Swift Package Manager: Simplifying Dependency Management in iOS Development
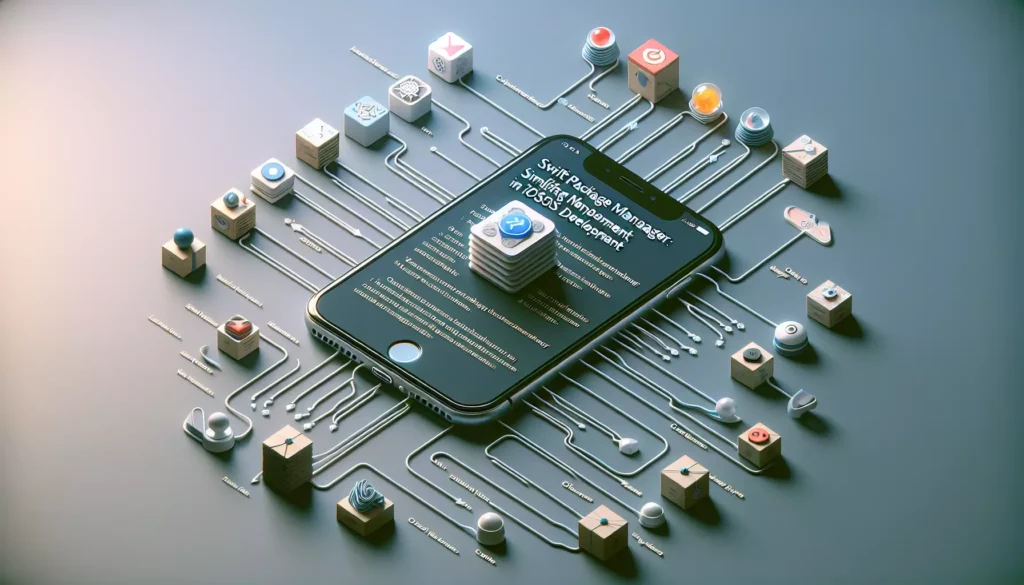
In the ever-evolving world of iOS development, managing dependencies efficiently is crucial for creating robust and maintainable applications. Enter the Swift Package Manager (SPM), a powerful tool that simplifies the process of incorporating external libraries and frameworks into your Swift projects. In this comprehensive guide, we’ll explore the ins and outs of the Swift Package Manager, its benefits, and how to leverage it effectively in your iOS development workflow.
What is the Swift Package Manager?
The Swift Package Manager is an open-source tool developed by Apple that automates the process of downloading, compiling, and linking dependencies for Swift projects. It was introduced alongside Swift 3.0 and has since become an integral part of the Swift ecosystem. SPM allows developers to easily manage third-party libraries, frameworks, and even their own modular code, streamlining the development process and promoting code reuse.
Key Features of Swift Package Manager
- Dependency Resolution: SPM automatically resolves and downloads the required dependencies for your project.
- Version Control: It supports semantic versioning, allowing you to specify version constraints for your dependencies.
- Xcode Integration: Since Xcode 11, SPM has been fully integrated into the IDE, making it easy to add and manage packages directly from the Xcode interface.
- Command-line Interface: SPM can be used via the command line, providing flexibility for developers who prefer terminal-based workflows.
- Cross-platform Support: It works across various Apple platforms, including iOS, macOS, tvOS, and watchOS.
- Package Creation: Developers can create their own packages to share code across projects or with the community.
Benefits of Using Swift Package Manager
1. Simplified Dependency Management
SPM eliminates the need for manual dependency management or third-party dependency managers like CocoaPods or Carthage. It streamlines the process of adding, updating, and removing dependencies, saving developers valuable time and effort.
2. Improved Project Organization
By using SPM, you can keep your project structure clean and organized. Dependencies are stored separately from your main project files, making it easier to manage and maintain your codebase.
3. Faster Build Times
SPM optimizes the build process by only compiling the necessary components of each dependency. This can lead to faster build times, especially for larger projects with multiple dependencies.
4. Version Control Integration
SPM integrates seamlessly with version control systems like Git. It uses a manifest file (Package.swift) to define dependencies, making it easy to track changes and collaborate with team members.
