String to Int in C++: A Comprehensive Guide
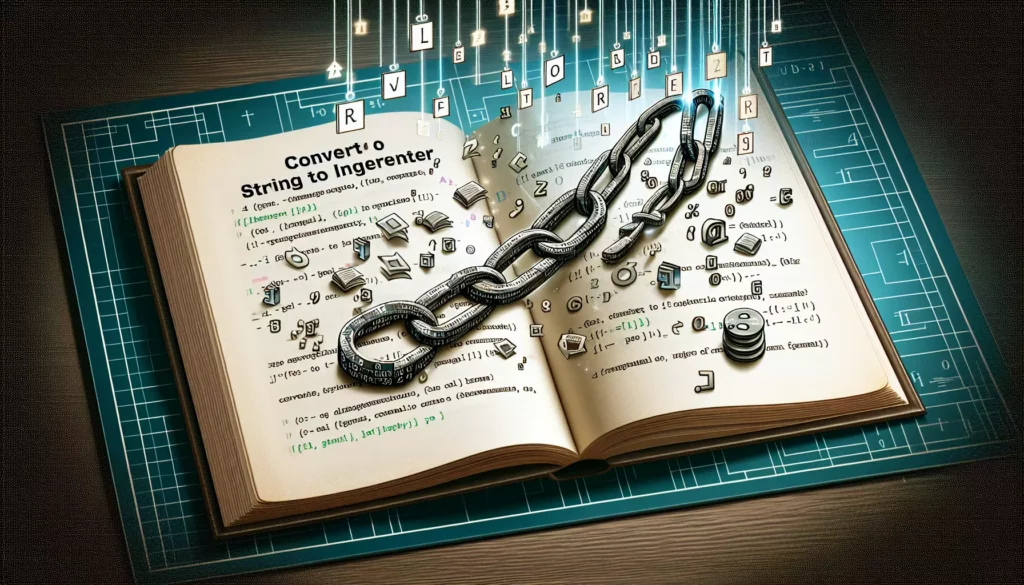
Converting a string to an integer is a common task in C++ programming. Whether you’re parsing user input, working with configuration files, or processing data from external sources, knowing how to efficiently and safely convert strings to integers is crucial. In this comprehensive guide, we’ll explore various methods to perform string to int conversions in C++, discuss their pros and cons, and provide practical examples to help you choose the best approach for your specific needs.
Table of Contents
- Introduction to String to Int Conversion
- Using the stoi() Function
- Using stringstream
- Using sscanf()
- Using atoi()
- Using std::from_chars()
- Custom Implementation
- Handling Errors and Edge Cases
- Performance Considerations
- Best Practices and Recommendations
- Conclusion
1. Introduction to String to Int Conversion
Converting a string to an integer is a process of parsing a sequence of characters representing a number and converting it into its corresponding integer value. This operation is essential in many programming scenarios, such as:
- Processing user input
- Reading data from files or databases
- Parsing command-line arguments
- Working with APIs that return numeric values as strings
C++ offers several built-in functions and methods to perform this conversion, each with its own advantages and limitations. Let’s explore these options in detail.
2. Using the stoi() Function
The stoi()
function, introduced in C++11, is a convenient and widely used method for converting strings to integers. It’s part of the <string>
header and provides a straightforward way to perform the conversion.
Syntax:
int stoi(const string& str, size_t* pos = nullptr, int base = 10);
Example:
#include <iostream>
#include <string>
int main() {
std::string str = "123";
int num = std::stoi(str);
std::cout << "Converted integer: " << num << std::endl;
return 0;
}
Advantages:
- Simple and easy to use
- Supports different bases (e.g., decimal, hexadecimal)
- Handles leading whitespace automatically
- Throws exceptions for invalid input
Disadvantages:
- Limited to 32-bit integers on most platforms
- May not be available in older C++ versions
3. Using stringstream
The std::stringstream
class, part of the <sstream>
header, provides a flexible way to convert strings to integers. It’s particularly useful when you need to perform multiple conversions or work with mixed data types.
Example:
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string str = "456";
std::stringstream ss(str);
int num;
if (ss >> num) {
std::cout << "Converted integer: " << num << std::endl;
} else {
std::cout << "Conversion failed" << std::endl;
}
return 0;
}
Advantages:
- Versatile and can handle multiple data types
- Provides error checking through the stream state
- Can be used for both input and output operations
Disadvantages:
- Slightly more verbose than other methods
- May have a performance overhead for simple conversions
4. Using sscanf()
The sscanf()
function, part of the C standard library, can be used to parse strings and convert them to integers. While it’s not a C++-specific function, it’s still widely used in C++ programs.
Syntax:
int sscanf(const char* str, const char* format, ...);
Example:
#include <iostream>
#include <cstdio>
int main() {
const char* str = "789";
int num;
if (sscanf(str, "%d", &num) == 1) {
std::cout << "Converted integer: " << num << std::endl;
} else {
std::cout << "Conversion failed" << std::endl;
}
return 0;
}
Advantages:
- Familiar to programmers with C background
- Can parse complex string formats
- Works with C-style strings
Disadvantages:
- Prone to buffer overflow if not used carefully
- Less type-safe compared to C++ alternatives
- Error handling is based on return values
5. Using atoi()
The atoi()
function is another C standard library function that can be used for string to integer conversion. It’s simple to use but has some limitations.
