Strategies for Solving Path Sum Problems in Binary Trees
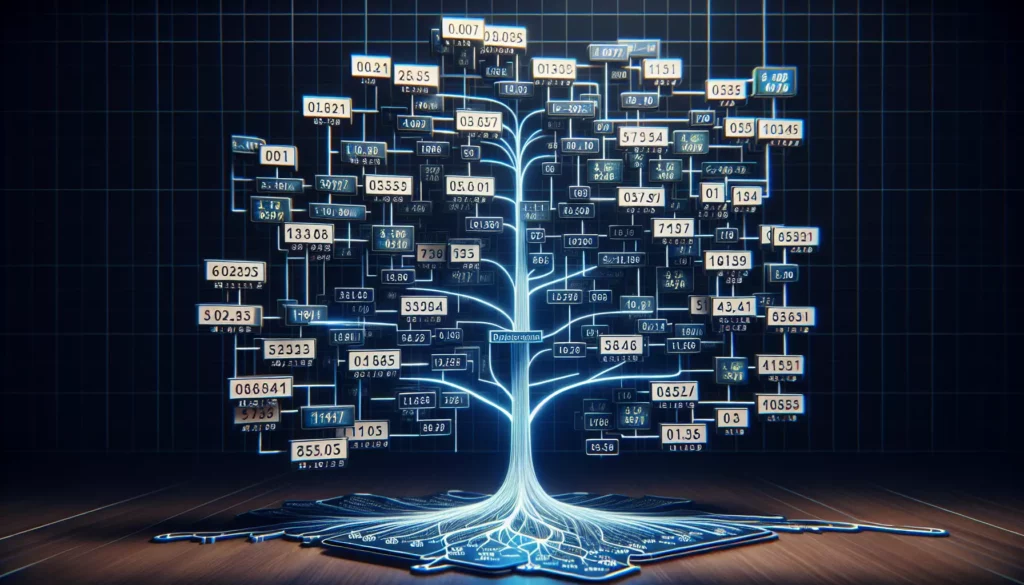
Path sum problems are a common category of coding challenges that frequently appear in technical interviews, especially for positions at major tech companies. These problems typically involve traversing a binary tree and finding paths that satisfy certain sum conditions. In this comprehensive guide, we’ll explore various strategies for solving path sum problems, providing you with the tools and techniques to tackle these challenges confidently.
Understanding Path Sum Problems
Before diving into the strategies, let’s first understand what path sum problems are and why they’re important in the context of coding interviews and algorithmic thinking.
What are Path Sum Problems?
Path sum problems involve binary trees and typically ask you to find paths from the root to a leaf (or sometimes between any two nodes) where the sum of node values along the path meets a specific criterion. Common variations include:
- Finding if a path exists with a given sum
- Counting the number of paths with a given sum
- Finding all paths with a given sum
- Finding the maximum path sum
Why are Path Sum Problems Important?
Path sum problems are significant for several reasons:
- Tree Traversal: They test your ability to navigate and process tree structures, a fundamental skill in computer science.
- Recursion: Many solutions involve recursive approaches, demonstrating your understanding of this powerful problem-solving technique.
- Problem-Solving Skills: These problems often require you to think creatively and consider multiple approaches.
- Interview Relevance: They are frequently asked in technical interviews, especially at FAANG (Facebook, Amazon, Apple, Netflix, Google) companies.
Basic Concepts and Data Structures
Before we delve into specific strategies, let’s review some fundamental concepts and data structures that are crucial for solving path sum problems.
Binary Tree Structure
A binary tree is a tree data structure where each node has at most two children, referred to as the left child and the right child. In many programming languages, a binary tree node can be represented as follows:
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int val) {
this.val = val;
this.left = null;
this.right = null;
}
}
Depth-First Search (DFS)
DFS is a common traversal method used in many path sum solutions. It explores as far as possible along each branch before backtracking. There are three main types of DFS traversals:
- Pre-order: Root, Left, Right
- In-order: Left, Root, Right
- Post-order: Left, Right, Root
Breadth-First Search (BFS)
BFS explores all the neighbor nodes at the present depth before moving to nodes at the next depth level. While less common in path sum problems, BFS can be useful in certain scenarios.
Strategy 1: Recursive DFS Approach
The recursive DFS approach is often the most intuitive and commonly used strategy for solving path sum problems. Let’s explore this strategy with a classic problem: determining if a path exists from root to leaf with a given sum.
Problem: Path Sum
Given the root of a binary tree and an integer targetSum, return true if the tree has a root-to-leaf path such that adding up all the values along the path equals targetSum. A leaf is a node with no children.
