Test Your Skills: Coding Challenges from Real Interviews
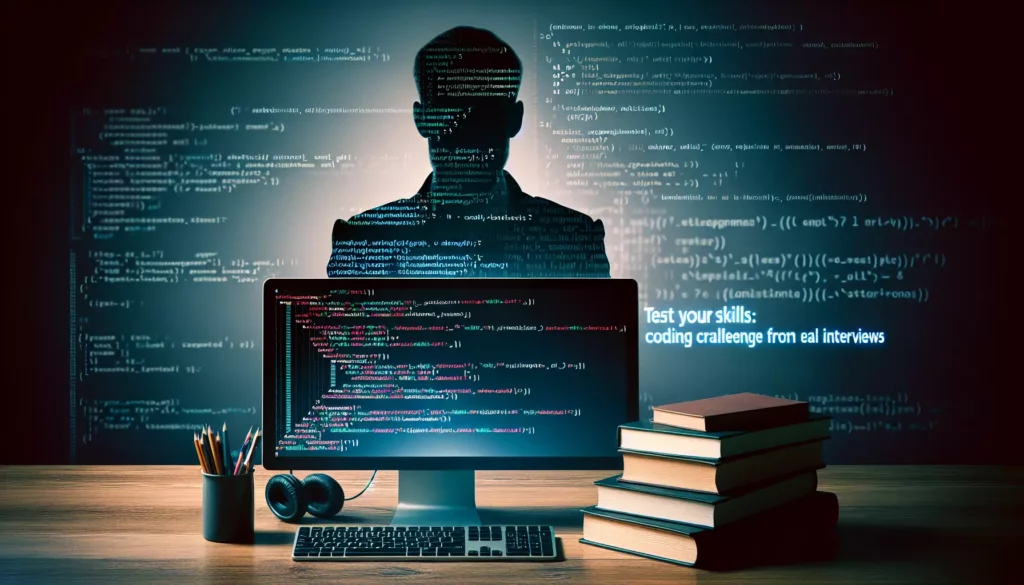
Are you preparing for a technical interview at a major tech company? Do you want to sharpen your coding skills and test your problem-solving abilities? Look no further! In this comprehensive guide, we’ll explore a collection of coding challenges that have been asked in real interviews at top tech companies. These challenges will not only help you prepare for your next interview but also enhance your algorithmic thinking and coding prowess.
Why Practice Coding Challenges?
Before we dive into the challenges, let’s understand why practicing coding problems is crucial for aspiring software developers:
- Interview Preparation: Many tech companies use coding challenges to assess candidates’ problem-solving skills and coding abilities.
- Skill Enhancement: Regular practice improves your algorithmic thinking and coding efficiency.
- Time Management: Solving problems under time constraints helps you perform better in real interview scenarios.
- Confidence Building: Successfully tackling various challenges boosts your confidence in your coding abilities.
Challenge 1: Two Sum
Let’s start with a classic problem that’s often asked in interviews:
Problem Statement:
Given an array of integers nums
and an integer target
, return indices of the two numbers in the array such that they add up to the target
. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Example:
Input: nums = [2,7,11,15], target = 9
Output: [0,1]
Explanation: Because nums[0] + nums[1] == 9, we return [0, 1].
Solution:
Here’s an efficient solution using a hash map:
class Solution {
public:
vector<int> twoSum(vector<int>& nums, int target) {
unordered_map<int, int> numMap;
for (int i = 0; i < nums.size(); i++) {
int complement = target - nums[i];
if (numMap.find(complement) != numMap.end()) {
return {numMap[complement], i};
}
numMap[nums[i]] = i;
}
return {}; // No solution found
}
};
This solution has a time complexity of O(n) and a space complexity of O(n), where n is the number of elements in the input array.
Challenge 2: Reverse a Linked List
Linked lists are a common data structure in coding interviews. Let’s tackle a popular problem involving linked lists.
Problem Statement:
Given the head of a singly linked list, reverse the list, and return the reversed list.
