The Anatomy of a Backend Interview: A Step-by-Step Guide to Success
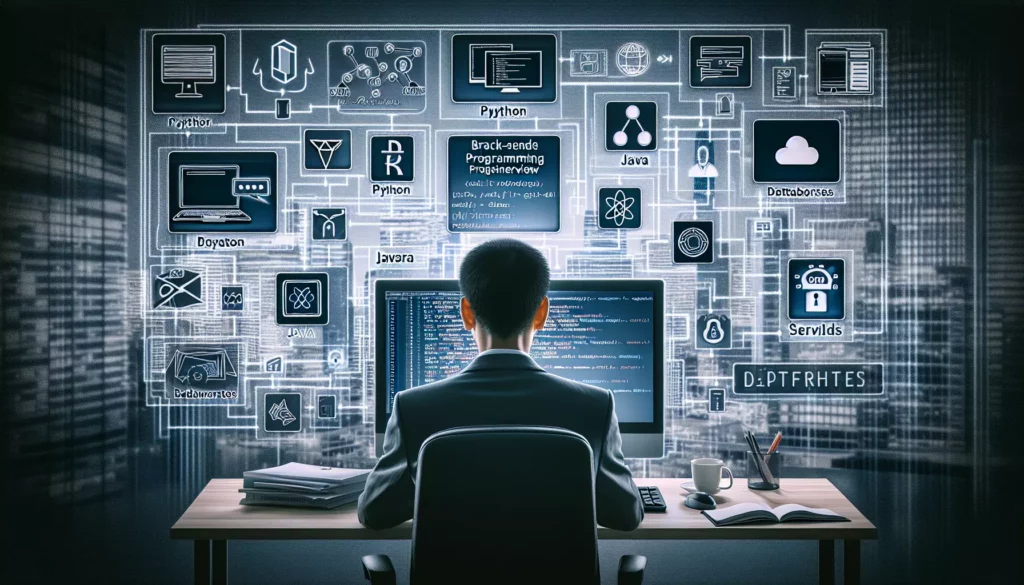
In the competitive world of tech, backend developer positions are highly sought after, and the interview process can be rigorous. Whether you’re a fresh graduate or an experienced developer looking to switch roles, understanding the structure of a backend interview is crucial for success. This comprehensive guide will walk you through each step of a typical backend interview, providing insights and strategies to help you shine.
1. Introduction and Icebreakers
The interview usually begins with introductions and some light conversation. This phase is more than just pleasantries; it’s an opportunity to make a strong first impression.
What to Expect:
- Questions about your background and experience
- Brief overview of your current role or recent projects
- Why you’re interested in the position
How to Prepare:
- Practice a concise self-introduction (30-60 seconds)
- Research the company and position thoroughly
- Prepare questions about the role and company culture
Remember, this is your chance to set a positive tone for the rest of the interview. Be confident, enthusiastic, and genuine in your responses.
2. Technical Background Assessment
After the introductions, the interviewer will likely dive into your technical background. This section aims to understand your experience level and areas of expertise.
What to Expect:
- Questions about technologies you’ve worked with
- Discussions about past projects and your role in them
- Inquiries about your preferred programming languages and frameworks
How to Prepare:
- Review your resume and be ready to discuss each item in detail
- Prepare examples of challenging problems you’ve solved
- Be honest about your skill level in various technologies
Pro tip: Use the STAR method (Situation, Task, Action, Result) when discussing your experiences. This structure helps you provide concise, relevant answers.
3. Conceptual Questions
Backend interviews often include a series of conceptual questions to assess your understanding of fundamental principles and best practices.
Common Topics:
- Database design and optimization
- RESTful API design
- Scalability and performance
- Microservices architecture
- Caching strategies
- Security best practices
How to Prepare:
- Review core computer science concepts
- Stay updated on current backend development trends
- Practice explaining complex concepts in simple terms
Example question: “Can you explain the differences between SQL and NoSQL databases, and when you would choose one over the other?”
