Strategies for Solving Infinite Data Stream Problems: Mastering the Flow of Endless Information
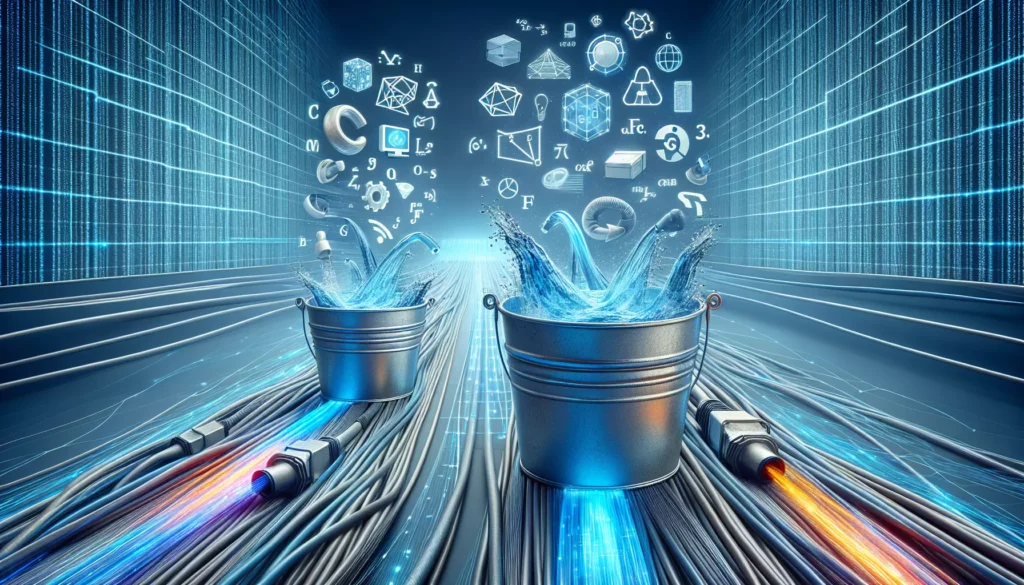
In the ever-evolving landscape of software engineering and data science, one of the most challenging yet fascinating areas is dealing with infinite data streams. These continuous flows of information present unique obstacles that require specialized techniques and algorithms to process, analyze, and extract meaningful insights. In this comprehensive guide, we’ll explore various strategies for solving infinite data stream problems, providing you with the tools and knowledge to tackle these complex scenarios effectively.
Understanding Infinite Data Streams
Before diving into specific strategies, it’s crucial to understand what infinite data streams are and why they pose unique challenges in the world of computing.
What are Infinite Data Streams?
An infinite data stream is a continuous sequence of data elements that arrive in real-time, without a predefined end. Unlike finite datasets, which have a known size and can be processed in batch, infinite streams require algorithms that can handle potentially endless input. Some common examples of infinite data streams include:
- Social media feeds
- Stock market tickers
- Sensor data from IoT devices
- Network traffic logs
- Click streams from web applications
Challenges of Processing Infinite Streams
Working with infinite data streams presents several unique challenges:
- Limited memory: You can’t store the entire stream in memory, as it’s potentially infinite.
- Real-time processing: Data must be processed as it arrives, often with strict latency requirements.
- Evolving patterns: The characteristics of the data may change over time, requiring adaptive algorithms.
- Out-of-order arrivals: Data elements may not arrive in a strictly sequential order.
- Fault tolerance: Systems must be robust enough to handle failures without losing critical information.
Key Strategies for Solving Infinite Data Stream Problems
Now that we understand the nature of infinite data streams, let’s explore some powerful strategies for solving problems in this domain.
1. Sliding Window Technique
The sliding window technique is a fundamental approach for processing infinite streams. It involves maintaining a “window” of the most recent n elements from the stream, allowing you to perform computations on a fixed-size subset of the data.
How it works:
- Define a window size n.
- As new elements arrive, add them to the window.
- When the window reaches size n+1, remove the oldest element.
- Perform computations on the elements within the window.
Example: Moving Average
Let’s implement a simple moving average calculator using the sliding window technique:
class MovingAverage {
private Queue<Integer> window;
private int maxSize;
private double sum;
public MovingAverage(int size) {
this.window = new LinkedList<>();
this.maxSize = size;
this.sum = 0.0;
}
public double next(int val) {
if (window.size() == maxSize) {
sum -= window.poll();
}
window.offer(val);
sum += val;
return sum / window.size();
}
}
This implementation maintains a queue of at most maxSize
elements, updating the sum and average as new values arrive.
