int> inventory;
public InventorySystem()
{
inventory = new Dictionary<string, int>();
}
// Add an item to the inventory
public bool AddItem(string itemName, int quantity)
{
// Check if adding the item would exceed the inventory size
if (inventory.Count >= MAX_INVENTORY_SIZE && !inventory.ContainsKey(itemName))
{
Console.WriteLine("Inventory is full. Cannot add new item.");
return false;
}
// If the item already exists, update its quantity
if (inventory.ContainsKey(itemName))
{
inventory[itemName] += quantity;
}
else
{
// Otherwise, add the new item
inventory.Add(itemName, quantity);
}
Console.WriteLine($"Added {quantity} {itemName}(s) to inventory.");
return true;
}
// Remove an item from the inventory
public bool RemoveItem(string itemName, int quantity)
{
if (!inventory.ContainsKey(itemName))
{
Console.WriteLine($"{itemName} not found in inventory.");
return false;
}
if (inventory[itemName] < quantity)
{
Console.WriteLine($"Not enough {itemName} in inventory.");
return false;
}
inventory[itemName] -= quantity;
// If the quantity reaches zero, remove the item entirely
if (inventory[itemName] == 0)
{
inventory.Remove(itemName);
}
Console.WriteLine($"Removed {quantity} {itemName}(s) from inventory.");
return true;
}
// Display the current inventory
public void DisplayInventory()
{
Console.WriteLine("Current Inventory:");
foreach (var item in inventory)
{
Console.WriteLine($"{item.Key}: {item.Value}");
}
}
}
Be prepared to explain your design choices, discuss potential improvements, and how you would integrate this system into a larger game architecture.
6. Soft Skills and Cultural Fit
While technical skills are crucial, game development is a collaborative process that requires strong soft skills. Interviewers will also assess your:
- Communication skills: Can you clearly explain complex technical concepts?
- Teamwork: How well do you work in a team environment?
- Problem-solving: How do you approach challenges and find solutions?
- Adaptability: Can you quickly learn new technologies and adapt to changing project requirements?
- Passion for gaming: Do you have a genuine interest in and knowledge of the gaming industry?
Be prepared to discuss your experiences working in team environments, how you’ve handled conflicts or challenges, and your approach to learning new technologies.
7. Industry Knowledge and Trends
Demonstrating awareness of current industry trends and future directions can set you apart in an interview. Be prepared to discuss topics such as:
- Emerging technologies in game development (e.g., ray tracing, cloud gaming)
- The impact of AI and machine learning on game development
- Cross-platform development and its challenges
- The rise of indie game development and its influence on the industry
- Monetization strategies in games (e.g., free-to-play models, microtransactions)
Show that you’re not just skilled in development, but also understand the broader context of the gaming industry.
8. Preparing for Specific Roles
Game development encompasses various specialized roles. Depending on the position you’re applying for, you may need to focus on specific areas:
8.1. Gameplay Programmer
Focus on demonstrating your ability to implement game mechanics, AI systems, and player interactions. Be prepared to discuss:
- Game loop implementation
- State machines for game logic
- Input handling and player controls
- AI behavior systems
8.2. Graphics Programmer
Emphasize your knowledge of rendering techniques, shader programming, and optimization. Key areas include:
- Graphics APIs (OpenGL, DirectX, Vulkan)
- Shader languages (HLSL, GLSL)
- Rendering pipelines and optimization
- Lighting and shadow techniques
8.3. Engine Programmer
Focus on your understanding of game engine architecture, performance optimization, and low-level systems. Be ready to discuss:
- Memory management
- Multithreading in game engines
- Asset pipeline and resource management
- Cross-platform development challenges
8.4. Network Programmer
Highlight your expertise in multiplayer game architecture and network programming. Key topics include:
- Client-server vs. peer-to-peer architectures
- Latency compensation techniques
- State synchronization and interpolation
- Security considerations in multiplayer games
9. Asking the Right Questions
Remember, an interview is a two-way process. Asking insightful questions not only helps you gather information about the role and company but also demonstrates your genuine interest and engagement. Consider asking:
- What are the biggest technical challenges the team is currently facing?
- How does the company approach work-life balance, especially during crunch periods?
- What opportunities are there for professional development and learning new technologies?
- Can you tell me about the team structure and how different roles collaborate on projects?
- What’s the typical development cycle for a game at this company?
10. Post-Interview Follow-Up
After the interview, it’s important to follow up professionally:
- Send a thank-you email within 24 hours, expressing your appreciation for the opportunity.
- Reiterate your interest in the position and the company.
- If there were any questions you struggled with or topics you’d like to elaborate on, this is a good opportunity to provide additional information.
- If you haven’t heard back within the timeframe they specified, it’s appropriate to send a polite follow-up email inquiring about the status of your application.
Conclusion
Navigating a game developer interview can be challenging, but with thorough preparation and a clear understanding of what to expect, you can approach it with confidence. Remember, the key to success lies not just in your technical skills, but also in your ability to communicate effectively, demonstrate your passion for game development, and showcase your problem-solving abilities.
As you prepare, leverage resources like AlgoCademy to hone your coding skills and algorithmic thinking. Practice implementing game-related algorithms, work on small game projects to build your portfolio, and stay updated with the latest trends in the gaming industry.
With dedication, continuous learning, and a genuine passion for creating immersive gaming experiences, you’ll be well-equipped to ace your game developer interview and embark on an exciting career in this dynamic and creative field. Good luck!