Strategies for Solving Array Manipulation Problems
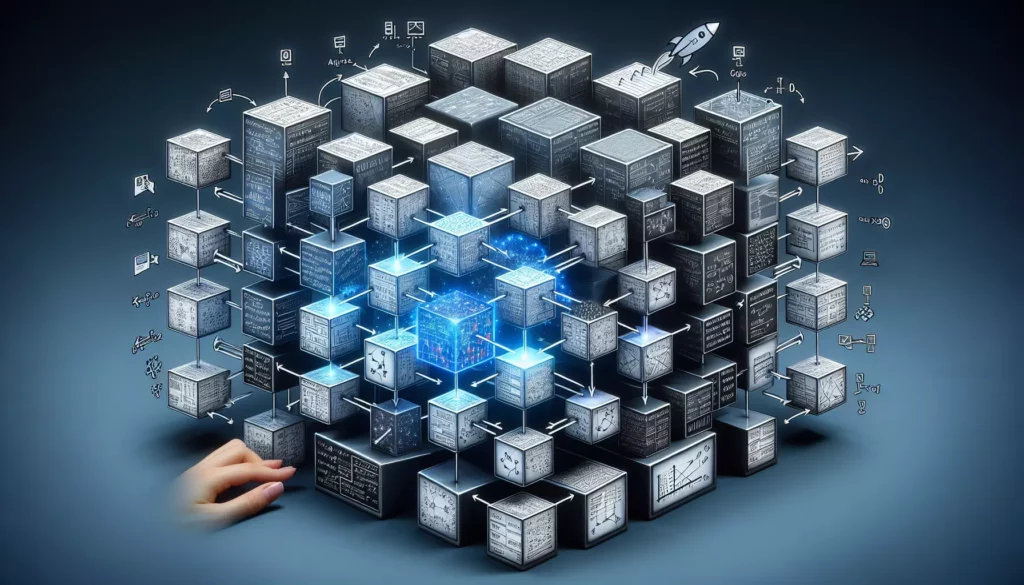
Array manipulation problems are a cornerstone of coding interviews and a fundamental skill for any programmer. Whether you’re preparing for a technical interview at a FAANG company or simply honing your problem-solving skills, mastering array manipulation is crucial. In this comprehensive guide, we’ll explore various strategies and techniques to tackle array manipulation problems efficiently and effectively.
Understanding Array Manipulation
Before diving into specific strategies, it’s essential to understand what array manipulation entails. Array manipulation involves modifying, rearranging, or extracting information from arrays. These operations can range from simple tasks like reversing an array to more complex problems like finding subarrays with specific properties.
Common array manipulation operations include:
- Inserting or deleting elements
- Searching for specific elements
- Sorting the array
- Merging or splitting arrays
- Rotating the array
- Finding subarrays with certain properties
Key Strategies for Array Manipulation
1. Two-Pointer Technique
The two-pointer technique is a powerful strategy for solving many array problems. It involves using two pointers that either move towards each other or in the same direction to solve the problem efficiently.
Example: Reversing an Array
Here’s how you can use the two-pointer technique to reverse an array:
def reverse_array(arr):
left = 0
right = len(arr) - 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
return arr
# Example usage
arr = [1, 2, 3, 4, 5]
print(reverse_array(arr)) # Output: [5, 4, 3, 2, 1]
When to Use Two-Pointer Technique
- Problems involving reversing or palindrome checks
- Finding pairs in a sorted array with a specific sum
- Removing duplicates from a sorted array
- Partitioning arrays (e.g., Dutch National Flag problem)
2. Sliding Window Technique
The sliding window technique is particularly useful for problems that involve subarrays or subsequences. It maintains a “window” that can expand or contract to solve the problem efficiently.
Example: Maximum Sum Subarray of Size K
def max_sum_subarray(arr, k):
n = len(arr)
if n < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, n):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
# Example usage
arr = [1, 4, 2, 10, 23, 3, 1, 0, 20]
k = 4
print(max_sum_subarray(arr, k)) # Output: 39
When to Use Sliding Window Technique
- Finding subarrays with a specific sum
- Longest substring with K distinct characters
- Minimum size subarray sum
- Maximum of all subarrays of size K
3. Prefix Sum Technique
The prefix sum technique involves creating an array where each element is the cumulative sum of all previous elements. This can significantly speed up range sum queries and other related problems.
Example: Range Sum Query
class NumArray:
def __init__(self, nums):
self.prefix_sum = [0]
for num in nums:
self.prefix_sum.append(self.prefix_sum[-1] + num)
def sumRange(self, left, right):
return self.prefix_sum[right + 1] - self.prefix_sum[left]
# Example usage
num_array = NumArray([1, 3, 5])
print(num_array.sumRange(0, 2)) # Output: 9
print(num_array.sumRange(1, 2)) # Output: 8
When to Use Prefix Sum Technique
- Range sum queries
- Finding subarrays with a given sum
- Calculating cumulative data
- Problems involving difference arrays
4. Divide and Conquer
The divide and conquer strategy involves breaking down a problem into smaller subproblems, solving them, and then combining the results to solve the original problem.
Example: Merge Sort
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Example usage
arr = [38, 27, 43, 3, 9, 82, 10]
print(merge_sort(arr)) # Output: [3, 9, 10, 27, 38, 43, 82]
When to Use Divide and Conquer
- Sorting algorithms (e.g., Merge Sort, Quick Sort)
- Binary Search
- Maximum Subarray Problem
- Matrix Multiplication
5. Hash Tables
Using hash tables (or dictionaries in Python) can significantly improve the efficiency of many array manipulation problems, especially those involving counting or looking up elements.