2. Reservoir Sampling
Reservoir sampling is a probabilistic algorithm used to maintain a representative sample of k items from an infinite stream. It ensures that each item in the stream has an equal probability of being included in the final sample.
How it works:
- Initialize a reservoir of size k with the first k elements from the stream.
- For each subsequent element i (where i > k):
- Generate a random number j between 1 and i.
- If j ≤ k, replace the j-th element in the reservoir with the new element.
Example: Reservoir Sampling Implementation
import java.util.Random;
class ReservoirSampling {
private int[] reservoir;
private int count;
private Random random;
public ReservoirSampling(int k) {
this.reservoir = new int[k];
this.count = 0;
this.random = new Random();
}
public void processElement(int item) {
count++;
if (count <= reservoir.length) {
reservoir[count - 1] = item;
} else {
int j = random.nextInt(count);
if (j < reservoir.length) {
reservoir[j] = item;
}
}
}
public int[] getSample() {
return reservoir;
}
}
This implementation maintains a reservoir of a fixed size, updating it probabilistically as new elements arrive in the stream.
3. Bloom Filters
Bloom filters are probabilistic data structures used to test whether an element is a member of a set. They are particularly useful for infinite streams where you need to quickly determine if an item has been seen before, without storing the entire history.
How it works:
- Initialize a bit array of m bits, all set to 0.
- Choose k independent hash functions.
- To add an element, compute its k hash values and set the corresponding bits to 1.
- To query an element, compute its k hash values and check if all corresponding bits are 1.
Example: Simple Bloom Filter Implementation
import java.util.BitSet;
import java.util.function.Function;
class BloomFilter {
private BitSet bitSet;
private int size;
private Function<String, Integer>[] hashFunctions;
@SafeVarargs
public BloomFilter(int size, Function<String, Integer>... hashFunctions) {
this.bitSet = new BitSet(size);
this.size = size;
this.hashFunctions = hashFunctions;
}
public void add(String item) {
for (Function<String, Integer> hashFunction : hashFunctions) {
int hash = Math.abs(hashFunction.apply(item) % size);
bitSet.set(hash);
}
}
public boolean mightContain(String item) {
for (Function<String, Integer> hashFunction : hashFunctions) {
int hash = Math.abs(hashFunction.apply(item) % size);
if (!bitSet.get(hash)) {
return false;
}
}
return true;
}
}
This Bloom filter implementation allows you to add items and check for their potential presence in the set, with a small probability of false positives but no false negatives.
4. Count-Min Sketch
The Count-Min Sketch is a probabilistic data structure used for estimating the frequency of items in a data stream. It’s particularly useful when you need to track the most frequent items without storing all unique elements.
How it works:
- Initialize a 2D array of w columns and d rows, all set to 0.
- Choose d independent hash functions.
- To update an item, increment the counters at positions determined by the d hash functions.
- To query an item’s frequency, return the minimum value among the d counters for that item.
Example: Count-Min Sketch Implementation
import java.util.Random;
class CountMinSketch {
private int[][] sketch;
private int width;
private int depth;
private int[] hashA;
private int[] hashB;
public CountMinSketch(int width, int depth) {
this.width = width;
this.depth = depth;
this.sketch = new int[depth][width];
this.hashA = new int[depth];
this.hashB = new int[depth];
Random random = new Random();
for (int i = 0; i
This Count-Min Sketch implementation allows you to add items with their counts and estimate the frequency of any item in the stream.
5. Exponential Histograms
Exponential histograms are used for maintaining approximate statistics over sliding windows in data streams. They are particularly useful for problems like counting the number of 1’s in the last N elements of a binary stream.
How it works:
- Maintain buckets of exponentially increasing sizes (1, 2, 4, 8, …).
- Each bucket stores a timestamp of its oldest element.
- As new elements arrive, create new buckets or merge existing ones to maintain the exponential structure.
- Remove buckets that fall outside the current window.