Syntax:
int atoi(const char* str);
Example:
#include <iostream>
#include <cstdlib>
int main() {
const char* str = "1234";
int num = atoi(str);
std::cout << "Converted integer: " << num << std::endl;
return 0;
}
Advantages:
- Simple and straightforward to use
- Available in both C and C++
- No exception handling required
Disadvantages:
- No error reporting for invalid input
- Undefined behavior for out-of-range values
- Only works with C-style strings
6. Using std::from_chars()
Introduced in C++17, std::from_chars()
is a modern, high-performance function for string to integer conversion. It’s part of the <charconv>
header and provides precise control over the conversion process.
Syntax:
from_chars_result from_chars(const char* first, const char* last, int& value, int base = 10);
Example:
#include <iostream>
#include <charconv>
#include <string>
int main() {
std::string str = "5678";
int num;
auto result = std::from_chars(str.data(), str.data() + str.size(), num);
if (result.ec == std::errc()) {
std::cout << "Converted integer: " << num << std::endl;
} else {
std::cout << "Conversion failed" << std::endl;
}
return 0;
}
Advantages:
- High performance and low overhead
- No dynamic memory allocation
- Precise control over the parsing process
- Supports different bases and error handling
Disadvantages:
- Requires C++17 or later
- Slightly more complex syntax compared to other methods
7. Custom Implementation
In some cases, you might want to implement your own string to integer conversion function. This can be useful for learning purposes or when you need fine-grained control over the conversion process.
Example:
#include <iostream>
#include <string>
int custom_stoi(const std::string& str) {
int result = 0;
int sign = 1;
size_t i = 0;
// Handle sign
if (str[0] == '-') {
sign = -1;
i = 1;
} else if (str[0] == '+') {
i = 1;
}
// Convert digits
for (; i < str.length(); ++i) {
if (str[i] >= '0' && str[i] <= '9') {
result = result * 10 + (str[i] - '0');
} else {
throw std::invalid_argument("Invalid input string");
}
}
return sign * result;
}
int main() {
std::string str = "-9876";
try {
int num = custom_stoi(str);
std::cout << "Converted integer: " << num << std::endl;
} catch (const std::exception& e) {
std::cout << "Error: " << e.what() << std::endl;
}
return 0;
}
Advantages:
- Complete control over the conversion process
- Can be tailored to specific requirements
- Educational value in understanding the conversion algorithm
Disadvantages:
- Requires careful implementation to handle all edge cases
- May be less efficient than built-in functions
- Needs thorough testing to ensure correctness
8. Handling Errors and Edge Cases
When converting strings to integers, it’s crucial to handle various error conditions and edge cases:
- Empty strings
- Non-numeric characters
- Overflow and underflow
- Leading and trailing whitespace
- Different number bases (e.g., hexadecimal)
Here’s an example of how to handle some of these cases using stoi()
:
#include <iostream>
#include <string>
#include <stdexcept>
#include <limits>
int safe_stoi(const std::string& str) {
try {
size_t pos;
int result = std::stoi(str, &pos);
// Check if the entire string was consumed
if (pos != str.length()) {
throw std::invalid_argument("Invalid characters in input");
}
return result;
} catch (const std::invalid_argument& e) {
std::cerr << "Invalid argument: " << e.what() << std::endl;
} catch (const std::out_of_range& e) {
std::cerr << "Out of range: " << e.what() << std::endl;
}
return 0; // Default value or error code
}
int main() {
std::cout << safe_stoi("123") << std::endl;
std::cout << safe_stoi("123abc") << std::endl;
std::cout << safe_stoi("9999999999999999999") << std::endl;
return 0;
}
9. Performance Considerations
When choosing a method for string to int conversion, consider the performance implications:
stoi()
andatoi()
are generally fast for simple conversions.stringstream
may have overhead for single conversions but can be efficient for multiple conversions.from_chars()
is designed for high performance and is often the fastest option in C++17 and later.- Custom implementations can be optimized for specific use cases but may not match the performance of well-optimized standard library functions.
Always profile your code with realistic data to determine the best method for your specific use case.
10. Best Practices and Recommendations
When working with string to int conversions in C++, consider the following best practices:
- Use
stoi()
orfrom_chars()
for most general-purpose conversions. - Prefer C++ standard library functions over C-style functions for better type safety and error handling.
- Always handle potential exceptions or error conditions.
- Be aware of the valid range for integers on your target platform.
- Use
stringstream
when working with mixed data types or multiple conversions. - Consider using
std::optional
(C++17) to represent conversion results that may or may not be valid. - Write unit tests to cover various input scenarios, including edge cases.
11. Conclusion
Converting strings to integers is a fundamental operation in C++ programming. This guide has explored various methods to perform this conversion, from simple functions like stoi()
to more advanced techniques like from_chars()
. Each method has its strengths and weaknesses, and the best choice depends on your specific requirements, performance needs, and the C++ version you’re working with.
Remember to always handle potential errors, consider edge cases, and choose the method that best fits your project’s needs. By mastering string to int conversion techniques, you’ll be better equipped to handle a wide range of programming challenges involving numeric data processing in C++.