Strategies for Learning from Codebase of Open Source Projects
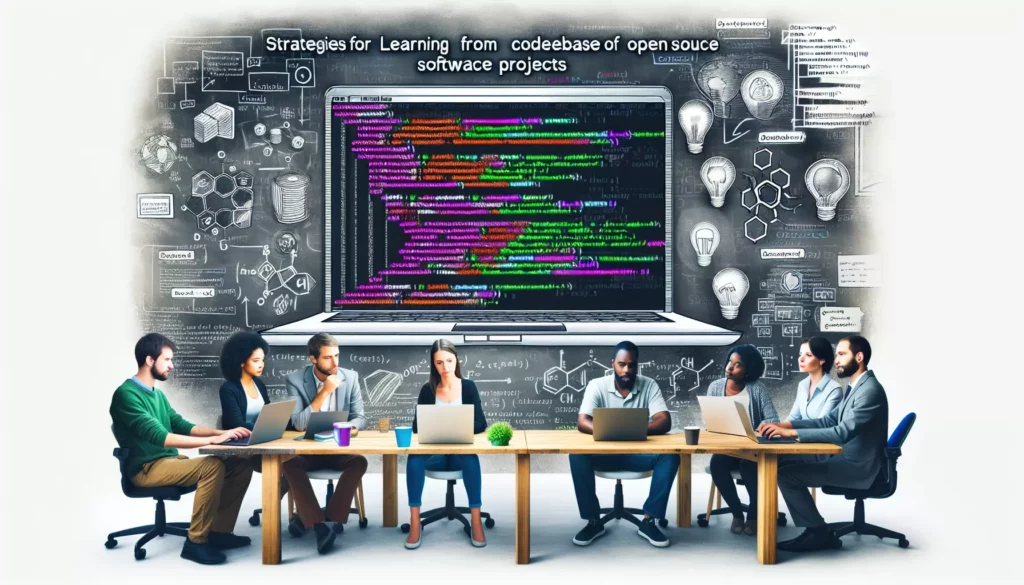
In the world of software development, open source projects serve as invaluable resources for learning and improving coding skills. These projects provide a wealth of real-world code examples, best practices, and collaborative environments that can significantly enhance a programmer’s knowledge and abilities. This article will explore effective strategies for learning from the codebase of open source projects, helping you leverage these resources to become a more proficient developer.
1. Choose the Right Project
The first step in learning from open source projects is selecting the right one to study. Consider the following factors when making your choice:
- Relevance to your goals: Choose a project that aligns with your learning objectives or the technologies you want to master.
- Project size and complexity: Start with smaller projects if you’re a beginner, and gradually move to larger, more complex ones as you gain experience.
- Active community: Look for projects with active contributors and regular updates, as these tend to have better documentation and support.
- Code quality: Opt for well-maintained projects with clean, readable code and good documentation.
2. Familiarize Yourself with the Project Structure
Once you’ve chosen a project, take time to understand its overall structure and organization. This will help you navigate the codebase more efficiently:
- Read the project’s README file and documentation to get an overview of its purpose and architecture.
- Examine the directory structure and identify key components or modules.
- Look for configuration files, build scripts, and other important project files.
- Understand the project’s branching strategy and version control workflow.
3. Start with Small, Manageable Chunks
Don’t try to understand the entire codebase at once. Instead, focus on specific parts or features:
- Begin with a single module or component that interests you.
- Trace the execution flow of a particular feature or functionality.
- Analyze how different parts of the code interact with each other.
- Gradually expand your understanding to encompass larger portions of the project.
4. Use Version Control History
Leverage the project’s version control history to gain insights into its evolution and development process:
- Study commit messages to understand the rationale behind code changes.
- Examine pull requests and code reviews to learn about best practices and coding standards.
- Track the development of specific features over time to see how they were implemented and refined.
- Use tools like
git blame
to identify when and why particular code changes were made.
5. Analyze Code Patterns and Techniques
Pay attention to recurring patterns and techniques used throughout the codebase:
- Identify design patterns and architectural styles employed in the project.
- Study how the code handles error handling, logging, and debugging.
- Examine performance optimization techniques and efficient algorithms.
- Look for creative solutions to common programming challenges.
6. Run and Debug the Code
To truly understand how the code works, set up the project locally and run it:
- Follow the project’s setup instructions to get it running on your machine.
- Use a debugger to step through the code execution and observe variable states.
- Experiment with modifying the code to see how it affects the project’s behavior.
- Write and run unit tests to better understand the code’s functionality.
7. Engage with the Community
Take advantage of the project’s community to enhance your learning experience:
- Join mailing lists, forums, or chat channels associated with the project.
- Ask questions and seek clarification on aspects of the code you don’t understand.
- Participate in discussions about feature requests and bug reports.
- Offer to help with documentation or small bug fixes to gain hands-on experience.
