Strategies for Handling Algorithm Edge Cases: Mastering the Art of Robust Code
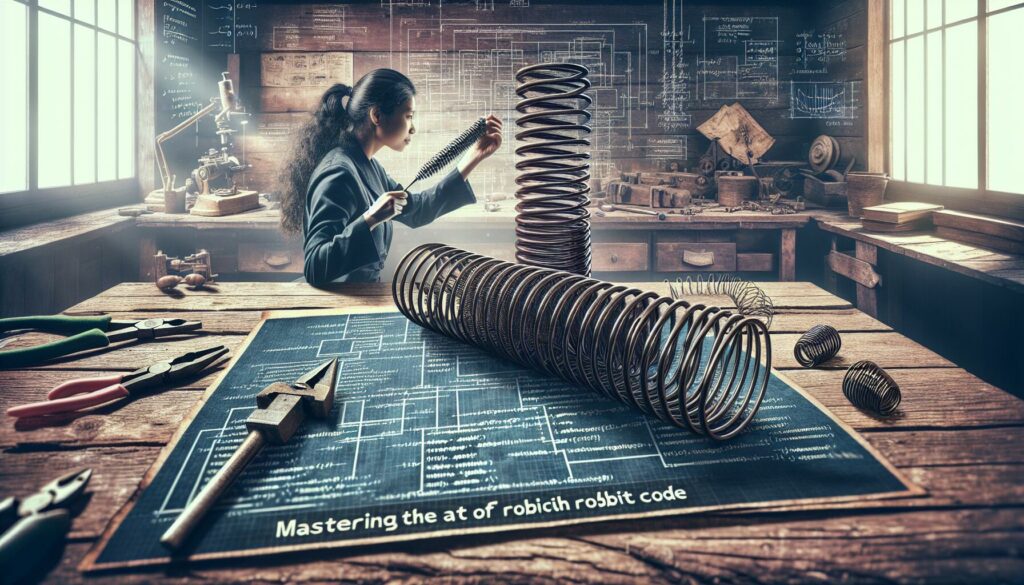
In the world of programming and algorithm design, edge cases are the bane of many developers’ existence. These sneaky scenarios that fall outside the normal operating parameters of an algorithm can cause unexpected behavior, crashes, or incorrect results if not properly handled. As aspiring software engineers or seasoned professionals aiming to level up their skills, mastering the art of handling edge cases is crucial for writing robust, reliable code that can stand up to real-world use.
In this comprehensive guide, we’ll explore various strategies for identifying, addressing, and testing edge cases in your algorithms. We’ll cover best practices, common pitfalls, and provide practical examples to help you become a master of edge case handling. Whether you’re preparing for technical interviews at top tech companies or simply want to improve the quality of your code, this article will equip you with the knowledge and techniques you need to tackle even the trickiest edge cases with confidence.
Understanding Edge Cases
Before we dive into strategies for handling edge cases, let’s first establish a clear understanding of what they are and why they’re important.
What Are Edge Cases?
Edge cases are inputs or scenarios that fall at the extreme ends of the possible range of values or conditions for an algorithm. These are situations that may be rare or unexpected but can still occur in real-world usage. Some common examples of edge cases include:
- Empty inputs (e.g., an empty array or string)
- Inputs with a single element
- Maximum or minimum possible values
- Null or undefined inputs
- Inputs with special characters or unusual formats
- Boundary conditions (e.g., the first or last element of a list)
Why Are Edge Cases Important?
Handling edge cases is crucial for several reasons:
- Robustness: Properly handling edge cases makes your code more resilient and less prone to crashes or unexpected behavior.
- Correctness: Ensuring your algorithm works correctly for all possible inputs, including edge cases, is essential for maintaining the integrity of your software.
- User Experience: Gracefully handling edge cases can improve the user experience by preventing errors and providing appropriate feedback.
- Debugging: Identifying and addressing edge cases during development can save time and effort in debugging later on.
- Interview Success: Many technical interviews, especially at top tech companies, focus on how well candidates handle edge cases in their solutions.
Strategies for Identifying Edge Cases
The first step in handling edge cases is identifying them. Here are some strategies to help you uncover potential edge cases in your algorithms:
1. Analyze Input Ranges
Consider the full range of possible inputs for your algorithm. Think about:
- Minimum and maximum values
- Empty or null inputs
- Single-element inputs
- Inputs at the boundaries of different categories or types
2. Consider Special Values
Look for special values that might cause unexpected behavior:
- Zero
- Negative numbers (if dealing with typically positive values)
- Infinity or very large numbers
- NaN (Not a Number) for floating-point operations
3. Examine Data Types
Think about different data types that could be input into your algorithm:
- Strings vs. numbers
- Integers vs. floating-point numbers
- Objects vs. primitives
- Different array types (e.g., sorted vs. unsorted)
4. Consider Environmental Factors
Don’t forget about external factors that could affect your algorithm:
- Concurrency issues
- Resource limitations (e.g., memory constraints)
- Network connectivity problems
- Platform-specific differences
5. Use the “What If” Technique
Ask yourself “What if…?” questions to uncover potential edge cases:
- What if the input is empty?
