The 17 Books Every Programmer Should Read
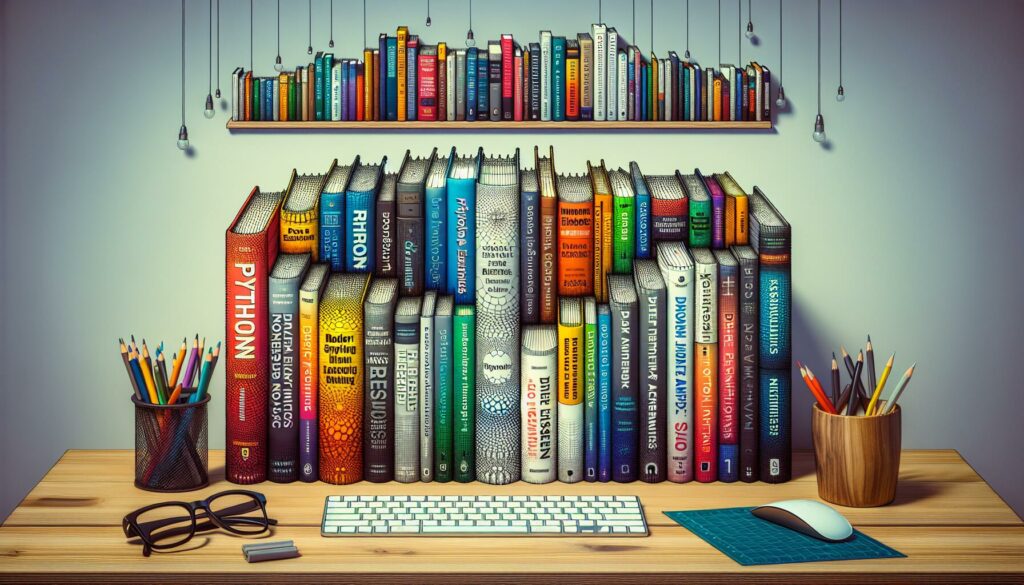
In the ever-evolving world of programming, staying up-to-date with the latest technologies and best practices is crucial. However, there are some timeless books that every programmer should read, regardless of their experience level or preferred programming language. These books offer invaluable insights into the fundamental concepts of computer science, software design, and the art of writing clean, efficient code.
In this comprehensive guide, we’ll explore 17 essential books that can significantly enhance your programming skills and broaden your understanding of the field. Whether you’re a beginner looking to build a solid foundation or an experienced developer aiming to refine your craft, these books have something to offer for everyone.
1. “Clean Code: A Handbook of Agile Software Craftsmanship” by Robert C. Martin
Often referred to as “Uncle Bob,” Robert C. Martin is a software engineer and author known for his expertise in software design and development practices. In “Clean Code,” he provides practical advice on writing code that is easy to read, understand, and maintain.
Key takeaways from this book include:
- The importance of meaningful names for variables, functions, and classes
- How to write functions that do one thing and do it well
- Techniques for organizing code and improving its readability
- Best practices for error handling and writing clean tests
This book is an essential read for programmers of all levels, as it teaches you how to write code that not only works but is also a pleasure to work with.
2. “Code Complete: A Practical Handbook of Software Construction” by Steve McConnell
Steve McConnell’s “Code Complete” is a comprehensive guide to software construction. It covers a wide range of topics, from code organization to debugging techniques, making it an invaluable resource for both novice and experienced programmers.
Some of the key areas covered in this book include:
- Software design techniques and best practices
- Code organization and structure
- Debugging strategies and tools
- The importance of code reviews and pair programming
- Techniques for improving software quality
“Code Complete” is often considered a must-read for anyone serious about software development, as it provides a wealth of practical advice that can be applied to real-world programming scenarios.
3. “The Pragmatic Programmer: Your Journey to Mastery” by Andrew Hunt and David Thomas
This classic book offers a collection of tips and techniques for improving your programming skills and becoming a more effective software developer. The authors draw from their extensive experience to provide practical advice on various aspects of software development.
Key concepts covered in “The Pragmatic Programmer” include:
- The importance of continuous learning and adapting to change
- Techniques for estimating and prioritizing tasks
- The value of automation in software development
- Best practices for debugging and testing
- The importance of clear communication in software projects
This book is particularly valuable for its emphasis on developing a pragmatic mindset, which can help you approach programming challenges more effectively.
