String to Int in Java: A Comprehensive Guide
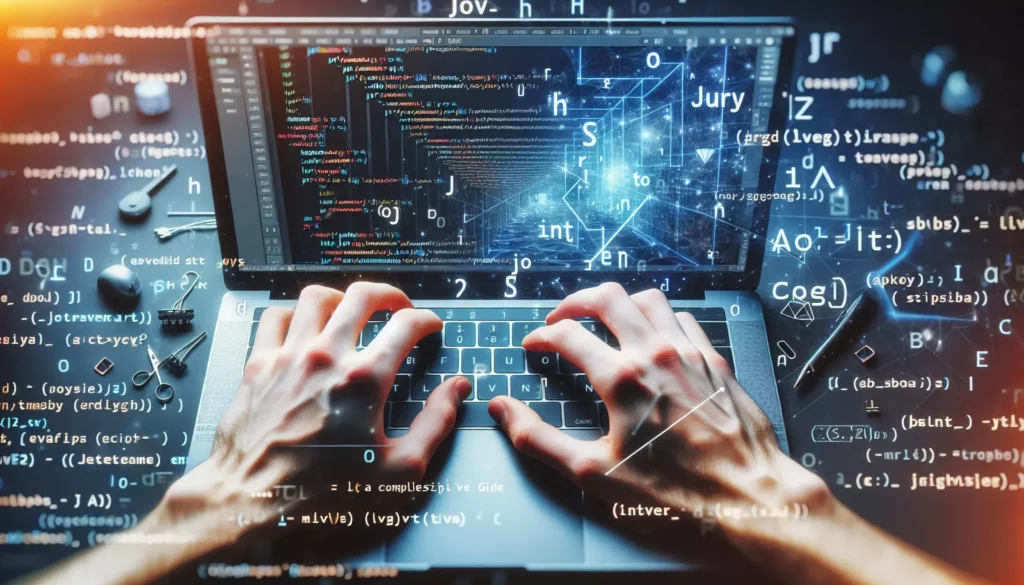
Converting a string to an integer is a common task in Java programming. Whether you’re working with user input, parsing data from files, or manipulating strings in various ways, knowing how to efficiently and safely convert strings to integers is essential. In this comprehensive guide, we’ll explore multiple methods to perform this conversion, discuss their pros and cons, and provide practical examples to help you master this fundamental skill.
Table of Contents
- Introduction
- Using Integer.parseInt()
- Using Integer.valueOf()
- Handling NumberFormatException
- Implementing Your Own atoi() Method
- Using Scanner Class
- Using Character Class Methods
- Best Practices and Performance Considerations
- Common Pitfalls and How to Avoid Them
- Advanced Techniques and Edge Cases
- Conclusion
1. Introduction
Converting strings to integers is a fundamental operation in Java programming. It’s often necessary when dealing with user input, reading data from files, or processing text-based information. Java provides several built-in methods to perform this conversion, each with its own advantages and use cases.
In this article, we’ll cover the most common and efficient ways to convert strings to integers in Java, including:
- Using Integer.parseInt()
- Using Integer.valueOf()
- Handling exceptions
- Implementing a custom atoi() method
- Using the Scanner class
- Leveraging Character class methods
We’ll also discuss best practices, performance considerations, and how to handle edge cases and potential errors.
2. Using Integer.parseInt()
The most straightforward and commonly used method to convert a string to an integer in Java is the Integer.parseInt()
method. This method takes a string as input and returns the corresponding integer value.
Basic Usage
String strNumber = "123";
int number = Integer.parseInt(strNumber);
System.out.println("Converted integer: " + number);
Output:
Converted integer: 123
Handling Different Radixes
The parseInt()
method also allows you to specify the radix (base) of the number system. This is particularly useful when dealing with numbers in different bases, such as binary, octal, or hexadecimal.
String binaryString = "1010";
int binaryToDecimal = Integer.parseInt(binaryString, 2);
System.out.println("Binary to Decimal: " + binaryToDecimal);
String hexString = "1A";
int hexToDecimal = Integer.parseInt(hexString, 16);
System.out.println("Hex to Decimal: " + hexToDecimal);
Output:
Binary to Decimal: 10
Hex to Decimal: 26
Advantages of Integer.parseInt()
- Simple and straightforward to use
- Supports different radixes
- Returns a primitive int, which is more memory-efficient for simple use cases
Disadvantages of Integer.parseInt()
- Throws NumberFormatException if the input is not a valid integer
- Cannot handle null inputs
3. Using Integer.valueOf()
Another common method for converting strings to integers is Integer.valueOf()
. This method is similar to parseInt()
, but it returns an Integer object instead of a primitive int.