4. Coding Challenges
Most backend interviews include one or more coding challenges. These are designed to test your problem-solving skills and coding ability under pressure.
What to Expect:
- Algorithm problems
- Data structure manipulations
- System design questions
- Debugging exercises
How to Prepare:
- Practice coding on a whiteboard or in a simple text editor
- Familiarize yourself with common algorithms and data structures
- Work on explaining your thought process as you code
- Study time and space complexity analysis
Here’s an example of a simple coding challenge you might encounter:
// Write a function that reverses a string
function reverseString(str) {
// Your code here
}
console.log(reverseString("hello")); // Should output: "olleh"
Remember, it’s not just about getting the right answer. Interviewers are often more interested in your problem-solving approach and how you communicate your thoughts.
5. System Design Questions
For more senior positions or roles that involve architecting solutions, you may face system design questions. These assess your ability to design scalable, efficient, and robust systems.
Common Scenarios:
- Designing a social media feed
- Creating a URL shortener service
- Building a distributed cache
- Implementing a real-time chat application
How to Prepare:
- Study common system design patterns and architectures
- Practice drawing system diagrams
- Learn to estimate system requirements (e.g., storage, bandwidth)
- Understand tradeoffs in different design choices
When tackling system design questions, remember to:
- Clarify requirements and constraints
- Start with a high-level design
- Dive into specific components as needed
- Discuss scalability and potential bottlenecks
- Consider failure scenarios and how to handle them
6. Behavioral Questions
While technical skills are crucial, companies also want to ensure you’re a good cultural fit and can work well in a team. Behavioral questions help assess these soft skills.
Common Themes:
- Teamwork and collaboration
- Conflict resolution
- Leadership experiences
- Handling pressure and deadlines
- Adaptability and learning from failures
How to Prepare:
- Reflect on past experiences that demonstrate key soft skills
- Use the STAR method to structure your responses
- Practice answering common behavioral questions
- Be honest and authentic in your answers
Example question: “Tell me about a time when you had to work with a difficult team member. How did you handle the situation?”
7. Database Knowledge
Backend developers often work closely with databases, so expect questions that test your understanding of database concepts and SQL.
Key Areas to Focus On:
- SQL query optimization
- Indexing strategies
- ACID properties
- Normalization and denormalization
- Transactions and locking mechanisms
How to Prepare:
- Practice writing complex SQL queries
- Understand the execution plan of queries
- Learn about different types of indexes and their use cases
- Study database scaling techniques (sharding, replication)
Here’s an example of a SQL question you might encounter:
-- Given a table 'employees' with columns (id, name, department, salary)
-- Write a query to find the top 3 highest paid employees in each department
SELECT department, name, salary
FROM (
SELECT department, name, salary,
DENSE_RANK() OVER (PARTITION BY department ORDER BY salary DESC) as rank
FROM employees
) ranked
WHERE rank <= 3
ORDER BY department, salary DESC;
8. API Design and RESTful Principles
As a backend developer, you’ll likely be involved in designing and implementing APIs. Understanding RESTful principles and best practices is crucial.
Key Concepts to Master:
- HTTP methods and their appropriate use
- Status codes and error handling
- Resource naming conventions
- Versioning strategies
- Authentication and authorization
- Rate limiting and throttling
How to Prepare:
- Design sample APIs for common scenarios
- Understand the principles of idempotency and safety in HTTP methods
- Learn about different API architectures (REST, GraphQL, gRPC)
- Practice explaining API design decisions and tradeoffs
Example question: “Design a RESTful API for a library management system. How would you structure the endpoints for books, users, and borrowing?”
9. Scalability and Performance
Backend systems often need to handle large amounts of data and traffic. Expect questions about scaling applications and optimizing performance.
Topics to Cover:
- Horizontal vs. vertical scaling
- Load balancing techniques
- Caching strategies (e.g., Redis, Memcached)
- Database optimization (indexing, query tuning)
- Asynchronous processing and message queues
- Content Delivery Networks (CDNs)
How to Prepare:
- Study common bottlenecks in backend systems
- Understand the pros and cons of different scaling approaches
- Learn about tools and technologies used for monitoring and profiling
- Practice identifying performance issues in sample architectures
Example scenario: “Our web application is experiencing slow response times during peak hours. Walk me through how you would approach diagnosing and solving this issue.”
