String Compression and Encoding Algorithms: A Comprehensive Guide
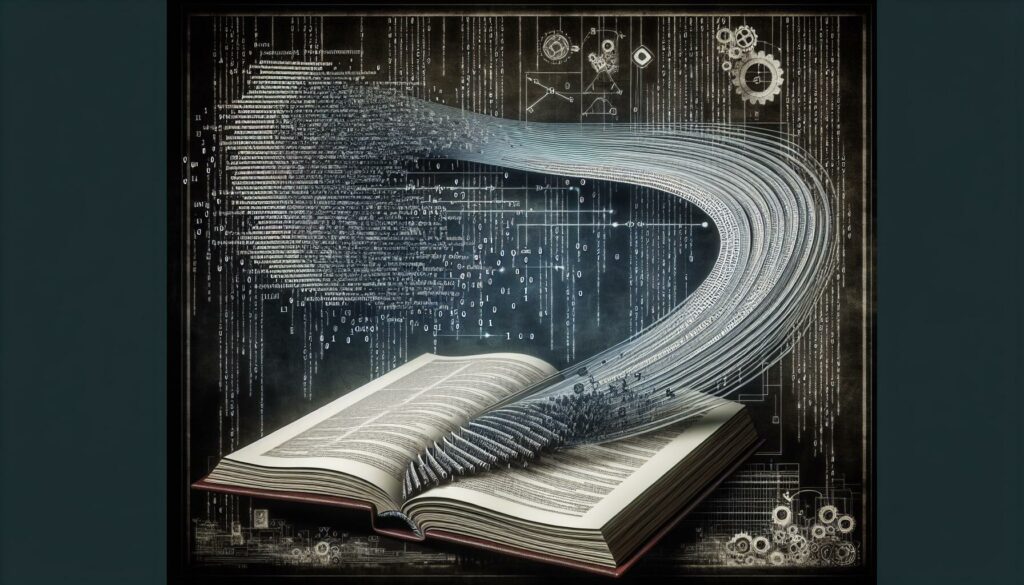
In the world of computer science and programming, efficient data storage and transmission are crucial. String compression and encoding algorithms play a vital role in achieving these goals by reducing the size of data without losing essential information. This comprehensive guide will dive deep into various string compression and encoding techniques, their implementations, and their applications in real-world scenarios.
1. Introduction to String Compression
String compression is the process of reducing the size of a string by encoding it in a more compact form. The primary goal is to represent the same information using fewer bits or characters. This technique is particularly useful when dealing with large amounts of text data or when bandwidth or storage is limited.
There are two main types of string compression:
- Lossless compression: This type of compression allows the original data to be perfectly reconstructed from the compressed data.
- Lossy compression: This type of compression reduces data size by eliminating some less critical information, resulting in a close approximation of the original data.
In this article, we’ll focus primarily on lossless compression techniques, as they are more commonly used in string compression scenarios.
2. Run-Length Encoding (RLE)
Run-Length Encoding is one of the simplest forms of data compression. It works by replacing consecutive occurrences of a character with a single instance of that character followed by the count of occurrences.
How RLE Works
For example, the string “AABBBCCCC” would be encoded as “2A3B4C”. This technique is particularly effective for strings with many repeated characters in sequence.
Implementation in Python
Here’s a simple implementation of Run-Length Encoding in Python:
def run_length_encode(string):
if not string:
return ""
result = []
count = 1
current_char = string[0]
for char in string[1:]:
if char == current_char:
count += 1
else:
result.append(f"{count}{current_char}")
current_char = char
count = 1
result.append(f"{count}{current_char}")
return "".join(result)
# Example usage
original = "AABBBCCCC"
compressed = run_length_encode(original)
print(f"Original: {original}")
print(f"Compressed: {compressed}")
This implementation will produce the following output:
Original: AABBBCCCC
Compressed: 2A3B4C
Advantages and Disadvantages of RLE
Advantages:
- Simple to implement and understand
- Fast encoding and decoding
- Effective for data with many repeated characters
Disadvantages:
- Can potentially increase the size of data with few repeated characters
- Not effective for complex or diverse data patterns
3. Huffman Coding
Huffman coding is a more sophisticated lossless data compression technique that assigns variable-length codes to characters based on their frequency of occurrence. More frequent characters are assigned shorter codes, while less frequent characters get longer codes.
How Huffman Coding Works
- Calculate the frequency of each character in the input string.
- Build a priority queue (min-heap) of nodes, where each node represents a character and its frequency.
- Repeatedly combine the two nodes with the lowest frequencies to create a new internal node until only one node remains (the root of the Huffman tree).
- Traverse the tree to assign binary codes to each character (0 for left branches, 1 for right branches).
