Strategies for Solving Word Break Problems: A Comprehensive Guide
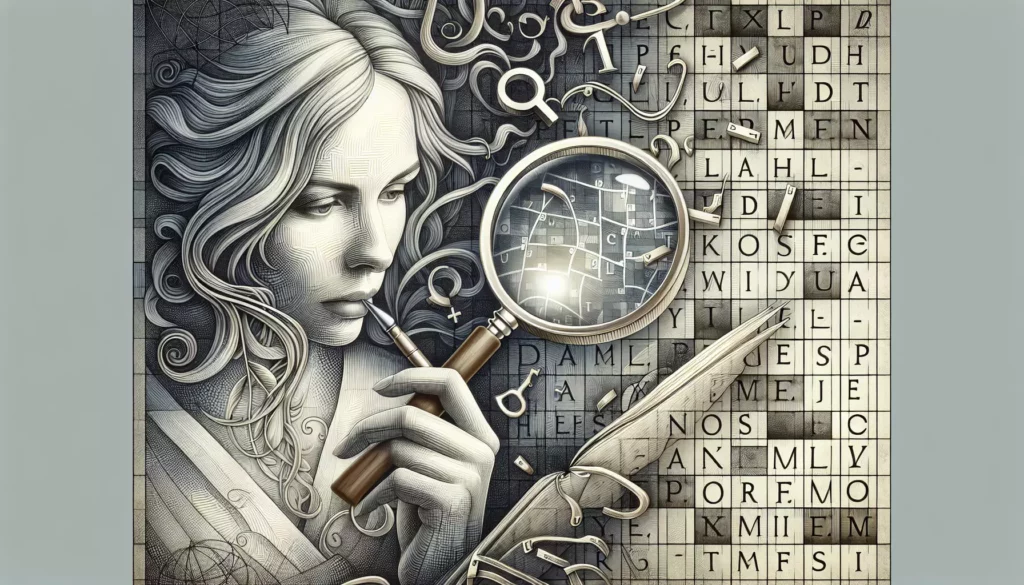
Word break problems are a common type of coding challenge that frequently appear in technical interviews, especially for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google). These problems test a candidate’s ability to think algorithmically and apply dynamic programming concepts. In this comprehensive guide, we’ll explore various strategies for solving word break problems, providing you with the tools you need to tackle these challenges confidently.
Understanding Word Break Problems
Before diving into the strategies, let’s first understand what word break problems are. In a typical word break problem, you’re given:
- A string
s
containing a sequence of characters without spaces - A dictionary of words
Your task is to determine whether the string can be segmented into a space-separated sequence of one or more dictionary words. In some variations, you might be asked to return all possible valid segmentations.
For example, given the string “leetcode” and a dictionary containing [“leet”, “code”], the answer would be true because “leetcode” can be segmented as “leet code”.
Strategy 1: Recursive Approach
The simplest approach to solving word break problems is using recursion. This method involves breaking down the problem into smaller subproblems and solving them recursively.
Algorithm:
- Start from the beginning of the string.
- Try all possible prefixes of the string that exist in the dictionary.
- If a prefix is found in the dictionary, recursively check if the remaining suffix can be segmented.
- If we reach the end of the string, return true.
Python Implementation:
def word_break(s, word_dict):
def can_break(start):
if start == len(s):
return True
for end in range(start + 1, len(s) + 1):
if s[start:end] in word_dict and can_break(end):
return True
return False
return can_break(0)
While this approach is intuitive, it has a time complexity of O(2^n) in the worst case, where n is the length of the string. This is because for each character, we have two choices: either to split at that character or not.
Strategy 2: Dynamic Programming
To optimize the recursive solution, we can use dynamic programming. This approach helps us avoid redundant computations by storing the results of subproblems.
Algorithm:
- Create a boolean array
dp
of lengthn+1
, wheren
is the length of the string. - Initialize
dp[0]
as true, representing an empty string. - Iterate through the string, for each index
i
: - Check all possible substrings ending at
i
. - If a substring is in the dictionary and the previous part of the string (before this substring) is breakable, mark
dp[i]
as true. - The final answer is stored in
dp[n]
.
Python Implementation:
def word_break_dp(s, word_dict):
n = len(s)
dp = [False] * (n + 1)
dp[0] = True
for i in range(1, n + 1):
for j in range(i):
if dp[j] and s[j:i] in word_dict:
dp[i] = True
break
return dp[n]
This dynamic programming solution has a time complexity of O(n^2 * m), where n is the length of the string and m is the maximum length of words in the dictionary. The space complexity is O(n).
Strategy 3: BFS (Breadth-First Search)
Another effective approach to solve word break problems is using Breadth-First Search (BFS). This method treats the problem as a graph traversal, where each index in the string is a node, and edges represent valid word breaks.
Algorithm:
- Initialize a queue with the starting index 0.
- While the queue is not empty:
- Dequeue an index.
- Try all possible words from this index.
- If a valid word is found, enqueue the end index of this word.
- If we reach the end of the string, return true.
- If we exhaust all possibilities without reaching the end, return false.
Python Implementation:
from collections import deque
def word_break_bfs(s, word_dict):
word_set = set(word_dict)
n = len(s)
queue = deque([0])
visited = set()
while queue:
start = queue.popleft()
if start == n:
return True
for end in range(start + 1, n + 1):
if end in visited:
continue
if s[start:end] in word_set:
queue.append(end)
visited.add(end)
return False
The BFS approach has a time complexity of O(n^2) and a space complexity of O(n), where n is the length of the string. This method can be particularly efficient for strings with many valid segmentations.