4. “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
Often referred to as “CLRS” (after the authors’ last names), this comprehensive textbook is considered the bible of algorithms. While it’s more academic in nature compared to some of the other books on this list, it provides an in-depth understanding of various algorithms and data structures.
Topics covered in this book include:
- Sorting and searching algorithms
- Graph algorithms
- Dynamic programming
- NP-completeness
- Computational geometry
While “Introduction to Algorithms” can be challenging, especially for beginners, it’s an invaluable resource for anyone looking to deepen their understanding of algorithmic thinking and problem-solving.
5. “Design Patterns: Elements of Reusable Object-Oriented Software” by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides
Often referred to as the “Gang of Four” book, this seminal work introduces 23 classic software design patterns that can be used to solve common programming problems. Understanding these patterns can help you write more flexible and maintainable code.
The book categorizes design patterns into three main types:
- Creational patterns: Dealing with object creation mechanisms
- Structural patterns: Concerning the composition of classes or objects
- Behavioral patterns: Characterizing the ways in which classes or objects interact and distribute responsibility
While some of the examples in the book use C++ and Smalltalk, the concepts can be applied to any object-oriented programming language.
6. “Refactoring: Improving the Design of Existing Code” by Martin Fowler
Martin Fowler’s “Refactoring” is a classic guide to the process of restructuring existing code without changing its external behavior. This book is essential for any programmer who wants to improve the quality and maintainability of their code.
Key topics covered in this book include:
- Identifying “code smells” that indicate the need for refactoring
- Step-by-step guides for various refactoring techniques
- How to improve code readability and reduce complexity
- Strategies for refactoring safely and effectively
The second edition of this book uses JavaScript for its code examples, making it more accessible to a wider range of programmers.
7. “The Mythical Man-Month: Essays on Software Engineering” by Frederick P. Brooks Jr.
While not strictly a programming book, “The Mythical Man-Month” offers valuable insights into software project management and the human factors involved in software development. Despite being first published in 1975, many of its observations remain relevant today.
Some key concepts from the book include:
- Brooks’s Law: “Adding manpower to a late software project makes it later”
- The importance of conceptual integrity in software design
- The challenges of communication in large software projects
- The “second-system effect” and its pitfalls
This book is particularly valuable for programmers who are moving into leadership roles or working on large-scale projects.
8. “Cracking the Coding Interview” by Gayle Laakmann McDowell
While primarily aimed at those preparing for technical interviews, “Cracking the Coding Interview” is an excellent resource for any programmer looking to improve their problem-solving skills. The book contains 189 programming questions and solutions, covering a wide range of topics.
Key features of this book include:
- Detailed explanations of various data structures and algorithms
- Tips for approaching and solving complex programming problems
- Insights into the hiring processes at top tech companies
- Advice on soft skills and behavioral aspects of technical interviews
Even if you’re not actively job hunting, working through the problems in this book can significantly enhance your coding skills and algorithmic thinking.
9. “Structure and Interpretation of Computer Programs” by Harold Abelson and Gerald Jay Sussman
Often referred to as SICP, this book is based on an introductory computer science course at MIT. It uses Scheme (a dialect of Lisp) to explore fundamental principles of programming and computer science.
Key topics covered in SICP include:
- Abstraction in software design
- Recursion and iteration
- Data structures and their implementation
- Metalinguistic abstraction
- Object-oriented programming concepts
While the use of Scheme might seem outdated, the concepts taught in this book are timeless and can be applied to any programming language.
10. “Pragmatic Thinking and Learning: Refactor Your Wetware” by Andy Hunt
This book focuses on the cognitive aspects of programming and how to improve your learning and problem-solving abilities. It draws on research from cognitive science and learning theory to provide practical advice for programmers.
Key concepts covered in the book include:
- The Dreyfus model of skill acquisition
- Techniques for more effective learning and retention
- How to manage focus and deal with distractions
- Strategies for debugging your brain
- The importance of reflection and feedback in learning
“Pragmatic Thinking and Learning” is an excellent resource for programmers looking to enhance their cognitive skills and become more effective learners.