Solution:
public boolean hasPathSum(TreeNode root, int targetSum) {
// Base case: if the root is null, return false
if (root == null) {
return false;
}
// If it's a leaf node and its value equals the remaining sum, we found a valid path
if (root.left == null && root.right == null && targetSum - root.val == 0) {
return true;
}
// Recursively check the left and right subtrees
return hasPathSum(root.left, targetSum - root.val) ||
hasPathSum(root.right, targetSum - root.val);
}
Explanation:
- We start by checking if the root is null. If so, there can’t be a path, so we return false.
- If we’re at a leaf node (both left and right children are null) and the remaining sum equals the node’s value, we’ve found a valid path.
- If neither of the above conditions is met, we recursively check the left and right subtrees, subtracting the current node’s value from the target sum.
This recursive approach effectively explores all possible paths from root to leaf, making it an elegant solution for this problem.
Strategy 2: Iterative DFS Using a Stack
While the recursive approach is clean and intuitive, it can lead to stack overflow for very deep trees. An iterative approach using a stack can solve this issue and is often preferred in production code.
Problem: Path Sum II
Given the root of a binary tree and an integer targetSum, return all root-to-leaf paths where the sum of the node values in the path equals targetSum. Each path should be returned as a list of the node values, not node references.
Solution:
public List<List<Integer>> pathSum(TreeNode root, int targetSum) {
List<List<Integer>> result = new ArrayList<>();
if (root == null) {
return result;
}
Stack<TreeNode> nodeStack = new Stack<>();
Stack<Integer> sumStack = new Stack<>();
Stack<List<Integer>> pathStack = new Stack<>();
nodeStack.push(root);
sumStack.push(targetSum - root.val);
pathStack.push(new ArrayList<>(Arrays.asList(root.val)));
while (!nodeStack.isEmpty()) {
TreeNode currentNode = nodeStack.pop();
int currentSum = sumStack.pop();
List<Integer> currentPath = pathStack.pop();
if (currentNode.left == null && currentNode.right == null && currentSum == 0) {
result.add(new ArrayList<>(currentPath));
}
if (currentNode.right != null) {
nodeStack.push(currentNode.right);
sumStack.push(currentSum - currentNode.right.val);
List<Integer> newPath = new ArrayList<>(currentPath);
newPath.add(currentNode.right.val);
pathStack.push(newPath);
}
if (currentNode.left != null) {
nodeStack.push(currentNode.left);
sumStack.push(currentSum - currentNode.left.val);
List<Integer> newPath = new ArrayList<>(currentPath);
newPath.add(currentNode.left.val);
pathStack.push(newPath);
}
}
return result;
}
Explanation:
- We use three stacks: one for nodes, one for the remaining sum, and one for the current path.
- We start by pushing the root node, the initial target sum minus the root’s value, and a path containing just the root’s value.
- In each iteration, we pop a node, its corresponding remaining sum, and the path to that node.
- If it’s a leaf node and the remaining sum is zero, we’ve found a valid path and add it to the result.
- We then push the right and left children (if they exist) onto the stacks, along with updated sums and paths.
- This process continues until we’ve explored all paths.
This iterative approach can handle deeper trees without the risk of stack overflow, making it more robust for larger inputs.
Strategy 3: Prefix Sum Technique
The prefix sum technique is particularly useful when dealing with path sum problems that don’t necessarily start at the root or end at a leaf. It’s based on the idea that if the sum from the root to node A is S1, and the sum from the root to node B is S2, then the sum of the path from A to B is S2 – S1.
Problem: Path Sum III
Given the root of a binary tree and an integer targetSum, return the number of paths where the sum of the values along the path equals targetSum. The path does not need to start or end at the root or a leaf, but it must go downwards (i.e., traveling only from parent nodes to child nodes).
Solution:
class Solution {
int count = 0;
Map<Long, Integer> prefixSumCount = new HashMap<>();
public int pathSum(TreeNode root, int targetSum) {
prefixSumCount.put(0L, 1);
dfs(root, 0, targetSum);
return count;
}
private void dfs(TreeNode node, long currentSum, int targetSum) {
if (node == null) {
return;
}
currentSum += node.val;
count += prefixSumCount.getOrDefault(currentSum - targetSum, 0);
prefixSumCount.put(currentSum, prefixSumCount.getOrDefault(currentSum, 0) + 1);
dfs(node.left, currentSum, targetSum);
dfs(node.right, currentSum, targetSum);
prefixSumCount.put(currentSum, prefixSumCount.get(currentSum) - 1);
}
}
Explanation:
- We use a HashMap to store the count of each prefix sum encountered.
- We initialize the map with a count of 1 for sum 0 (representing an empty path).
- As we traverse the tree, we keep track of the current sum from the root to the current node.
- At each node, we check if there’s a prefix sum that, when subtracted from the current sum, equals the target sum. If so, we’ve found path(s) that sum to the target.
