Strategies for Solving Matrix Path Problems: A Comprehensive Guide
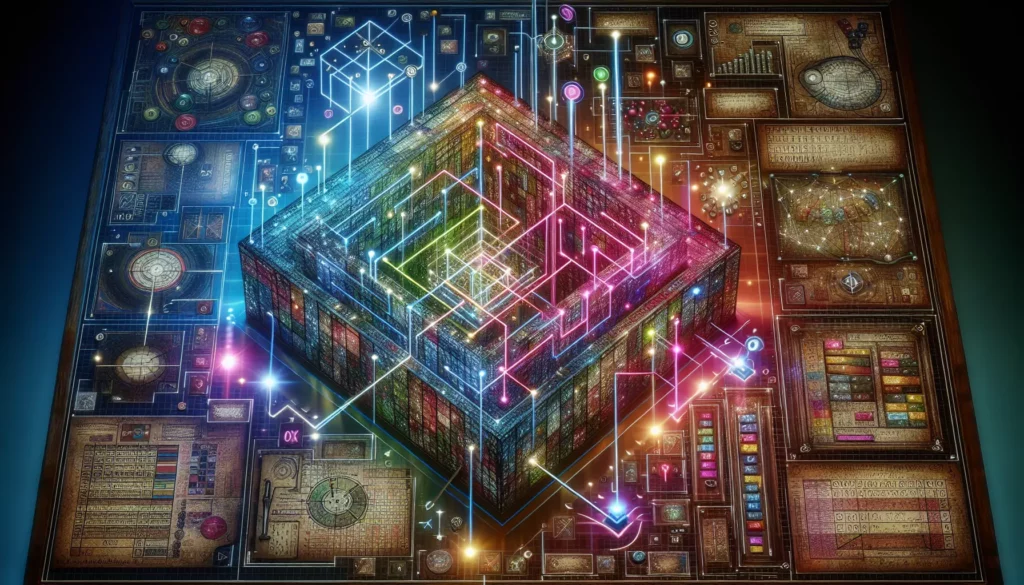
Matrix path problems are a common class of algorithmic challenges that frequently appear in coding interviews, particularly for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). These problems typically involve navigating through a 2D grid or matrix, often with the goal of finding an optimal path or counting the number of possible paths. In this comprehensive guide, we’ll explore various strategies and techniques for tackling matrix path problems, providing you with the tools you need to excel in your coding interviews and improve your algorithmic thinking skills.
Table of Contents
- Introduction to Matrix Path Problems
- Types of Matrix Path Problems
- Basic Concepts and Terminology
- Brute Force Approach
- Dynamic Programming Solutions
- Depth-First Search (DFS) Approach
- Breadth-First Search (BFS) Approach
- A* Algorithm for Pathfinding
- Optimization Techniques
- Common Matrix Path Problems and Solutions
- Practice Problems and Resources
- Conclusion
1. Introduction to Matrix Path Problems
Matrix path problems are a subset of graph problems that involve navigating through a 2D grid or matrix. These problems are popular in coding interviews because they test a candidate’s ability to think algorithmically, implement efficient solutions, and handle edge cases. Some common scenarios in matrix path problems include:
- Finding the shortest path between two points
- Counting the number of possible paths from one corner to another
- Finding a path that maximizes or minimizes a certain value
- Navigating through a maze with obstacles
- Solving puzzle-like problems on a grid
Understanding how to approach these problems is crucial for success in technical interviews and for developing strong problem-solving skills in general.
2. Types of Matrix Path Problems
Matrix path problems come in various forms, each with its own set of challenges and optimal solution strategies. Some common types include:
2.1 Shortest Path Problems
These problems involve finding the shortest path between two points in a matrix, often with obstacles or weighted cells. Examples include maze-solving problems and finding the quickest route on a map.
2.2 Path Counting Problems
In these problems, the goal is to count the number of possible paths from one point to another, usually with restrictions on movement (e.g., only moving right or down).
2.3 Maximum/Minimum Path Sum Problems
These problems require finding a path that maximizes or minimizes the sum of values along the path. Examples include finding the path with the maximum gold collection in a gold mine problem.
2.4 Obstacle Avoidance Problems
These involve navigating through a matrix with obstacles, often requiring finding a valid path or the shortest path while avoiding certain cells.
2.5 Multi-Dimensional Path Problems
While most problems deal with 2D matrices, some advanced problems may involve 3D or higher-dimensional grids, adding an extra layer of complexity.
3. Basic Concepts and Terminology
Before diving into specific strategies, it’s important to understand some basic concepts and terminology related to matrix path problems:
- Matrix/Grid: A 2D array representing the problem space.
