Tackling Dynamic Programming Problems in Interviews
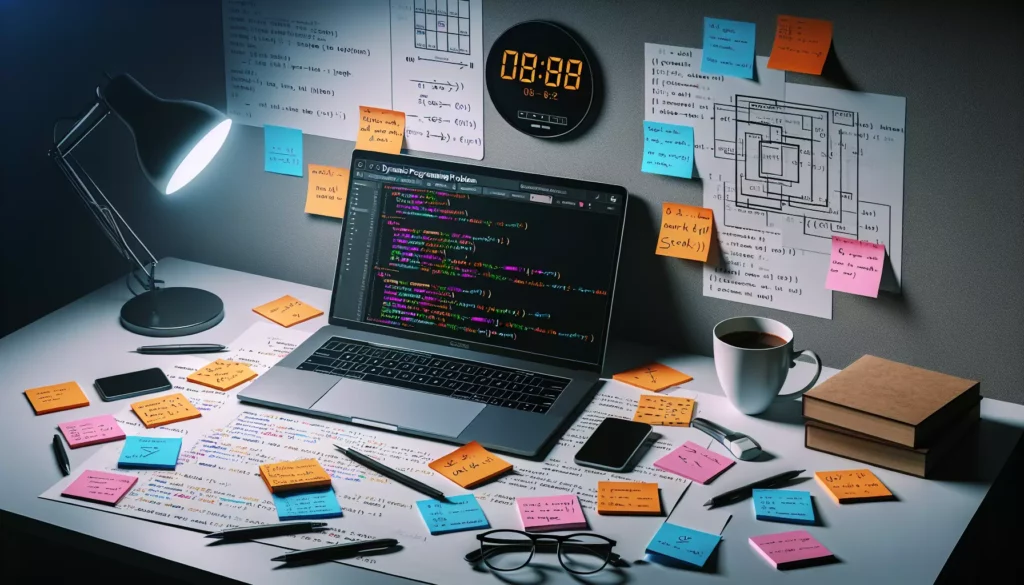
Dynamic programming (DP) is a powerful algorithmic technique that can solve complex problems by breaking them down into simpler subproblems. It’s a favorite topic among interviewers at top tech companies, including FAANG (Facebook, Amazon, Apple, Netflix, and Google), because it tests a candidate’s ability to think algorithmically and optimize solutions. In this comprehensive guide, we’ll explore how to approach and solve dynamic programming problems in coding interviews, providing you with the tools and strategies you need to excel.
Understanding Dynamic Programming
Before diving into specific problem-solving strategies, it’s crucial to understand what dynamic programming is and why it’s so important in computer science and coding interviews.
What is Dynamic Programming?
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It is applicable when the problem has the following properties:
- Overlapping Subproblems: The problem can be broken down into subproblems which are reused several times.
- Optimal Substructure: The optimal solution to the problem can be constructed from optimal solutions of its subproblems.
The key idea behind DP is to store the results of subproblems so that we don’t have to recompute them when needed later. This technique is called memoization.
Why is Dynamic Programming Important in Interviews?
Dynamic programming is a favorite topic among interviewers for several reasons:
- It tests a candidate’s ability to recognize patterns and optimize solutions.
- It demonstrates problem-solving skills and algorithmic thinking.
- Many real-world problems can be solved efficiently using DP.
- It’s a challenging topic that separates strong candidates from average ones.
Common Types of Dynamic Programming Problems
Before we delve into solving strategies, let’s look at some common types of DP problems you might encounter in interviews:
1. Fibonacci Sequence
The Fibonacci sequence is a classic example used to introduce DP. Each number is the sum of the two preceding ones.
2. Longest Common Subsequence (LCS)
Find the longest subsequence present in given two sequences in the same order.
3. Knapsack Problem
Given a set of items, each with a weight and a value, determine the number of each item to include in a collection so that the total weight is less than or equal to a given limit and the total value is as large as possible.
4. Longest Increasing Subsequence (LIS)
Find a subsequence of a given sequence in which the subsequence’s elements are in sorted order, lowest to highest, and in which the subsequence is as long as possible.
5. Edit Distance
Find the minimum number of operations required to convert one string into another.
Strategies for Solving Dynamic Programming Problems
Now that we’ve covered the basics and common types of DP problems, let’s explore strategies for tackling these problems in interviews.
1. Identify if the Problem is a DP Problem
The first step is to recognize if a problem can be solved using dynamic programming. Look for these characteristics:
- The problem asks for an optimal solution (maximum or minimum)
- There are multiple ways to arrive at a solution
- The problem can be broken down into smaller, similar subproblems
- There’s a recursive relation between subproblems
2. Define the Subproblem
Once you’ve identified that DP is applicable, define the subproblem. This is crucial as it forms the basis of your recursive relation. Ask yourself:
- What’s the smallest instance of the problem?
- How can larger instances be expressed in terms of smaller ones?
3. Write the Recurrence Relation
The recurrence relation is the heart of your DP solution. It expresses how the solution to a larger problem can be constructed from solutions to smaller subproblems. This is often the most challenging step and requires practice to master.
4. Identify the Base Cases
Base cases are the simplest instances of the problem that can be solved directly. They serve as the foundation for building up to more complex cases.
5. Decide on a DP Approach: Top-Down or Bottom-Up
There are two main approaches to implementing a DP solution:
- Top-Down (Memoization): Start with the original problem and recursively break it down. Use memoization to store results of subproblems.
- Bottom-Up (Tabulation): Start from the base cases and iteratively build up to the solution of the original problem.
