Strategies for Learning Multiple Programming Languages: A Comprehensive Guide
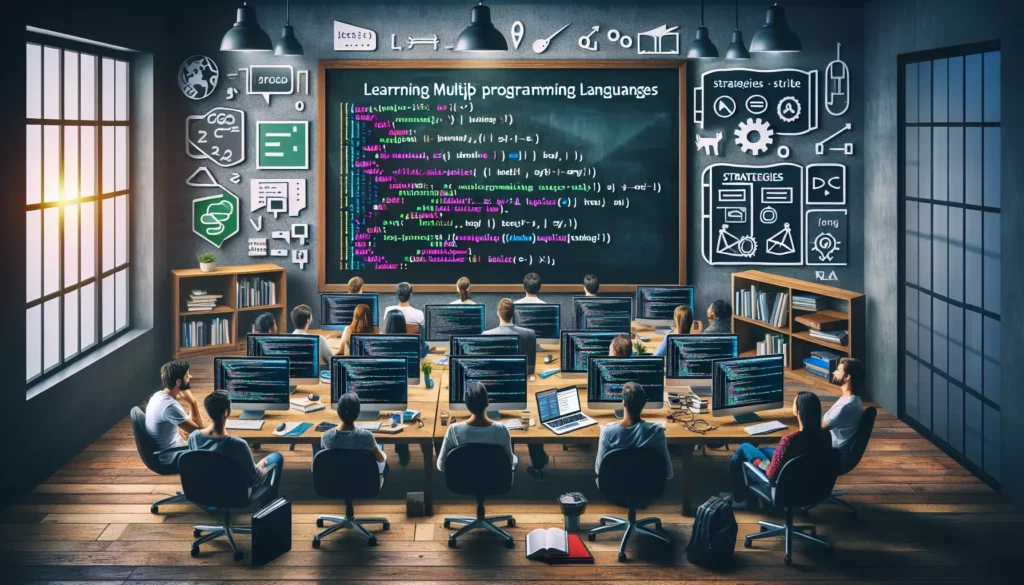
In today’s rapidly evolving tech landscape, mastering multiple programming languages has become increasingly important for developers. Whether you’re a beginner looking to expand your skill set or an experienced programmer aiming to stay competitive, learning multiple languages can open up new opportunities and enhance your problem-solving abilities. This comprehensive guide will explore effective strategies for learning multiple programming languages, helping you navigate the challenges and maximize your learning potential.
1. Start with a Strong Foundation
Before diving into multiple languages, it’s crucial to have a solid understanding of programming fundamentals. These core concepts are often transferable across different languages and will make your learning process smoother.
Key Fundamentals to Master:
- Variables and data types
- Control structures (if-else statements, loops)
- Functions and methods
- Object-oriented programming concepts
- Data structures (arrays, lists, dictionaries)
- Algorithms and problem-solving techniques
By mastering these fundamentals, you’ll be better equipped to recognize similarities and differences between languages, making it easier to adapt to new syntax and paradigms.
2. Choose Languages Strategically
When deciding which languages to learn, consider your goals, industry trends, and the relationships between different languages. Here are some factors to keep in mind:
Career Goals:
- Web Development: JavaScript, Python, Ruby, PHP
- Mobile Development: Swift (iOS), Kotlin (Android), JavaScript (React Native)
- Data Science: Python, R, SQL
- Systems Programming: C, C++, Rust
- Game Development: C#, C++, JavaScript
Language Families:
Learning languages from different families can broaden your perspective and make it easier to pick up related languages in the future. Consider exploring:
- C-style syntax: Java, C#, JavaScript
- Dynamic languages: Python, Ruby
- Functional languages: Haskell, Scala, F#
3. Leverage Similarities Between Languages
Many programming languages share common concepts and structures. By identifying these similarities, you can accelerate your learning process and transfer knowledge between languages more effectively.
Example: Loops in Different Languages
Let’s compare how for loops are implemented in several languages:
Python:
for i in range(5):
print(i)
JavaScript:
for (let i = 0; i < 5; i++) {
console.log(i);
}
Java:
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
By recognizing the similar structure and purpose of these loops, you can quickly adapt to the syntax differences when learning a new language.
