Strategies for Solving Recursive Problems: Mastering the Art of Self-Referential Algorithms
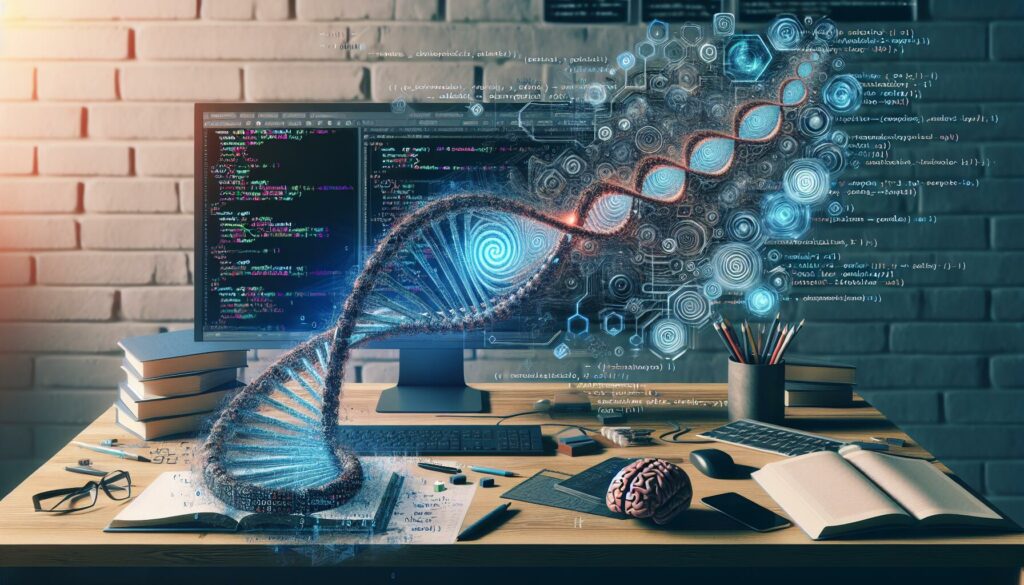
Recursion is a powerful programming concept that allows developers to solve complex problems by breaking them down into smaller, more manageable sub-problems. It’s a fundamental technique in computer science and a crucial skill for aspiring programmers, especially those aiming to excel in technical interviews at top tech companies. In this comprehensive guide, we’ll explore various strategies for solving recursive problems, providing you with the tools and mindset needed to tackle even the most challenging algorithmic puzzles.
Understanding Recursion: The Foundation
Before diving into specific strategies, it’s essential to have a solid grasp of what recursion is and how it works. At its core, recursion is a method where a function calls itself to solve a problem. This self-referential nature allows for elegant solutions to problems that have a recursive structure.
Key components of a recursive function include:
- Base case: The condition that stops the recursion
- Recursive case: The part where the function calls itself
- Progress towards the base case: Ensuring the problem gets smaller with each recursive call
Understanding these components is crucial for implementing effective recursive solutions. Let’s look at a simple example to illustrate these concepts:
def factorial(n):
# Base case
if n == 0 or n == 1:
return 1
# Recursive case
else:
return n * factorial(n - 1) # Progress towards base case
In this factorial function, the base case is when n is 0 or 1, and the recursive case multiplies n by the factorial of n-1, making progress towards the base case with each call.
Strategy 1: Identify the Base Case
The first and most crucial step in solving any recursive problem is identifying the base case. This is the simplest scenario where the problem can be solved directly without further recursion. Failing to define a proper base case can lead to infinite recursion and stack overflow errors.
Tips for identifying base cases:
- Look for the smallest possible input that has a trivial solution
- Consider edge cases or boundary conditions
- Ensure the base case is reachable through the recursive process
Example: Finding the length of a linked list recursively
def length(head):
# Base case: empty list
if head is None:
return 0
# Recursive case
return 1 + length(head.next)
Here, the base case is an empty list (None), which has a length of 0.
Strategy 2: Define the Recursive Case
Once you’ve identified the base case, the next step is to define how the problem can be broken down into smaller sub-problems. This is where the recursive case comes into play. The key is to express the solution to the current problem in terms of solutions to smaller instances of the same problem.
