Strategies for Solving Word Search Problems: A Comprehensive Guide
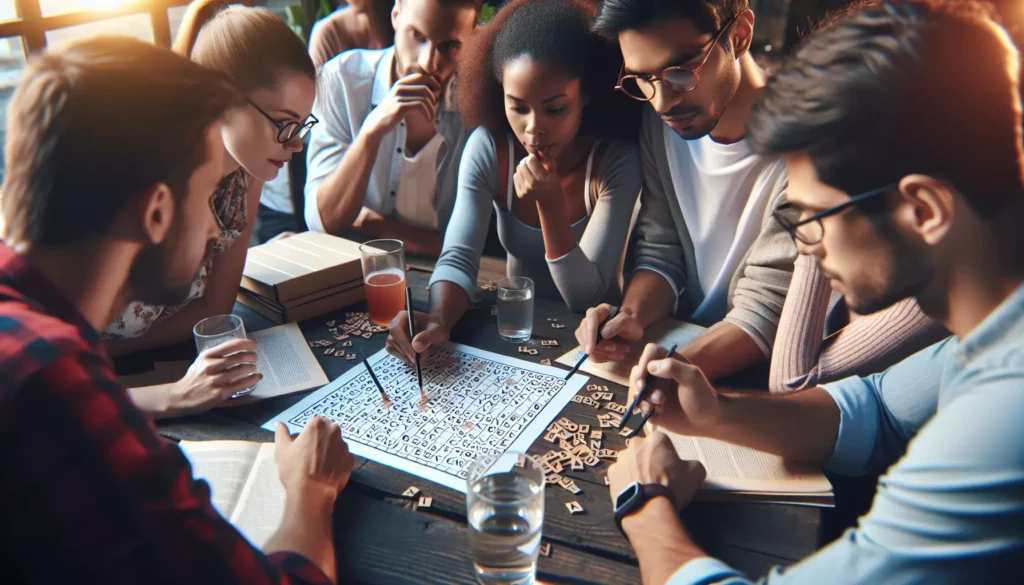
Word search problems are a common type of puzzle that appears in many programming interviews and coding challenges. These problems typically involve finding specific words within a grid of letters, and they can be an excellent way to test and improve your algorithmic thinking and problem-solving skills. In this comprehensive guide, we’ll explore various strategies and techniques for solving word search problems efficiently, along with practical implementations in popular programming languages.
Understanding Word Search Problems
Before diving into the strategies, let’s first understand what a typical word search problem looks like:
Given a 2D grid of characters and a list of words, your task is to find all the words in the grid. Words can be formed by connecting adjacent characters horizontally, vertically, or diagonally, and they may appear in any direction (forward, backward, up, down, or diagonally).
Here’s an example of a word search grid:
A B C D E
F G H I J
K L M N O
P Q R S T
U V W X Y
And a list of words to find: [“ABC”, “HIJ”, “KLM”, “UVW”, “AFK”, “EJO”, “EY”]
Basic Approach: Brute Force
The simplest approach to solving a word search problem is using a brute force method. This involves checking every possible direction from each cell in the grid for each word in the list. While this method is straightforward, it can be inefficient for large grids or long word lists.
Here’s a basic implementation of the brute force approach in Python:
def find_word(grid, word):
rows, cols = len(grid), len(grid[0])
directions = [(-1,-1),(-1,0),(-1,1),(0,-1),(0,1),(1,-1),(1,0),(1,1)]
def dfs(i, j, index):
if index == len(word):
return True
if (i < 0 or i >= rows or j < 0 or j >= cols or
grid[i][j] != word[index]):
return False
for di, dj in directions:
if dfs(i + di, j + dj, index + 1):
return True
return False
for i in range(rows):
for j in range(cols):
if dfs(i, j, 0):
return True
return False
def word_search(grid, words):
return [word for word in words if find_word(grid, word)]
# Example usage
grid = [
['A','B','C','D','E'],
['F','G','H','I','J'],
['K','L','M','N','O'],
['P','Q','R','S','T'],
['U','V','W','X','Y']
]
words = ["ABC", "HIJ", "KLM", "UVW", "AFK", "EJO", "EY"]
print(word_search(grid, words))
This approach works well for small grids and short word lists, but it can become slow for larger inputs. Let’s explore some more advanced strategies to optimize our solution.
Strategy 1: Trie Data Structure
One of the most effective strategies for solving word search problems efficiently is to use a Trie (prefix tree) data structure. A Trie allows us to quickly check if a sequence of characters is a prefix of any word in our list, which can significantly reduce the number of unnecessary searches.