5. Native Apple Support
As an official Apple tool, SPM receives regular updates and improvements alongside new Swift and Xcode releases. This ensures long-term support and compatibility with the latest iOS development technologies.
Getting Started with Swift Package Manager
Adding a Package to Your Xcode Project
To add a package to your Xcode project using SPM, follow these steps:
- Open your Xcode project.
- Go to File > Swift Packages > Add Package Dependency.
- Enter the URL of the package’s Git repository.
- Choose the version or branch you want to use.
- Select the target where you want to add the package.
- Click Finish.
Xcode will then download and integrate the package into your project.
Using SPM via Command Line
For those who prefer working with the command line, you can use SPM outside of Xcode. Here’s a basic example of how to create a new package:
mkdir MyPackage
cd MyPackage
swift package init --type library
This creates a new Swift package with a basic structure. You can then edit the Package.swift file to define your package’s dependencies and targets.
Creating Your Own Swift Package
Creating your own Swift package is a great way to modularize your code and share it across projects or with the community. Here’s a step-by-step guide to creating a simple Swift package:
1. Create the Package Structure
Use the Swift package init command to create a new package:
swift package init --name MyAwesomePackage --type library
This command creates a basic package structure with the following files and directories:
- Package.swift: The manifest file that defines your package’s name, dependencies, and targets.
- Sources/MyAwesomePackage: Directory for your package’s source code.
- Tests/MyAwesomePackageTests: Directory for your package’s unit tests.
2. Define Your Package in Package.swift
Open the Package.swift file and modify it to define your package’s structure, dependencies, and targets. Here’s an example:
// swift-tools-version:5.3
import PackageDescription
let package = Package(
name: "MyAwesomePackage",
platforms: [
.iOS(.v13),
.macOS(.v10_15)
],
products: [
.library(
name: "MyAwesomePackage",
targets: ["MyAwesomePackage"]),
],
dependencies: [
.package(url: "https://github.com/Alamofire/Alamofire.git", .upToNextMajor(from: "5.4.0"))
],
targets: [
.target(
name: "MyAwesomePackage",
dependencies: ["Alamofire"]),
.testTarget(
name: "MyAwesomePackageTests",
dependencies: ["MyAwesomePackage"]),
]
)
This example defines a package that:
- Supports iOS 13+ and macOS 10.15+
- Includes one library product named “MyAwesomePackage”
- Depends on the Alamofire networking library
- Has one main target and one test target
3. Implement Your Package Functionality
Add your Swift code to the Sources/MyAwesomePackage directory. For example, you might create a file called MyAwesomeFeature.swift:
import Foundation
import Alamofire
public struct MyAwesomeFeature {
public static func fetchData(from url: URL, completion: @escaping (Result<Data, Error>) -> Void) {
AF.request(url).responseData { response in
switch response.result {
case .success(let data):
completion(.success(data))
case .failure(let error):
completion(.failure(error))
}
}
}
}
4. Write Tests
Add unit tests to the Tests/MyAwesomePackageTests directory to ensure your package functions correctly. Here’s a simple example:
import XCTest
@testable import MyAwesomePackage
final class MyAwesomePackageTests: XCTestCase {
func testFetchData() {
let expectation = self.expectation(description: "Fetch data")
let url = URL(string: "https://api.example.com/data")!
MyAwesomeFeature.fetchData(from: url) { result in
switch result {
case .success(let data):
XCTAssertFalse(data.isEmpty)
case .failure(let error):
XCTFail("Fetch failed with error: \(error)")
}
expectation.fulfill()
}
waitForExpectations(timeout: 5, handler: nil)
}
}
5. Build and Test Your Package
Use the following commands to build and test your package:
swift build
swift test
6. Version and Publish Your Package
Once you’re satisfied with your package, you can version it using Git tags and publish it to a Git repository:
git init
git add .
git commit -m "Initial commit"
git tag 1.0.0
git remote add origin https://github.com/yourusername/MyAwesomePackage.git
git push origin master --tags
Now your package is ready to be used by other developers!