4. Focus on One Language at a Time
While the goal is to learn multiple languages, it’s generally more effective to focus on one language at a time, especially when starting out. This approach allows you to:
- Develop a deeper understanding of the language’s nuances
- Build confidence in your skills
- Avoid confusion between similar syntax or concepts
- Complete projects and see tangible progress
Once you’ve gained proficiency in one language, you can more easily branch out to others, using your existing knowledge as a foundation.
5. Practice Active Learning
Passive learning through reading or watching tutorials is not enough to truly master a programming language. Engage in active learning techniques to reinforce your understanding and improve retention:
Coding Exercises:
Regularly solve coding challenges on platforms like LeetCode, HackerRank, or CodeWars. These exercises help you apply your knowledge and improve problem-solving skills.
Build Projects:
Create small projects that utilize the features of the language you’re learning. This hands-on experience is invaluable for understanding how to use the language in real-world scenarios.
Pair Programming:
Collaborate with other learners or experienced developers. This can expose you to different coding styles and problem-solving approaches.
Code Reviews:
Participate in code reviews or submit your code for feedback. This helps you learn best practices and improve the quality of your code.
6. Understand Language-Specific Paradigms and Best Practices
Each programming language has its own idioms, design patterns, and best practices. To truly master a language, it’s essential to understand and adopt these language-specific approaches.
Example: Python’s Zen of Python
Python has a set of guiding principles known as the “Zen of Python.” Understanding and applying these principles will help you write more Pythonic code:
import this
This command in Python will display the Zen of Python, which includes principles like:
- Explicit is better than implicit.
- Simple is better than complex.
- Readability counts.
Example: JavaScript’s Functional Programming Features
JavaScript supports functional programming concepts. Understanding and using these can lead to more efficient and maintainable code:
// Using map, filter, and reduce
const numbers = [1, 2, 3, 4, 5];
const doubledEvenNumbers = numbers
.filter(num => num % 2 === 0)
.map(num => num * 2);
console.log(doubledEvenNumbers); // Output: [4, 8]
7. Utilize Language-Specific Tools and Ecosystems
Each programming language comes with its own set of tools, frameworks, and libraries. Familiarizing yourself with these ecosystems can greatly enhance your productivity and understanding of the language.
Example: Python’s Scientific Computing Stack
When learning Python for data science, it’s crucial to understand libraries like NumPy, Pandas, and Matplotlib:
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# Create a simple dataset
data = {'x': np.arange(0, 10), 'y': np.random.randn(10)}
df = pd.DataFrame(data)
# Plot the data
plt.figure(figsize=(10, 6))
plt.plot(df['x'], df['y'], marker='o')
plt.title('Random Data Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
Example: JavaScript’s Node.js and npm
For JavaScript, understanding Node.js and the npm ecosystem is essential for server-side development:
// Install a package
npm install express
// Use the package in your code
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
8. Learn to Read Documentation Effectively
As you learn multiple languages, you’ll frequently refer to documentation. Developing the skill to quickly find and understand information in documentation is crucial. Here are some tips:
- Familiarize yourself with the structure of official documentation for each language.
- Practice reading and interpreting code examples in the documentation.
- Use the search function effectively to find specific information.
- Pay attention to version-specific features and deprecation notices.
9. Engage with the Developer Community
Joining developer communities can accelerate your learning and keep you motivated. Consider these options:
- Join language-specific forums or subreddits (e.g., r/learnprogramming, r/Python, r/javascript)
- Participate in local meetups or coding groups
- Contribute to open-source projects
- Attend conferences or workshops
Engaging with the community not only helps you learn but also exposes you to different perspectives and real-world applications of the languages you’re studying.
10. Develop a Comparative Mindset
As you learn multiple languages, develop the habit of comparing and contrasting them. This comparative approach can deepen your understanding and help you choose the right tool for specific tasks.
Example: String Interpolation Comparison
Let’s compare string interpolation across different languages:
Python (f-strings):
name = "Alice"
age = 30
print(f"My name is {name} and I am {age} years old.")
JavaScript (Template literals):
const name = "Alice";
const age = 30;
console.log(`My name is ${name} and I am ${age} years old.`);
Ruby:
name = "Alice"
age = 30
puts "My name is #{name} and I am #{age} years old."
