The Anatomy of a Front End Interview: What to Do at Each Step
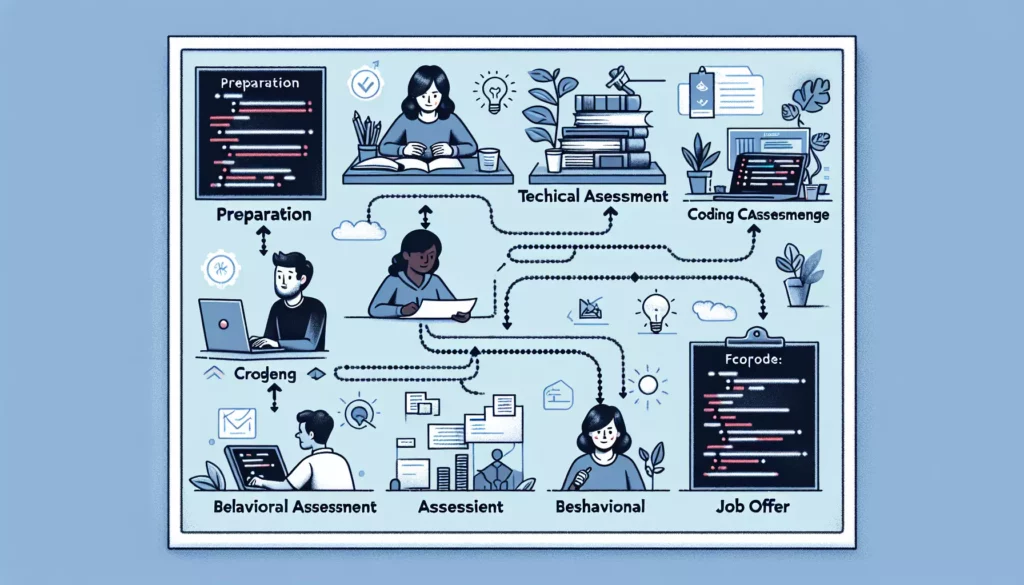
Landing a job as a front-end developer at a top tech company is a dream for many aspiring programmers. However, the interview process can be daunting, especially if you’re not sure what to expect. In this comprehensive guide, we’ll break down the anatomy of a front-end interview and provide you with valuable insights on what to do at each step. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech firm, this article will help you prepare and increase your chances of success.
Table of Contents
- Understanding the Front-End Interview Process
- Preparation: Before the Interview
- Initial Screening: Phone or Video Interview
- Technical Assessment: Coding Challenges and Take-Home Projects
- On-Site Interview: Face-to-Face Evaluations
- System Design and Architecture Discussion
- Behavioral Interview: Soft Skills Assessment
- Closing Interview and Questions
- Post-Interview Follow-Up
- Common Mistakes to Avoid
- Resources for Further Preparation
- Conclusion
1. Understanding the Front-End Interview Process
Before diving into the specifics of each interview stage, it’s crucial to understand the overall structure of a typical front-end interview process. While the exact steps may vary between companies, most follow a similar pattern:
- Initial application and resume screening
- Phone or video screening interview
- Technical assessment (online coding challenge or take-home project)
- On-site interviews (multiple rounds)
- System design and architecture discussion
- Behavioral interview
- Closing interview and opportunity for questions
- Decision and offer negotiation
Understanding this structure will help you prepare adequately for each stage and manage your expectations throughout the process.