Example: Two Sum Problem
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Example usage
nums = [2, 7, 11, 15]
target = 9
print(two_sum(nums, target)) # Output: [0, 1]
When to Use Hash Tables
- Finding pairs with a specific sum
- Counting frequencies of elements
- Checking for duplicates
- Implementing caches or memoization
Advanced Techniques for Array Manipulation
1. Binary Search on Arrays
Binary search is not just for searching; it can be applied creatively to solve various array problems, especially those involving sorted arrays or where the search space can be reduced logarithmically.
Example: Search in Rotated Sorted Array
def search_rotated(nums, target):
left, right = 0, len(nums) - 1
while left <= right:
mid = (left + right) // 2
if nums[mid] == target:
return mid
# Check which half is sorted
if nums[left] <= nums[mid]:
if nums[left] <= target < nums[mid]:
right = mid - 1
else:
left = mid + 1
else:
if nums[mid] < target <= nums[right]:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
nums = [4, 5, 6, 7, 0, 1, 2]
target = 0
print(search_rotated(nums, target)) # Output: 4
When to Use Binary Search on Arrays
- Searching in sorted arrays
- Finding the smallest or largest element satisfying a condition
- Optimizing time complexity from O(n) to O(log n)
- Problems involving “peak” finding
2. Dynamic Programming on Arrays
Dynamic programming can be a powerful tool for solving complex array problems, especially those involving optimizing some value over subarrays.
Example: Maximum Subarray Sum
def max_subarray_sum(nums):
max_sum = current_sum = nums[0]
for num in nums[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Example usage
nums = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
print(max_subarray_sum(nums)) # Output: 6
When to Use Dynamic Programming on Arrays
- Problems involving optimizing over subarrays
- Longest Increasing Subsequence
- Edit Distance
- Coin Change Problem
3. Bit Manipulation Techniques
Bit manipulation can be incredibly useful for certain types of array problems, especially those involving integers or when you need to optimize space usage.
Example: Find Single Number in Array where Every Element Appears Twice
def find_single_number(nums):
result = 0
for num in nums:
result ^= num
return result
# Example usage
nums = [4, 1, 2, 1, 2]
print(find_single_number(nums)) # Output: 4
When to Use Bit Manipulation
- Problems involving XOR operations
- Optimizing space usage
- Manipulating binary representations of numbers
- Solving problems related to powers of 2
Common Pitfalls and How to Avoid Them
When solving array manipulation problems, there are several common pitfalls that programmers often encounter. Being aware of these can help you avoid errors and write more efficient code.
1. Off-by-One Errors
Off-by-one errors are very common, especially when dealing with array indices. Always double-check your loop conditions and array access.
How to Avoid:
- Use < instead of <= when iterating through array indices
- Be careful with zero-indexing
- Test your code with edge cases (e.g., arrays of length 0 or 1)
2. Not Considering Edge Cases
Failing to consider edge cases like empty arrays, arrays with a single element, or arrays with all identical elements can lead to bugs.
How to Avoid:
- Always test your code with edge cases
- Consider what happens when the input is empty or has only one element
- Think about extreme values (very large or very small numbers)
3. Inefficient Time Complexity
Using nested loops when unnecessary or not optimizing your algorithm can lead to poor performance, especially with large inputs.
How to Avoid:
- Analyze the time complexity of your solution
- Look for opportunities to use more efficient data structures (e.g., hash tables)
- Consider using techniques like sliding window or two pointers to reduce time complexity
4. Modifying the Input Array Unintentionally
Some problems require you to avoid modifying the input array. Accidentally doing so can lead to incorrect results or side effects.
How to Avoid:
- Make a copy of the input array if you need to modify it
- Use slicing or list comprehension to create new arrays instead of modifying the original
- Be explicit about whether your function modifies the input or returns a new array
Practice and Improvement Strategies
Mastering array manipulation problems requires consistent practice and a structured approach to learning. Here are some strategies to improve your skills:
1. Solve Problems Regularly
Consistency is key. Set aside time each day to solve at least one array manipulation problem. Platforms like LeetCode, HackerRank, and AlgoCademy offer a wide variety of problems to practice with.
2. Implement Common Algorithms from Scratch
Implement sorting algorithms, searching algorithms, and other common array operations from scratch. This will deepen your understanding of how these algorithms work and improve your coding skills.
3. Analyze Multiple Solutions
For each problem you solve, look at different solutions. Understand the trade-offs between different approaches in terms of time complexity, space complexity, and readability.
4. Focus on Problem-Solving Patterns
Identify common patterns in array manipulation problems. The more problems you solve, the more you’ll recognize these patterns and be able to apply them to new problems.
5. Time Your Problem-Solving Sessions
Practice solving problems under time constraints. This will help you prepare for coding interviews where you’ll need to think and code quickly.
6. Review and Reflect
After solving a problem, take time to reflect on your approach. Could you have solved it more efficiently? Are there edge cases you missed? This reflection will help you improve over time.
Conclusion
Array manipulation is a fundamental skill in programming and a common focus in coding interviews. By mastering the strategies and techniques outlined in this guide, you’ll be well-equipped to tackle a wide range of array problems. Remember that improvement comes with practice, so make solving array problems a regular part of your coding routine.
As you continue to develop your skills, you’ll find that many of these strategies apply not just to arrays, but to other data structures and problem types as well. The problem-solving mindset you develop while working on array manipulation problems will serve you well throughout your programming career.
Keep practicing, stay curious, and don’t be afraid to tackle challenging problems. With time and effort, you’ll become proficient in array manipulation and be well-prepared for any coding challenge that comes your way.