Example: Simple Exponential Histogram
import java.util.ArrayList;
import java.util.List;
class ExponentialHistogram {
private List<Bucket> buckets;
private int windowSize;
private int currentTime;
public ExponentialHistogram(int windowSize) {
this.buckets = new ArrayList<>();
this.windowSize = windowSize;
this.currentTime = 0;
}
public void add(boolean value) {
currentTime++;
if (value) {
buckets.add(0, new Bucket(1, currentTime));
mergeBuckets();
}
removeExpiredBuckets();
}
public int count() {
int total = 0;
for (Bucket bucket : buckets) {
total += bucket.size;
}
return total;
}
private void mergeBuckets() {
int i = 0;
while (i < buckets.size() - 1) {
if (buckets.get(i).size == buckets.get(i + 1).size) {
Bucket merged = new Bucket(buckets.get(i).size * 2, buckets.get(i + 1).timestamp);
buckets.set(i, merged);
buckets.remove(i + 1);
} else {
i++;
}
}
}
private void removeExpiredBuckets() {
while (!buckets.isEmpty() && buckets.get(buckets.size() - 1).timestamp <= currentTime - windowSize) {
buckets.remove(buckets.size() - 1);
}
}
private static class Bucket {
int size;
int timestamp;
Bucket(int size, int timestamp) {
this.size = size;
this.timestamp = timestamp;
}
}
}
This exponential histogram implementation maintains an approximate count of 1’s in a sliding window of binary values.
Advanced Techniques for Infinite Data Streams
While the strategies mentioned above form a solid foundation for handling infinite data streams, there are several advanced techniques that can further enhance your ability to process and analyze continuous flows of information.
6. Adaptive Windowing (ADWIN)
Adaptive Windowing (ADWIN) is an algorithm that automatically adjusts the size of the sliding window based on the rate of change in the data stream. This technique is particularly useful when dealing with concept drift, where the statistical properties of the target variable change over time.
Key features of ADWIN:
- Dynamically grows the window when no change is apparent
- Shrinks the window when a change is detected
- Provides guarantees on the false positive and false negative rates of change detection
Example: ADWIN Implementation Sketch
import java.util.LinkedList;
class ADWIN {
private LinkedList<Double> window;
private double delta;
private int maxBucketNum;
public ADWIN(double delta) {
this.window = new LinkedList<>();
this.delta = delta;
this.maxBucketNum = 5; // Example value, can be adjusted
}
public boolean update(double value) {
boolean change = false;
window.addFirst(value);
while (detectChange()) {
int cutPoint = findCutPoint();
for (int i = window.size() - 1; i >= cutPoint; i--) {
window.removeLast();
}
change = true;
}
return change;
}
private boolean detectChange() {
// Implement change detection logic
// Compare statistics of subwindows
return false; // Placeholder
}
private int findCutPoint() {
// Implement logic to find the optimal cut point
return 0; // Placeholder
}
public double getWindowMean() {
return window.stream().mapToDouble(Double::doubleValue).average().orElse(0.0);
}
}
This ADWIN sketch provides a framework for implementing the adaptive windowing algorithm. The actual implementation would require more complex statistical calculations for change detection and cut point determination.
7. Hoeffding Trees
Hoeffding Trees, also known as Very Fast Decision Trees (VFDT), are a class of decision tree algorithms designed for streaming data. They allow for incremental learning and can make split decisions based on a small subset of the data, using the Hoeffding bound to guarantee that the split is nearly identical to the one that would be chosen using infinite examples.
Key features of Hoeffding Trees:
- Incremental learning: can update the model with each new example
- Ability to handle concept drift through techniques like adaptive trees
- Theoretical guarantees on decision quality
Example: Basic Hoeffding Tree Node
import java.util.HashMap;
import java.util.Map;
class HoeffdingTreeNode {
private Map<String, Integer> classCount;
private Map<String, Map<String, Integer>> attributeCount;
private HoeffdingTreeNode[] children;
private String splitAttribute;
public HoeffdingTreeNode() {
this.classCount = new HashMap<>();
this.attributeCount = new HashMap<>();
this.children = null;
this.splitAttribute = null;
}
public void updateStatistics(Map<String, String> instance, String className) {
classCount.put(className, classCount.getOrDefault(className, 0) + 1);
for (Map.Entry<String, String> entry : instance.entrySet()) {
String attribute = entry.getKey();
String value = entry.getValue();
attributeCount.putIfAbsent(attribute, new HashMap<>());
Map<String, Integer> valueCount = attributeCount.get(attribute);
valueCount.put(value, valueCount.getOrDefault(value, 0) + 1);
}
}
public boolean attemptSplit(double delta) {
// Implement split attempt logic using Hoeffding bound
// If split condition is met, create children nodes
return false; // Placeholder
}
public String classify(Map<String, String> instance) {
if (children == null) {
// Return the majority class at this leaf
return classCount.entrySet().stream()
.max(Map.Entry.comparingByValue())
.map(Map.Entry::getKey)
.orElse(null);
} else {
// Navigate to the appropriate child based on the split attribute
String value = instance.get(splitAttribute);
// Assume children are indexed by attribute value
return children[Integer.parseInt(value)].classify(instance);
}
}
}
This basic Hoeffding Tree node implementation provides a starting point for building a complete Hoeffding Tree classifier for streaming data.
