Synchronous vs Asynchronous Programming: Understanding the Key Differences
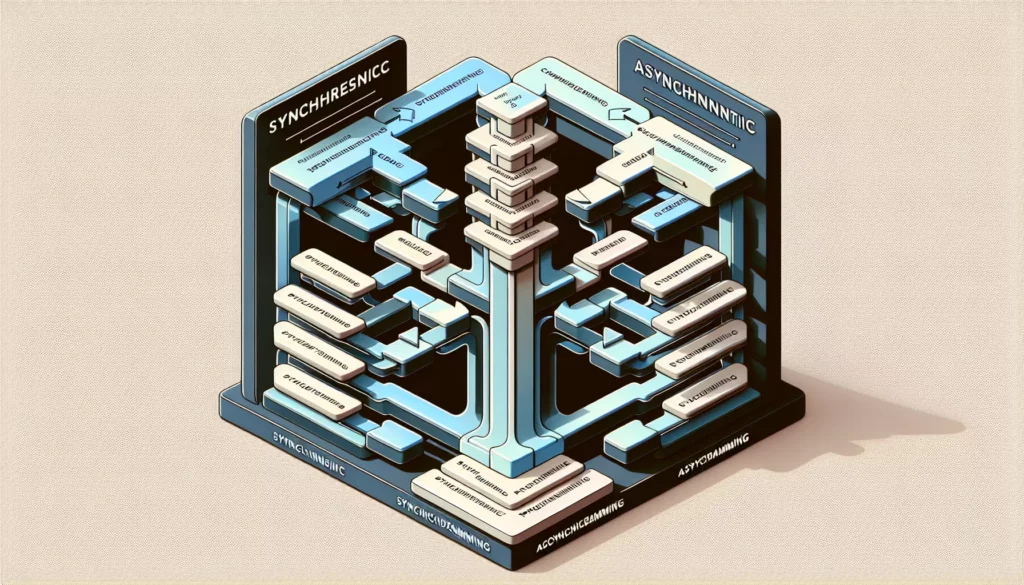
In the world of software development, understanding the difference between synchronous and asynchronous programming is crucial for creating efficient and responsive applications. Whether you’re a beginner coder or preparing for technical interviews at major tech companies, grasping these concepts will significantly enhance your programming skills. In this comprehensive guide, we’ll dive deep into the distinctions between synchronous and asynchronous programming, explore their use cases, and provide practical examples to solidify your understanding.
Table of Contents
- What is Synchronous Programming?
- What is Asynchronous Programming?
- Key Differences Between Synchronous and Asynchronous Programming
- Pros and Cons of Synchronous and Asynchronous Programming
- Use Cases for Synchronous and Asynchronous Programming
- Implementation Examples
- Best Practices for Choosing Between Synchronous and Asynchronous Programming
- Common Challenges and Solutions in Asynchronous Programming
- Future Trends in Synchronous and Asynchronous Programming
- Conclusion
1. What is Synchronous Programming?
Synchronous programming is a traditional programming paradigm where tasks are executed sequentially, one after another. In this model, when a program encounters a time-consuming operation, it waits for that operation to complete before moving on to the next task. This approach is straightforward and easy to understand, as it follows a linear flow of execution.
Characteristics of Synchronous Programming:
- Sequential execution of tasks
- Blocking nature: Each task must complete before the next one starts
- Predictable order of execution
- Easier to reason about and debug
Here’s a simple example of synchronous programming in Python:
def task1():
print("Task 1 started")
# Simulating a time-consuming operation
time.sleep(2)
print("Task 1 completed")
def task2():
print("Task 2 started")
# Simulating a time-consuming operation
time.sleep(1)
print("Task 2 completed")
# Synchronous execution
task1()
task2()
In this example, task2() will only start after task1() has completed, regardless of how long task1() takes to execute.