- Cell: An individual element in the matrix, often representing a position or containing a value.
- Adjacent Cells: Cells that are next to each other, typically in four directions (up, down, left, right) or sometimes including diagonals.
- Path: A sequence of adjacent cells from a start point to an end point.
- Obstacles: Cells that cannot be traversed or have special properties.
- Valid Move: A movement from one cell to an adjacent cell that follows the problem’s rules.
- Visited Cells: Cells that have already been explored in the current path or algorithm.
4. Brute Force Approach
The brute force approach involves systematically enumerating all possible paths and checking each one for validity or optimality. While this method is straightforward and guarantees finding the correct solution, it’s often inefficient for larger matrices.
4.1 Recursive Brute Force
A common implementation of the brute force approach uses recursion to explore all possible paths. Here’s a basic example in Python for finding all paths from the top-left to the bottom-right of a matrix, moving only right or down:
def find_all_paths(matrix, row, col, path, all_paths):
if row == len(matrix) - 1 and col == len(matrix[0]) - 1:
all_paths.append(path)
return
# Move right
if col < len(matrix[0]) - 1:
find_all_paths(matrix, row, col + 1, path + [(row, col + 1)], all_paths)
# Move down
if row < len(matrix) - 1:
find_all_paths(matrix, row + 1, col, path + [(row + 1, col)], all_paths)
matrix = [[0, 0, 0], [0, 0, 0], [0, 0, 0]]
all_paths = []
find_all_paths(matrix, 0, 0, [(0, 0)], all_paths)
print(f"Number of paths: {len(all_paths)}")
4.2 Advantages and Disadvantages
Advantages:
- Simple to implement and understand
- Guarantees finding all possible solutions
- Suitable for small matrices or when all paths need to be enumerated
Disadvantages:
- Exponential time complexity (O(2^(m+n)) for an m x n matrix)
- Inefficient for large matrices
- May lead to stack overflow for deep recursion
5. Dynamic Programming Solutions
Dynamic Programming (DP) is a powerful technique for solving matrix path problems, especially when the goal is to find an optimal path or count the number of possible paths. DP works by breaking down the problem into smaller subproblems and storing their solutions to avoid redundant computations.
5.1 Bottom-Up DP Approach
The bottom-up approach builds the solution iteratively, starting from the smallest subproblems. Here’s an example of using DP to count the number of paths from the top-left to the bottom-right of a matrix, moving only right or down:
def count_paths(m, n):
dp = [[1 for _ in range(n)] for _ in range(m)]
for i in range(1, m):
for j in range(1, n):
dp[i][j] = dp[i-1][j] + dp[i][j-1]
return dp[m-1][n-1]
m, n = 3, 3
print(f"Number of paths in a {m}x{n} matrix: {count_paths(m, n)}")
5.2 Top-Down DP Approach (Memoization)
The top-down approach uses recursion with memoization to solve the problem. Here’s the same problem solved using the top-down approach:
def count_paths_memo(m, n, memo={}):
if m == 1 or n == 1:
return 1
if (m, n) in memo:
return memo[(m, n)]
memo[(m, n)] = count_paths_memo(m-1, n, memo) + count_paths_memo(m, n-1, memo)
return memo[(m, n)]
m, n = 3, 3
print(f"Number of paths in a {m}x{n} matrix: {count_paths_memo(m, n)}")
5.3 Advantages and Disadvantages
Advantages:
- Efficient for many types of matrix path problems
- Can solve problems with optimal substructure
- Often provides polynomial time complexity
Disadvantages:
- May require additional space for memoization or tabulation
- Not suitable for all types of path problems (e.g., those without optimal substructure)
- Can be challenging to formulate the DP solution for complex problems
6. Depth-First Search (DFS) Approach
Depth-First Search is a graph traversal algorithm that can be adapted for matrix path problems. It explores as far as possible along each branch before backtracking. DFS is particularly useful for finding paths, checking connectivity, and solving maze-like problems.