Best Practices for Using Swift Package Manager
1. Keep Your Dependencies Up to Date
Regularly update your dependencies to benefit from bug fixes, performance improvements, and new features. You can update packages in Xcode by selecting File > Swift Packages > Update to Latest Package Versions.
2. Use Semantic Versioning
When specifying version requirements for dependencies, use semantic versioning to ensure compatibility. For example:
.package(url: "https://github.com/example/package.git", from: "1.0.0")
3. Minimize Dependencies
While it’s tempting to add many third-party packages to your project, try to minimize dependencies to reduce potential conflicts and maintain a smaller codebase. Only include packages that provide significant value to your project.
4. Use Package Collections
Package Collections, introduced in Swift 5.5, allow you to group related packages together. This feature can help you discover and manage packages more efficiently, especially for larger projects or teams.
5. Leverage Local Packages for Development
When developing your own packages, you can use local package dependencies to test changes without pushing to a remote repository. In your Package.swift file, use:
.package(path: "../MyLocalPackage")
6. Document Your Packages
If you’re creating packages for others to use, provide clear documentation on how to integrate and use your package. Include a README.md file with installation instructions, usage examples, and API documentation.
Advanced Swift Package Manager Features
1. Conditional Dependencies
SPM allows you to specify conditional dependencies based on platforms or Swift versions. This is useful when certain dependencies are only needed for specific platforms:
package.dependencies = [
.package(url: "https://github.com/example/ios-only-package.git", from: "1.0.0"),
]
package.targets = [
.target(
name: "MyTarget",
dependencies: [
.target(name: "MyOtherTarget"),
.product(name: "iOSOnlyPackage", package: "ios-only-package", condition: .when(platforms: [.iOS]))
]
)
]
2. Resources and Localization
SPM supports including resources like images, JSON files, and localized strings in your packages. To include resources, add them to your target in the Package.swift file:
.target(
name: "MyTarget",
dependencies: [],
resources: [
.process("Resources"),
.copy("Config.plist")
]
)
3. Binary Targets
For frameworks that don’t provide source code, you can use binary targets in SPM. This is useful for integrating closed-source SDKs:
.binaryTarget(
name: "MyBinaryFramework",
url: "https://example.com/MyFramework.xcframework.zip",
checksum: "4ee89dbee7f5552f59c1120dba35f297e4c9e83fb75390cd0a908e19704b02f2"
)
4. Plugin Support
Swift Package Manager now supports plugins, allowing you to extend the build process or add custom commands. This feature is particularly useful for tasks like code generation or asset processing.
Troubleshooting Common Swift Package Manager Issues
1. Version Conflicts
If you encounter version conflicts between packages, try updating to the latest compatible versions or specify more precise version requirements in your Package.swift file.
2. Build Errors
For build errors, ensure that all dependencies are compatible with your Swift version and target platforms. You may need to update your package or project settings.
3. Missing Packages
If Xcode fails to fetch packages, check your internet connection and ensure the package URLs are correct. You can also try cleaning the build folder and derived data.
4. Integration Issues
If you’re having trouble integrating a package into your project, make sure you’ve added it to the correct target and imported it properly in your Swift files.
Conclusion
The Swift Package Manager has revolutionized dependency management in iOS development, offering a streamlined, integrated solution for handling external libraries and frameworks. By leveraging SPM, developers can focus more on writing great code and less on managing dependencies.
As you continue your journey in iOS development, mastering the Swift Package Manager will prove invaluable. It not only simplifies your workflow but also opens up opportunities to create and share your own packages, contributing to the vibrant Swift community.
Remember, effective use of SPM is just one aspect of becoming a proficient iOS developer. To truly excel in your coding career, it’s essential to continually expand your knowledge and skills across various areas of software development. Platforms like AlgoCademy offer comprehensive resources and interactive tutorials to help you progress from beginner-level coding to tackling complex algorithmic challenges and preparing for technical interviews at top tech companies.
By combining your growing expertise in tools like the Swift Package Manager with a solid foundation in algorithmic thinking and problem-solving skills, you’ll be well-equipped to tackle any challenge in your iOS development journey and beyond. Keep learning, keep coding, and embrace the power of Swift and its ecosystem to build amazing applications!