2. What is Asynchronous Programming?
Asynchronous programming is a programming paradigm that allows multiple tasks to be executed concurrently without blocking the main program flow. In this model, when a time-consuming operation is encountered, the program can continue executing other tasks while waiting for the operation to complete. This approach is particularly useful for I/O-bound operations and can significantly improve the overall performance and responsiveness of applications.
Characteristics of Asynchronous Programming:
- Non-blocking execution of tasks
- Concurrent handling of multiple operations
- Improved performance for I/O-bound tasks
- Event-driven architecture
- Callbacks, Promises, or async/await syntax for handling asynchronous operations
Here’s a simple example of asynchronous programming in JavaScript using Promises:
function task1() {
return new Promise((resolve) => {
console.log("Task 1 started");
// Simulating a time-consuming operation
setTimeout(() => {
console.log("Task 1 completed");
resolve();
}, 2000);
});
}
function task2() {
return new Promise((resolve) => {
console.log("Task 2 started");
// Simulating a time-consuming operation
setTimeout(() => {
console.log("Task 2 completed");
resolve();
}, 1000);
});
}
// Asynchronous execution
Promise.all([task1(), task2()]).then(() => {
console.log("All tasks completed");
});
In this asynchronous example, task1() and task2() are executed concurrently, and the program doesn’t wait for one task to complete before starting the other.
3. Key Differences Between Synchronous and Asynchronous Programming
Understanding the key differences between synchronous and asynchronous programming is essential for choosing the right approach for your specific use case. Let’s explore the main distinctions:
1. Execution Flow
- Synchronous: Tasks are executed sequentially, one after another.
- Asynchronous: Tasks can be executed concurrently, allowing for parallel processing.
2. Blocking vs. Non-blocking
- Synchronous: Blocking operations halt the execution of the entire program until they complete.
- Asynchronous: Non-blocking operations allow the program to continue executing other tasks while waiting for time-consuming operations to finish.
3. Performance
- Synchronous: May lead to performance bottlenecks, especially for I/O-bound operations.
- Asynchronous: Can significantly improve performance by utilizing system resources more efficiently, particularly for I/O-bound tasks.
4. Complexity
- Synchronous: Generally simpler to understand and implement, with a straightforward flow of execution.
- Asynchronous: Can be more complex to reason about and debug, often requiring additional constructs like callbacks, promises, or async/await syntax.
5. Resource Utilization
- Synchronous: May underutilize system resources, as the program waits for each task to complete before moving on.
- Asynchronous: Allows for better utilization of system resources by performing multiple tasks concurrently.
6. Error Handling
- Synchronous: Straightforward error handling using traditional try-catch blocks.
- Asynchronous: May require special error handling mechanisms, such as error callbacks or promise rejection handling.
7. Scalability
- Synchronous: Limited scalability, especially for applications dealing with many concurrent operations.
- Asynchronous: Better scalability for applications that need to handle multiple concurrent operations or a large number of users.
4. Pros and Cons of Synchronous and Asynchronous Programming
Both synchronous and asynchronous programming have their advantages and disadvantages. Let’s examine the pros and cons of each approach:
Synchronous Programming
Pros:
- Simple and intuitive to understand
- Easier to reason about the program flow
- Straightforward debugging process
- Predictable order of execution
- Suitable for CPU-bound tasks
Cons:
- Can lead to performance bottlenecks, especially for I/O-bound operations
- May result in poor user experience in interactive applications
- Limited scalability for concurrent operations
- Inefficient use of system resources
Asynchronous Programming
Pros:
- Improved performance for I/O-bound operations
- Better responsiveness in interactive applications
- Efficient utilization of system resources
- Enhanced scalability for concurrent operations
- Suitable for event-driven architectures
Cons:
- Increased complexity in code structure and flow
- More challenging to debug and reason about
- Potential for race conditions and other concurrency issues
- Requires careful error handling
- May lead to callback hell if not properly managed
5. Use Cases for Synchronous and Asynchronous Programming
Choosing between synchronous and asynchronous programming depends on the specific requirements of your application. Here are some common use cases for each approach:
Synchronous Programming Use Cases:
- Simple, linear tasks with minimal I/O operations
- CPU-intensive computations
- Small-scale applications with predictable execution flows
- Scenarios where maintaining the exact order of operations is crucial
- Batch processing jobs
Asynchronous Programming Use Cases:
- Web applications handling multiple concurrent user requests
- I/O-bound operations (e.g., file operations, network requests, database queries)
- Real-time applications (e.g., chat applications, live updates)
- User interface responsiveness in desktop or mobile applications
- Long-running background tasks in server applications
- Microservices architectures with inter-service communication
6. Implementation Examples
To better understand the practical differences between synchronous and asynchronous programming, let’s look at some implementation examples in different programming languages:
Synchronous Example in Python
import time
def fetch_data_from_database():
print("Fetching data from database...")