Basic Usage
String strNumber = "456";
Integer number = Integer.valueOf(strNumber);
System.out.println("Converted integer: " + number);
Output:
Converted integer: 456
Advantages of Integer.valueOf()
- Returns an Integer object, which can be useful in certain contexts (e.g., when working with collections)
- Caches frequently used integer values (-128 to 127) for better performance
- Can be used directly in autoboxing contexts
Disadvantages of Integer.valueOf()
- Slightly slower than parseInt() for values outside the cached range
- Still throws NumberFormatException for invalid inputs
When to Use valueOf() vs parseInt()
Choose valueOf()
when:
- You need an Integer object rather than a primitive int
- You’re working with values that are likely to be in the cached range (-128 to 127)
- You’re using the result in a context that requires autoboxing
Choose parseInt()
when:
- You need a primitive int
- You’re working with a large number of conversions and performance is critical
- You’re dealing with values outside the cached range
4. Handling NumberFormatException
Both Integer.parseInt()
and Integer.valueOf()
can throw a NumberFormatException if the input string is not a valid integer. It’s important to handle this exception to prevent your program from crashing when dealing with potentially invalid input.
Basic Exception Handling
String input = "123abc";
try {
int number = Integer.parseInt(input);
System.out.println("Converted number: " + number);
} catch (NumberFormatException e) {
System.out.println("Invalid input: " + input);
}
Output:
Invalid input: 123abc
Creating a Safe Conversion Method
You can create a utility method that safely converts a string to an integer, returning a default value if the conversion fails:
public static int safeParseInt(String str, int defaultValue) {
try {
return Integer.parseInt(str);
} catch (NumberFormatException e) {
return defaultValue;
}
}
// Usage
String validInput = "789";
String invalidInput = "456def";
int result1 = safeParseInt(validInput, 0);
int result2 = safeParseInt(invalidInput, -1);
System.out.println("Result 1: " + result1);
System.out.println("Result 2: " + result2);
Output:
Result 1: 789
Result 2: -1
5. Implementing Your Own atoi() Method
While Java provides built-in methods for string to integer conversion, implementing your own atoi()
(ASCII to Integer) method can be a valuable exercise in understanding the conversion process and handling edge cases.
Basic atoi() Implementation
public static int atoi(String str) {
if (str == null || str.length() == 0) {
return 0;
}
int index = 0;
int result = 0;
int sign = 1;
// Handle whitespace
while (index < str.length() && Character.isWhitespace(str.charAt(index))) {
index++;
}
// Handle sign
if (index < str.length() && (str.charAt(index) == '+' || str.charAt(index) == '-')) {
sign = (str.charAt(index) == '+') ? 1 : -1;
index++;
}
// Convert digits
while (index < str.length() && Character.isDigit(str.charAt(index))) {
int digit = str.charAt(index) - '0';
// Check for overflow
if (result > Integer.MAX_VALUE / 10 || (result == Integer.MAX_VALUE / 10 && digit > Integer.MAX_VALUE % 10)) {
return (sign == 1) ? Integer.MAX_VALUE : Integer.MIN_VALUE;
}
result = result * 10 + digit;
index++;
}
return sign * result;
}
// Usage
System.out.println(atoi(" -42"));
System.out.println(atoi("4193 with words"));
System.out.println(atoi("-91283472332"));
Output:
-42
4193
-2147483648
This implementation handles several edge cases:
- Leading whitespace
- Positive and negative signs
- Overflow conditions
- Invalid characters after the number
6. Using Scanner Class
The Scanner class in Java provides a convenient way to parse various types of input, including integers. While it’s more commonly used for reading input from the console or files, it can also be used to parse strings.
Basic Usage of Scanner for String to Int Conversion
import java.util.Scanner;
public class ScannerExample {
public static void main(String[] args) {
String input = "123 456 789";
Scanner scanner = new Scanner(input);
while (scanner.hasNextInt()) {
int number = scanner.nextInt();
System.out.println("Scanned integer: " + number);
}
scanner.close();
}
}
Output:
Scanned integer: 123
Scanned integer: 456
Scanned integer: 789
Advantages of Using Scanner
- Can parse multiple integers from a single string
- Handles whitespace automatically
- Can be used to parse other data types as well (e.g., float, double)
Disadvantages of Using Scanner
- More overhead compared to parseInt() or valueOf() for simple conversions
- Requires proper resource management (closing the Scanner)
7. Using Character Class Methods
For more fine-grained control over the conversion process, you can use methods from the Character class to manually convert each digit in the string to its integer value.