6.1 Recursive DFS Implementation
Here’s an example of using DFS to find a path from the top-left to the bottom-right of a matrix with obstacles:
def dfs_path(matrix, row, col, path):
if row < 0 or row >= len(matrix) or col < 0 or col >= len(matrix[0]) or matrix[row][col] == 1:
return False
if row == len(matrix) - 1 and col == len(matrix[0]) - 1:
path.append((row, col))
return True
path.append((row, col))
# Explore in all four directions
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)]
for dx, dy in directions:
if dfs_path(matrix, row + dx, col + dy, path):
return True
path.pop()
return False
matrix = [
[0, 0, 0, 0],
[1, 1, 0, 1],
[0, 0, 0, 0],
[0, 1, 1, 0]
]
path = []
if dfs_path(matrix, 0, 0, path):
print(f"Path found: {path}")
else:
print("No path found")
6.2 Advantages and Disadvantages
Advantages:
- Memory-efficient for deep graphs
- Can be easily implemented using recursion
- Useful for finding paths and connectivity
Disadvantages:
- May not find the shortest path
- Can get stuck in infinite loops if not implemented carefully
- Not ideal for finding all possible paths in large matrices
7. Breadth-First Search (BFS) Approach
Breadth-First Search is another graph traversal algorithm that can be applied to matrix path problems. Unlike DFS, BFS explores all the neighbor nodes at the present depth before moving to the nodes at the next depth level. This makes BFS particularly useful for finding the shortest path in unweighted graphs or matrices.
7.1 BFS Implementation
Here’s an example of using BFS to find the shortest path from the top-left to the bottom-right of a matrix with obstacles:
from collections import deque
def bfs_shortest_path(matrix):
if not matrix or not matrix[0]:
return None
rows, cols = len(matrix), len(matrix[0])
queue = deque([(0, 0, [(0, 0)])])
visited = set([(0, 0)])
while queue:
row, col, path = queue.popleft()
if row == rows - 1 and col == cols - 1:
return path
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)]
for dx, dy in directions:
new_row, new_col = row + dx, col + dy
if (0 <= new_row < rows and 0 <= new_col < cols and
matrix[new_row][new_col] == 0 and (new_row, new_col) not in visited):
queue.append((new_row, new_col, path + [(new_row, new_col)]))
visited.add((new_row, new_col))
return None
matrix = [
[0, 0, 0, 0],
[1, 1, 0, 1],
[0, 0, 0, 0],
[0, 1, 1, 0]
]
shortest_path = bfs_shortest_path(matrix)
if shortest_path:
print(f"Shortest path: {shortest_path}")
else:
print("No path found")
7.2 Advantages and Disadvantages
Advantages:
- Guarantees finding the shortest path in unweighted graphs
- Explores nodes in order of their distance from the start
- Useful for finding the shortest path or minimum number of steps
Disadvantages:
- More memory-intensive than DFS, especially for large matrices
- May be slower than DFS for finding any path (not necessarily the shortest)
- Not as efficient for deep, narrow graphs
8. A* Algorithm for Pathfinding
The A* algorithm is an informed search algorithm that combines the benefits of both Dijkstra’s algorithm and Best-First Search. It’s particularly useful for finding the shortest path in weighted graphs or matrices with varying terrain costs.
8.1 A* Implementation
Here’s an example of using the A* algorithm to find the shortest path in a weighted matrix:
import heapq
def manhattan_distance(a, b):
return abs(a[0] - b[0]) + abs(a[1] - b[1])
def astar(matrix, start, goal):
rows, cols = len(matrix), len(matrix[0])
def get_neighbors(pos):
r, c = pos
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)]
return [(r + dr, c + dc) for dr, dc in directions
if 0 <= r + dr < rows and 0 <= c + dc < cols]
g_score = {start: 0}
f_score = {start: manhattan_distance(start, goal)}
open_set = [(f_score[start], start)]
came_from = {}
while open_set:
current = heapq.heappop(open_set)[1]
if current == goal:
path = []
while current in came_from:
path.append(current)
current = came_from[current]
path.append(start)
return path[::-1]
for neighbor in get_neighbors(current):
tentative_g_score = g_score[current] + matrix[neighbor[0]][neighbor[1]]
if tentative_g_score < g_score.get(neighbor, float('inf')):
came_from[neighbor] = current
g_score[neighbor] = tentative_g_score
f_score[neighbor] = g_score[neighbor] + manhattan_distance(neighbor, goal)
heapq.heappush(open_set, (f_score[neighbor], neighbor))
return None
matrix = [
[1, 1, 1, 1],
[2, 2, 2, 1],
[1, 1, 1, 1],
[1, 2, 2, 1]
]
start = (0, 0)
goal = (3, 3)
path = astar(matrix, start, goal)
if path:
print(f"Shortest path: {path}")
else:
print("No path found")
8.2 Advantages and Disadvantages
Advantages:
- Finds the optimal path in weighted graphs
- Generally faster than Dijkstra’s algorithm due to heuristic guidance
- Can handle large search spaces efficiently
Disadvantages:
- More complex to implement than simpler algorithms like BFS or DFS
- Performance depends on the quality of the heuristic function
- May use more memory than uninformed search algorithms
9. Optimization Techniques
When solving matrix path problems, several optimization techniques can be applied to improve the efficiency of your solutions:
9.1 Memoization
Memoization involves storing the results of expensive function calls and returning the cached result when the same inputs occur again. This is particularly useful in recursive solutions and dynamic programming approaches.