- Use these codes to encode the original string.
Implementation in Python
Here’s a basic implementation of Huffman coding in Python:
import heapq
from collections import Counter
class HuffmanNode:
def __init__(self, char, freq):
self.char = char
self.freq = freq
self.left = None
self.right = None
def __lt__(self, other):
return self.freq < other.freq
def build_huffman_tree(string):
# Count frequency of each character
freq_dict = Counter(string)
# Create a priority queue of HuffmanNodes
pq = [HuffmanNode(char, freq) for char, freq in freq_dict.items()]
heapq.heapify(pq)
# Build the Huffman tree
while len(pq) > 1:
left = heapq.heappop(pq)
right = heapq.heappop(pq)
internal = HuffmanNode(None, left.freq + right.freq)
internal.left = left
internal.right = right
heapq.heappush(pq, internal)
return pq[0] # Return the root of the Huffman tree
def generate_huffman_codes(root):
codes = {}
def traverse(node, code):
if node.char:
codes[node.char] = code
return
traverse(node.left, code + "0")
traverse(node.right, code + "1")
traverse(root, "")
return codes
def huffman_encode(string):
root = build_huffman_tree(string)
codes = generate_huffman_codes(root)
return "".join(codes[char] for char in string), codes
# Example usage
original = "abracadabra"
encoded, codes = huffman_encode(original)
print(f"Original: {original}")
print(f"Encoded: {encoded}")
print("Huffman Codes:")
for char, code in codes.items():
print(f"{char}: {code}")
This implementation will produce output similar to:
Original: abracadabra
Encoded: 01011000110100101010110
Huffman Codes:
a: 0
b: 111
r: 10
c: 110
d: 101
Advantages and Disadvantages of Huffman Coding
Advantages:
- Provides optimal lossless compression for known character frequencies
- Adaptable to different types of data
- Can achieve significant compression ratios for suitable data
Disadvantages:
- More complex to implement than simpler methods like RLE
- Requires storing or transmitting the Huffman tree or codes along with the compressed data
- Less effective for small amounts of data or data with uniform character distributions
4. Lempel-Ziv-Welch (LZW) Compression
Lempel-Ziv-Welch (LZW) is a universal lossless compression algorithm that is particularly effective for text compression. It works by building a dictionary of substrings encountered in the input and replacing them with shorter codes.
How LZW Works
- Initialize a dictionary with single-character strings.
- Read input characters and build longer substrings.
- If a substring is not in the dictionary, add it and output the code for the previous substring.
- If a substring is in the dictionary, continue building a longer substring.
- Repeat until the entire input is processed.
Implementation in Python
Here’s a basic implementation of LZW compression in Python:
def lzw_compress(string):
dictionary = {chr(i): i for i in range(256)}
next_code = 256
result = []
current_string = ""
for char in string:
current_string += char
if current_string not in dictionary:
result.append(dictionary[current_string[:-1]])
dictionary[current_string] = next_code
next_code += 1
current_string = char
if current_string:
result.append(dictionary[current_string])
return result
def lzw_decompress(compressed):
dictionary = {i: chr(i) for i in range(256)}
next_code = 256
result = ""
current_string = chr(compressed[0])
result += current_string
for code in compressed[1:]:
if code in dictionary:
entry = dictionary[code]
elif code == next_code:
entry = current_string + current_string[0]
else:
raise ValueError("Invalid compressed data")
result += entry
dictionary[next_code] = current_string + entry[0]
next_code += 1
current_string = entry
return result
# Example usage
original = "TOBEORNOTTOBEORTOBEORNOT"
compressed = lzw_compress(original)
decompressed = lzw_decompress(compressed)
print(f"Original: {original}")
print(f"Compressed: {compressed}")
print(f"Decompressed: {decompressed}")
print(f"Compression ratio: {len(compressed) / len(original):.2f}")
This implementation will produce output similar to:
Original: TOBEORNOTTOBEORTOBEORNOT
Compressed: [84, 79, 66, 69, 79, 82, 78, 79, 84, 256, 258, 260, 265, 259, 261, 263]
Decompressed: TOBEORNOTTOBEORTOBEORNOT
Compression ratio: 0.64
Advantages and Disadvantages of LZW
Advantages:
- Adaptive compression that doesn’t require prior knowledge of the input
- Generally provides good compression ratios for text data
- Fast compression and decompression
Disadvantages:
- Can be less effective for small inputs or highly random data
- Dictionary size can grow large for complex inputs
- Patent issues (now expired) previously limited its use in some applications
5. Burrows-Wheeler Transform (BWT)
The Burrows-Wheeler Transform is not a compression algorithm itself, but a reversible transformation that can make data more compressible. It’s often used as a preprocessing step for other compression algorithms.