Manual Conversion Using Character Methods
public static int stringToInt(String str) {
if (str == null || str.isEmpty()) {
throw new IllegalArgumentException("Input string is null or empty");
}
int result = 0;
boolean isNegative = false;
int i = 0;
// Handle sign
if (str.charAt(0) == '-') {
isNegative = true;
i = 1;
} else if (str.charAt(0) == '+') {
i = 1;
}
// Convert digits
for (; i < str.length(); i++) {
char c = str.charAt(i);
if (!Character.isDigit(c)) {
throw new NumberFormatException("Invalid character in input: " + c);
}
int digit = Character.getNumericValue(c);
result = result * 10 + digit;
}
return isNegative ? -result : result;
}
// Usage
System.out.println(stringToInt("-12345"));
System.out.println(stringToInt("+9876"));
System.out.println(stringToInt("42"));
Output:
-12345
9876
42
Advantages of Manual Conversion
- Complete control over the conversion process
- Can implement custom error handling and validation
- Useful for educational purposes or when working with non-standard number formats
Disadvantages of Manual Conversion
- More complex and error-prone than using built-in methods
- May be less efficient for simple use cases
- Requires careful implementation to handle all edge cases
8. Best Practices and Performance Considerations
When converting strings to integers in Java, it’s important to follow best practices and consider performance implications. Here are some guidelines to keep in mind:
1. Use the Right Method for Your Needs
- Use
Integer.parseInt()
for simple conversions to primitive int - Use
Integer.valueOf()
when you need an Integer object or are working with values likely to be in the cache range (-128 to 127) - Use custom implementations only when necessary for specific requirements
2. Handle Exceptions Properly
- Always catch and handle NumberFormatException when using parseInt() or valueOf()
- Consider creating utility methods that provide safe conversion with default values
3. Validate Input
- Check for null or empty strings before conversion
- Implement appropriate input validation based on your application’s requirements
4. Consider Performance
- For high-performance scenarios, benchmark different methods to find the best fit
- Be aware of autoboxing overhead when using Integer objects
- Use primitive ints when possible to avoid unnecessary object creation
5. Use Appropriate Radix
- Specify the correct radix when dealing with numbers in different bases
- Be cautious when parsing user input with different radixes
6. Handle Overflow
- Be aware of integer overflow when dealing with large numbers
- Consider using long or BigInteger for very large numbers
9. Common Pitfalls and How to Avoid Them
When converting strings to integers, there are several common pitfalls that developers may encounter. Being aware of these issues and knowing how to avoid them can help you write more robust and error-free code.
1. Ignoring NumberFormatException
Pitfall: Not catching NumberFormatException can lead to unexpected program termination.
Solution: Always handle NumberFormatException, either by catching it explicitly or by using a safe conversion method.
public static int safeParseInt(String str) {
try {
return Integer.parseInt(str);
} catch (NumberFormatException e) {
System.err.println("Invalid number format: " + str);
return 0; // or any other default value
}
}
2. Not Handling Null or Empty Strings
Pitfall: Attempting to parse null or empty strings will result in exceptions.
Solution: Check for null or empty strings before parsing.
public static int parseIntSafely(String str) {
if (str == null || str.isEmpty()) {
return 0; // or throw an exception, or return a special value
}
return Integer.parseInt(str);
}
3. Ignoring Leading/Trailing Whitespace
Pitfall: Extra whitespace can cause parsing errors.
Solution: Trim the input string before parsing.
String input = " 123 ";
int number = Integer.parseInt(input.trim());
4. Overlooking Locale-Specific Formatting
Pitfall: Different locales may use different number formats (e.g., commas as decimal separators).
Solution: Use NumberFormat for locale-aware parsing.
import java.text.NumberFormat;
import java.text.ParseException;
import java.util.Locale;
public static int parseLocaleInt(String str, Locale locale) throws ParseException {
NumberFormat format = NumberFormat.getInstance(locale);
return format.parse(str).intValue();
}
// Usage
int number = parseLocaleInt("1.234", Locale.GERMAN); // Parses as 1234
5. Not Considering Integer Overflow
Pitfall: Parsing very large numbers can lead to integer overflow.
Solution: Use long or BigInteger for large numbers, or implement overflow checking.
public static int parseIntWithOverflowCheck(String str) {
long result = Long.parseLong(str);
if (result < Integer.MIN_VALUE || result > Integer.MAX_VALUE) {
throw new ArithmeticException("Integer overflow");
}
return (int) result;
}
6. Incorrect Radix Usage
Pitfall: Using the wrong radix can lead to incorrect parsing.
Solution: Always specify the correct radix when parsing non-decimal numbers.
String binaryString = "1010";
int binaryNumber = Integer.parseInt(binaryString, 2);
String hexString = "1A";
int hexNumber = Integer.parseInt(hexString, 16);
10. Advanced Techniques and Edge Cases
While the basic methods for converting strings to integers cover most common scenarios, there are some advanced techniques and edge cases that you might encounter in more complex situations. Let’s explore some of these scenarios and how to handle them.
