Techniques for Optimizing Nested Loop Solutions
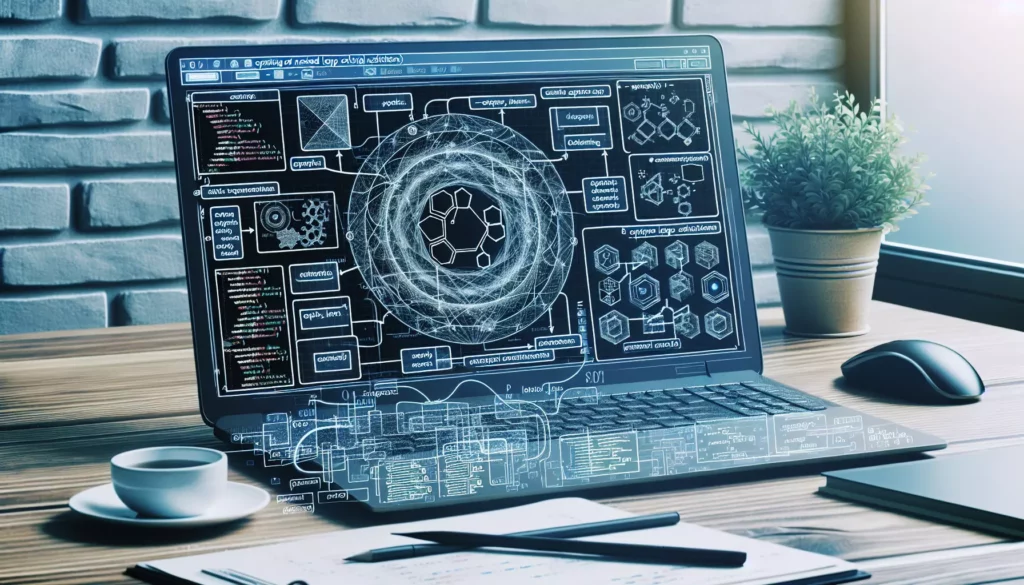
Nested loops are a common programming construct used to solve complex problems that require iterating over multiple dimensions of data. While powerful, nested loops can quickly become a performance bottleneck if not implemented efficiently. In this comprehensive guide, we’ll explore various techniques for optimizing nested loop solutions, helping you write more efficient and scalable code.
Understanding Nested Loops
Before diving into optimization techniques, let’s briefly review what nested loops are and why they’re important in programming.
Nested loops occur when one loop is placed inside another. This structure allows programmers to perform repetitive tasks on multi-dimensional data structures or to solve problems that require multiple levels of iteration. Here’s a simple example of a nested loop in Python:
for i in range(5):
for j in range(5):
print(f"({i}, {j})")
While nested loops are powerful, they can also be computationally expensive, especially when dealing with large datasets or complex algorithms. The time complexity of nested loops is often O(n^m), where n is the size of the input and m is the number of nested levels. This exponential growth in complexity makes optimization crucial for maintaining performance as the input size increases.
Optimization Technique 1: Loop Invariant Code Motion
Loop invariant code motion is a technique that involves moving computations that don’t change within a loop to outside the loop. This reduces the number of redundant calculations performed in each iteration.
Consider this example:
for i in range(n):
for j in range(n):
result = expensive_function()
# Use result in some calculation
If expensive_function()
doesn’t depend on i
or j
, we can optimize this by moving it outside the loops:
result = expensive_function()
for i in range(n):
for j in range(n):
# Use result in some calculation
This simple change can significantly reduce the number of function calls, especially for large values of n
.
Optimization Technique 2: Loop Unrolling
Loop unrolling is a technique where we perform multiple iterations of a loop within a single iteration, reducing the overall number of iterations. This can improve performance by reducing loop overhead and allowing for better instruction-level parallelism.
Here’s an example of loop unrolling:
# Before unrolling
for i in range(0, n, 1):
# Perform operation
# After unrolling
for i in range(0, n, 4):
# Perform operation for i
# Perform operation for i+1
# Perform operation for i+2
# Perform operation for i+3
While this technique can be effective, it’s important to note that modern compilers often perform loop unrolling automatically. Manual unrolling should be done judiciously and with careful benchmarking to ensure it actually improves performance.
Optimization Technique 3: Early Termination
Early termination involves breaking out of loops as soon as a certain condition is met, rather than continuing to iterate unnecessarily. This can be particularly effective in nested loops where finding a single result is sufficient.
Consider this example of searching for a value in a 2D array:
def find_value(matrix, target):
for i in range(len(matrix)):
for j in range(len(matrix[i])):
if matrix[i][j] == target:
return True
return False
In this case, we return True
as soon as we find the target value, avoiding unnecessary iterations through the rest of the matrix.
Optimization Technique 4: Loop Fusion
Loop fusion involves combining multiple loops that iterate over the same range into a single loop. This can reduce loop overhead and improve cache utilization.