- What if all elements are the same?
- What if the input is already sorted (for sorting algorithms)?
- What if the input contains duplicate elements?
Strategies for Handling Edge Cases
Once you’ve identified potential edge cases, it’s time to implement strategies to handle them effectively. Here are some approaches you can use:
1. Input Validation
One of the most fundamental strategies for handling edge cases is to validate your inputs before processing them. This can help catch and handle unexpected inputs early, preventing errors from propagating through your algorithm.
Example in Python:
def calculate_average(numbers):
if not numbers:
return 0 # Handle empty list
if not all(isinstance(num, (int, float)) for num in numbers):
raise ValueError("All elements must be numbers")
return sum(numbers) / len(numbers)
2. Defensive Programming
Defensive programming involves anticipating and handling potential issues before they occur. This can include checking for null values, handling exceptions, and providing default values or fallback behavior.
Example in Java:
public String processName(String name) {
if (name == null || name.isEmpty()) {
return "Unknown"; // Provide a default value
}
return name.trim().toLowerCase();
}
3. Boundary Checking
When dealing with algorithms that involve ranges or indices, always check that you’re operating within the valid boundaries. This can prevent index out of bounds errors and other related issues.
Example in C++:
int getElementAtIndex(const vector<int>& arr, int index) {
if (index < 0 || index >= arr.size()) {
throw out_of_range("Index out of bounds");
}
return arr[index];
}
4. Type Checking and Conversion
In languages with dynamic typing or when dealing with user input, it’s important to check and convert types as necessary to ensure your algorithm works with the expected data types.
Example in JavaScript:
function add(a, b) {
if (typeof a !== 'number' || typeof b !== 'number') {
throw new TypeError("Both arguments must be numbers");
}
return a + b;
}
5. Error Handling and Exceptions
Implement proper error handling and use exceptions (where appropriate) to deal with edge cases that cannot be handled normally. This allows you to gracefully manage unexpected situations and provide meaningful feedback.
Example in Python:
def divide(a, b):
try:
return a / b
except ZeroDivisionError:
raise ValueError("Cannot divide by zero")
except TypeError:
raise TypeError("Both arguments must be numbers")
6. Default Values and Fallback Behavior
Provide sensible default values or fallback behavior for edge cases where appropriate. This can help your algorithm continue functioning even when faced with unexpected inputs.
Example in JavaScript:
function greet(name = "Guest") {
return `Hello, ${name}!`;
}
7. Modular Design
Break your algorithm into smaller, modular functions that each handle a specific task. This can make it easier to identify and handle edge cases for each component separately.
Example in Python:
def process_data(data):
validated_data = validate_input(data)
cleaned_data = clean_data(validated_data)
return analyze_data(cleaned_data)
def validate_input(data):
# Handle input validation edge cases
pass
def clean_data(data):
# Handle data cleaning edge cases
pass
def analyze_data(data):
# Handle analysis edge cases
pass
Testing Strategies for Edge Cases
Identifying and implementing strategies for handling edge cases is only part of the battle. It’s equally important to thoroughly test your algorithm to ensure it behaves correctly in all scenarios. Here are some testing strategies specifically focused on edge cases:
1. Unit Testing
Write comprehensive unit tests that specifically target edge cases. This includes testing with minimum and maximum values, empty inputs, single-element inputs, and other boundary conditions.
Example using Python’s unittest framework:
import unittest
class TestCalculateAverage(unittest.TestCase):
def test_empty_list(self):
self.assertEqual(calculate_average([]), 0)
def test_single_element(self):
self.assertEqual(calculate_average([5]), 5)
def test_negative_numbers(self):
self.assertEqual(calculate_average([-1, -2, -3]), -2)
def test_large_numbers(self):
self.assertEqual(calculate_average([1e100, 2e100, 3e100]), 2e100)
def test_mixed_types(self):
with self.assertRaises(ValueError):
calculate_average([1, 2, "3"])
if __name__ == '__main__':
unittest.main()
2. Property-Based Testing
Use property-based testing frameworks to generate a wide range of inputs, including edge cases, and verify that your algorithm maintains certain properties or invariants.