1. Parsing Numbers with Thousand Separators
When dealing with large numbers, you might encounter strings with thousand separators (e.g., “1,000,000”). Here’s how you can handle such cases:
import java.text.NumberFormat;
import java.text.ParseException;
import java.util.Locale;
public static int parseNumberWithSeparators(String str) throws ParseException {
NumberFormat format = NumberFormat.getInstance(Locale.US);
Number number = format.parse(str);
return number.intValue();
}
// Usage
String largeNumber = "1,234,567";
int result = parseNumberWithSeparators(largeNumber);
System.out.println("Parsed number: " + result);
2. Handling Scientific Notation
Sometimes, numbers might be represented in scientific notation (e.g., “1.23e4”). Here’s how to parse such strings:
public static int parseScientificNotation(String str) {
double doubleValue = Double.parseDouble(str);
return (int) doubleValue;
}
// Usage
String scientificNumber = "1.23e4";
int result = parseScientificNotation(scientificNumber);
System.out.println("Parsed scientific notation: " + result);
3. Custom Number Formats
For more complex number formats, you can use DecimalFormat with a custom pattern:
import java.text.DecimalFormat;
import java.text.ParseException;
public static int parseCustomFormat(String str, String pattern) throws ParseException {
DecimalFormat format = new DecimalFormat(pattern);
Number number = format.parse(str);
return number.intValue();
}
// Usage
String customNumber = "(12345)";
int result = parseCustomFormat(customNumber, "(#)");
System.out.println("Parsed custom format: " + result);
4. Handling Very Large Numbers
When dealing with numbers that might exceed the range of int, consider using BigInteger:
import java.math.BigInteger;
public static BigInteger parseLargeNumber(String str) {
return new BigInteger(str);
}
// Usage
String veryLargeNumber = "12345678901234567890";
BigInteger result = parseLargeNumber(veryLargeNumber);
System.out.println("Parsed large number: " + result);
5. Parsing Fractional Parts
If you need to parse strings that include fractional parts but only want the integer portion:
public static int parseIntegerPart(String str) {
double doubleValue = Double.parseDouble(str);
return (int) doubleValue;
}
// Usage
String fractionalNumber = "123.45";
int result = parseIntegerPart(fractionalNumber);
System.out.println("Parsed integer part: " + result);
6. Handling Different Bases Dynamically
For scenarios where you need to parse numbers in different bases dynamically:
public static int parseWithDynamicBase(String str) {
if (str.startsWith("0x") || str.startsWith("0X")) {
return Integer.parseInt(str.substring(2), 16);
} else if (str.startsWith("0b") || str.startsWith("0B")) {
return Integer.parseInt(str.substring(2), 2);
} else if (str.startsWith("0")) {
return Integer.parseInt(str, 8);
} else {
return Integer.parseInt(str);
}
}
// Usage
System.out.println(parseWithDynamicBase("123")); // Decimal
System.out.println(parseWithDynamicBase("0x1A")); // Hexadecimal
System.out.println(parseWithDynamicBase("0b1010")); // Binary
System.out.println(parseWithDynamicBase("0123")); // Octal
These advanced techniques cover a wide range of scenarios you might encounter when converting strings to integers in Java. By understanding these methods, you’ll be better equipped to handle complex parsing requirements in your applications.
11. Conclusion
Converting strings to integers is a fundamental operation in Java programming, and as we’ve seen, there are multiple ways to accomplish this task. From the simple and widely-used Integer.parseInt()
method to more advanced techniques for handling complex number formats, each approach has its own strengths and use cases.
Key takeaways from this guide include:
- Use
Integer.parseInt()
for simple, efficient conversions to primitive int. - Opt for
Integer.valueOf()
when you need an Integer object or are working with small numbers that benefit from caching. - Always handle exceptions, particularly NumberFormatException, to prevent unexpected program termination.
- Be aware of potential pitfalls like null inputs, whitespace, and integer overflow.
- For more complex scenarios, consider using NumberFormat, DecimalFormat, or custom parsing methods.
- When dealing with very large numbers or different number bases, use appropriate tools like BigInteger or specify the correct radix.
By mastering these techniques and understanding their implications, you’ll be well-equipped to handle string to integer conversions efficiently and robustly in your Java applications. Remember to always consider the specific requirements of your project, including performance needs, input formats, and potential edge cases, when choosing the most appropriate conversion method.
As you continue to develop your Java programming skills, keep exploring and practicing these conversion techniques. They are not only essential for basic data processing but also form the foundation for more advanced string and number manipulation tasks you’ll encounter in your programming journey.