9.2 Tabulation
Tabulation is the bottom-up approach in dynamic programming where you build a table of results for subproblems and use it to solve larger problems. This can often be more efficient than recursive approaches.
9.3 Space Optimization
In many DP problems, you can optimize space usage by using only a 1D array instead of a 2D array, or by using only two rows of a 2D array if you only need information from the previous row.
9.4 Bit Manipulation
For certain types of problems, especially those involving sets or visited states, bit manipulation can be used to reduce space complexity and improve performance.
9.5 Pruning
In search algorithms like DFS or A*, pruning involves eliminating paths that are guaranteed not to lead to a solution, reducing the search space.
10. Common Matrix Path Problems and Solutions
Let’s explore some common matrix path problems and their solutions:
10.1 Unique Paths
Problem: Count the number of unique paths from the top-left to the bottom-right of a matrix, moving only right or down.
Solution: This can be solved efficiently using dynamic programming.
def unique_paths(m, n):
dp = [[1] * n for _ in range(m)]
for i in range(1, m):
for j in range(1, n):
dp[i][j] = dp[i-1][j] + dp[i][j-1]
return dp[m-1][n-1]
print(unique_paths(3, 3)) # Output: 6
10.2 Minimum Path Sum
Problem: Find the path from top-left to bottom-right with the minimum sum of numbers along the path.
Solution: This can also be solved using dynamic programming.
def min_path_sum(grid):
m, n = len(grid), len(grid[0])
dp = [[0] * n for _ in range(m)]
dp[0][0] = grid[0][0]
for i in range(1, m):
dp[i][0] = dp[i-1][0] + grid[i][0]
for j in range(1, n):
dp[0][j] = dp[0][j-1] + grid[0][j]
for i in range(1, m):
for j in range(1, n):
dp[i][j] = min(dp[i-1][j], dp[i][j-1]) + grid[i][j]
return dp[m-1][n-1]
grid = [[1,3,1],[1,5,1],[4,2,1]]
print(min_path_sum(grid)) # Output: 7
10.3 Robot in a Grid
Problem: Find a path for a robot from the top-left to the bottom-right of a grid, avoiding obstacles.
Solution: This can be solved using DFS or BFS.
def find_path(grid):
if not grid or not grid[0]:
return None
path = []
if dfs_path(grid, 0, 0, path):
return path
return None
def dfs_path(grid, row, col, path):
if row >= len(grid) or col >= len(grid[0]) or grid[row][col] == 1:
return False
is_at_end = (row == len(grid) - 1) and (col == len(grid[0]) - 1)
if is_at_end or dfs_path(grid, row, col + 1, path) or dfs_path(grid, row + 1, col, path):
path.append((row, col))
return True
return False
grid = [
[0, 0, 0],
[0, 1, 0],
[0, 0, 0]
]
print(find_path(grid)) # Output: [(2, 2), (1, 2), (0, 2), (0, 1), (0, 0)]
11. Practice Problems and Resources
To improve your skills in solving matrix path problems, consider practicing with the following resources:
- LeetCode: Offers a wide range of matrix and path-finding problems with varying difficulty levels.
- HackerRank: Provides a “Problem Solving” track with many matrix-related challenges.
- GeeksforGeeks: Offers tutorials and practice problems on matrix and graph algorithms.
- Codeforces: Features competitive programming problems, including many that involve matrices and paths.
- Project Euler: Contains mathematical problems that often involve grid-based calculations.
Some specific problems to try:
- LeetCode 62: Unique Paths
- LeetCode 63: Unique Paths II
- LeetCode 64: Minimum Path Sum
- LeetCode 980: Unique Paths III
- LeetCode 1091: Shortest Path in Binary Matrix
12. Conclusion
Matrix path problems are a fundamental class of algorithmic challenges that test a wide range of problem-solving skills. By mastering the strategies and techniques discussed in this guide – from brute force approaches to dynamic programming, DFS, BFS, and A* algorithms – you’ll be well-equipped to tackle a variety of matrix path problems in coding interviews and real-world applications.
Remember that the key to success is not just knowing these algorithms, but understanding when and how to apply them. Practice regularly, analyze different problem patterns, and don’t hesitate to explore multiple approaches to the same problem. With time and experience, you’ll develop the intuition to quickly identify the most efficient solution for any given matrix path problem.
As you continue your journey in algorithmic problem-solving, keep exploring more advanced topics and stay updated with the latest techniques. The field of computer science is ever-evolving, and there’s always more to learn and discover. Happy coding!