Example using Python’s hypothesis library:
from hypothesis import given, strategies as st
@given(st.lists(st.integers()))
def test_sort_idempotent(lst):
sorted_once = sorted(lst)
sorted_twice = sorted(sorted_once)
assert sorted_once == sorted_twice
3. Fuzz Testing
Employ fuzz testing techniques to generate random, unexpected, or malformed inputs to stress-test your algorithm and uncover potential edge cases you might have missed.
4. Boundary Value Analysis
Systematically test values at and around the boundaries of input ranges. This includes testing with values just below, at, and just above the minimum and maximum allowed values.
5. Error Injection
Deliberately introduce errors or unexpected conditions in your testing environment to simulate edge cases that might occur in real-world scenarios (e.g., network failures, out-of-memory conditions).
6. Code Review
Conduct thorough code reviews with a specific focus on edge case handling. Fresh eyes can often spot potential issues or overlooked scenarios.
7. Continuous Integration and Regression Testing
Implement a robust CI/CD pipeline that includes automated tests for edge cases. This helps catch any regressions that might be introduced as the codebase evolves.
Common Pitfalls in Edge Case Handling
Even with the best intentions, developers can fall into certain traps when dealing with edge cases. Here are some common pitfalls to watch out for:
1. Assuming “Normal” Input
One of the most common mistakes is assuming that your algorithm will always receive “normal” or expected input. Always validate and handle unexpected inputs.
2. Ignoring Performance Impact
Sometimes, handling edge cases can introduce significant performance overhead. Be mindful of the trade-offs between robustness and efficiency.
3. Over-Engineering
While it’s important to handle edge cases, be careful not to over-engineer your solution. Focus on realistic scenarios and avoid trying to handle every conceivable edge case at the expense of code clarity.
4. Inconsistent Error Handling
Ensure that your error handling is consistent throughout your codebase. Use similar patterns and error messages for similar types of edge cases.
5. Neglecting Documentation
Document the edge cases your algorithm handles (and doesn’t handle) in your code comments and API documentation. This helps users and other developers understand the limitations and expected behavior of your code.
6. Failing to Update Edge Case Handling
As your algorithm evolves, new edge cases may emerge. Regularly review and update your edge case handling strategies as part of your maintenance process.
Real-World Examples of Edge Case Handling
To illustrate the importance of proper edge case handling, let’s look at some real-world examples where edge cases have caused significant issues:
1. The Ariane 5 Rocket Explosion
In 1996, the Ariane 5 rocket exploded just 40 seconds after launch due to a software error. The root cause was a conversion of a 64-bit floating-point number to a 16-bit signed integer, which caused an overflow when the rocket’s horizontal velocity exceeded the maximum 16-bit value. This edge case was not properly handled, leading to a catastrophic failure.
2. The Y2K Bug
The Y2K bug is a classic example of an edge case that was not initially considered. Many early computer systems represented years with two digits, assuming the century would always be “19”. As the year 2000 approached, there were concerns about how these systems would handle the transition to a new century.
3. Integer Overflow in Boeing 787 Dreamliner
In 2015, it was discovered that a software bug in the Boeing 787 Dreamliner could cause all electrical power to be lost if the aircraft remained powered continuously for 248 days. This was due to an integer overflow in the generator control units, where a counter would eventually reach its maximum value and roll over to zero.
4. The Therac-25 Radiation Therapy Machine
The Therac-25 was a radiation therapy machine that caused several accidents, delivering lethal radiation doses to patients. One of the issues was a race condition that could occur if the operator quickly changed the machine’s settings. This edge case in the software’s timing and synchronization led to tragic consequences.
Conclusion
Handling algorithm edge cases is a critical skill for any software developer. By mastering the strategies outlined in this article, you’ll be better equipped to write robust, reliable code that can handle even the most unexpected inputs and scenarios. Remember that edge case handling is not just about preventing errors; it’s about creating software that is trustworthy, user-friendly, and capable of standing up to the complexities of real-world use.
As you continue to develop your skills, make edge case analysis and handling an integral part of your development process. Practice identifying potential edge cases, implement appropriate handling strategies, and rigorously test your code to ensure it behaves correctly in all situations. By doing so, you’ll not only improve the quality of your code but also set yourself apart as a thoughtful and thorough developer – qualities that are highly valued in technical interviews and professional settings alike.
Whether you’re preparing for a coding interview at a top tech company or working on a critical software project, the ability to effectively handle edge cases will serve you well throughout your career. Embrace the challenge of edge cases, and use them as opportunities to showcase your problem-solving skills and attention to detail. With practice and diligence, you’ll be well on your way to mastering the art of robust algorithm design.