How BWT Works
- Append a unique end-of-string character to the input string.
- Generate all rotations of the string and sort them lexicographically.
- Extract the last column of the sorted rotations.
Implementation in Python
Here’s a basic implementation of the Burrows-Wheeler Transform in Python:
def bwt_encode(s):
# Append a unique end-of-string character
s = s + "$"
# Generate all rotations and sort them
rotations = sorted(s[i:] + s[:i] for i in range(len(s)))
# Return the last column
return "".join(rotation[-1] for rotation in rotations)
def bwt_decode(bwt):
# Create a list of (character, index) pairs
pairs = sorted((char, i) for i, char in enumerate(bwt))
# Initialize variables for decoding
result = []
current = 0
# Reconstruct the original string
for _ in range(len(bwt) - 1): # -1 because we don't include the $
char, current = pairs[current]
result.append(char)
return "".join(result)
# Example usage
original = "BANANA"
encoded = bwt_encode(original)
decoded = bwt_decode(encoded)
print(f"Original: {original}")
print(f"BWT Encoded: {encoded}")
print(f"Decoded: {decoded}")
This implementation will produce the following output:
Original: BANANA
BWT Encoded: BNN$AAA
Decoded: BANANA
Advantages and Disadvantages of BWT
Advantages:
- Can significantly improve compression ratios when used with other algorithms
- Particularly effective for text with repeated substrings
- Reversible transformation
Disadvantages:
- Not a compression algorithm on its own
- Can be computationally expensive for large inputs
- Requires additional processing steps in the compression pipeline
6. Applications of String Compression and Encoding
String compression and encoding algorithms have numerous practical applications across various domains:
6.1 Data Storage and Transmission
- Reducing file sizes for efficient storage and backup
- Minimizing bandwidth usage in network communications
- Improving load times for web content
6.2 Text Processing and Natural Language Processing (NLP)
- Efficient storage and processing of large text corpora
- Improved performance in text analysis and search algorithms
- Compact representation of language models
6.3 Bioinformatics
- Compressing and storing large genomic sequences
- Efficient comparison and analysis of DNA and protein sequences
- Reducing storage requirements for biological databases
6.4 Image and Multimedia Compression
- Compressing text-based metadata in image and video files
- Efficient storage of subtitles and closed captions
- Reducing size of text-based assets in games and multimedia applications
6.5 Data Encryption and Security
- Compressing data before encryption to reduce attack surface
- Implementing secure communication protocols with minimal overhead
- Efficient storage and transmission of encrypted data
7. Choosing the Right Compression Algorithm
Selecting the appropriate string compression or encoding algorithm depends on various factors:
- Data characteristics: Consider the type of data you’re working with (e.g., text, binary, repetitive patterns).
- Compression ratio: Evaluate the trade-off between compression effectiveness and computational complexity.
- Speed requirements: Consider the importance of fast compression and decompression times for your application.
- Memory constraints: Take into account the available memory for both compression and decompression processes.
- Lossless vs. lossy: Determine whether perfect reconstruction of the original data is necessary.
- Implementation complexity: Consider the development time and maintenance requirements for different algorithms.
- Compatibility: Ensure the chosen algorithm is compatible with your target systems and platforms.
8. Conclusion
String compression and encoding algorithms play a crucial role in modern computing, enabling efficient storage, transmission, and processing of data. From simple techniques like Run-Length Encoding to more sophisticated methods like Huffman coding and LZW compression, each algorithm offers unique advantages and trade-offs.
As a programmer or computer scientist, understanding these algorithms and their applications is essential for developing efficient and scalable systems. By mastering string compression techniques, you’ll be better equipped to tackle challenges related to data management, optimization, and algorithm design.
Remember that the field of data compression is constantly evolving, with new algorithms and techniques being developed to address emerging challenges. Stay curious and keep exploring new approaches to improve your skills in this fascinating area of computer science.
9. Further Reading and Resources
- “Introduction to Data Compression” by Khalid Sayood
- “Data Compression: The Complete Reference” by David Salomon
- “Compression Algorithms for Real Programmers” by Peter Wayner
- The zlib compression library documentation (https://zlib.net/)
- The Burrows-Wheeler Transform: Data Compression, Suffix Arrays, and Pattern Matching (https://www.cs.jhu.edu/~langmea/resources/bwt_and_fm_index.pdf)
- Online data compression resources and tools (https://compression.ru/index_en.htm)
By exploring these resources and implementing the algorithms discussed in this guide, you’ll develop a deeper understanding of string compression and encoding techniques, enabling you to create more efficient and powerful software solutions.