2. Preparation: Before the Interview
Proper preparation is key to succeeding in a front-end interview. Here are some essential steps to take before your interview:
Review Core Front-End Technologies
Ensure you have a solid grasp of the following technologies:
- HTML5
- CSS3 (including Flexbox and Grid)
- JavaScript (ES6+ features)
- Popular frameworks (e.g., React, Vue, Angular)
- CSS preprocessors (e.g., Sass, Less)
- Build tools (e.g., Webpack, Babel)
- Version control (Git)
Practice Coding Problems
Utilize platforms like LeetCode, HackerRank, or CodeSignal to practice coding problems, focusing on JavaScript implementations. Pay special attention to:
- Array manipulation
- String operations
- DOM manipulation
- Asynchronous programming (Promises, async/await)
- Basic algorithms and data structures
Study System Design Concepts
Familiarize yourself with front-end system design principles, including:
- Component architecture
- State management
- Performance optimization
- Responsive design
- Progressive enhancement
Prepare Your Portfolio
Curate a portfolio of your best projects, ensuring they showcase a range of skills and technologies. Be prepared to discuss these projects in detail during your interview.
Research the Company
Learn about the company’s products, culture, and recent developments. This knowledge will help you tailor your responses and ask informed questions during the interview.
3. Initial Screening: Phone or Video Interview
The initial screening is typically a brief phone or video call with a recruiter or hiring manager. This stage aims to assess your basic qualifications and interest in the role. Here’s what to do:
Prepare a Brief Self-Introduction
Craft a concise “elevator pitch” that highlights your relevant skills, experience, and interest in the position. For example:
"Hi, I'm [Your Name], a front-end developer with [X] years of experience specializing in React and modern JavaScript. I've worked on projects ranging from e-commerce platforms to data visualization dashboards, and I'm particularly passionate about creating accessible and performant user interfaces. I'm excited about the opportunity to bring my skills to [Company Name] and contribute to [specific product or project]."
Be Ready to Discuss Your Background
Prepare to elaborate on your resume, focusing on projects and experiences most relevant to the position. Use the STAR method (Situation, Task, Action, Result) to structure your responses.
Ask Thoughtful Questions
Prepare a few questions about the role, team, and company. This shows your genuine interest and helps you gather valuable information. Some examples include:
- Can you tell me more about the team I’d be working with?
- What are the biggest challenges facing the front-end team right now?
- How does the company approach continuous learning and professional development for its engineers?
Technical Questions to Expect
While the initial screening is often non-technical, be prepared to answer basic questions about your technical skills, such as:
- What front-end frameworks have you worked with?
- Can you explain the difference between == and === in JavaScript?
- How do you approach responsive design?
4. Technical Assessment: Coding Challenges and Take-Home Projects
Many companies include a technical assessment as part of their interview process. This can take the form of an online coding challenge or a take-home project. Here’s how to approach each:
Online Coding Challenges
These are typically timed assessments conducted on platforms like HackerRank or CodeSignal. To excel in these challenges:
- Read the problem statement carefully and ask for clarification if needed
- Plan your approach before starting to code
- Write clean, well-commented code
- Test your solution with various inputs, including edge cases
- Optimize your solution if time permits
Here’s an example of a typical front-end coding challenge:
/*
Implement a function that takes an array of integers and returns the two numbers that add up to a given target.
If no such pair exists, return an empty array.
Example:
Input: numbers = [2, 7, 11, 15], target = 9
Output: [2, 7]
*/
function findTwoSum(numbers, target) {
const numMap = new Map();
for (let i = 0; i < numbers.length; i++) {
const complement = target - numbers[i];
if (numMap.has(complement)) {
return [complement, numbers[i]];
}
numMap.set(numbers[i], i);
}
return [];
}
// Test the function
console.log(findTwoSum([2, 7, 11, 15], 9)); // Should output [2, 7]
console.log(findTwoSum([3, 2, 4], 6)); // Should output [2, 4]
console.log(findTwoSum([3, 3], 6)); // Should output [3, 3]
console.log(findTwoSum([1, 2, 3, 4], 10)); // Should output []
Take-Home Projects
Take-home projects allow you to showcase your skills in a more realistic scenario. When working on a take-home project:
- Read the requirements thoroughly and ask questions if anything is unclear
- Plan your architecture and component structure before coding
- Focus on code quality, maintainability, and best practices
- Implement proper error handling and edge case management
- Include unit tests for critical functionality
- Provide clear documentation, including setup instructions and any assumptions made
- Go the extra mile with additional features or optimizations if time allows
A typical take-home project might involve building a small application, such as a todo list or a weather app, using a specified framework or library.