time.sleep(2) # Simulating database query
return "Data from database"
def process_data(data):
print("Processing data...")
time.sleep(1) # Simulating data processing
return f"Processed {data}"
def main():
start_time = time.time()
data = fetch_data_from_database()
result = process_data(data)
print(result)
print(f"Total execution time: {time.time() - start_time:.2f} seconds")
if __name__ == "__main__":
main()
In this synchronous example, the program waits for each function to complete before moving on to the next one.
Asynchronous Example in JavaScript
function fetchDataFromDatabase() {
return new Promise((resolve) => {
console.log("Fetching data from database...");
setTimeout(() => {
resolve("Data from database");
}, 2000); // Simulating database query
});
}
function processData(data) {
return new Promise((resolve) => {
console.log("Processing data...");
setTimeout(() => {
resolve(`Processed ${data}`);
}, 1000); // Simulating data processing
});
}
async function main() {
const startTime = Date.now();
const data = await fetchDataFromDatabase();
const result = await processData(data);
console.log(result);
console.log(`Total execution time: ${(Date.now() - startTime) / 1000} seconds`);
}
main();
In this asynchronous example using JavaScript’s async/await syntax, the program can handle other tasks while waiting for the asynchronous operations to complete.
Asynchronous Example in C# (.NET)
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
var startTime = DateTime.Now;
var data = await FetchDataFromDatabaseAsync();
var result = await ProcessDataAsync(data);
Console.WriteLine(result);
Console.WriteLine($"Total execution time: {(DateTime.Now - startTime).TotalSeconds:F2} seconds");
}
static async Task<string> FetchDataFromDatabaseAsync()
{
Console.WriteLine("Fetching data from database...");
await Task.Delay(2000); // Simulating database query
return "Data from database";
}
static async Task<string> ProcessDataAsync(string data)
{
Console.WriteLine("Processing data...");
await Task.Delay(1000); // Simulating data processing
return $"Processed {data}";
}
}
This C# example demonstrates asynchronous programming using the async/await pattern, which is commonly used in .NET applications.
7. Best Practices for Choosing Between Synchronous and Asynchronous Programming
When deciding between synchronous and asynchronous programming approaches, consider the following best practices:
- Analyze your application’s requirements: Determine if your application is I/O-bound or CPU-bound, and choose the appropriate approach accordingly.
- Consider scalability needs: If your application needs to handle many concurrent operations or users, asynchronous programming may be more suitable.
- Evaluate the complexity trade-off: Asynchronous programming can improve performance but may increase code complexity. Ensure that the benefits outweigh the added complexity.
- Use asynchronous programming for I/O-bound operations: For tasks involving file I/O, network requests, or database queries, asynchronous programming can significantly improve performance.
- Stick to synchronous programming for CPU-bound tasks: For computationally intensive operations, synchronous programming may be more appropriate and easier to implement.
- Consider the user experience: For interactive applications, asynchronous programming can help maintain responsiveness and provide a better user experience.
- Use language-specific features: Leverage built-in language features and libraries for asynchronous programming, such as async/await in C# or JavaScript, or asyncio in Python.
- Be consistent: Try to maintain a consistent programming style throughout your application to improve readability and maintainability.