By comparing these approaches, you can appreciate the similarities and differences, making it easier to switch between languages and choose the most appropriate one for a given task.
11. Embrace Continuous Learning
The field of programming is constantly evolving, with new languages, frameworks, and best practices emerging regularly. To stay competitive and proficient in multiple languages, adopt a mindset of continuous learning:
- Set aside time regularly to explore new features or updates in the languages you know.
- Experiment with new languages or paradigms to broaden your perspective.
- Follow industry trends and be open to learning emerging technologies.
- Reflect on your learning process and adjust your strategies as needed.
12. Leverage Cross-Language Projects
One effective way to reinforce your skills in multiple languages is to work on projects that utilize more than one language. This approach allows you to see how different languages can work together and helps you understand their strengths and weaknesses in various contexts.
Example: Full-Stack Web Application
Create a web application that uses multiple languages:
- Frontend: HTML, CSS, JavaScript (React)
- Backend: Python (Django or Flask)
- Database: SQL
This type of project allows you to practice several languages in a real-world scenario, reinforcing your skills and highlighting the interplay between different technologies.
13. Use Language-Specific Features to Solve Problems
Each programming language has unique features that make certain tasks easier or more efficient. As you learn multiple languages, try to identify and utilize these language-specific strengths.
Example: List Comprehensions in Python
Python’s list comprehensions provide a concise way to create lists based on existing lists:
# Creating a list of squares
squares = [x**2 for x in range(10)]
print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
# Filtering even numbers
even_numbers = [x for x in range(20) if x % 2 == 0]
print(even_numbers) # Output: [0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
Example: Destructuring in JavaScript
JavaScript’s destructuring assignment syntax can simplify working with arrays and objects:
// Array destructuring
const [first, second, ...rest] = [1, 2, 3, 4, 5];
console.log(first, second, rest); // Output: 1 2 [3, 4, 5]
// Object destructuring
const person = { name: 'Alice', age: 30, city: 'New York' };
const { name, age } = person;
console.log(name, age); // Output: Alice 30
14. Practice Code Translation
A valuable exercise for reinforcing your understanding of multiple languages is to practice translating code from one language to another. This helps you identify similarities and differences between languages and strengthens your ability to think in different programming paradigms.
Example: Fibonacci Sequence
Let’s implement a function to generate the Fibonacci sequence in Python and JavaScript:
Python:
def fibonacci(n):
fib = [0, 1]
for i in range(2, n):
fib.append(fib[i-1] + fib[i-2])
return fib[:n]
print(fibonacci(10)) # Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
JavaScript:
function fibonacci(n) {
const fib = [0, 1];
for (let i = 2; i < n; i++) {
fib[i] = fib[i-1] + fib[i-2];
}
return fib.slice(0, n);
}
console.log(fibonacci(10)); // Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
By translating algorithms between languages, you reinforce your understanding of both the algorithm itself and the specific syntax and features of each language.
15. Develop Language-Agnostic Problem-Solving Skills
While it’s important to learn the specifics of each language, developing strong, language-agnostic problem-solving skills is equally crucial. These skills allow you to approach problems conceptually before implementing a solution in any particular language.
Key Problem-Solving Steps:
- Understand the problem thoroughly
- Break down the problem into smaller, manageable parts
- Plan your approach using pseudocode or flowcharts
- Implement the solution in your chosen language
- Test and refine your solution
By focusing on these problem-solving steps, you’ll be better equipped to tackle challenges in any programming language.
Conclusion
Learning multiple programming languages is a valuable skill that can significantly enhance your capabilities as a developer. By following these strategies, you can approach the learning process more effectively and efficiently. Remember that becoming proficient in multiple languages takes time and consistent effort. Stay curious, practice regularly, and don’t be afraid to challenge yourself with new concepts and paradigms.
As you continue your journey in mastering multiple programming languages, keep in mind that the goal is not just to accumulate knowledge of syntax and features, but to develop a deeper understanding of programming concepts and problem-solving techniques. This broader perspective will make you a more versatile and valuable developer, capable of choosing the right tool for any given task and adapting to new technologies as they emerge.
Happy coding, and may your multi-language programming adventures be both challenging and rewarding!