11. “Working Effectively with Legacy Code” by Michael Feathers
In the real world, programmers often have to work with existing codebases that may be poorly structured or difficult to understand. Michael Feathers’ book provides strategies for working with and improving such “legacy” code.
Key topics covered in this book include:
- Techniques for safely modifying legacy code
- Strategies for adding tests to untested code
- How to identify and break dependencies in tightly coupled code
- Refactoring techniques for improving code quality
This book is particularly valuable for programmers who frequently work on maintaining or extending existing systems.
12. “The Art of Computer Programming” by Donald Knuth
Donald Knuth’s multi-volume work is considered one of the most comprehensive treatments of computer programming. While it’s not a book you’re likely to read cover-to-cover, it’s an invaluable reference for in-depth exploration of algorithms and data structures.
The volumes cover:
- Fundamental Algorithms
- Seminumerical Algorithms
- Sorting and Searching
- Combinatorial Algorithms (in progress)
Knuth’s work is known for its mathematical rigor and depth, making it an excellent resource for those looking to gain a deep understanding of computer science fundamentals.
13. “Programming Pearls” by Jon Bentley
This classic book presents a collection of programming problems and their solutions, focusing on the process of problem-solving and algorithm design. Each chapter presents a problem and walks through the thought process of solving it efficiently.
Key features of this book include:
- Emphasis on algorithm design and problem-solving techniques
- Discussion of trade-offs in algorithm and data structure choices
- Tips for writing efficient and maintainable code
- Insights into the art of programming from a master practitioner
“Programming Pearls” is an excellent resource for honing your problem-solving skills and learning to think more deeply about algorithm design.
14. “Patterns of Enterprise Application Architecture” by Martin Fowler
For programmers working on large-scale enterprise applications, this book provides a catalog of common architectural patterns. Fowler describes various approaches to structuring complex software systems and discusses their pros and cons.
Topics covered in this book include:
- Domain Logic Patterns
- Data Source Architectural Patterns
- Object-Relational Behavioral Patterns
- Web Presentation Patterns
- Concurrency Patterns
While some of the specific technologies mentioned in the book may be outdated, the architectural principles remain relevant and valuable.
15. “Head First Design Patterns” by Eric Freeman and Elisabeth Robson
If you found the “Gang of Four” book on design patterns challenging, “Head First Design Patterns” offers a more approachable introduction to the subject. Using a visually rich format and a conversational style, this book makes design patterns more accessible to beginners.
Key features of this book include:
- Clear explanations of common design patterns
- Real-world examples and use cases for each pattern
- Visual aids and diagrams to reinforce concepts
- Exercises and puzzles to engage readers and promote active learning
This book is an excellent starting point for those new to design patterns or those who prefer a more visual learning style.
16. “The C Programming Language” by Brian Kernighan and Dennis Ritchie
Often referred to as “K&R” (after its authors), this book is the definitive guide to the C programming language. While C may not be as widely used for application development as it once was, understanding C can provide valuable insights into how computers work at a lower level.
Key aspects of this book include:
- A complete guide to the C language syntax and semantics
- Explanations of fundamental programming concepts
- Examples of well-written C code
- Discussion of the design philosophy behind C
Even if you don’t plan to write C code regularly, reading this book can deepen your understanding of programming languages in general.
17. “Grokking Algorithms” by Aditya Bhargava
For those who find traditional algorithm books intimidating, “Grokking Algorithms” offers a friendlier introduction to the subject. Using clear explanations, simple examples, and plenty of illustrations, Bhargava makes complex algorithmic concepts more accessible.
Topics covered in this book include:
- Basic algorithms like binary search and quicksort
- Graph algorithms
- Dynamic programming
- Machine learning algorithms
This book is particularly useful for visual learners or those looking for a gentler introduction to algorithms.
Conclusion
These 17 books cover a wide range of topics essential to becoming a well-rounded programmer. From the fundamentals of clean coding and algorithm design to more advanced topics like design patterns and enterprise architecture, these books offer invaluable insights that can help you at every stage of your programming career.
Remember, the goal isn’t necessarily to read all of these books cover-to-cover (although that would certainly be an impressive feat!). Instead, consider this list as a valuable resource that you can turn to as you progress in your programming journey. Pick the books that align with your current interests and needs, and don’t hesitate to revisit them as you gain more experience.
Happy reading, and happy coding!