8. Sketch-based Algorithms
Sketch-based algorithms are probabilistic techniques that maintain a summary or “sketch” of the data stream using sub-linear space. These methods are particularly useful for approximating various statistics and properties of the stream.
Popular sketch-based algorithms include:
- Count-Sketch: For estimating the frequency of items in a stream
- AMS Sketch: For estimating the second moment of a data stream
- HyperLogLog: For estimating the number of distinct elements in a stream
Example: HyperLogLog Implementation
import java.util.BitSet;
import java.util.Random;
class HyperLogLog {
private int[] registers;
private int numRegisters;
private Random random;
public HyperLogLog(int precision) {
this.numRegisters = 1 << precision;
this.registers = new int[numRegisters];
this.random = new Random();
}
public void add(String item) {
long hash = hash(item);
int bucket = (int) (hash & (numRegisters - 1));
int leadingZeros = Long.numberOfLeadingZeros(hash | numRegisters) + 1;
registers[bucket] = Math.max(registers[bucket], leadingZeros);
}
public long cardinality() {
double sum = 0;
for (int value : registers) {
sum += Math.pow(2, -value);
}
double estimate = (numRegisters / sum) * numRegisters * 0.79402; // Alpha correction
return Math.round(estimate);
}
private long hash(String item) {
// Implement a proper hash function here
return random.nextLong(); // Placeholder
}
}
This HyperLogLog implementation provides an efficient way to estimate the number of distinct elements in a data stream using a small, fixed amount of memory.
Practical Considerations for Infinite Data Stream Processing
When implementing solutions for infinite data stream problems, there are several practical considerations to keep in mind:
1. Scalability
Ensure your algorithms and data structures can handle the expected volume and velocity of the data stream. Consider distributed processing frameworks like Apache Flink, Apache Spark Streaming, or Apache Kafka Streams for high-throughput scenarios.
2. Fault Tolerance
Implement mechanisms to handle failures gracefully, such as checkpointing, state persistence, and exactly-once processing semantics. This is crucial for maintaining accuracy in long-running stream processing applications.
3. Latency Management
Balance the trade-off between processing latency and result accuracy. Some applications may require real-time responses, while others can tolerate some delay for improved precision.
4. Adaptive Algorithms
Consider using adaptive algorithms that can adjust to changing data distributions and concept drift. This is particularly important for long-running streams where the underlying patterns may evolve over time.
5. Resource Efficiency
Optimize your implementations for memory and CPU usage. Streaming algorithms often need to process data at high rates with limited resources.
6. Monitoring and Debugging
Implement robust monitoring and logging mechanisms to track the performance of your stream processing system. This is crucial for identifying bottlenecks, detecting anomalies, and ensuring the overall health of the system.
Conclusion
Solving infinite data stream problems requires a unique set of skills and techniques that differ significantly from traditional batch processing approaches. By mastering strategies like sliding windows, reservoir sampling, probabilistic data structures, and advanced techniques like adaptive windowing and Hoeffding trees, you’ll be well-equipped to tackle the challenges of real-time, continuous data processing.
Remember that the field of stream processing is continually evolving, with new algorithms and techniques being developed to address emerging challenges. Stay curious, keep experimenting, and don’t hesitate to combine multiple strategies to create robust solutions for your specific use cases.
As you continue your journey in mastering infinite data stream processing, consider exploring more advanced topics such as distributed stream processing frameworks, real-time machine learning on streams, and complex event processing. With practice and persistence, you’ll be able to harness the power of infinite data streams to drive insights, make real-time decisions, and build innovative applications that can keep up with the ever-flowing river of data in our digital world.