Here’s an example:
# Before fusion
for i in range(n):
# Perform operation A
for i in range(n):
# Perform operation B
# After fusion
for i in range(n):
# Perform operation A
# Perform operation B
Loop fusion can be particularly effective when the operations in each loop are independent of each other and can be safely combined.
Optimization Technique 5: Use of Appropriate Data Structures
Choosing the right data structure can significantly impact the performance of nested loops. For example, using a hash table for lookups instead of nested loops can reduce time complexity from O(n^2) to O(n) in many cases.
Consider this example of finding common elements in two lists:
# Inefficient nested loop approach
def find_common_elements(list1, list2):
common = []
for item1 in list1:
for item2 in list2:
if item1 == item2:
common.append(item1)
return common
# Optimized approach using a set
def find_common_elements_optimized(list1, list2):
set1 = set(list1)
return [item for item in list2 if item in set1]
The optimized version using a set has a time complexity of O(n), which is much more efficient for large lists.
Optimization Technique 6: Vectorization
Vectorization involves replacing loop-based operations with vector operations that can be executed more efficiently by modern CPUs. Many programming languages and libraries provide vectorized operations that can significantly speed up computations on large datasets.
For example, in Python, using NumPy’s vectorized operations instead of nested loops can lead to substantial performance improvements:
import numpy as np
# Nested loop approach
def matrix_multiply(A, B):
n = len(A)
C = [[0 for _ in range(n)] for _ in range(n)]
for i in range(n):
for j in range(n):
for k in range(n):
C[i][j] += A[i][k] * B[k][j]
return C
# Vectorized approach
def matrix_multiply_vectorized(A, B):
return np.dot(A, B)
The vectorized approach is not only more concise but also significantly faster, especially for large matrices.
Optimization Technique 7: Caching and Memoization
Caching and memoization involve storing the results of expensive function calls and returning the cached result when the same inputs occur again. This can be particularly effective in recursive algorithms or when dealing with repeated computations in nested loops.
Here’s an example using memoization to optimize the Fibonacci sequence calculation:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
This memoized version of the Fibonacci function avoids redundant calculations, significantly improving performance for large values of n.
Optimization Technique 8: Parallelization
For computationally intensive nested loops, parallelization can be a powerful optimization technique. By distributing the work across multiple cores or even multiple machines, we can significantly reduce execution time.
Here’s a simple example using Python’s multiprocessing
module:
from multiprocessing import Pool
def process_chunk(chunk):
result = []
for item in chunk:
# Perform some computation
result.append(processed_item)
return result
def parallel_processing(data, num_processes=4):
chunk_size = len(data) // num_processes
chunks = [data[i:i+chunk_size] for i in range(0, len(data), chunk_size)]
with Pool(num_processes) as pool:
results = pool.map(process_chunk, chunks)
return [item for sublist in results for item in sublist]
This approach can be particularly effective for embarrassingly parallel problems where each iteration of the loop is independent of the others.
Optimization Technique 9: Algorithm Redesign
Sometimes, the most effective optimization is to rethink the algorithm entirely. A different approach might eliminate the need for nested loops or reduce their complexity.
For example, consider the problem of finding the maximum sum subarray. A naive nested loop solution would have O(n^2) complexity:
def max_subarray_sum_naive(arr):
n = len(arr)
max_sum = float('-inf')
for i in range(n):
current_sum = 0
for j in range(i, n):
current_sum += arr[j]
max_sum = max(max_sum, current_sum)
return max_sum
However, we can solve this problem in O(n) time using Kadane’s algorithm:
def max_subarray_sum_kadane(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
This dramatic improvement in time complexity showcases the power of algorithm redesign as an optimization technique.
Optimization Technique 10: Compiler Optimizations
Modern compilers are incredibly sophisticated and can perform various optimizations automatically. Understanding and leveraging these compiler optimizations can lead to significant performance improvements without changing your code.
Some common compiler optimizations include:
- Loop unrolling
- Function inlining
- Constant folding and propagation
- Dead code elimination
- Instruction reordering
To take advantage of these optimizations, make sure you’re using the appropriate compiler flags. For example, in GCC, you might use flags like -O2
or -O3
for aggressive optimizations:
gcc -O3 -o myprogram myprogram.c
However, it’s important to note that while compiler optimizations can significantly improve performance, they can sometimes lead to unexpected behavior, especially in programs with undefined behavior or reliance on specific execution order.
Conclusion
Optimizing nested loop solutions is a crucial skill for writing efficient and scalable code. The techniques we’ve explored in this article – from loop invariant code motion and loop unrolling to algorithm redesign and leveraging compiler optimizations – provide a comprehensive toolkit for tackling performance bottlenecks in nested loops.
Remember that optimization is often a trade-off between performance, code readability, and development time. Always profile your code to identify the actual bottlenecks, and apply these optimization techniques judiciously where they’ll have the most impact.
As you continue to develop your programming skills, practice applying these techniques to real-world problems. Over time, you’ll develop an intuition for when and how to optimize your code effectively, making you a more proficient and valuable developer.
Happy coding, and may your loops always run efficiently!