Here’s how we can implement a Trie and use it to solve the word search problem:
class TrieNode:
def __init__(self):
self.children = {}
self.is_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_word = True
def word_search_trie(board, words):
trie = Trie()
for word in words:
trie.insert(word)
rows, cols = len(board), len(board[0])
result = set()
def dfs(i, j, node, path):
if node.is_word:
result.add(path)
if (i < 0 or i >= rows or j < 0 or j >= cols or
board[i][j] not in node.children):
return
char = board[i][j]
board[i][j] = '#' # Mark as visited
for di, dj in [(-1,0), (1,0), (0,-1), (0,1)]:
dfs(i+di, j+dj, node.children[char], path + char)
board[i][j] = char # Restore the character
for i in range(rows):
for j in range(cols):
dfs(i, j, trie.root, "")
return list(result)
# Example usage
board = [
['A','B','C','D','E'],
['F','G','H','I','J'],
['K','L','M','N','O'],
['P','Q','R','S','T'],
['U','V','W','X','Y']
]
words = ["ABC", "HIJ", "KLM", "UVW", "AFK", "EJO", "EY"]
print(word_search_trie(board, words))
Using a Trie allows us to efficiently check if a path in the grid is a prefix of any word we’re looking for. This can significantly reduce the search space and improve the overall performance of our algorithm.
Strategy 2: Directional DFS
Another strategy to optimize our word search solution is to use a directional depth-first search (DFS). Instead of checking all eight directions for each cell, we can perform four separate DFS searches in the horizontal, vertical, and two diagonal directions. This approach can be particularly effective when dealing with long words or when words are more likely to appear in specific directions.
Here’s an implementation of the directional DFS strategy:
def word_search_directional(board, words):
rows, cols = len(board), len(board[0])
result = set()
def dfs(i, j, word, index, di, dj):
if index == len(word):
result.add(word)
return
if (i < 0 or i >= rows or j < 0 or j >= cols or
board[i][j] != word[index]):
return
board[i][j] = '#' # Mark as visited
dfs(i+di, j+dj, word, index+1, di, dj)
board[i][j] = word[index] # Restore the character
directions = [(0,1), (1,0), (1,1), (1,-1)]
for word in words:
for i in range(rows):
for j in range(cols):
for di, dj in directions:
dfs(i, j, word, 0, di, dj)
dfs(i, j, word[::-1], 0, di, dj) # Check reverse word
return list(result)
# Example usage
board = [
['A','B','C','D','E'],
['F','G','H','I','J'],
['K','L','M','N','O'],
['P','Q','R','S','T'],
['U','V','W','X','Y']
]
words = ["ABC", "HIJ", "KLM", "UVW", "AFK", "EJO", "EY"]
print(word_search_directional(board, words))
This directional DFS approach can be particularly useful when you know that words are more likely to appear in certain directions or when dealing with very large grids where checking all eight directions for each cell would be too time-consuming.
Strategy 3: Bit Manipulation
For certain types of word search problems, especially those involving smaller grids or shorter words, we can use bit manipulation techniques to optimize our solution. This approach involves representing the grid and words as bit vectors, allowing for faster comparisons and pattern matching.
Here’s an example of how we can use bit manipulation to solve a simplified word search problem where we only need to find words in horizontal and vertical directions:
def word_search_bit(board, words):
rows, cols = len(board), len(board[0])
result = []
# Convert board to bit representation
bit_board = [[1 << (ord(c) - ord('A')) for c in row] for row in board]
# Convert words to bit representation
bit_words = {word: sum(1 << (ord(c) - ord('A')) for c in word) for word in words}
# Check horizontal words
for i in range(rows):
for j in range(cols - len(max(words, key=len)) + 1):
word_bits = sum(bit_board[i][j+k] for k in range(len(max(words, key=len))))
for word, bits in bit_words.items():
if bits == (word_bits & ((1 << len(word)*26) - 1)):
result.append(word)
# Check vertical words
for j in range(cols):
for i in range(rows - len(max(words, key=len)) + 1):
word_bits = sum(bit_board[i+k][j] for k in range(len(max(words, key=len))))
for word, bits in bit_words.items():
if bits == (word_bits & ((1 << len(word)*26) - 1)):
result.append(word)
return list(set(result))
# Example usage
board = [
['A','B','C','D','E'],
['F','G','H','I','J'],
['K','L','M','N','O'],
['P','Q','R','S','T'],
['U','V','W','X','Y']
]
words = ["ABC", "HIJ", "KLM", "UVW", "AFK", "EJO", "EY"]
print(word_search_bit(board, words))
While this bit manipulation approach may not be as versatile as the previous strategies, it can be extremely fast for certain types of word search problems, especially those with constraints on word directions or grid sizes.