Example:
Input: head = [1,2,3,4,5]
Output: [5,4,3,2,1]
Solution:
Here’s an iterative solution to reverse a linked list:
class Solution {
public:
ListNode* reverseList(ListNode* head) {
ListNode* prev = nullptr;
ListNode* current = head;
while (current != nullptr) {
ListNode* nextTemp = current->next;
current->next = prev;
prev = current;
current = nextTemp;
}
return prev;
}
};
This solution has a time complexity of O(n) and a space complexity of O(1), where n is the number of nodes in the linked list.
Challenge 3: Valid Parentheses
String manipulation and stack-based problems are also common in coding interviews. Let’s look at a problem that combines both concepts.
Problem Statement:
Given a string s
containing just the characters ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘ and ‘]’, determine if the input string is valid. An input string is valid if:
- Open brackets must be closed by the same type of brackets.
- Open brackets must be closed in the correct order.
- Every close bracket has a corresponding open bracket of the same type.
Example:
Input: s = "()[]{}"
Output: true
Input: s = "([)]"
Output: false
Solution:
Here’s a solution using a stack:
class Solution {
public:
bool isValid(string s) {
stack<char> st;
for (char c : s) {
if (c == '(' || c == '{' || c == '[') {
st.push(c);
} else {
if (st.empty()) return false;
if (c == ')' && st.top() != '(') return false;
if (c == '}' && st.top() != '{') return false;
if (c == ']' && st.top() != '[') return false;
st.pop();
}
}
return st.empty();
}
};
This solution has a time complexity of O(n) and a space complexity of O(n), where n is the length of the input string.
Challenge 4: Merge Two Sorted Lists
Merging sorted data structures is a common operation in computer science. Let’s look at a problem that involves merging two sorted linked lists.
Problem Statement:
You are given the heads of two sorted linked lists list1
and list2
. Merge the two lists into one sorted list. The list should be made by splicing together the nodes of the first two lists. Return the head of the merged linked list.
Example:
Input: list1 = [1,2,4], list2 = [1,3,4]
Output: [1,1,2,3,4,4]
Solution:
Here’s a recursive solution to merge two sorted linked lists:
class Solution {
public:
ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) {
if (l1 == nullptr) return l2;
if (l2 == nullptr) return l1;
if (l1->val <= l2->val) {
l1->next = mergeTwoLists(l1->next, l2);
return l1;
} else {
l2->next = mergeTwoLists(l1, l2->next);
return l2;
}
}
};
This solution has a time complexity of O(n + m), where n and m are the lengths of the two input lists, and a space complexity of O(n + m) due to the recursive call stack.
Challenge 5: Maximum Subarray
Dynamic programming is a powerful technique used to solve optimization problems. Let’s look at a classic dynamic programming problem that’s often asked in interviews.
Problem Statement:
Given an integer array nums
, find the contiguous subarray (containing at least one number) which has the largest sum and return its sum.
Example:
Input: nums = [-2,1,-3,4,-1,2,1,-5,4]
Output: 6
Explanation: [4,-1,2,1] has the largest sum = 6.
Solution:
Here’s a solution using Kadane’s algorithm:
class Solution {
public:
int maxSubArray(vector<int>& nums) {
int maxSum = nums[0];
int currentSum = nums[0];
for (int i = 1; i < nums.size(); i++) {
currentSum = max(nums[i], currentSum + nums[i]);
maxSum = max(maxSum, currentSum);
}
return maxSum;
}
};
This solution has a time complexity of O(n) and a space complexity of O(1), where n is the number of elements in the input array.
Challenge 6: Binary Tree Level Order Traversal
Tree traversal problems are common in coding interviews, especially for roles that involve working with hierarchical data structures. Let’s look at a problem that involves traversing a binary tree in level order.
Problem Statement:
Given the root of a binary tree, return the level order traversal of its nodes’ values. (i.e., from left to right, level by level).
Example:
Input: root = [3,9,20,null,null,15,7]
Output: [[3],[9,20],[15,7]]
Solution:
Here’s a solution using a queue for breadth-first search:
class Solution {
public:
vector<vector<int>> levelOrder(TreeNode* root) {
vector<vector<int>> result;
if (root == nullptr) return result;
queue<TreeNode*> q;
q.push(root);
while (!q.empty()) {
int levelSize = q.size();
vector<int> currentLevel;
for (int i = 0; i < levelSize; i++) {
TreeNode* node = q.front();
q.pop();
currentLevel.push_back(node->val);
if (node->left) q.push(node->left);
if (node->right) q.push(node->right);
}
result.push_back(currentLevel);
}
return result;
}
};
This solution has a time complexity of O(n) and a space complexity of O(n), where n is the number of nodes in the binary tree.
