Tackling Multiple Problems: How to Manage Time and Focus When Faced with Multiple Interview Questions
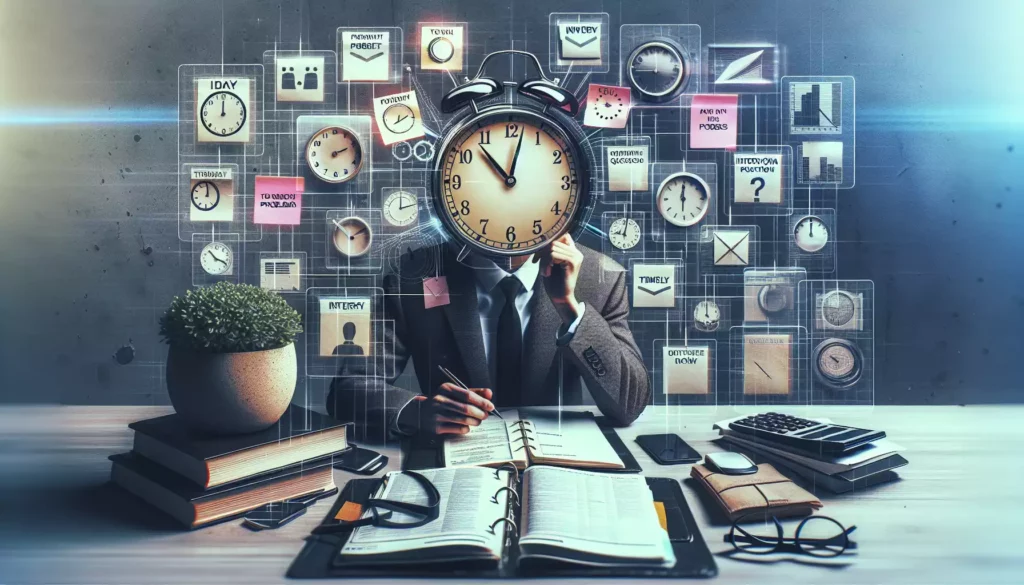
Technical interviews can be challenging, especially when you’re presented with multiple problems to solve within a limited timeframe. The ability to manage your time effectively and maintain focus is crucial for success. In this comprehensive guide, we’ll explore strategies to help you navigate through multiple interview questions, prioritize effectively, and showcase your problem-solving skills to potential employers.
Understanding the Challenge of Multiple Interview Questions
Before diving into strategies, it’s important to understand why interviewers might present multiple problems during a single interview session:
- To assess your ability to handle pressure and manage time
- To evaluate your problem-solving skills across different domains
- To gauge your prioritization and decision-making abilities
- To simulate real-world scenarios where multiple tasks need attention
Recognizing these objectives can help you approach the challenge with the right mindset and demonstrate the skills that interviewers are looking for.
Strategies for Managing Time and Focus
1. Quick Assessment and Prioritization
When faced with multiple problems, your first step should be a rapid assessment of all questions. This initial overview allows you to:
- Gauge the complexity of each problem
- Identify any immediate connections or similarities between questions
- Estimate the time required for each problem
- Prioritize based on difficulty, familiarity, and potential impact
Spend about 2-3 minutes on this initial assessment. It’s a crucial step that sets the foundation for your entire approach.
2. Start with the Low-Hanging Fruit
After your initial assessment, consider starting with the problem that seems most straightforward or familiar to you. This approach has several benefits:
- Builds confidence and momentum
- Ensures you have at least one solid solution to present
- Allows your subconscious to process the more difficult problems
However, be cautious not to spend too much time perfecting this initial solution. Remember, your goal is to address all problems to some degree.
3. Time Boxing
Time boxing is a crucial technique for managing multiple problems. Here’s how to implement it:
- Allocate a specific amount of time for each problem based on your initial assessment
- Set a timer for each problem and stick to it rigorously
- When the time is up, move on to the next problem, even if you haven’t fully solved the current one
- Reserve some time at the end for review and refinement
For example, if you have 60 minutes and three problems, you might allocate:
- 20 minutes for Problem A (most familiar)
- 25 minutes for Problem B (most challenging)
- 15 minutes for Problem C (moderate difficulty)
This leaves you with 5 minutes for a final review and any necessary adjustments.