Tips for defining recursive cases:
- Identify how the problem can be divided into smaller, similar sub-problems
- Ensure that each recursive call moves closer to the base case
- Keep the recursive logic simple and focused on a single step
Example: Reversing a string recursively
def reverse_string(s):
# Base case: empty string or single character
if len(s) <= 1:
return s
# Recursive case
return reverse_string(s[1:]) + s[0]
In this example, the recursive case reverses the substring starting from the second character and then appends the first character to the end.
Strategy 3: Visualize the Recursion Tree
Visualizing the recursion tree can be immensely helpful in understanding how a recursive algorithm works and in identifying potential optimizations. A recursion tree represents all the recursive calls made during the execution of the algorithm, with each node representing a function call and its parameters.
Steps to visualize a recursion tree:
- Start with the initial function call as the root of the tree
- For each recursive call, create a child node
- Continue this process until you reach the base cases
- Analyze the tree structure to understand the flow and potential redundancies
Example: Fibonacci sequence recursion tree
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
Visualizing the recursion tree for fibonacci(5) would show:
fib(5)
/ \
fib(4) fib(3)
/ \ / \
fib(3) fib(2) fib(2) fib(1)
/ \ / \
fib(2) fib(1) fib(1) fib(0)
/ \
fib(1) fib(0)
This visualization helps identify overlapping subproblems, which can lead to optimizations like memoization or dynamic programming.
Strategy 4: Use Helper Functions
Sometimes, the recursive solution becomes cleaner and more efficient by using a helper function. This strategy is particularly useful when you need to maintain additional state or when the original function signature doesn’t provide enough flexibility for the recursive implementation.
Benefits of helper functions:
- Keep the main function’s interface simple
- Allow for additional parameters to track state
- Facilitate tail recursion optimization in some cases
Example: Binary tree inorder traversal
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorderTraversal(root):
def inorder_helper(node, result):
if node:
inorder_helper(node.left, result)
result.append(node.val)
inorder_helper(node.right, result)
result = []
inorder_helper(root, result)
return result
Here, the helper function allows us to accumulate the result without modifying the original function signature.
Strategy 5: Implement Tail Recursion
Tail recursion is a special form of recursion where the recursive call is the last operation in the function. This form of recursion can be optimized by many compilers to use constant stack space, effectively turning the recursion into iteration.
Benefits of tail recursion:
- Potential for compiler optimization
- Reduced risk of stack overflow for deep recursions
- Often leads to clearer and more efficient code
Example: Factorial calculation with tail recursion
def factorial_tail(n, accumulator=1):
if n == 0:
return accumulator
return factorial_tail(n - 1, n * accumulator)
In this version, the recursive call is the last operation, and the accumulator parameter carries the intermediate result.
Strategy 6: Memoization for Overlapping Subproblems
When a recursive problem involves solving the same subproblems multiple times, memoization can significantly improve performance. Memoization involves caching the results of expensive function calls and returning the cached result when the same inputs occur again.
Steps to implement memoization:
- Create a cache (usually a dictionary) to store results
- Before computing, check if the result is already in the cache
- If not in the cache, compute the result and store it in the cache
- Return the cached result
Example: Fibonacci sequence with memoization
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
return memo[n]
This memoized version of the Fibonacci function dramatically reduces the time complexity from O(2^n) to O(n).
Strategy 7: Divide and Conquer
The divide and conquer strategy is a powerful approach for solving recursive problems. It involves breaking down a problem into two or more sub-problems of the same or related type, solving these sub-problems recursively, and then combining their results to solve the original problem.
Steps in the divide and conquer approach:
- Divide: Break the problem into smaller sub-problems
- Conquer: Recursively solve the sub-problems
- Combine: Merge the solutions of the sub-problems to create a solution to the original problem
Example: Merge Sort algorithm
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
This implementation of merge sort demonstrates the divide and conquer strategy by recursively sorting the left and right halves of the array and then merging them.