- Profile and measure performance: Use profiling tools to identify bottlenecks and measure the impact of switching between synchronous and asynchronous approaches.
- Handle errors properly: Implement robust error handling mechanisms, especially for asynchronous operations, to ensure your application remains stable and responsive.
8. Common Challenges and Solutions in Asynchronous Programming
While asynchronous programming offers many benefits, it also comes with its own set of challenges. Here are some common issues developers face when working with asynchronous code and their solutions:
1. Callback Hell
Challenge: Nested callbacks can lead to code that is difficult to read and maintain, often referred to as “callback hell” or the “pyramid of doom.”
Solution: Use Promises, async/await syntax, or other abstractions provided by your programming language to flatten the code structure and improve readability.
2. Error Handling
Challenge: Proper error handling in asynchronous code can be more complex than in synchronous code.
Solution: Use try-catch blocks with async/await, or leverage .catch() methods with Promises to handle errors consistently.
3. Race Conditions
Challenge: Asynchronous operations may complete in an unpredictable order, leading to race conditions.
Solution: Use synchronization primitives like mutexes or semaphores, or leverage higher-level abstractions like Promise.all() to coordinate multiple asynchronous operations.
4. Debugging Complexity
Challenge: Debugging asynchronous code can be more difficult due to its non-linear execution flow.
Solution: Use debugging tools that support asynchronous code, such as breakpoints in async functions, and leverage logging to track the flow of asynchronous operations.
5. Managing Concurrency
Challenge: Determining the appropriate level of concurrency for optimal performance can be challenging.
Solution: Use techniques like connection pooling, worker pools, or rate limiting to manage concurrency and prevent resource exhaustion.
6. Testing Asynchronous Code
Challenge: Writing and maintaining tests for asynchronous code can be more complex than for synchronous code.
Solution: Use testing frameworks that support asynchronous testing, such as Jest for JavaScript or pytest for Python, and leverage mocking libraries to simulate asynchronous behaviors.
9. Future Trends in Synchronous and Asynchronous Programming
As software development continues to evolve, several trends are shaping the future of synchronous and asynchronous programming:
- Increased adoption of asynchronous programming: With the growing demand for responsive and scalable applications, asynchronous programming is likely to become even more prevalent across various domains.
- Improved language support: Programming languages and frameworks are continuously improving their support for asynchronous programming, making it easier for developers to write efficient asynchronous code.
- Reactive programming: The reactive programming paradigm, which is closely related to asynchronous programming, is gaining popularity for building responsive and resilient applications.
- Serverless architectures: The rise of serverless computing is driving the need for efficient asynchronous programming techniques to handle event-driven workloads.
- Machine learning and AI integration: As AI becomes more integrated into software development, asynchronous programming will play a crucial role in managing complex, long-running ML tasks.
- Enhanced tooling: Development tools and IDEs are likely to provide better support for writing, debugging, and profiling asynchronous code.
- Hybrid approaches: We may see the emergence of new programming models that combine the benefits of both synchronous and asynchronous approaches, offering developers more flexibility in designing their applications.
10. Conclusion
Understanding the differences between synchronous and asynchronous programming is crucial for developing efficient and responsive applications. While synchronous programming offers simplicity and predictability, asynchronous programming provides improved performance and scalability, especially for I/O-bound operations.
As you progress in your coding journey and prepare for technical interviews, particularly for major tech companies, it’s essential to master both paradigms and know when to apply each one. By understanding the strengths and weaknesses of synchronous and asynchronous programming, you’ll be better equipped to design and implement robust, high-performance software solutions.
Remember to consider factors such as your application’s requirements, scalability needs, and the complexity trade-offs when choosing between synchronous and asynchronous approaches. With practice and experience, you’ll develop the intuition to make the right choice for each specific use case, ultimately leading to more efficient and maintainable code.
As you continue to hone your skills in algorithmic thinking and problem-solving, keep in mind that the ability to effectively utilize both synchronous and asynchronous programming techniques will be a valuable asset in your software development career.