Challenge 7: Longest Palindromic Substring
String manipulation problems are frequently asked in coding interviews. Let’s tackle a more challenging problem involving palindromes.
Problem Statement:
Given a string s
, return the longest palindromic substring in s
.
Example:
Input: s = "babad"
Output: "bab"
Explanation: "aba" is also a valid answer.
Solution:
Here’s an efficient solution using dynamic programming:
class Solution {
public:
string longestPalindrome(string s) {
int n = s.length();
if (n < 2) return s;
int start = 0, maxLength = 1;
vector<vector<bool>> dp(n, vector<bool>(n, false));
// All substrings of length 1 are palindromes
for (int i = 0; i < n; i++) {
dp[i][i] = true;
}
// Check for substrings of length 2
for (int i = 0; i < n - 1; i++) {
if (s[i] == s[i+1]) {
dp[i][i+1] = true;
start = i;
maxLength = 2;
}
}
// Check for substrings of length 3 or more
for (int len = 3; len <= n; len++) {
for (int i = 0; i < n - len + 1; i++) {
int j = i + len - 1;
if (s[i] == s[j] && dp[i+1][j-1]) {
dp[i][j] = true;
if (len > maxLength) {
start = i;
maxLength = len;
}
}
}
}
return s.substr(start, maxLength);
}
};
This solution has a time complexity of O(n^2) and a space complexity of O(n^2), where n is the length of the input string.
Challenge 8: Implement a Trie (Prefix Tree)
Advanced data structures like Tries are sometimes asked about in interviews, especially for roles involving search or autocomplete functionality. Let’s implement a Trie from scratch.
Problem Statement:
Implement a trie with insert
, search
, and startsWith
methods.
Example:
Trie trie = new Trie();
trie.insert("apple");
trie.search("apple"); // returns true
trie.search("app"); // returns false
trie.startsWith("app"); // returns true
trie.insert("app");
trie.search("app"); // returns true
Solution:
Here’s an implementation of a Trie:
class TrieNode {
public:
TrieNode* children[26];
bool isEndOfWord;
TrieNode() {
for (int i = 0; i < 26; i++) {
children[i] = nullptr;
}
isEndOfWord = false;
}
};
class Trie {
private:
TrieNode* root;
public:
Trie() {
root = new TrieNode();
}
void insert(string word) {
TrieNode* current = root;
for (char c : word) {
int index = c - 'a';
if (current->children[index] == nullptr) {
current->children[index] = new TrieNode();
}
current = current->children[index];
}
current->isEndOfWord = true;
}
bool search(string word) {
TrieNode* node = searchPrefix(word);
return (node != nullptr && node->isEndOfWord);
}
bool startsWith(string prefix) {
return searchPrefix(prefix) != nullptr;
}
private:
TrieNode* searchPrefix(string prefix) {
TrieNode* current = root;
for (char c : prefix) {
int index = c - 'a';
if (current->children[index] == nullptr) {
return nullptr;
}
current = current->children[index];
}
return current;
}
};
The time complexity for insert, search, and startsWith operations is O(m), where m is the length of the key. The space complexity of the Trie is O(26 * n * m), where n is the number of keys and m is the average length of the keys.
Conclusion
These coding challenges represent just a small sample of the types of problems you might encounter in technical interviews at top tech companies. By practicing these and similar problems, you’ll develop stronger problem-solving skills, improve your coding efficiency, and gain confidence in your abilities.
Remember, the key to success in coding interviews is not just about memorizing solutions, but understanding the underlying concepts and being able to apply them to new problems. As you practice, focus on:
- Understanding the problem thoroughly before coding
- Considering edge cases and potential optimizations
- Communicating your thought process clearly
- Writing clean, readable code
- Testing your solution with various inputs
Keep practicing, stay curious, and never stop learning. With dedication and consistent effort, you’ll be well-prepared to tackle any coding challenge that comes your way in your next technical interview. Good luck!