Strategy 4: Trie-based Approach
For cases where the dictionary is large, using a Trie (prefix tree) data structure can significantly improve the efficiency of word lookups.
Algorithm:
- Build a Trie from the dictionary words.
- Use either DP or BFS approach, but instead of checking if substrings exist in the dictionary, traverse the Trie.
Python Implementation:
class TrieNode:
def __init__(self):
self.children = {}
self.is_word = False
def build_trie(words):
root = TrieNode()
for word in words:
node = root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_word = True
return root
def word_break_trie(s, word_dict):
root = build_trie(word_dict)
n = len(s)
dp = [False] * (n + 1)
dp[0] = True
for i in range(1, n + 1):
node = root
for j in range(i - 1, -1, -1):
if s[j] not in node.children:
break
node = node.children[s[j]]
if node.is_word and dp[j]:
dp[i] = True
break
return dp[n]
The Trie-based approach can reduce the time complexity to O(n^2 * k), where k is the average length of words in the dictionary. This can be a significant improvement when dealing with a large dictionary.
Advanced Variations and Extensions
Word break problems can have several variations that test different aspects of problem-solving and algorithm design. Here are some common extensions:
1. Return All Possible Segmentations
Instead of just determining if a segmentation is possible, you might be asked to return all valid segmentations. This requires a backtracking approach combined with dynamic programming for efficiency.
Python Implementation:
def word_break_all(s, word_dict):
def backtrack(start):
if start == len(s):
return [[]]
results = []
for end in range(start + 1, len(s) + 1):
word = s[start:end]
if word in word_dict:
sub_results = backtrack(end)
for sub_result in sub_results:
results.append([word] + sub_result)
return results
return backtrack(0)
# Example usage
s = "catsanddog"
word_dict = ["cat", "cats", "and", "sand", "dog"]
print(word_break_all(s, word_dict))
# Output: [['cat', 'sand', 'dog'], ['cats', 'and', 'dog']]
2. Minimum Number of Segmentations
This variation asks for the minimum number of words needed to segment the string. It can be solved using dynamic programming with a slight modification to our earlier DP approach.
Python Implementation:
def min_word_break(s, word_dict):
n = len(s)
dp = [float('inf')] * (n + 1)
dp[0] = 0
for i in range(1, n + 1):
for j in range(i):
if s[j:i] in word_dict:
dp[i] = min(dp[i], dp[j] + 1)
return dp[n] if dp[n] != float('inf') else -1
# Example usage
s = "leetcode"
word_dict = ["leet", "code", "lee", "t"]
print(min_word_break(s, word_dict)) # Output: 2
3. Word Break with Wildcards
In this challenging variation, the dictionary words may contain wildcards (e.g., ‘?’ representing any single character). This requires modifying our word matching logic to handle wildcards.
Python Implementation:
def is_match(word, pattern):
if len(word) != len(pattern):
return False
for w, p in zip(word, pattern):
if p != '?' and w != p:
return False
return True
def word_break_wildcard(s, word_dict):
n = len(s)
dp = [False] * (n + 1)
dp[0] = True
for i in range(1, n + 1):
for j in range(i):
if dp[j]:
for word in word_dict:
if is_match(s[j:i], word):
dp[i] = True
break
if dp[i]:
break
return dp[n]
# Example usage
s = "catcog"
word_dict = ["cat", "c?g", "do?"]
print(word_break_wildcard(s, word_dict)) # Output: True
Performance Optimization Tips
When dealing with word break problems, especially in a coding interview setting, consider these optimization tips:
- Use Sets for Dictionary Lookup: Convert the word dictionary to a set for O(1) lookup time.
- Memoization in Recursive Approaches: If using recursion, implement memoization to avoid redundant computations.
- Early Termination: In DP and BFS approaches, return early if a solution is found to avoid unnecessary computations.
- Trie for Large Dictionaries: For very large dictionaries, consider using a Trie data structure for efficient prefix matching.
- Length-based Pruning: Before attempting to match a substring, check if its length is within the range of dictionary word lengths.
Common Pitfalls and How to Avoid Them
When solving word break problems, be aware of these common pitfalls:
- Overlooking Empty Strings: Always consider the case of an empty string in your solution.
- Ignoring Time Complexity: The naive recursive solution can be extremely slow for long strings. Always analyze and optimize your solution’s time complexity.
- Forgetting to Handle Edge Cases: Consider scenarios like all characters being the same, or the dictionary containing only very short or very long words.
- Inefficient String Operations: In languages like Java, repeated string concatenation can be slow. Consider using StringBuilder or similar efficient string manipulation techniques.
- Not Considering Space Complexity: While focusing on time optimization, don’t neglect space complexity, especially for large inputs.
Conclusion
Word break problems are a fascinating class of algorithmic challenges that test a wide range of problem-solving skills. From recursive thinking to dynamic programming, from graph traversal to advanced data structures like Tries, these problems offer a comprehensive workout for your coding muscles.
As you practice these problems, remember that the key to mastery lies not just in solving them, but in understanding the underlying patterns and principles. Each strategy we’ve discussed – recursion, dynamic programming, BFS, and Trie-based approaches – has its strengths and ideal use cases. By familiarizing yourself with these approaches and their variations, you’ll be well-equipped to tackle not just word break problems, but a wide array of string and dynamic programming challenges in your coding interviews and beyond.
Keep practicing, analyzing different approaches, and most importantly, enjoy the process of problem-solving. With dedication and the right strategies, you’ll find yourself breaking down these word break problems with ease and confidence!