5. On-Site Interview: Face-to-Face Evaluations
The on-site interview is often the most comprehensive part of the process, consisting of multiple rounds with different interviewers. Here’s what to expect and how to prepare:
Coding Interviews
You’ll likely face one or more coding interviews where you’ll be asked to solve problems on a whiteboard or computer. To excel in these sessions:
- Think out loud and communicate your thought process
- Ask clarifying questions before diving into the solution
- Start with a brute force approach, then optimize
- Consider edge cases and potential errors
- Test your solution with sample inputs
Here’s an example of a front-end specific coding question you might encounter:
/*
Implement a debounce function that takes a function and a delay as arguments.
The debounced function should only execute after the specified delay has passed since the last call.
Example usage:
const debouncedSearch = debounce(searchAPI, 300);
input.addEventListener('input', debouncedSearch);
*/
function debounce(func, delay) {
let timeoutId;
return function (...args) {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => {
func.apply(this, args);
}, delay);
};
}
// Test the debounce function
function logMessage(message) {
console.log(message);
}
const debouncedLog = debounce(logMessage, 1000);
debouncedLog("Hello"); // This will not log immediately
debouncedLog("World"); // This cancels the previous call and sets a new timeout
// After 1 second, "World" will be logged to the console
UI/UX Design Discussions
Be prepared to discuss UI/UX principles and showcase your design sensibilities. You might be asked to:
- Critique an existing design
- Propose improvements to a user interface
- Explain your approach to creating responsive layouts
Cross-Browser Compatibility
Demonstrate your knowledge of cross-browser issues and how to address them. Be ready to discuss:
- Feature detection vs. browser detection
- Polyfills and their implementation
- CSS prefixing strategies
Performance Optimization
Show your understanding of front-end performance optimization techniques, such as:
- Lazy loading of assets
- Code splitting and bundling
- Minimizing reflows and repaints
- Efficient DOM manipulation
6. System Design and Architecture Discussion
For more senior positions, you may be asked to participate in a system design discussion. This evaluates your ability to design scalable and maintainable front-end architectures. To prepare:
Understand Common Architectures
Familiarize yourself with various front-end architectures, including:
- Micro-frontends
- Server-side rendering (SSR) vs. Client-side rendering (CSR)
- Static site generation (SSG)
- Progressive Web Apps (PWAs)
Know Your Design Patterns
Be ready to discuss and apply common design patterns in front-end development, such as:
- Observer pattern
- Factory pattern
- Module pattern
- MVC/MVVM patterns
Consider Scalability and Performance
When designing a system, always consider:
- How it will scale to handle increased load
- Performance optimizations for large datasets
- Caching strategies
- Load balancing for static assets
Example System Design Question
Here’s an example of a system design question you might encounter:
“Design a real-time collaborative drawing application that allows multiple users to draw on the same canvas simultaneously. Consider aspects such as data synchronization, latency management, and scalability.”
When approaching this question, you might discuss:
- WebSocket implementation for real-time updates
- Canvas API usage for drawing functionality
- State management and conflict resolution strategies
- Optimistic UI updates to handle latency
- Caching and offline support using Service Workers
- Scalable backend architecture to handle multiple concurrent sessions
7. Behavioral Interview: Soft Skills Assessment
The behavioral interview assesses your soft skills, work style, and cultural fit. To excel in this stage:
Use the STAR Method
Structure your responses using the STAR (Situation, Task, Action, Result) method to provide concise and relevant answers.
Prepare Stories
Have several stories ready that demonstrate key competencies such as:
- Teamwork and collaboration
- Problem-solving and critical thinking
- Adaptability and learning from failure
- Leadership and initiative
Common Behavioral Questions
Be prepared to answer questions like:
- Tell me about a time when you had to deal with a difficult team member.
