Strategies for Learning Coding Concepts Deeply, Not Just Memorizing
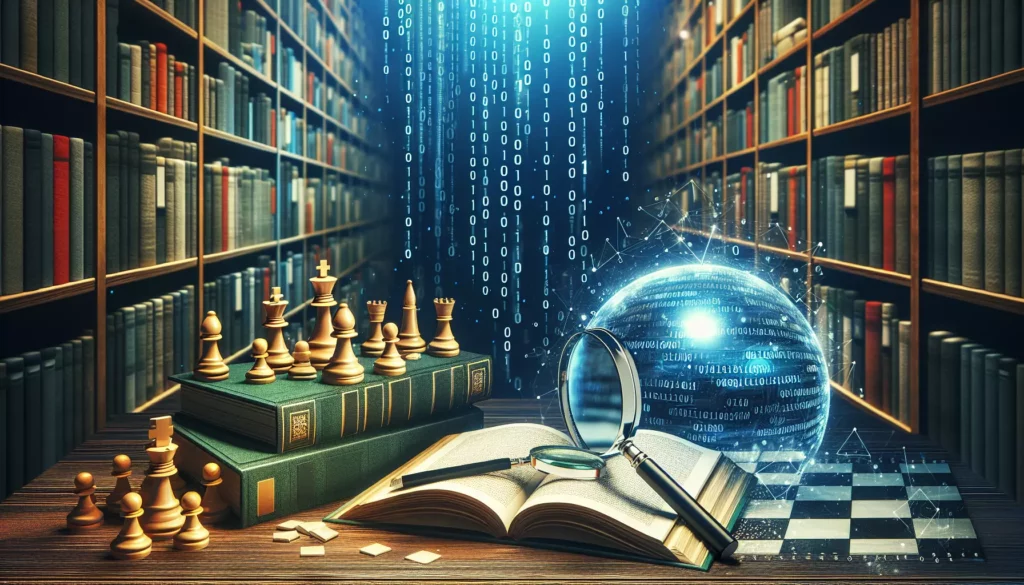
In the world of programming and software development, there’s a significant difference between truly understanding coding concepts and simply memorizing syntax or solutions. While memorization can help you pass a test or complete a specific task, deep learning of coding concepts is what separates great programmers from average ones. This article will explore various strategies to help you grasp coding concepts on a fundamental level, enabling you to apply your knowledge creatively and effectively across different programming challenges.
1. Understand the ‘Why’ Behind the Code
One of the most crucial aspects of learning coding deeply is understanding why certain approaches work and others don’t. Instead of just copying and pasting code snippets or memorizing solutions, take the time to analyze and comprehend the reasoning behind different coding practices.
Practice:
- When learning a new concept, ask yourself, “Why does this work?”
- Explore alternative solutions and compare their efficiency
- Read documentation and explanations from reliable sources
Example:
Consider the concept of using a hash table for efficient lookups. Don’t just memorize that hash tables are fast; understand why they provide O(1) average-case time complexity for insertions and lookups.
// Python example of a simple hash table implementation
class HashTable:
def __init__(self, size):
self.size = size
self.table = [[] for _ in range(self.size)]
def _hash(self, key):
return hash(key) % self.size
def insert(self, key, value):
hash_index = self._hash(key)
for item in self.table[hash_index]:
if item[0] == key:
item[1] = value
return
self.table[hash_index].append([key, value])
def get(self, key):
hash_index = self._hash(key)
for item in self.table[hash_index]:
if item[0] == key:
return item[1]
raise KeyError(key)
By understanding how the hash function works to distribute keys and how collisions are handled, you’ll gain a deeper appreciation for the efficiency of hash tables in various scenarios.
2. Build Projects from Scratch
One of the best ways to deeply understand coding concepts is to apply them in real-world projects. Building something from the ground up forces you to think through every aspect of the problem and how different components interact.
Practice:
- Start with small projects and gradually increase complexity
- Implement features without relying on external libraries at first
- Document your thought process and design decisions
Example Project: Building a Simple Web Server
Instead of using a framework like Flask or Express, try building a basic HTTP server from scratch using only the standard library of your chosen language. This will help you understand the fundamentals of how web servers work.
// JavaScript example of a simple HTTP server
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!');
});
server.listen(3000, 'localhost', () => {
console.log('Server running at http://localhost:3000/');
});
By implementing this yourself, you’ll gain insights into request handling, response formatting, and the HTTP protocol that you might miss when using a high-level framework.
