Study Software Sustainability Practices: Developing Maintainable and Scalable Software
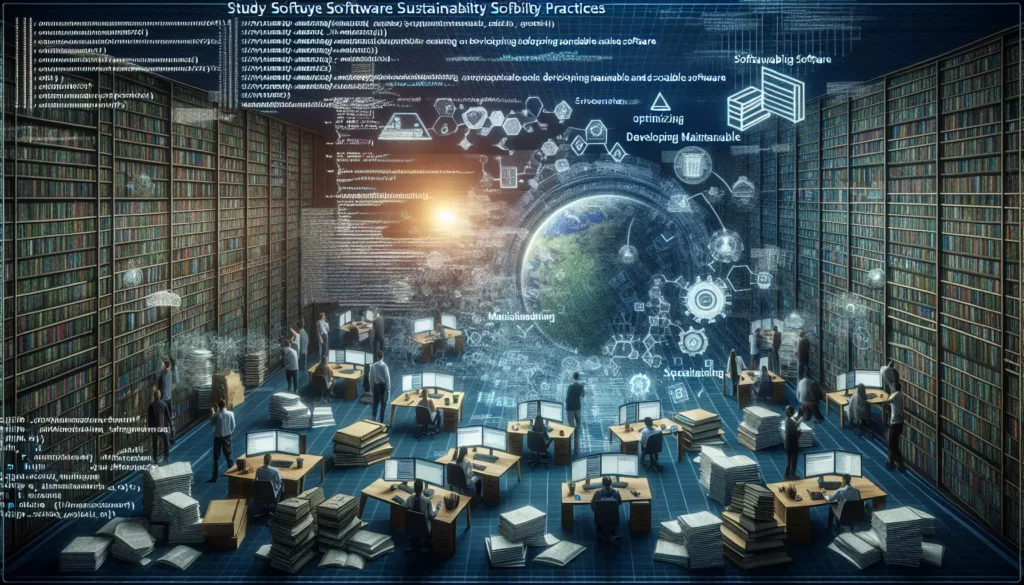
In the ever-evolving landscape of software development, creating sustainable, maintainable, and scalable software is crucial for long-term success. As coding education platforms like AlgoCademy continue to grow and adapt to the needs of learners preparing for technical interviews at major tech companies, it’s essential to understand and implement best practices for software sustainability. This comprehensive guide will explore the importance of sustainable software development and provide practical strategies for creating maintainable and scalable applications.
Understanding Software Sustainability
Software sustainability refers to the ability of a software system to endure and evolve over time while maintaining its functionality, performance, and reliability. Sustainable software is designed to be easily maintained, updated, and expanded without requiring significant rewrites or causing disruptions to existing features. This concept is particularly important for platforms like AlgoCademy, which must continuously adapt to new programming languages, algorithms, and industry trends to provide relevant coding education.
Key Aspects of Software Sustainability
- Maintainability: The ease with which software can be modified, updated, or repaired
- Scalability: The ability of the software to handle increased workload or growth
- Adaptability: The capacity to incorporate new features or technologies
- Reliability: Consistent performance and stability over time
- Efficiency: Optimal use of resources and energy
Best Practices for Developing Maintainable Software
Maintainable software is essential for long-term success, especially in the context of coding education platforms like AlgoCademy. Here are some best practices to ensure your software remains maintainable:
1. Write Clean, Readable Code
Clean code is easier to understand, modify, and debug. Follow these guidelines:
- Use meaningful variable and function names
- Keep functions small and focused on a single task
- Follow consistent coding standards and style guides
- Use comments judiciously to explain complex logic or algorithms
Example of clean, readable code:
// Calculate the factorial of a number
function calculateFactorial(number) {
if (number <= 1) {
return 1;
}
return number * calculateFactorial(number - 1);
}
// Usage
const result = calculateFactorial(5);
console.log(`Factorial of 5 is: ${result}`);
2. Implement Modular Design
Modular design involves breaking down your software into smaller, independent components. This approach offers several benefits:
- Easier to understand and maintain individual components
- Facilitates code reuse and reduces duplication
- Simplifies testing and debugging
- Allows for easier updates and feature additions
Example of modular design in a coding education platform:
// User authentication module
const authModule = {
login: (username, password) => { /* ... */ },
logout: () => { /* ... */ },
resetPassword: (email) => { /* ... */ }
};
// Course management module
const courseModule = {
createCourse: (courseData) => { /* ... */ },
updateCourse: (courseId, updatedData) => { /* ... */ },
deleteCourse: (courseId) => { /* ... */ }
};
// Progress tracking module
const progressModule = {
updateProgress: (userId, courseId, progress) => { /* ... */ },
getProgress: (userId, courseId) => { /* ... */ }
};
3. Use Version Control Systems
Version control systems like Git are essential for maintaining software projects. They offer:
- Tracking changes over time
- Collaboration among team members
- Easy rollback to previous versions
- Branch management for feature development and experimentation
Example of basic Git commands:
git init # Initialize a new Git repository
git add . # Stage all changes
git commit -m "Add new feature: interactive code editor"
git push origin main # Push changes to remote repository
git branch feature-user-profiles # Create a new branch
git checkout feature-user-profiles # Switch to the new branch
4. Write Comprehensive Documentation
Good documentation is crucial for maintainable software. It should include:
- README files explaining project setup and basic usage
- API documentation for all public interfaces
- Inline comments for complex algorithms or business logic
- Architecture diagrams and system design documents
Example of a README.md file for AlgoCademy:
# AlgoCademy
## About
AlgoCademy is an interactive coding education platform designed to help learners progress from beginner-level coding to preparing for technical interviews at major tech companies.