8. Contribute to the Project
One of the best ways to learn from an open source project is to actively contribute to it:
- Start with small, manageable tasks like fixing typos or improving documentation.
- Look for “good first issue” labels or beginner-friendly tasks in the project’s issue tracker.
- Submit pull requests for bug fixes or small feature enhancements.
- Seek feedback on your contributions and learn from code reviews.
9. Compare Different Implementations
Expand your learning by comparing different implementations of similar features across projects:
- Study how different projects solve similar problems or implement common functionalities.
- Analyze the trade-offs between different approaches and their impact on performance, maintainability, and scalability.
- Look for innovative solutions that you can apply to your own projects.
10. Document Your Learning
Keep track of what you’ve learned and the insights you’ve gained:
- Maintain a learning journal or blog to document your observations and discoveries.
- Create diagrams or flowcharts to visualize complex parts of the codebase.
- Write summaries of key concepts, patterns, or techniques you’ve encountered.
- Share your findings with others through blog posts, tutorials, or presentations.
11. Implement Similar Features in Your Own Projects
Apply what you’ve learned to your own coding projects:
- Try to implement similar features or functionalities in your personal projects.
- Experiment with adapting design patterns or architectural styles you’ve observed.
- Challenge yourself to improve upon the solutions you’ve studied.
12. Analyze Test Suites
Study the project’s test suites to gain insights into best testing practices:
- Examine how unit tests, integration tests, and end-to-end tests are structured.
- Learn about different testing frameworks and methodologies used in the project.
- Understand how edge cases and error scenarios are handled in tests.
- Practice writing your own tests for the codebase to reinforce your understanding.
13. Study Code Reviews
Dive into the project’s code review process to learn about best practices and coding standards:
- Read through past code reviews to understand common feedback patterns.
- Pay attention to how senior developers provide constructive criticism.
- Learn about the project’s coding style guidelines and conventions.
- Practice reviewing code yourself to develop a critical eye for code quality.
14. Explore Dependencies and Libraries
Analyze how the project integrates with external dependencies and libraries:
- Study the project’s dependency management system and configuration.
- Investigate how third-party libraries are used and integrated into the codebase.
- Learn about best practices for managing and updating dependencies.
- Explore alternatives to the chosen libraries and understand the reasons behind specific choices.
15. Analyze Performance Optimizations
Look for performance optimization techniques employed in the project:
- Study how the code handles resource-intensive operations.
- Examine caching strategies and data storage optimizations.
- Analyze algorithms used for efficient data processing and manipulation.
- Look for concurrency and parallelism techniques to improve performance.
16. Understand Security Practices
Pay attention to security measures implemented in the codebase:
- Study how authentication and authorization are handled.
- Examine input validation and sanitization techniques.
- Look for measures to prevent common security vulnerabilities (e.g., SQL injection, XSS).
- Analyze how sensitive data is stored and transmitted securely.
17. Learn from Project History
Dive into the project’s historical development to understand its evolution:
- Study early versions of the codebase to see how it has grown and improved over time.
- Analyze major architectural changes and the reasons behind them.
- Look for deprecated features and understand why they were removed or replaced.
- Examine how the project has adapted to new technologies or industry trends.
18. Explore Different Branches
Investigate different branches in the project’s repository:
- Compare development, staging, and production branches to understand the release process.
- Study feature branches to see how new functionalities are developed and integrated.
- Examine long-running branches for major refactoring or architectural changes.
19. Analyze Error Handling and Logging
Study how the project handles errors and implements logging:
- Examine error handling strategies and exception management.
- Look at how errors are reported and communicated to users or developers.
- Analyze logging practices for debugging and monitoring purposes.
- Study how the project integrates with monitoring and alerting systems.
20. Explore Continuous Integration and Deployment
Investigate the project’s CI/CD pipeline:
- Study the configuration of CI/CD tools and services used in the project.
- Examine automated testing and deployment processes.
- Analyze how code quality checks and linting are integrated into the pipeline.
- Learn about deployment strategies and release management practices.
Conclusion
Learning from the codebase of open source projects is a powerful way to enhance your programming skills and gain real-world experience. By following these strategies, you can effectively navigate and understand complex codebases, learn best practices, and apply newfound knowledge to your own projects. Remember that learning from open source is an ongoing process, and consistent engagement with different projects will continually broaden your expertise and keep you up-to-date with the latest trends and techniques in software development.
As you embark on this learning journey, keep in mind that every developer, regardless of their experience level, can benefit from studying open source projects. Whether you’re a beginner looking to understand basic coding patterns or an experienced developer seeking advanced architectural insights, there’s always something new to discover in the vast world of open source software.
Finally, don’t forget to give back to the open source community as you grow. By contributing your own improvements, fixing bugs, or helping others understand the code, you not only reinforce your own learning but also become part of the collaborative spirit that makes open source software so valuable. Happy coding and learning!