Strategy 4: Suffix Arrays
For word search problems involving very large grids or when you need to perform multiple searches on the same grid, using suffix arrays can be an effective strategy. Suffix arrays allow for efficient string matching and can be particularly useful when combined with longest common prefix (LCP) arrays.
Here’s a basic implementation of a suffix array-based approach for word searching:
import functools
def build_suffix_array(s):
suffixes = sorted(range(len(s)), key=lambda i: s[i:])
return suffixes
def build_lcp_array(s, suffix_array):
n = len(s)
rank = [0] * n
for i in range(n):
rank[suffix_array[i]] = i
k = 0
lcp = [0] * (n - 1)
for i in range(n):
if rank[i] == n - 1:
k = 0
continue
j = suffix_array[rank[i] + 1]
while i + k < n and j + k < n and s[i+k] == s[j+k]:
k += 1
lcp[rank[i]] = k
if k > 0:
k -= 1
return lcp
def word_search_suffix_array(board, words):
# Flatten the board into a single string
s = '#'.join(''.join(row) for row in board) + '$'
suffix_array = build_suffix_array(s)
lcp_array = build_lcp_array(s, suffix_array)
result = []
for word in words:
# Binary search on suffix array
left, right = 0, len(s)
while left < right:
mid = (left + right) // 2
if s[suffix_array[mid]:] < word:
left = mid + 1
else:
right = mid
if s[suffix_array[left]:].startswith(word):
result.append(word)
return result
# Example usage
board = [
['A','B','C','D','E'],
['F','G','H','I','J'],
['K','L','M','N','O'],
['P','Q','R','S','T'],
['U','V','W','X','Y']
]
words = ["ABC", "HIJ", "KLM", "UVW", "AFK", "EJO", "EY"]
print(word_search_suffix_array(board, words))
This suffix array-based approach can be particularly effective for very large grids or when you need to perform multiple searches on the same grid. However, it’s worth noting that this implementation only finds words that appear consecutively in the flattened string representation of the grid. To find words in all directions, you would need to modify the approach to include additional string representations for different directions.
Optimizing for Different Scenarios
When solving word search problems, it’s important to consider the specific characteristics of your input data and requirements. Here are some tips for optimizing your solution based on different scenarios:
- Large grid, few words: Use the Trie-based approach for efficient prefix checking.
- Small grid, many words: The bit manipulation technique can be very fast for smaller grids.
- Words mostly in specific directions: The directional DFS approach can be more efficient.
- Very large grid with multiple searches: Consider using suffix arrays for efficient string matching.
- Memory constraints: The basic DFS approach uses the least additional memory but may be slower for large inputs.
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of the different approaches:
- Brute Force DFS:
- Time: O(m * n * 4^L), where m and n are the dimensions of the grid, and L is the maximum word length.
- Space: O(L) for the recursion stack.
- Trie-based approach:
- Time: O(m * n * 4^L), but often much faster in practice due to early termination.
- Space: O(N), where N is the total number of characters in all words.
- Directional DFS:
- Time: O(m * n * L * D), where D is the number of directions checked (usually 4 or 8).
- Space: O(L) for the recursion stack.
- Bit Manipulation:
- Time: O(m * n * W), where W is the number of words.
- Space: O(m * n) for the bit representation of the grid.
- Suffix Array:
- Time: O(m * n * log(m * n)) for construction, O(W * log(m * n)) for searching.
- Space: O(m * n) for the suffix and LCP arrays.
Conclusion
Solving word search problems efficiently requires a good understanding of various algorithmic techniques and data structures. By choosing the right strategy based on your specific input characteristics and requirements, you can significantly improve the performance of your solution.
Remember that the best approach often depends on the specific constraints of your problem. Don’t hesitate to experiment with different strategies and combinations to find the optimal solution for your particular word search challenge.
As you practice solving word search problems, you’ll develop a better intuition for which techniques to apply in different scenarios. This skill will not only help you in coding interviews but also in tackling real-world algorithmic challenges in your programming career.
Keep practicing, experimenting with different approaches, and analyzing the trade-offs between time and space complexity. With time and experience, you’ll become proficient at solving word search problems and similar algorithmic challenges efficiently and elegantly.