Structuring Your Programming Self-Learning Journey: A Comprehensive Guide
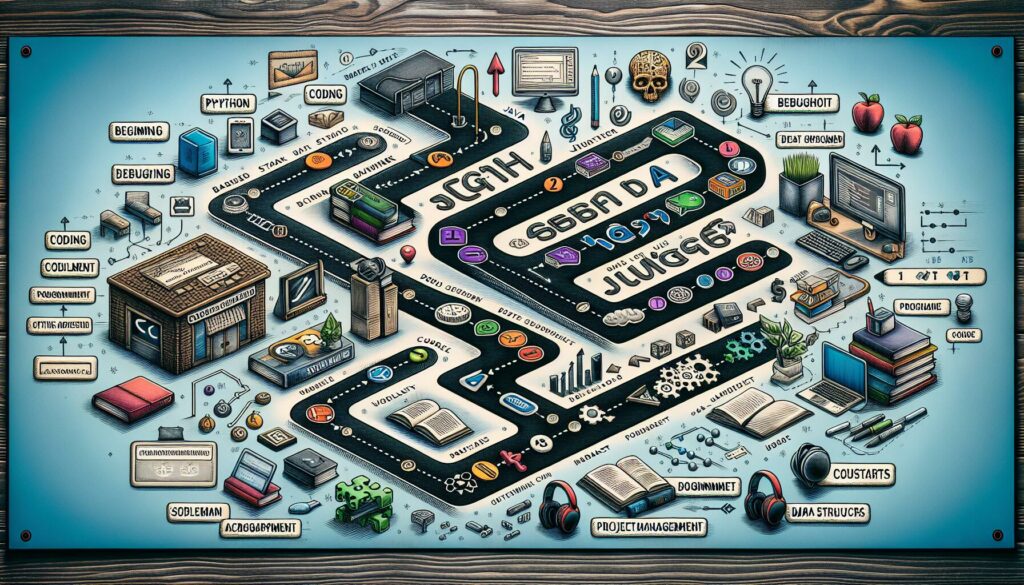
In today’s digital age, programming has become an essential skill across various industries. Whether you’re looking to switch careers, enhance your current job prospects, or simply explore a new hobby, learning to code can be an exciting and rewarding journey. However, with the vast array of programming languages, resources, and learning paths available, it’s easy to feel overwhelmed when starting out. This comprehensive guide will help you structure your programming self-learning journey, providing you with a clear roadmap to success.
1. Define Your Goals
Before diving into the world of programming, it’s crucial to define your goals. Ask yourself:
- Why do you want to learn programming?
- What do you hope to achieve with your new skills?
- Are you interested in web development, mobile app creation, data science, or something else?
- Do you have a specific project in mind?
Having clear objectives will help you choose the right programming languages and resources to focus on, saving you time and keeping you motivated throughout your learning journey.
2. Choose Your First Programming Language
Selecting your first programming language can be daunting, but it’s important to remember that many programming concepts are transferable between languages. Here are some popular options for beginners:
- Python: Known for its simplicity and readability, Python is an excellent choice for beginners. It’s versatile and used in web development, data science, artificial intelligence, and more.
- JavaScript: If you’re interested in web development, JavaScript is essential. It’s used for both front-end and back-end development and has a vast ecosystem of libraries and frameworks.
- Java: A popular choice for enterprise applications and Android development, Java has a steeper learning curve but offers robust object-oriented programming features.
- C#: Ideal for Windows application development and game development using Unity, C# is another versatile language with a syntax similar to Java.
Choose a language that aligns with your goals and interests. Remember, the first language you learn will lay the foundation for your programming journey, so focus on understanding core concepts rather than trying to master every aspect of the language.
3. Set Up Your Development Environment
Once you’ve chosen a language, you’ll need to set up your development environment. This typically involves:
- Installing a code editor or Integrated Development Environment (IDE)
- Setting up the necessary compilers or interpreters for your chosen language
- Configuring any required tools or libraries
Popular code editors and IDEs include:
- Visual Studio Code: A lightweight, customizable editor suitable for multiple languages
- PyCharm: An IDE specifically designed for Python development
- IntelliJ IDEA: A powerful IDE for Java development
- Sublime Text: A fast, minimalist editor for various programming languages
Many of these editors offer free versions or trials, so experiment to find the one that suits your needs and preferences.
4. Start with the Basics
Begin your learning journey by mastering the fundamental concepts of programming. These typically include:
- Variables and data types
- Control structures (if statements, loops)
- Functions and methods
- Basic input/output operations
- Error handling
Focus on understanding these core concepts thoroughly, as they form the building blocks for more advanced programming topics. Here’s a simple example of some basic concepts in Python:
# Variables and data types
name = "Alice"
age = 30
height = 1.75
# Control structures
if age >= 18:
print(f"{name} is an adult.")
else:
print(f"{name} is a minor.")
# Functions
def greet(person):
return f"Hello, {person}!"
# Using the function
message = greet(name)
print(message)
5. Practice, Practice, Practice
Programming is a skill that improves with practice. As you learn new concepts, apply them by writing code regularly. Here are some ways to practice:
- Coding challenges: Websites like LeetCode, HackerRank, and CodeWars offer programming challenges of varying difficulty levels.
- Personal projects: Start small projects that interest you, such as a calculator app or a simple game.