4. Use the “Outline First” Approach
For each problem, start by creating a high-level outline of your solution. This approach helps in several ways:
- Provides a roadmap for your implementation
- Allows you to quickly capture your thought process
- Makes it easier to explain your approach, even if you don’t complete the full implementation
Here’s an example of how you might outline a solution:
// Problem: Find the longest palindromic substring
// Outline:
// 1. Define a helper function to expand around center
// 2. Iterate through each character in the string
// 3. For each character, consider it as a center and expand
// 4. Keep track of the longest palindrome found
// 5. Return the longest palindrome
This outline gives you a clear structure to follow and helps you organize your thoughts quickly.
5. Implement a Minimum Viable Solution First
When dealing with multiple problems, it’s crucial to have at least a basic solution for each before diving deep into optimizations. Follow these steps:
- Implement the simplest solution that works, even if it’s not the most efficient
- Ensure your solution handles the basic test cases
- Briefly comment on potential optimizations or improvements
- Move on to the next problem
This approach ensures you have something to show for each problem, which is better than having one perfect solution and nothing for the others.
6. Communicate Your Thought Process
Throughout the interview, maintain clear communication with your interviewer. This is especially important when managing multiple problems:
- Explain your prioritization decisions
- Verbalize your thought process as you work through each problem
- Ask clarifying questions when needed
- Provide updates on your progress and any challenges you’re facing
Good communication can compensate for incomplete solutions and demonstrates your problem-solving approach.
7. Use Pseudocode for Complex Problems
For more challenging problems, consider using pseudocode to quickly capture your solution strategy. This is particularly useful when you’re short on time:
// Problem: Implement a LRU Cache
// Pseudocode:
// 1. Create a doubly linked list to store key-value pairs
// 2. Create a hash map to store keys and their corresponding nodes
// 3. Implement get():
// - If key exists, move node to front of list and return value
// - If key doesn't exist, return -1
// 4. Implement put():
// - If key exists, update value and move node to front
// - If key doesn't exist:
// - If cache is full, remove least recently used item
// - Add new key-value pair to front of list and hash map
// 5. Ensure all operations are O(1) time complexity
Pseudocode allows you to demonstrate your understanding of the problem and your approach to solving it, even if you don’t have time for a full implementation.
Prioritizing Problems Based on Different Factors
When faced with multiple problems, effective prioritization is key. Consider the following factors:
1. Difficulty Level
Assess the complexity of each problem and consider:
- Starting with easier problems to build confidence
- Tackling the most difficult problem when you’re most alert and focused
- Balancing your time between easy and hard problems
2. Time Constraints
Factor in the time available and the estimated time for each problem:
- Prioritize problems that you can reasonably complete within the given timeframe
- Allocate more time to problems that carry more weight or complexity
- Reserve time for reviewing and refining your solutions
3. Familiarity with Topics
Consider your strengths and weaknesses:
- Start with problems in areas where you have strong knowledge
- Use familiar problems to showcase your best skills
- Don’t avoid unfamiliar topics entirely, as they may carry significant weight
4. Problem Interdependencies
Look for connections between problems:
- Identify if solving one problem might provide insights for another
- Consider the logical order of problems if they build upon each other
- Group similar problems together to maintain focus on a particular concept
5. Potential Impact
Evaluate the importance of each problem:
- Prioritize problems that align closely with the job requirements
- Focus on problems that demonstrate skills the company values most
- Consider which problems might lead to more interesting discussions
Practical Examples: Handling Multiple Interview Questions
Let’s walk through a practical example of how to apply these strategies in a real interview scenario.