- Describe a situation where you had to learn a new technology quickly.
- How do you handle competing priorities and deadlines?
- Can you share an example of a project that didn’t go as planned? How did you handle it?
Ask Thoughtful Questions
Prepare questions that show your interest in the company culture and your potential role, such as:
- How does the team approach code reviews and knowledge sharing?
- What opportunities are there for professional growth and learning?
- Can you describe a typical day for a front-end developer in this role?
8. Closing Interview and Questions
The closing interview is your opportunity to leave a lasting impression and gather any remaining information you need. Here’s how to make the most of it:
Summarize Your Strengths
Briefly reiterate why you’re a great fit for the role, highlighting key points from your earlier interviews.
Address Any Concerns
If you sensed any hesitation or concerns during the interview process, address them proactively.
Ask Final Questions
Use this opportunity to ask any remaining questions about the role, team, or company. Some good closing questions include:
- What are the next steps in the hiring process?
- Is there anything else I can provide to help you make your decision?
- Based on our discussions, do you have any concerns about my fit for this role?
Express Your Interest
If you’re excited about the opportunity, make sure to express your enthusiasm and reaffirm your interest in the position.
9. Post-Interview Follow-Up
After the interview process is complete, follow up appropriately to maintain a positive impression:
Send Thank-You Notes
Within 24 hours of your interview, send personalized thank-you emails to each interviewer. Reference specific points from your conversations to show your attentiveness and continued interest.
Provide Any Requested Information
If the interviewers asked for any additional information or materials, ensure you provide them promptly.
Follow Up on Timeline
If you haven’t heard back within the expected timeframe, it’s appropriate to send a polite follow-up email inquiring about the status of your application.
10. Common Mistakes to Avoid
To increase your chances of success, avoid these common pitfalls during the front-end interview process:
Neglecting Fundamentals
Don’t focus solely on framework-specific knowledge. Ensure you have a solid grasp of JavaScript, HTML, and CSS fundamentals.
Failing to Ask Questions
Not asking questions can make you appear disinterested. Always have thoughtful questions prepared for each stage of the interview.
Overlooking Soft Skills
Technical skills are crucial, but don’t neglect the importance of communication, teamwork, and problem-solving abilities.
Not Managing Time Effectively
During coding challenges, manage your time wisely. It’s better to have a working solution that’s not fully optimized than an incomplete optimized solution.
Failing to Test Code
Always test your code with various inputs, including edge cases. This demonstrates thoroughness and attention to detail.
Neglecting to Research the Company
Showing up without knowledge of the company’s products, culture, or recent news can make you appear unprepared or disinterested.
11. Resources for Further Preparation
To continue improving your front-end interview skills, consider the following resources:
Online Platforms
- LeetCode: For practicing coding problems
- Frontend Mentor: For real-world project practice
- freeCodeCamp: For comprehensive web development courses
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “You Don’t Know JS” series by Kyle Simpson
- “Eloquent JavaScript” by Marijn Haverbeke
Blogs and Websites
- CSS-Tricks: For in-depth CSS tutorials and techniques
- MDN Web Docs: For comprehensive web technology documentation
- Smashing Magazine: For articles on web design and development
YouTube Channels
- Traversy Media: For web development tutorials
- Web Dev Simplified: For explanations of web development concepts
- DevTips: For front-end development tips and tricks
12. Conclusion
Mastering the front-end interview process requires a combination of technical proficiency, problem-solving skills, and effective communication. By understanding the anatomy of a front-end interview and preparing thoroughly for each stage, you can significantly increase your chances of landing your dream job at a top tech company.
Remember that interview success is not just about having the right answers, but also about demonstrating your thought process, ability to learn, and passion for front-end development. Stay curious, keep practicing, and approach each interview as an opportunity to learn and grow.
With dedication and the right preparation, you’ll be well-equipped to navigate the complexities of front-end interviews and showcase your skills effectively. Good luck with your interviews, and may your journey in front-end development be both challenging and rewarding!