Strategy 8: Recursive Backtracking
Recursive backtracking is a technique used to build a solution incrementally, abandoning solutions that fail to meet the problem’s constraints. This strategy is particularly useful for solving combinatorial problems, such as finding all possible configurations or paths.
Steps in recursive backtracking:
- Choose: Select a candidate for the solution
- Constraint: Check if the candidate violates any constraints
- Goal: Check if the candidate solution solves the problem
- Recurse: Recursively apply the algorithm to build the rest of the solution
- Unchoose: If the recursion fails, undo the choice and try another candidate
Example: Generating all permutations of a string
def generate_permutations(s):
def backtrack(start):
if start == len(s):
result.append(''.join(s))
for i in range(start, len(s)):
s[start], s[i] = s[i], s[start] # Choose
backtrack(start + 1) # Recurse
s[start], s[i] = s[i], s[start] # Unchoose
result = []
backtrack(0)
return result
# Usage
s = list("abc")
permutations = generate_permutations(s)
print(permutations)
This algorithm generates all permutations of a given string by recursively swapping characters and backtracking when necessary.
Strategy 9: Recursion with Multiple Base Cases
Some problems require multiple base cases to cover all possible scenarios. Recognizing when multiple base cases are needed and implementing them correctly is crucial for solving more complex recursive problems.
Tips for handling multiple base cases:
- Identify all the simplest scenarios that don’t require further recursion
- Ensure that all base cases are mutually exclusive
- Order the base cases from most specific to most general
Example: Climbing stairs problem (Leetcode #70)
def climbStairs(n):
if n == 0:
return 1 # Base case 1: There's one way to climb 0 stairs
if n == 1:
return 1 # Base case 2: There's one way to climb 1 stair
return climbStairs(n-1) + climbStairs(n-2)
This problem requires two base cases: one for 0 stairs and another for 1 stair. The recursive case combines the solutions for climbing n-1 and n-2 stairs.
Strategy 10: Thinking Recursively
Perhaps the most important strategy is developing the ability to think recursively. This involves training your mind to see problems in terms of their recursive structure and to approach problem-solving with a recursive mindset.
Tips for developing recursive thinking:
- Practice breaking down problems into smaller, similar sub-problems
- Look for patterns and self-similarity in problem structures
- Start with the simplest case and build up to more complex scenarios
- Visualize the problem-solving process as a tree of function calls
- Trust the recursive process and focus on solving one level at a time
Example: Thinking recursively about the Tower of Hanoi problem
def tower_of_hanoi(n, source, auxiliary, destination):
if n == 1:
print(f"Move disk 1 from {source} to {destination}")
return
tower_of_hanoi(n-1, source, destination, auxiliary)
print(f"Move disk {n} from {source} to {destination}")
tower_of_hanoi(n-1, auxiliary, source, destination)
# Usage
tower_of_hanoi(3, 'A', 'B', 'C')
This classic problem demonstrates recursive thinking by breaking down the movement of n disks into moving n-1 disks, then the largest disk, and then n-1 disks again.
Conclusion: Mastering Recursive Problem-Solving
Recursion is a powerful tool in a programmer’s arsenal, enabling elegant solutions to complex problems. By mastering these strategies for solving recursive problems, you’ll be well-equipped to tackle a wide range of algorithmic challenges, from basic coding exercises to advanced technical interview questions at top tech companies.
Remember that becoming proficient with recursion takes practice. As you work through various problems, you’ll develop an intuition for when and how to apply recursive solutions effectively. Keep these strategies in mind, and don’t hesitate to revisit and refine your approach as you gain more experience.
Recursive problem-solving is not just about writing code; it’s about developing a mindset that allows you to see the inherent structure in complex problems. With persistence and practice, you’ll find that many seemingly intricate problems can be elegantly solved using the power of recursion.
As you continue your journey in programming and prepare for technical interviews, make recursive problem-solving a core part of your skill set. It will not only help you solve specific types of problems more efficiently but also enhance your overall algorithmic thinking and problem-solving abilities.
Happy coding, and may your recursive adventures be stack-overflow-free!