Scenario: 60-Minute Interview with Three Problems
Imagine you’re given the following three problems in a 60-minute technical interview:
- Implement a function to reverse a linked list
- Design a system to handle millions of concurrent web socket connections
- Find the kth largest element in an unsorted array
Step 1: Quick Assessment (3 minutes)
- Problem 1: Easy, familiar data structure problem
- Problem 2: Complex system design question, requires careful consideration
- Problem 3: Moderate difficulty, involves sorting or selection algorithms
Step 2: Prioritization and Time Allocation
- Problem 1: 15 minutes (start with the familiar problem)
- Problem 2: 25 minutes (allocate more time for the complex problem)
- Problem 3: 15 minutes
- Final review: 5 minutes
Step 3: Tackling the Problems
Problem 1: Reverse a Linked List (15 minutes)
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverseLinkedList(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
# Test the function
# Create a linked list: 1 -> 2 -> 3 -> 4 -> 5
head = ListNode(1)
head.next = ListNode(2)
head.next.next = ListNode(3)
head.next.next.next = ListNode(4)
head.next.next.next.next = ListNode(5)
# Reverse the linked list
reversed_head = reverseLinkedList(head)
# Print the reversed list
current = reversed_head
while current:
print(current.val, end=" ")
current = current.next
# Output: 5 4 3 2 1
Problem 2: Design a System for Concurrent Web Socket Connections (25 minutes)
For this system design question, we’ll create a high-level outline:
- Load Balancing:
- Use multiple load balancers to distribute incoming connections
- Implement round-robin or least-connections algorithm
- Web Socket Servers:
- Deploy multiple servers to handle connections
- Use asynchronous I/O to manage many connections per server
- Implement connection pooling to reuse resources
- Message Queue:
- Use a distributed message queue (e.g., Kafka, RabbitMQ) for communication between servers
- Implement pub/sub pattern for efficient message distribution
- Database:
- Use a distributed NoSQL database for scalability
- Implement sharding for better data distribution
- Caching:
- Implement a distributed cache (e.g., Redis) to reduce database load
- Cache frequently accessed data and session information
- Monitoring and Scaling:
- Implement robust monitoring system
- Use auto-scaling to handle traffic spikes
Problem 3: Find the kth Largest Element (15 minutes)
import heapq
def findKthLargest(nums, k):
# Use a min heap to keep track of the k largest elements
heap = []
for num in nums:
if len(heap) < k:
heapq.heappush(heap, num)
elif num > heap[0]:
heapq.heapreplace(heap, num)
# The root of the heap will be the kth largest element
return heap[0]
# Test the function
nums = [3, 2, 1, 5, 6, 4]
k = 2
result = findKthLargest(nums, k)
print(f"The {k}th largest element is: {result}")
# Output: The 2th largest element is: 5
# Note: This solution has O(n log k) time complexity and O(k) space complexity.
# For follow-up, mention that there's an O(n) average time solution using QuickSelect algorithm.
Step 4: Final Review (5 minutes)
- Quickly review each solution for correctness
- Add any additional comments or optimizations
- Prepare to discuss trade-offs and alternative approaches
Common Pitfalls to Avoid
When managing multiple interview questions, be aware of these common mistakes:
- Spending too much time on one problem: It’s easy to get caught up in perfecting one solution, but remember to allocate time for all questions.
- Neglecting communication: Don’t fall into silent coding. Keep explaining your thought process throughout.
- Ignoring time constraints: Always keep an eye on the clock and stick to your time allocations.
- Failing to prioritize effectively: Don’t just tackle problems in the order they’re presented. Assess and prioritize based on the factors we discussed.
- Giving up on difficult problems: Even if you can’t fully solve a problem, always try to provide some insights or partial solutions.
- Not asking clarifying questions: If you’re unsure about a problem’s requirements, don’t hesitate to ask for clarification.
- Overlooking edge cases: In your rush to solve multiple problems, don’t forget to consider and handle edge cases for each solution.
Conclusion
Handling multiple interview questions is a skill that can be developed with practice. By implementing the strategies outlined in this guide, you can approach these challenging situations with confidence and demonstrate your problem-solving abilities effectively.
Remember, the key aspects are:
- Quick assessment and prioritization
- Effective time management through techniques like time boxing
- Clear communication of your thought process
- Balancing thoroughness with breadth of coverage
- Adapting your approach based on problem difficulty, time constraints, and your familiarity with the topics
As you prepare for interviews, practice these techniques with sample problem sets. Simulate time constraints and work on explaining your thought process out loud. With time and effort, you’ll become more adept at navigating the challenges of multi-problem interviews, setting yourself up for success in your technical interviews and future career in software development.