3. Teach Others
Teaching is one of the most effective ways to solidify your understanding of a concept. When you explain something to someone else, you’re forced to break it down into simpler terms and often discover gaps in your own knowledge.
Practice:
- Start a blog or YouTube channel to share your learning journey
- Participate in coding forums and help answer questions
- Mentor junior developers or study groups
Example: Explaining Recursion
Try explaining the concept of recursion to a beginner programmer. You might start with a simple example like calculating factorial:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
# Example usage
print(factorial(5)) # Output: 120
As you explain this, you’ll likely find yourself delving into concepts like base cases, call stacks, and the importance of having a termination condition. This process of breaking down and articulating complex ideas will significantly enhance your own understanding.
4. Implement Data Structures and Algorithms from Scratch
While it’s important to know how to use built-in data structures and algorithms, implementing them from scratch provides a much deeper understanding of their inner workings and trade-offs.
Practice:
- Implement common data structures like linked lists, trees, and graphs
- Code classic algorithms such as sorting and searching algorithms
- Analyze the time and space complexity of your implementations
Example: Implementing a Binary Search Tree
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = Node(value)
else:
self._insert_recursive(node.right, value)
def search(self, value):
return self._search_recursive(self.root, value)
def _search_recursive(self, node, value):
if node is None or node.value == value:
return node
if value < node.value:
return self._search_recursive(node.left, value)
return self._search_recursive(node.right, value)
# Example usage
bst = BinarySearchTree()
bst.insert(5)
bst.insert(3)
bst.insert(7)
bst.insert(1)
bst.insert(9)
print(bst.search(7)) # Output: <Node object at ...>
print(bst.search(10)) # Output: None
By implementing a BST yourself, you’ll gain a deeper understanding of tree traversal, recursive algorithms, and the performance characteristics of tree-based data structures.
5. Analyze and Optimize Code
Understanding how to write efficient code is crucial for becoming a proficient programmer. Regularly analyzing and optimizing your code will help you develop a keen eye for performance issues and best practices.
Practice:
- Use profiling tools to identify bottlenecks in your code
- Experiment with different algorithms and data structures to solve the same problem
- Learn about time and space complexity analysis
Example: Optimizing a Fibonacci Function
Consider the following naive recursive implementation of the Fibonacci sequence:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
# Example usage
print(fibonacci(30)) # This will take a long time
This implementation has exponential time complexity. Let’s optimize it using dynamic programming:
def fibonacci_optimized(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
# Example usage
print(fibonacci_optimized(30)) # This will be much faster
By analyzing and optimizing this function, you’ll gain insights into the trade-offs between time and space complexity, as well as the principles of dynamic programming.
6. Read and Analyze Open Source Code
Reading well-written, production-grade code can provide valuable insights into best practices, design patterns, and advanced coding techniques.
Practice:
- Choose popular open-source projects in your preferred language or domain
- Start with smaller, well-documented projects and gradually move to larger ones
- Try to contribute to open-source projects by fixing bugs or adding features
Example: Analyzing Python’s Built-in Functions
Let’s look at the implementation of the built-in sum()
function in Python’s source code:
static PyObject *
sum(PyObject *self, PyObject *args, PyObject *kwds)
{
PyObject *seq;
PyObject *result = NULL;
PyObject *temp, *item, *iter;
static char *kwlist[] = {"iterable", "start", NULL};
if (!PyArg_ParseTupleAndKeywords(args, kwds, "O|O:sum", kwlist, &seq, &result))
return NULL;
iter = PyObject_GetIter(seq);
if (iter == NULL)
return NULL;
if (result == NULL) {
result = PyLong_FromLong(0);
if (result == NULL) {
Py_DECREF(iter);
return NULL;
}
} else {
Py_INCREF(result);
}
for (;;) {
item = PyIter_Next(iter);
if (item == NULL) {
if (PyErr_Occurred()) {
Py_DECREF(result);
result = NULL;
}
break;
}
temp = PyNumber_Add(result, item);
Py_DECREF(item);
if (temp == NULL) {
Py_DECREF(result);
result = NULL;
break;
}
Py_DECREF(result);
result = temp;
}
Py_DECREF(iter);
return result;
}
Analyzing this code can teach you about memory management, error handling, and how Python handles different numeric types internally.