## Features
- Interactive coding tutorials
- AI-powered assistance
- Problem-solving challenges
- FAANG interview preparation
## Getting Started
1. Clone the repository: `git clone https://github.com/algocademy/platform.git`
2. Install dependencies: `npm install`
3. Set up environment variables: `cp .env.example .env` and edit as needed
4. Run the development server: `npm run dev`
## Contributing
Please read our [Contributing Guide](CONTRIBUTING.md) for details on our code of conduct and the process for submitting pull requests.
## License
This project is licensed under the MIT License - see the [LICENSE](LICENSE) file for details.
5. Implement Automated Testing
Automated tests help maintain software quality and catch regressions early. Implement various types of tests:
- Unit tests for individual functions and components
- Integration tests for interactions between modules
- End-to-end tests for complete user flows
- Performance tests to ensure scalability
Example of a unit test using Jest:
// Function to test
function isPalindrome(str) {
const cleanStr = str.toLowerCase().replace(/[^a-z0-9]/g, '');
return cleanStr === cleanStr.split('').reverse().join('');
}
// Test suite
describe('isPalindrome function', () => {
test('returns true for palindromes', () => {
expect(isPalindrome('A man, a plan, a canal: Panama')).toBe(true);
expect(isPalindrome('race a car')).toBe(false);
expect(isPalindrome('Was it a car or a cat I saw?')).toBe(true);
});
test('handles empty strings', () => {
expect(isPalindrome('')).toBe(true);
});
test('is case-insensitive', () => {
expect(isPalindrome('Madam')).toBe(true);
});
});
Strategies for Developing Scalable Software
Scalability is crucial for platforms like AlgoCademy that may experience rapid growth in users and content. Here are strategies to ensure your software can scale effectively:
1. Design for Horizontal Scaling
Horizontal scaling involves adding more machines to your system to distribute the load. This approach is often more flexible and cost-effective than vertical scaling (adding more resources to a single machine). To design for horizontal scaling:
- Use stateless components where possible
- Implement load balancing to distribute traffic
- Use distributed caching systems like Redis
- Design your database schema for sharding
Example of a simple load balancer configuration using Nginx:
http {
upstream backend {
server backend1.example.com;
server backend2.example.com;
server backend3.example.com;
}
server {
listen 80;
location / {
proxy_pass http://backend;
}
}
}
2. Implement Caching Strategies
Caching can significantly improve performance and reduce load on your servers. Implement caching at various levels:
- Browser caching for static assets
- Application-level caching for frequently accessed data
- Database query result caching
- Content Delivery Networks (CDNs) for global distribution
Example of implementing Redis caching in a Node.js application:
const redis = require('redis');
const client = redis.createClient();
async function getCachedData(key) {
return new Promise((resolve, reject) => {
client.get(key, (err, data) => {
if (err) reject(err);
if (data !== null) {
resolve(JSON.parse(data));
} else {
resolve(null);
}
});
});
}
async function setCachedData(key, data, expirationInSeconds) {
return new Promise((resolve, reject) => {
client.setex(key, expirationInSeconds, JSON.stringify(data), (err) => {
if (err) reject(err);
resolve();
});
});
}
// Usage
async function getUserProfile(userId) {
const cacheKey = `user:${userId}`;
let userProfile = await getCachedData(cacheKey);
if (!userProfile) {
userProfile = await fetchUserProfileFromDatabase(userId);
await setCachedData(cacheKey, userProfile, 3600); // Cache for 1 hour
}
return userProfile;
}
3. Use Asynchronous Processing
Asynchronous processing can improve the responsiveness and scalability of your application by offloading time-consuming tasks to background processes. This is particularly useful for coding education platforms that may need to compile and run user-submitted code. Implement asynchronous processing using:
- Message queues (e.g., RabbitMQ, Apache Kafka)
- Background job processors (e.g., Sidekiq for Ruby, Celery for Python)
- Serverless functions for event-driven processing
Example of using a message queue for asynchronous code execution:
const amqp = require('amqplib');
// Producer: Submit code for execution
async function submitCodeForExecution(code, language) {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'code_execution_queue';
await channel.assertQueue(queue, { durable: true });
channel.sendToQueue(queue, Buffer.from(JSON.stringify({ code, language })), { persistent: true });