10. Security Best Practices
Security is a critical concern in backend development. Be prepared to discuss common vulnerabilities and how to prevent them.
Key Security Concepts:
- Cross-Site Scripting (XSS) prevention
- SQL injection protection
- Cross-Site Request Forgery (CSRF) mitigation
- Secure password storage (hashing, salting)
- HTTPS and TLS
- Input validation and sanitization
How to Prepare:
- Study the OWASP Top 10 web application security risks
- Understand how to implement authentication and authorization correctly
- Learn about secure coding practices in your preferred language
- Practice explaining security concepts to non-technical stakeholders
Example question: “How would you securely store user passwords in a database, and what additional measures would you take to protect user accounts?”
11. Coding Best Practices and Design Patterns
Interviewers often assess your knowledge of software design principles and your ability to write clean, maintainable code.
Areas to Focus On:
- SOLID principles
- Common design patterns (e.g., Singleton, Factory, Observer)
- Code refactoring techniques
- Test-Driven Development (TDD)
- Version control best practices
How to Prepare:
- Review clean code principles and practice applying them
- Implement common design patterns in your preferred language
- Learn to identify code smells and how to refactor them
- Practice writing unit tests for your code
Here’s an example of applying the Single Responsibility Principle:
// Before: A class that does too much
class User {
constructor(name, email) {
this.name = name;
this.email = email;
}
saveToDatabase() {
// Save user to database
}
sendWelcomeEmail() {
// Send welcome email
}
}
// After: Separate responsibilities
class User {
constructor(name, email) {
this.name = name;
this.email = email;
}
}
class UserRepository {
saveUser(user) {
// Save user to database
}
}
class EmailService {
sendWelcomeEmail(user) {
// Send welcome email
}
}
12. Specific Technologies and Frameworks
Depending on the job requirements, you may be asked about specific technologies or frameworks relevant to the role.
Common Backend Technologies:
- Node.js and Express.js
- Django or Flask (Python)
- Ruby on Rails
- Spring Boot (Java)
- ASP.NET Core
How to Prepare:
- Review the job description for required technologies
- Brush up on the core concepts of the relevant frameworks
- Understand the pros and cons of different technologies
- Be prepared to discuss your experience with these tools
Example question: “Can you explain the event loop in Node.js and how it handles asynchronous operations?”
13. Closing the Interview
The final part of the interview is your chance to ask questions and leave a lasting impression.
What to Expect:
- Opportunity to ask questions about the role or company
- Discussion about next steps in the hiring process
- Potential timeline for decision-making
How to Prepare:
- Prepare thoughtful questions about the company’s technology stack, culture, and growth opportunities
- Express your enthusiasm for the position
- Thank the interviewer for their time
- Ask about the next steps and when you can expect to hear back
Remember, this is your last chance to make a positive impression. Show genuine interest in the role and company, and leave the interviewer with a clear understanding of why you’re the right fit for the position.
Conclusion: Putting It All Together
Navigating a backend interview can be challenging, but with proper preparation and the right mindset, you can showcase your skills effectively. Here are some final tips to help you succeed:
- Practice regularly: Use platforms like LeetCode, HackerRank, or AlgoCademy to sharpen your coding skills.
- Stay current: Keep up with the latest trends and technologies in backend development.
- Mock interviews: Practice with friends or mentors to get comfortable with the interview format.
- Be honest: If you don’t know something, admit it and show your willingness to learn.
- Show passion: Demonstrate your enthusiasm for backend development and problem-solving.
- Follow up: Send a thank-you email after the interview, reiterating your interest in the position.
Remember, each interview is a learning experience. Even if you don’t get the job, you’ll gain valuable insights that will help you in future interviews. Stay positive, keep learning, and your dream backend developer role will be within reach.
Good luck with your interviews!