6. Implement the Solution
Once you’ve decided on an approach, implement your solution. Be sure to handle edge cases and optimize for space and time complexity where possible.
7. Analyze Time and Space Complexity
After implementing your solution, analyze its time and space complexity. This is an important step that interviewers often ask about.
Example: Solving the Fibonacci Sequence Problem
Let’s apply these strategies to solve the Fibonacci sequence problem, a classic DP problem.
Problem Statement:
Write a function to calculate the nth Fibonacci number. The Fibonacci sequence is defined as follows:
F(0) = 0
F(1) = 1
F(n) = F(n-1) + F(n-2) for n > 1
Step 1: Identify if it’s a DP Problem
Yes, it’s a DP problem because:
- It has overlapping subproblems (we calculate the same Fibonacci numbers multiple times)
- It has optimal substructure (the nth Fibonacci number can be calculated from the (n-1)th and (n-2)th numbers)
Step 2: Define the Subproblem
The subproblem is to calculate F(k) for any k ≤ n.
Step 3: Write the Recurrence Relation
The recurrence relation is given in the problem statement:
F(n) = F(n-1) + F(n-2) for n > 1
Step 4: Identify the Base Cases
The base cases are also given:
F(0) = 0
F(1) = 1
Step 5: Decide on a DP Approach
Let’s implement both top-down and bottom-up approaches.
Step 6: Implement the Solution
Top-Down (Memoization) Approach:
def fibonacci_top_down(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_top_down(n-1, memo) + fibonacci_top_down(n-2, memo)
return memo[n]
Bottom-Up (Tabulation) Approach:
def fibonacci_bottom_up(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
Step 7: Analyze Time and Space Complexity
For both approaches:
- Time Complexity: O(n)
- Space Complexity: O(n)
The bottom-up approach can be further optimized for space:
def fibonacci_optimized(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
This optimized version has:
- Time Complexity: O(n)
- Space Complexity: O(1)
Common Pitfalls and How to Avoid Them
When solving DP problems in interviews, be aware of these common pitfalls:
1. Overlooking the DP Approach
Pitfall: Sometimes, candidates fail to recognize that a problem can be solved using DP and instead opt for a less efficient brute-force approach.
How to Avoid: Always consider if the problem exhibits overlapping subproblems and optimal substructure. If it does, explore a DP solution.
2. Incorrect Recurrence Relation
Pitfall: Formulating an incorrect recurrence relation can lead to wrong solutions.
How to Avoid: Take time to think through the problem carefully. Test your recurrence relation with small examples to verify its correctness.
3. Forgetting Base Cases
Pitfall: Overlooking or incorrectly defining base cases can cause errors in your solution.
How to Avoid: Always clearly define and implement the base cases before moving on to the recursive cases.
4. Inefficient Memoization
Pitfall: In top-down approaches, inefficient memoization can lead to unnecessary recalculations.
How to Avoid: Ensure you’re storing and retrieving memoized results correctly. Use appropriate data structures (like dictionaries in Python) for efficient lookup.
5. Unnecessary Space Usage
Pitfall: Some DP solutions use more space than necessary, which can be a concern in interviews.
How to Avoid: After implementing a working solution, consider if you can optimize space usage. Often, you can reduce space complexity from O(n) to O(1) by only keeping track of the last few states.
Advanced DP Techniques
As you become more comfortable with basic DP problems, you may encounter more advanced techniques in interviews. Here are a few to be aware of:
1. State Compression
In some DP problems, the state space can be very large. State compression involves finding ways to represent the state more efficiently, often using bit manipulation techniques.
2. Digit DP
Digit DP is a technique used to solve problems involving digit properties of numbers in a given range.
3. DP on Trees
This involves applying DP techniques to problems involving tree data structures, often using techniques like rerooting or tree diameter computation.
4. DP with Bitmasks
This technique uses bitmasks to represent sets efficiently in DP solutions, often used in problems involving subsets or permutations.
5. DP on Graphs
Applying DP to graph problems, such as shortest path algorithms like Floyd-Warshall or solving the Traveling Salesman Problem.
Practice Makes Perfect
The key to mastering dynamic programming is practice. Here are some tips to improve your DP skills:
- Solve Many Problems: Start with easy problems and gradually move to more difficult ones. Websites like LeetCode, HackerRank, and AlgoExpert offer many DP problems.
- Understand Each Step: For each problem you solve, make sure you understand why DP is applicable and how the solution works.
- Implement Both Approaches: Try implementing both top-down and bottom-up approaches for the same problem to understand their trade-offs.
- Optimize Your Solutions: After solving a problem, think about how you can optimize it further in terms of time and space complexity.
- Explain Your Thought Process: Practice explaining your approach out loud, as you would in an interview.
Conclusion
Dynamic programming is a powerful technique that can solve a wide range of complex problems efficiently. By breaking down problems into smaller subproblems and storing their solutions, DP allows us to avoid redundant computations and optimize our algorithms.
In coding interviews, especially for top tech companies like FAANG, mastering dynamic programming can give you a significant advantage. It demonstrates your ability to think algorithmically, recognize patterns, and optimize solutions – all highly valued skills in the tech industry.
Remember, the key to success with DP problems is practice and a systematic approach. Start by identifying if a problem is suitable for DP, define the subproblems and recurrence relation, choose between top-down and bottom-up approaches, and always analyze the time and space complexity of your solution.
As you continue to practice and refine your skills, you’ll find that dynamic programming becomes an invaluable tool in your problem-solving toolkit, not just for interviews, but for tackling complex algorithmic challenges in your professional career as well.