console.log(`Code submitted for execution: ${code}`);
await channel.close();
await connection.close();
}
// Consumer: Execute code and store results
async function executeCodeWorker() {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const queue = 'code_execution_queue';
await channel.assertQueue(queue, { durable: true });
channel.prefetch(1);
console.log('Waiting for code execution tasks...');
channel.consume(queue, async (msg) => {
const { code, language } = JSON.parse(msg.content.toString());
console.log(`Executing ${language} code: ${code}`);
// Simulate code execution
const result = await executeCode(code, language);
// Store the result (e.g., in a database)
await storeExecutionResult(result);
channel.ack(msg);
}, { noAck: false });
}
// Start the worker
executeCodeWorker();
4. Optimize Database Performance
Database optimization is crucial for maintaining performance as your data grows. Consider the following strategies:
- Index frequently queried columns
- Use database connection pooling
- Implement database sharding for horizontal scaling
- Optimize queries and use query caching
- Consider using NoSQL databases for certain use cases
Example of creating an index in SQL:
CREATE INDEX idx_user_email ON users (email);
-- Query that benefits from the index
SELECT * FROM users WHERE email = 'user@example.com';
5. Implement Microservices Architecture
A microservices architecture can improve scalability by breaking down your application into smaller, independently deployable services. This approach offers several benefits:
- Services can be scaled independently based on demand
- Easier to maintain and update individual components
- Allows for using different technologies for different services
- Improves fault isolation and overall system resilience
Example of a microservices architecture for AlgoCademy:
// User Service
const userService = {
createUser: (userData) => { /* ... */ },
getUserProfile: (userId) => { /* ... */ },
updateUser: (userId, updates) => { /* ... */ }
};
// Course Service
const courseService = {
createCourse: (courseData) => { /* ... */ },
getCourseDetails: (courseId) => { /* ... */ },
updateCourse: (courseId, updates) => { /* ... */ }
};
// Code Execution Service
const codeExecutionService = {
executeCode: (code, language) => { /* ... */ },
getExecutionResult: (executionId) => { /* ... */ }
};
// Progress Tracking Service
const progressService = {
updateProgress: (userId, courseId, progress) => { /* ... */ },
getProgress: (userId, courseId) => { /* ... */ }
};
// API Gateway
const apiGateway = {
handleRequest: (request) => {
switch (request.path) {
case '/users':
return userService.handleRequest(request);
case '/courses':
return courseService.handleRequest(request);
case '/execute':
return codeExecutionService.handleRequest(request);
case '/progress':
return progressService.handleRequest(request);
default:
throw new Error('Not Found');
}
}
};
Continuous Improvement and Monitoring
Developing sustainable software is an ongoing process. Implement these practices to ensure continuous improvement:
- Set up monitoring and logging to track system performance and errors
- Regularly review and refactor code to improve maintainability
- Conduct code reviews to ensure adherence to best practices
- Stay updated with new technologies and industry trends
- Gather and act on user feedback to improve the platform
Conclusion
Developing maintainable and scalable software is crucial for the long-term success of coding education platforms like AlgoCademy. By implementing best practices for clean code, modular design, automated testing, and scalable architecture, you can create a robust foundation that can grow and adapt to meet the evolving needs of learners and the tech industry.
Remember that software sustainability is an ongoing process that requires continuous attention and improvement. Regularly assess your codebase, architecture, and development practices to ensure they align with your goals for maintainability and scalability. By prioritizing these aspects of software development, you’ll be better equipped to provide a high-quality, reliable platform that can effectively support learners on their journey from beginner coding to FAANG interview preparation.
As you continue to develop and improve your coding education platform, keep in mind the importance of balancing feature development with maintaining a sustainable codebase. This approach will not only benefit your development team but also provide a more stable and efficient learning experience for your users, ultimately contributing to the success of both your platform and the aspiring developers it serves.