- Contribute to open-source: Once you’ve gained some confidence, consider contributing to open-source projects on platforms like GitHub.
Remember, making mistakes is part of the learning process. Don’t be afraid to experiment and learn from your errors.
6. Utilize Online Resources
There’s a wealth of online resources available for learning programming. Some popular options include:
- Online courses: Platforms like Coursera, edX, and Udacity offer structured courses, often from renowned universities.
- Video tutorials: YouTube channels and websites like freeCodeCamp provide free video tutorials on various programming topics.
- Interactive learning platforms: Websites like Codecademy and FreeCodeCamp offer interactive coding lessons.
- Documentation: Official documentation for programming languages and libraries is an invaluable resource for learning and reference.
Mix and match these resources to find a learning style that works best for you. Remember to supplement your learning with practical coding exercises.
7. Join a Community
Learning to code can sometimes feel isolating, but you don’t have to go it alone. Joining a programming community can provide support, motivation, and valuable insights. Consider:
- Joining online forums like Stack Overflow or Reddit’s programming communities
- Participating in local coding meetups or hackathons
- Engaging with other learners through social media or Discord channels
- Finding a mentor who can guide you and provide feedback on your progress
Interacting with other programmers can expose you to different perspectives, problem-solving approaches, and coding best practices.
8. Learn Version Control
As you progress in your programming journey, learning version control becomes crucial. Git is the most widely used version control system, and understanding its basics will serve you well in both personal and professional projects. Start by:
- Installing Git on your computer
- Learning basic Git commands (init, add, commit, push, pull)
- Creating a GitHub account and pushing your projects to remote repositories
Here’s a simple example of basic Git commands:
# Initialize a new Git repository
git init
# Add files to the staging area
git add .
# Commit changes
git commit -m "Initial commit"
# Push changes to a remote repository
git push origin main
9. Dive Deeper into Your Chosen Language
Once you’ve mastered the basics, it’s time to explore more advanced concepts in your chosen programming language. This might include:
- Object-oriented programming (OOP) principles
- Data structures and algorithms
- File I/O and database operations
- Asynchronous programming
- Testing and debugging techniques
As you learn these concepts, try to apply them in your projects to reinforce your understanding. Here’s an example of a simple class definition in Python, demonstrating some OOP principles:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.odometer = 0
def drive(self, miles):
self.odometer += miles
print(f"Drove {miles} miles. Total: {self.odometer} miles.")
def get_info(self):
return f"{self.year} {self.make} {self.model}, Mileage: {self.odometer}"
# Using the Car class
my_car = Car("Toyota", "Corolla", 2022)
my_car.drive(100)
print(my_car.get_info())
10. Explore Frameworks and Libraries
As you become more proficient, start exploring frameworks and libraries relevant to your area of interest. These tools can significantly boost your productivity and open up new possibilities. Some popular examples include:
- Web development: React, Angular, Vue.js (JavaScript), Django, Flask (Python)
- Data science: NumPy, Pandas, Scikit-learn (Python)
- Mobile development: React Native (JavaScript), Flutter (Dart)
- Game development: Unity (C#), Pygame (Python)
Learning to use these tools effectively can make you a more versatile and efficient programmer.
11. Build a Portfolio
As you progress in your learning journey, start building a portfolio of projects. This serves two purposes:
- It demonstrates your skills and creativity to potential employers or clients
- It reinforces your learning by applying your skills to real-world problems
Your portfolio can include:
- Personal projects you’ve built from scratch
- Contributions to open-source projects
- Code samples demonstrating your proficiency in various concepts
- A personal website showcasing your skills and projects
Host your portfolio on platforms like GitHub Pages or Netlify to make it easily accessible to others.
12. Stay Updated and Continue Learning
The field of programming is constantly evolving, with new languages, frameworks, and best practices emerging regularly. To stay relevant and continue growing as a programmer:
- Follow tech blogs and news sites to stay informed about industry trends
- Attend webinars, conferences, or workshops to learn from experts
- Experiment with new technologies and programming paradigms
- Consider obtaining relevant certifications to validate your skills
Remember that learning to code is a lifelong journey. Even experienced programmers are constantly learning and adapting to new technologies.
13. Develop Soft Skills
While technical skills are crucial, don’t neglect the importance of soft skills in your programming journey. These include:
- Problem-solving: Break down complex problems into manageable parts
- Communication: Clearly explain technical concepts to both technical and non-technical audiences
- Collaboration: Work effectively in team environments, both in-person and remotely
- Time management: Prioritize tasks and manage your learning and project timelines effectively
- Adaptability: Be open to learning new technologies and approaches as the field evolves
These skills will complement your technical abilities and make you a well-rounded programmer.
Conclusion
Embarking on a programming self-learning journey can be challenging, but with the right structure and mindset, it can also be incredibly rewarding. Remember to:
- Set clear goals and choose a learning path that aligns with your objectives
- Start with the basics and gradually build your skills
- Practice regularly and work on projects that interest you
- Utilize a variety of learning resources and join a supportive community
- Build a portfolio to showcase your skills
- Stay updated with industry trends and continue learning throughout your career
By following these steps and maintaining your passion for coding, you’ll be well on your way to becoming a proficient programmer. Remember that everyone’s learning journey is unique, so be patient with yourself and celebrate your progress along the way. Happy coding!