- We update the count of the current prefix sum in the map.
- After exploring a node’s subtrees, we decrement the count of its prefix sum (backtracking).
This technique allows us to find paths that don’t necessarily start at the root, making it more versatile than the previous strategies.
Strategy 4: Dynamic Programming Approach
While not as common for tree-based path sum problems, dynamic programming can be useful for certain variations, especially when dealing with grid-based path sums or when optimization is required.
Problem: Maximum Path Sum
Given a binary tree, find the maximum path sum. The path may start and end at any node in the tree.
Solution:
class Solution {
int maxSum = Integer.MIN_VALUE;
public int maxPathSum(TreeNode root) {
maxGain(root);
return maxSum;
}
private int maxGain(TreeNode node) {
if (node == null) {
return 0;
}
int leftGain = Math.max(maxGain(node.left), 0);
int rightGain = Math.max(maxGain(node.right), 0);
int priceNewPath = node.val + leftGain + rightGain;
maxSum = Math.max(maxSum, priceNewPath);
return node.val + Math.max(leftGain, rightGain);
}
}
Explanation:
- We use a recursive helper function maxGain that computes the maximum gain one can obtain from a subtree.
- For each node, we calculate the maximum gain from its left and right subtrees.
- We then compute the value of a new path passing through the node, which is the sum of the node’s value and the gains from its left and right subtrees.
- We update the global maximum sum if this new path sum is greater.
- Finally, we return the maximum gain obtainable from this node, which is its value plus the maximum of the left or right gain.
This approach effectively uses dynamic programming principles by building solutions for larger subtrees from solutions of smaller subtrees.
Advanced Techniques and Optimizations
As you become more comfortable with basic path sum problems, you may encounter more complex variations that require advanced techniques or optimizations. Here are a few to consider:
1. Using Bitwise Operations
In some cases, especially when dealing with binary trees where node values are limited to 0 and 1, bitwise operations can lead to more efficient solutions.
2. Morris Traversal
Morris Traversal allows for constant space traversal of binary trees without using recursion or a stack. While not directly applicable to all path sum problems, understanding this technique can be valuable for space-optimized solutions.
3. Segment Trees
For problems involving frequent updates and range queries on paths, segment trees can provide efficient solutions, though they’re more complex to implement.
4. Parallel Processing
In scenarios where the binary tree is extremely large, parallel processing techniques can be employed to divide the problem across multiple processors or threads.
Common Pitfalls and How to Avoid Them
When solving path sum problems, there are several common mistakes that even experienced programmers can make. Being aware of these can help you avoid them and write more robust solutions:
1. Forgetting to Handle Null Nodes
Always include null checks at the beginning of your recursive functions or when accessing node properties.
2. Incorrect Backtracking
In DFS approaches, ensure you’re properly backtracking by removing nodes from your path or adjusting your sum when moving back up the tree.
3. Overlooking Negative Numbers
Don’t assume all node values or path sums will be positive. This can lead to incorrect pruning of paths.
4. Mishandling Integer Overflow
For problems involving large sums, be mindful of potential integer overflow. Consider using long instead of int when necessary.
5. Inefficient Use of Data Structures
Choose appropriate data structures. For example, using a linked list instead of an array list for frequent insertions at the beginning.
Practice Problems
To reinforce your understanding and skills in solving path sum problems, here are some practice problems you can try:
- Binary Tree Paths: Given a binary tree, return all root-to-leaf paths in any order.
- Sum Root to Leaf Numbers: Given a binary tree containing digits from 0-9 only, each root-to-leaf path could represent a number. Find the total sum of all root-to-leaf numbers.
- Path Sum IV: If the depth of a tree is smaller than 5, consider this tree a full binary tree and use array representation.
- Longest Univalue Path: Given the root of a binary tree, return the length of the longest path, where each node in the path has the same value.
- Path In Zigzag Labelled Binary Tree: In an infinite binary tree where every node is labeled with an integer such that each node’s left child is labeled 2 * label and its right child is labeled 2 * label + 1, find the path from the root to the node with a given label.
Conclusion
Path sum problems are a crucial category of coding challenges that test your understanding of tree structures, recursion, and problem-solving skills. By mastering the strategies outlined in this guide – from basic recursive approaches to advanced techniques like prefix sums and dynamic programming – you’ll be well-equipped to tackle a wide range of path sum problems in your coding interviews and beyond.
Remember, the key to success with these problems lies not just in memorizing solutions, but in understanding the underlying principles and being able to adapt your approach to different variations of the problem. Practice regularly, analyze your mistakes, and don’t hesitate to explore multiple solutions to the same problem.
As you continue your journey in algorithmic problem-solving, keep challenging yourself with more complex tree problems and explore how these techniques can be applied to other data structures and problem domains. Happy coding!