7. Practice Explaining Complex Concepts Simply
The ability to explain complex coding concepts in simple terms is a hallmark of deep understanding. This skill not only helps you communicate effectively with others but also reinforces your own grasp of the subject.
Practice:
- Use analogies and real-world examples to explain technical concepts
- Break down complex ideas into smaller, more manageable parts
- Create visual aids or diagrams to illustrate concepts
Example: Explaining Big O Notation
Try explaining Big O notation using a simple analogy:
“Imagine you’re baking cookies. O(1) is like having a recipe that always takes the same amount of time, regardless of how many cookies you’re making. O(n) is like a recipe where the time increases linearly with the number of cookies – doubling the cookies doubles the time. O(n²) is like a recipe where for each cookie, you need to compare it with every other cookie – making a lot of cookies takes a really long time.”
By simplifying complex concepts like this, you’re forced to distill the core ideas, which deepens your own understanding.
8. Explore Different Programming Paradigms
Understanding different programming paradigms can broaden your perspective and help you approach problems from multiple angles.
Practice:
- Learn and practice object-oriented, functional, and procedural programming
- Implement the same problem using different paradigms
- Study the strengths and weaknesses of each approach
Example: Implementing a Counter in Different Paradigms
Procedural:
counter = 0
def increment():
global counter
counter += 1
def get_count():
return counter
# Usage
increment()
increment()
print(get_count()) # Output: 2
Object-Oriented:
class Counter:
def __init__(self):
self._count = 0
def increment(self):
self._count += 1
def get_count(self):
return self._count
# Usage
counter = Counter()
counter.increment()
counter.increment()
print(counter.get_count()) # Output: 2
Functional (using closure):
def create_counter():
count = 0
def increment():
nonlocal count
count += 1
return count
return increment
# Usage
counter = create_counter()
print(counter()) # Output: 1
print(counter()) # Output: 2
By implementing the same functionality in different paradigms, you’ll gain insights into the trade-offs and design principles of each approach.
9. Engage in Code Reviews
Participating in code reviews, both as a reviewer and as someone whose code is being reviewed, can significantly enhance your understanding of coding best practices and common pitfalls.
Practice:
- Offer to review your peers’ code and welcome reviews of your own code
- Focus on readability, maintainability, and efficiency in your reviews
- Learn to give and receive constructive feedback
Example: Code Review Checklist
When reviewing code, consider the following aspects:
- Correctness: Does the code do what it’s supposed to do?
- Efficiency: Are there any performance bottlenecks?
- Readability: Is the code easy to understand?
- Maintainability: How easy would it be to modify or extend this code?
- Error handling: Are edge cases and potential errors properly handled?
- Testing: Are there adequate unit tests?
- Documentation: Is the code well-commented and documented?
By consistently applying these criteria in code reviews, you’ll develop a keen eye for quality code and deepen your understanding of software engineering principles.
10. Solve Programming Puzzles and Challenges
Engaging with programming puzzles and challenges can help you apply your knowledge in creative ways and expose you to a wide variety of problem-solving techniques.
Practice:
- Participate in coding competitions on platforms like LeetCode, HackerRank, or CodeForces
- Solve daily coding challenges to build consistency
- Analyze and learn from others’ solutions after solving a problem
Example: Solving the “Two Sum” Problem
Let’s look at the classic “Two Sum” problem and explore different approaches to solve it:
def two_sum_brute_force(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return []
def two_sum_optimized(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Example usage
nums = [2, 7, 11, 15]
target = 9
print(two_sum_brute_force(nums, target)) # Output: [0, 1]
print(two_sum_optimized(nums, target)) # Output: [0, 1]
By solving problems like this and exploring different solutions, you’ll develop a repertoire of problem-solving strategies and gain a deeper understanding of algorithm design and optimization.
Conclusion
Learning coding concepts deeply requires a multifaceted approach that goes beyond mere memorization. By understanding the ‘why’ behind the code, building projects from scratch, teaching others, implementing core data structures and algorithms, analyzing and optimizing code, reading open-source projects, practicing clear explanations, exploring different programming paradigms, engaging in code reviews, and solving programming challenges, you can develop a profound understanding of programming principles.
Remember that deep learning is an ongoing process. As you apply these strategies, you’ll not only become a more skilled programmer but also develop the ability to adapt to new technologies and solve complex problems creatively. Embrace the journey of continuous learning and improvement, and you’ll find that your capacity for understanding and applying coding concepts will grow exponentially over time.